 最下面的黑色节点是哨兵节点 通常面试的时候都不给出头节点,有头节点会使红黑树变的更加容易 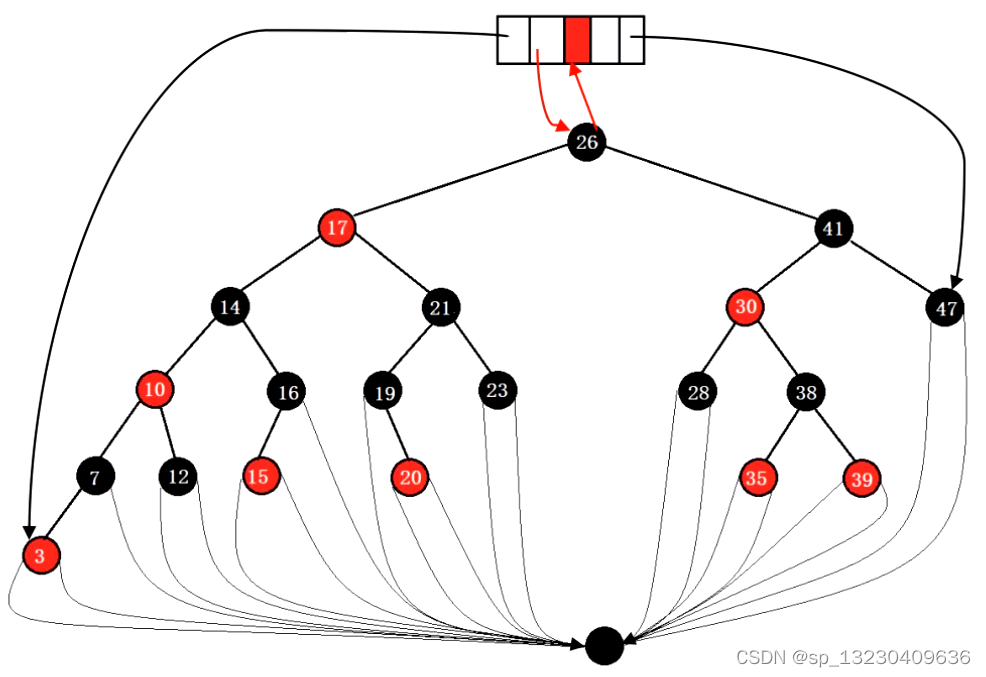 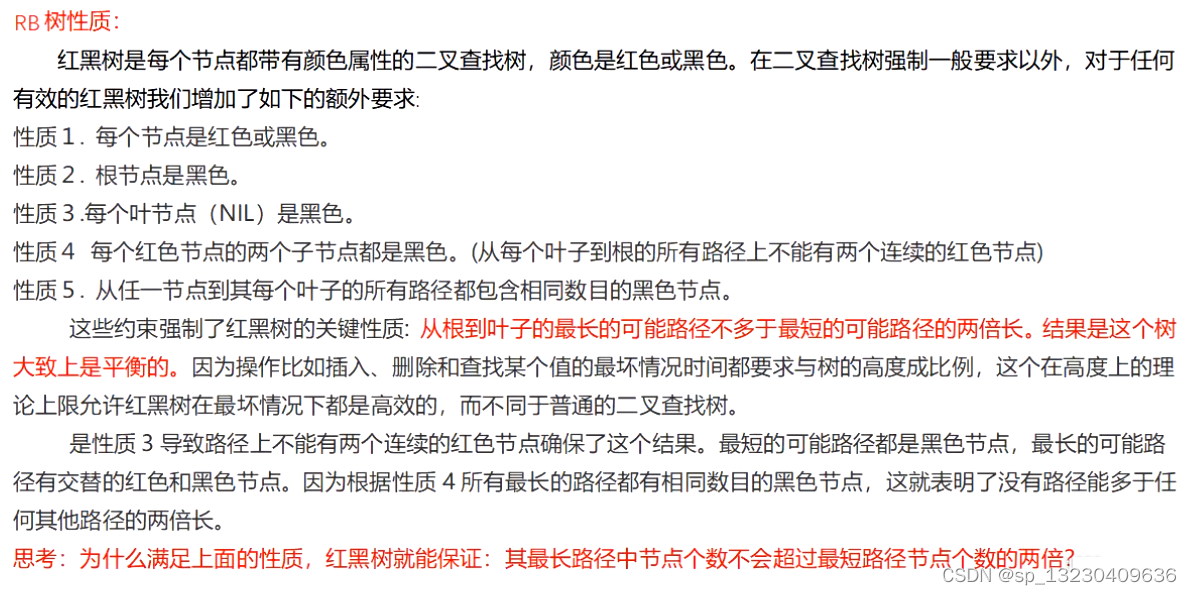 红黑树能不能给出三种颜色?就相当于是AVL树,AVL树是三种状态
RB树只要求是大致平衡的,不想AVL树是完全平衡的
下面两个图是等价的,所有路径都包含相同数目的黑色节点 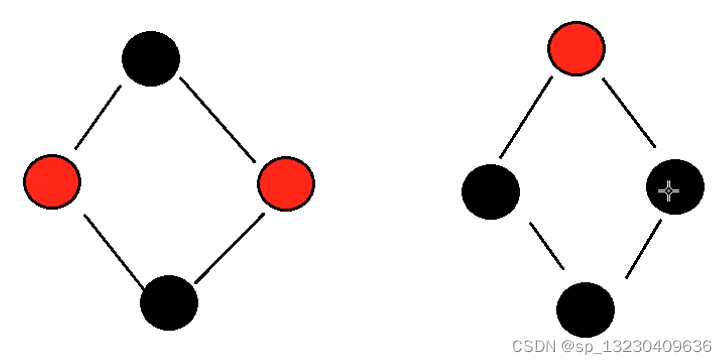 每次新建节点的默认颜色都是红色
typedef enum {RED = 0,BLACK = 1}ColorType;
typedef int KeyType;
typedef struct rb_node
{
rb_node* leftchild;
rb_node* parent;
rb_node* rightchild;
ColorType color;
KeyType key;
}rb_node,*RBTree;
rb_node* BuyNode();
static rb_node* Nil = BuyNode();
rb_node* BuyNode()
{
rb_node* s = (rb_node*)malloc(sizeof(rb_node));
if (nullptr == s) exit(1);
memset(s, 0, sizeof(rb_node));
s->leftchild = Nil;
s->rightchild = Nil;
s->color = RED;
return s;
}
rb_node* MakeRoot(KeyType kx)
{
rb_node* root = BuyNode();
root->color = BLACK;
root->key = kx;
return root;
}
void RotateLeft(rb_node*& tree, rb_node* ptr)
{
rb_node* newroot = ptr->rightchild;
newroot->parent = ptr->parent;
ptr->rightchild = newroot->leftchild;
if (newroot->leftchild != nullptr)
{
newroot->leftchild->parent = ptr;
}
newroot->leftchild = ptr;
if (ptr == tree)
{
tree = newroot;
}
else
{
if (ptr->parent->leftchild == ptr)
{
ptr->parent->leftchild = newroot;
}
else
{
ptr->parent->rightchild = newroot;
}
}
ptr->parent = newroot;
}
void RotateRight(rb_node*& tree, rb_node* ptr)
{
rb_node* newroot = ptr->leftchild;
newroot->parent = ptr->parent;
ptr->leftchild = newroot->rightchild;
if (newroot->rightchild != nullptr)
{
newroot->rightchild->parent = ptr;
}
newroot->rightchild = ptr;
if (ptr == tree)
{
tree = newroot;
}
else
{
if (ptr->parent->leftchild == ptr)
