字符串常用操作有分割取子串,取分割长度,分割得到数组,替换,去除两边空格,查找等。本次实现替换和剔除空格方法。
头文件
#pragma onece
#include <iostream>
#include <cstring>
#include <vector>
using namespace std;
void dolertrimleft(string &retStr,string source);
void dolertrimright(string &retStr,string source);
void dolertrim(string &retStr,string source);
void dolerreplace(string &retStr,string source,string orgStr,string repStr);
void dolerp(string &retStr,string source,string split,int index);
void dolersplit(vector<string> &retvec,string source,string split);
int dolerl(string source,string split);
实现cpp
#include "mutil.h"
void dolertrimleft(string &retStr,string source)
{
string retStrTmp="";
if(source=="")
{
retStr=retStrTmp;
return;
}
int sourceLen=source.length();
int startIndex=-1;
for(int i=0;i<sourceLen;i++)
{
char one=source[i];
if(one!=' ')
{
startIndex=i;
break;
}
}
if(startIndex==-1)
{
retStr=retStrTmp;
return;
}
retStrTmp=source.substr(startIndex,sourceLen-startIndex);
retStr=retStrTmp;
}
void dolertrimright(string &retStr,string source)
{
string retStrTmp="";
if(source=="")
{
retStr=retStrTmp;
return;
}
int sourceLen=source.length();
int endIndex=-1;
for(int i=sourceLen-1;i>=0;i--)
{
char one=source[i];
if(one!=' ')
{
endIndex=i;
break;
}
}
if(endIndex==-1)
{
retStr=retStrTmp;
return;
}
retStrTmp=source.substr(0,endIndex+1);
retStr=retStrTmp;
}
void dolertrim(string &retStr,string source)
{
string tmpstr;
dolertrimleft(tmpstr,source);
dolertrimright(retStr,tmpstr);
}
void dolerreplace(string &retStr,string source,string orgStr,string repStr)
{
string retStrTmp="";
if(source=="")
{
retStr=retStrTmp;
return;
}
if(orgStr=="")
{
retStrTmp=source;
retStr=retStrTmp;
return;
}
int sourceLen=source.length();
int orgLen=orgStr.length();
int curIndex=0;
int startIndex=0;
for(int i=0;i<sourceLen;i++)
{
if(i>=orgLen&&source[i]==orgStr[orgLen-1])
{
bool isCommon=true;
for(int j=1;j<orgLen;j++)
{
if(source[i-j]!=orgStr[orgLen-1-j])
{
isCommon=false;
break;
}
}
if(isCommon==true)
{
curIndex++;
int len=i-orgLen-startIndex+1;
if(curIndex>1)
{
retStrTmp+=repStr+source.substr(startIndex,len);
}
else
{
retStrTmp=source.substr(startIndex,len);
}
startIndex=i+1;
i+=orgLen;
}
}
}
if(startIndex<sourceLen)
{
if(curIndex>1)
{
retStrTmp+=repStr+source.substr(startIndex,sourceLen-startIndex);
}
else
{
retStrTmp=source.substr(startIndex,sourceLen-startIndex);
}
}
retStr=retStrTmp;
}
void dolerp(string &retStr,string source,string split,int index)
{
string retStrTmp="";
if(source=="")
{
