多线程示例图
下图为多线程运行示例图,在输出界面回发现各个线程ID不相同,如次的话则线程创建运行成功 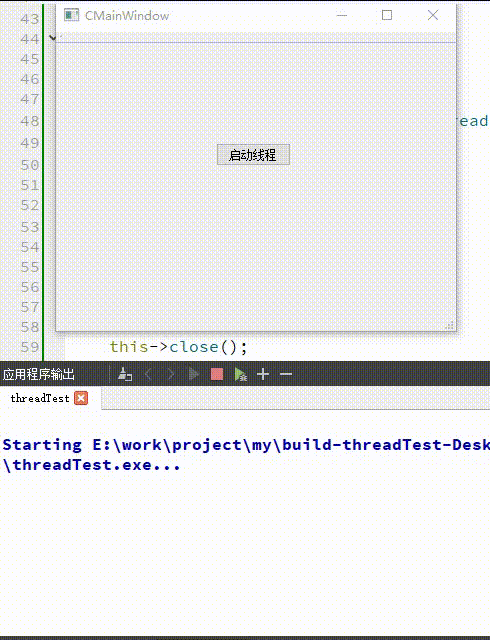
启动QThread的两种方法
- 通过继承QThread类的自定义线程类实现多线程
- 通过moveToThread方法实现多线程
废话少说上源码
方法一:
CThread.h
#ifndef CTHREAD_H
#define CTHREAD_H
#include <QObject>
#include <QThread>
class CThread : public QThread
{
Q_OBJECT
public:
explicit CThread(QObject *parent = nullptr);
void run();
};
#endif
CThread.cpp
#include "CThread.h"
#include <QDebug>
CThread::CThread(QObject *parent)
: QThread(parent)
{
}
void CThread::run()
{
while (true)
{
qDebug() << QThread::currentThreadId() << "我是线程ID, 继承线程类方法实现的";
QThread::sleep(1);
}
}
方法二
CMoveFuncClass.h
#ifndef CMOVEFUNCCLASS_H
#define CMOVEFUNCCLASS_H
#include <QObject>
class CMoveFuncClass : public QObject
{
Q_OBJECT
public:
explicit CMoveFuncClass(QObject *parent = nullptr);
public slots:
void doSomething();
};
#endif
CMoveFuncClass.cpp
#include "CMoveFuncClass.h"
#include <QDebug>
#include <QThread>
CMoveFuncClass::CMoveFuncClass(QObject *parent)
: QObject(parent)
{
}
void CMoveFuncClass::doSomething()
{
while (true)
{
qDebug() << QThread::currentThreadId() << "我是线程ID, moveToThread方法实现的";
QThread::sleep(1);
}
}
调用类
CMainWindow.h
#ifndef CMAINWINDOW_H
#define CMAINWINDOW_H
#include <QMainWindow>
#include "CThread.h"
#include "CMoveFuncClass.h"
namespace Ui {
class CMainWindow;
}
class CMainWindow : public QMainWindow
{
Q_OBJECT
public:
explicit CMainWindow(QWidget *parent = 0);
~CMainWindow();
signals:
void startMoveThread();
private slots:
void on_startBtn_clicked();
private:
Ui::CMainWindow *ui;
CThread *m_cThread;
CMoveFuncClass *m_moveFunc;
QThread *m_thread;
};
#endif
CMainWindow.cpp
#include "CMainWindow.h"
#include "ui_CMainWindow.h"
#include <QDebug>
CMainWindow::CMainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::CMainWindow)
{
ui->setupUi(this);
m_cThread = new CThread;
m_moveFunc = new CMoveFuncClass;
m_thread = new QThread;
m_thread->start();
connect(this, &CMainWindow::startMoveThread, m_moveFunc, &CMoveFuncClass::doSomething);
connect(m_thread, &QThread::finished, m_moveFunc, &QObject::deleteLater);
m_moveFunc->moveToThread(m_thread);
}
CMainWindow::~CMainWindow()
{
delete m_cThread;
delete m_moveFunc;
delete m_thread;
delete ui;
}
void CMainWindow::on_startBtn_clicked()
{
m_cThread->start();
emit startMoveThread();
int flag = 0;
while(++flag != 5)
{
qDebug() << flag;
qDebug() << QThread::currentThreadId() << "我是主类ID,创建和调用线程类的";
QThread::sleep(1);
}
m_cThread->quit();
m_cThread->wait(1);
m_thread->quit();
m_thread->wait(1);
this->close();
}
注意:QThread::sleep在主线程使用时会导致主线程停止,如果设置的时间太长系统会当作是程序卡死,的情况,所以在主线程要么不使用sleep,要么在sleep时不操作程序窗口
总结
线程在很多时候是很有用的,当需要减少主线程负担时可以使用,需要后台重写某文件等情况可以使用,并且在线程运行时还不会影响主线程。当然了使用线程也得小心,如内存释放等问题,以及线程数量限制的问题(这里可以去了解线程池的用法)。
注:本文仅为作者编程过程中所遇到的问题和总结,并非代表绝对正确,若有错误欢迎指出。 注:如有侵权,联系作者删除
|