{
ptr->parent->leftchild = newroot;
}
else
{
ptr->parent->rightchild = newroot;
}
}
ptr->parent = newroot;
}
void PassRBTree(rb_node*& tree, rb_node* p)
{
rb_node* _X = nullptr;
for (; p != tree && p->parent->color == RED;)
{
if (p->parent->parent->rightchild == p->parent)
{
_X = p->parent->parent->leftchild;
if (_X->color == RED)
{
_X->color = BLACK;
p->parent->color = BLACK;
p->parent->parent->color = RED;
p = p->parent->parent;
}
else
{
if (p->parent->leftchild == p)
{
p = p->parent;
RotateRight(tree, p);
}
p->parent->color = BLACK;
p->parent->parent->color = RED;
RotateLeft(tree, p->parent->parent);
}
}
else
{
_X = p->parent->parent->rightchild;
if (_X->color == RED)
{
_X->color = BLACK;
p->parent->color = BLACK;
p->parent->parent->color = RED;
p = p->parent->parent;
}
else
{
if (p->parent->rightchild == p)
{
p = p->parent;
RotateRight(tree, p);
}
p->parent->color = BLACK;
p->parent->parent->color = RED;
RotateRight(tree, p->parent->parent);
}
}
}
tree->color = BLACK;
}
bool Insert(rb_node*& tree, KeyType kx)
{
if (tree == nullptr)
{
tree = MakeRoot(kx);
return true;
}
rb_node* pa = nullptr;
rb_node* p = tree;
while (p != nullptr && p->key != kx)
{
pa = p;
p = kx < p->key ? p->leftchild : p->rightchild;
}
if (p != nullptr && p->key == kx)return false;
p = BuyNode();
p->key = kx;
p->parent = pa;
if (kx < pa->key)
{
pa->leftchild = p;
}
else
{
pa->rightchild = p;
}
PassRBTree(tree, p);
return true;
}
int main()
{
int ar[] = { 16,3,7,11,9,26,18,14,15 };
AVLTree root = nullptr;
for (auto& x : ar)
{
cout << Insert(root, x) << endl;
}
InOrder(root);
cout << endl;
return 0;
}
|