retStr=retStrTmp;
return;
}
if(split=="")
{
retStr=retStrTmp;
return;
}
if(index<1)
{
retStr=retStrTmp;
return;
}
int sourceLen=source.length();
if(sourceLen==0)
{
retStr=retStrTmp;
return;
}
int spLen=split.length();
if(spLen==0)
{
retStr=retStrTmp;
return;
}
int curIndex=0;
int startIndex=0;
int endIndex=0;
for(int i=0;i<sourceLen;i++)
{
if(i>=spLen&&source[i]==split[spLen-1])
{
bool isCommon=true;
for(int j=1;j<spLen;j++)
{
if(source[i-j]!=split[spLen-1-j])
{
isCommon=false;
break;
}
}
if(isCommon==true)
{
curIndex++;
if(curIndex==index)
{
endIndex=i-spLen;
break;
}
if(curIndex==index-1)
{
startIndex=i+1;
}
i+=spLen;
}
}
}
if(curIndex==0&&index==1)
{
retStrTmp=source;
retStr=retStrTmp;
return;
}
else if(curIndex<index-1)
{
retStr=retStrTmp;
return;
}
else
{
if(startIndex>endIndex)
{
endIndex=sourceLen-1;
}
int retArrLen=endIndex-startIndex+1;
char retArr[retArrLen];
for(int k=startIndex;k<=endIndex;k++)
{
retStrTmp+=source[k];
}
retStr=retStrTmp;
return;
}
}
int dolerl(string source,string split)
{
int ret=1;
if(source=="")
{
return ret;
}
if(split=="")
{
return ret;
}
int sourceLen=source.length();
if(sourceLen==0)
{
return ret;
}
int spLen=split.length();
if(spLen==0)
{
return ret;
}
int curIndex=0;
int startIndex=0;
int endIndex=0;
for(int i=0;i<sourceLen;i++)
{
if(i>=spLen&&source[i]==split[spLen-1])
{
bool isCommon=true;
for(int j=1;j<spLen;j++)
{
if(source[i-j]!=split[spLen-1-j])
{
isCommon=false;
break;
}
}
if(isCommon==true)
{
ret++;
i+=spLen;
}
}
}
return ret;
}
void dolersplit(vector<string> &retvec,string source,string split)
{
retvec.clear();
if(source=="")
{
return;
}
if(split=="")
{
return;
}
int sourceLen=source.length();
if(sourceLen==0)
{
return;
}
int spLen=split.length();
if(spLen==0)
{
return;
}
int curIndex=0;
int startIndex=0;
int endIndex=0;
for(int i=0;i<sourceLen;i++)
{
if(i>=spLen&&source[i]==split[spLen-1])
{
bool isCommon=true;
for(int j=1;j<spLen;j++)
{
if(source[i-j]!=split[spLen-1-j])
{
isCommon=false;
break;
}
}
if(isCommon==true)
{
int len=i-spLen-startIndex+1;
retvec.push_back(source.substr(startIndex,len));
startIndex=i;
i+=spLen;
}
}
}
if(startIndex<sourceLen)
{
retvec.push_back(source.substr(startIndex,sourceLen-startIndex));
}
}
测试cpp
#include "mutil.h"
int main(int argc, char* argv[])
{
string all="张三^29岁^男^433127199101211234";
string sp="^";
string ret;
cout<<endl;
cout<<"测试分割取子串*********************"<<endl;
dolerp(ret,all,sp,1);
cout<<"第一位为:"<<ret<<endl;
dolerp(ret,all,sp,2);
cout<<"第二位为:"<<ret<<endl;
dolerp(ret,all,sp,3);
cout<<"第三位为:"<<ret<<endl;
dolerp(ret,all,sp,4);
cout<<"第四位为:"<<ret<<endl;
dolerp(ret,all,"29",2);
cout<<"29分割第二位为:"<<ret<<endl;
dolerp(ret,all,"298",2);
cout<<"298分割第二位为:"<<ret<<endl;
dolerp(ret,all,"433",1);
cout<<"433分割第一位为:"<<ret<<endl;
cout<<endl;
cout<<"测试分割向量*********************"<<endl;
vector<string> vecarr;
dolersplit(vecarr,all,sp);
for(int i=0;i<vecarr.size();i++)
{
cout<<"数组第"<<i<<"位为:"<<vecarr[i]<<endl;
}
cout<<endl;
cout<<"测试分割得到长度*********************"<<endl;
int len=dolerl(all,sp);
cout<<"长度为:"<<len<<endl;
for(int i=1;i<=len;i++)
{
string childStr;
dolerp(childStr,all,sp,i);
cout<<"取第"<<i<<"个字串:"<<childStr<<endl;
}
cout<<endl;
cout<<"测试替换字符串*********************"<<endl;
string allrepstr="张三^29岁^男^433127199101211234";
string repret;
dolerreplace(repret,allrepstr,"^","$@$");
cout<<allrepstr<<",替换:^"<<"为:$@$得到:"<<repret<<endl;
string repret1;
repret="张三$@$29岁$@$男$@$433127199101211234";
dolerreplace(repret1,repret,"$@$","%ZSP%");
cout<<repret<<",替换:$@$"<<"为:%ZSP%得到:"<<repret1<<endl;
cout<<endl;
cout<<"测试剔除空格*********************"<<endl;
string hasSpaceStr=" 张三 男 29岁 ";
string trimRet;
dolertrimleft(trimRet,hasSpaceStr);
cout<<"去除左空格:"<<trimRet<<endl;
dolertrimright(trimRet,hasSpaceStr);
cout<<"去除右空格:"<<trimRet<<endl;
dolertrim(trimRet,hasSpaceStr);
cout<<"去除两边空格:"<<trimRet<<endl;
return 0;
}
cmake
cmake_minimum_required (VERSION 3.8)
project ("mutil")
add_executable (mutil "mutil.cpp" "mutil.h" "main.cpp")
测试效果
[zhanglianzhu@zlzlinux mutil]$
[zhanglianzhu@zlzlinux mutil]$ make
Consolidate compiler generated dependencies of target mutil
[ 33%] Building CXX object CMakeFiles/mutil.dir/main.cpp.o
[ 66%] Linking CXX executable mutil
[100%] Built target mutil
[zhanglianzhu@zlzlinux mutil]$ ./mutil
测试分割取子串*********************
第一位为:张三
第二位为:29岁
第三位为:男
第四位为:433127199101211234
29分割第二位为:岁^男^433127199101211234
298分割第二位为:
433分割第一位为:张三^29岁^男^
测试分割向量*********************
数组第0位为:张三
数组第1位为:^29岁
数组第2位为:^男
数组第3位为:^433127199101211234
测试分割得到长度*********************
长度为:4
取第1个字串:张三
取第2个字串:29岁
取第3个字串:男
取第4个字串:433127199101211234
测试替换字符串*********************
张三^29岁^男^433127199101211234,替换:^为:$@$得到:张三$@$29岁$@$男$@$433127199101211234
张三$@$29岁$@$男$@$433127199101211234,替换:$@$为:%ZSP%得到:张三%ZSP%29岁%ZSP%男%ZSP%433127199101211234
测试剔除空格*********************
去除左空格:张三 男 29岁
去除右空格: 张三 男 29岁
去除两边空格:张三 男 29岁
[zhanglianzhu@zlzlinux mutil]$
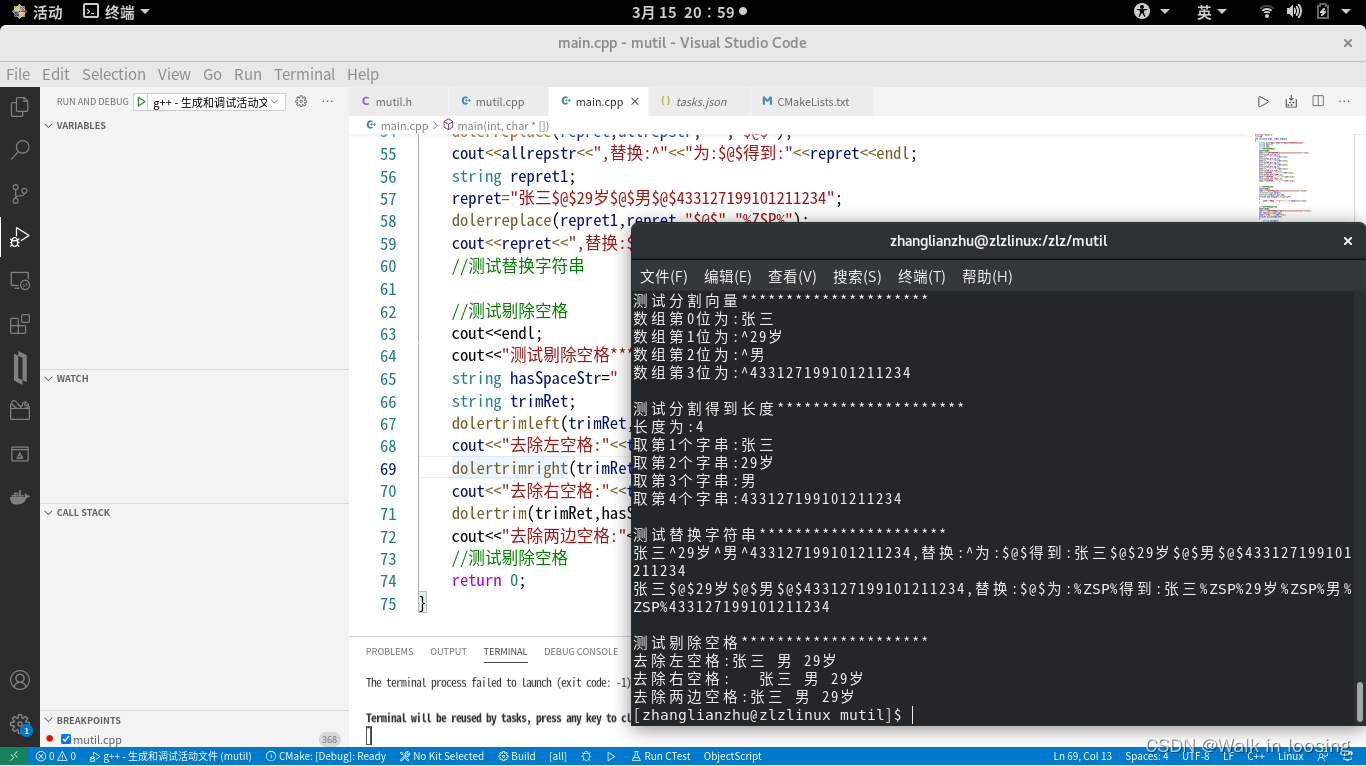
抽空逐渐把这个包装成好用的函数库。
|