系列文章目录
本次设计主要基于QT5.9平台设计了一款智能控制系统的上位机软件客户端,该软件可实现对电表、水表等仪器进行远程的数据读取与显示。为了保证客户的隐私,添加了用户登录等功能。具体效果如下: 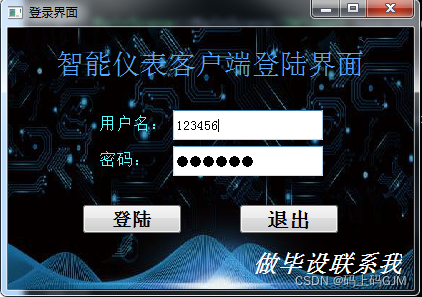
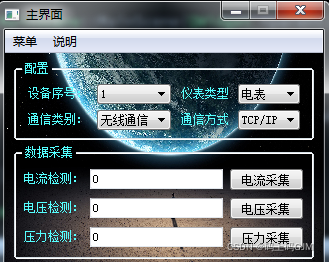
一、主程序代码
#include "workscene.h"
#include "ui_workscene.h"
#include <QDebug>
#include <QTime>
#include <QPainter>
WorkScene::WorkScene(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::WorkScene)
{
ui->setupUi(this);
currentTime = new QLabel(this);
time_cp = startTimer(1000);
time_updata_map = startTimer(10);
my_init_status();
ui->statusbar->setSizeGripEnabled(false);
myser = new MySerial(this);
connect(ui->ser_config,&QAction::triggered,[=](){
myser->show();
});
curr_equipment_num->setText(ui->box_equip_num->currentText());
this->setWindowTitle("主界面");
}
WorkScene::~WorkScene()
{
delete ui;
}
void WorkScene::timeUpdate()
{
QDateTime time_data = QDateTime::currentDateTime();
QString str = time_data.toString("yyyy/MM/dd hh:mm:ss");
currentTime->setText(str);
}
void WorkScene::mapUpdate()
{
calcData(Myvalue->get_ans_value());
ui->lineEdit_ans1->setText(QString::number(dianliu));
ui->lineEdit_ans2->setText(QString::number(dianya));
ui->lineEdit_ans3->setText(QString::number(yali));
curr_equipment_num->setText(ui->box_equip_num->currentText());
curr_equipment_type->setText(ui->box_equip_type->currentText());
}
void WorkScene::timerEvent(QTimerEvent * event)
{
if(event->timerId() == time_cp)
{
timeUpdate();
}
if(event->timerId() == time_updata_map)
{
mapUpdate();
}
}
void WorkScene::valvv()
{
Myvalue = new MyValue(this);
QString str = Myvalue->get_ans_value() + "mmm";
qDebug() << str;
qDebug() << "kk";
}
void WorkScene::paintEvent(QPaintEvent *)
{
QPainter painter(this);
QPixmap pix;
pix.load(":/res/444.png");
painter.drawPixmap(0,0,this->width(),this->height(),pix);
}
void WorkScene::calcData(QByteArray data)
{
QByteArray data_num;
if(data[0] == '\xAA' && data[1] == '\xAA')
{
data_num.append(data[2]);
data_num.append(data[3]);
bool ok;
dianliu = (data_num.toHex().toInt(&ok,16)) / 100.00;
}
else if(data[0] == '\xBB' && data[1] == '\xBB')
{
data_num.append(data[2]);
data_num.append(data[3]);
bool ok;
dianya = (data_num.toHex().toInt(&ok,16)) / 100.00;
}
else if(data[0] == '\xCC' && data[1] == '\xCC')
{
data_num.append(data[2]);
data_num.append(data[3]);
bool ok;
yali = (data_num.toHex().toInt(&ok,16)) / 100.00;
}
else
{
dianliu = 0;
dianya = 0;
yali = 0;
}
}
void WorkScene::on_btn_dianliu_clicked()
{
qDebug() << "电流采集命令已发送!";
myser->tx_dianliu_code();
}
void WorkScene::on_btn_dianya_clicked()
{
qDebug() << "电压采集命令已发送!";
myser->tx_dianya_code();
}
void WorkScene::on_btn_yali_clicked()
{
qDebug() << "压力采集命令已发送!";
myser->tx_yali_code();
}
void WorkScene::my_init_status()
{
curr_equipment_num_text = new QLabel("仪表设备号:",this);
curr_equipment_num = new QLabel("",this);
curr_equipment_type_text = new QLabel("仪表类型:");
curr_equipment_type = new QLabel("",this);
currentTime->setStyleSheet("color:white;");
curr_equipment_num_text->setStyleSheet("color:white;");
curr_equipment_num->setStyleSheet("color:white;");
curr_equipment_type->setStyleSheet("color:white;");
curr_equipment_type_text->setStyleSheet("color:white;");
curr_equipment_num_text->setFixedWidth(70);
curr_equipment_num->setFixedWidth(15);
curr_equipment_type_text->setFixedWidth(60);
curr_equipment_type->setFixedWidth(30);
ui->statusbar->addPermanentWidget(currentTime);
ui->statusbar->addWidget(curr_equipment_num_text);
ui->statusbar->addWidget(curr_equipment_num);
ui->statusbar->addWidget(curr_equipment_type_text);
ui->statusbar->addWidget(curr_equipment_type);
}
二、串口代码
#include "myserial.h"
#include "ui_myserial.h"
#include <QtSerialPort/QSerialPortInfo>
#include "myvalue.h"
#include <QDebug>
MySerial::MySerial(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MySerial)
{
ui->setupUi(this);
serialPort = new QSerialPort(this);
on_btn_get_serial_clicked();
connect(this,&MySerial::has_data,this,[=](){
qDebug() << "接收到数据了";
});
}
MySerial::~MySerial()
{
delete ui;
}
void MySerial::on_btn_get_serial_clicked()
{
ui->box_choose_serial->clear();
const auto infos = QSerialPortInfo::availablePorts();
for(const QSerialPortInfo &info : infos)
{
QSerialPort serial;
serial.setPort(info);
if(serial.open(QIODevice::ReadWrite))
{
ui->box_choose_serial->addItem(info.portName());
serial.close();
}
}
}
void MySerial::on_btn_open_close_clicked()
{
if(ui->btn_open_close->text() == tr("打开串口"))
{
serialPort->setPortName(ui->box_choose_serial->currentText());
qDebug() <<ui->box_choose_serial->currentText();
if(serialPort->open(QIODevice::ReadWrite))
{
switch (ui->box_rate->currentIndex()) {
case 0:
serialPort->setBaudRate(QSerialPort::Baud115200);
break;
case 1:
serialPort->setBaudRate(QSerialPort::Baud9600);
break;
case 2:
serialPort->setBaudRate(QSerialPort::Baud4800);
break;
default:
break;
}
switch (ui->box_shuju->currentIndex())
{
case 0:
serialPort->setDataBits(QSerialPort::Data8);
break;
case 1:
serialPort->setDataBits(QSerialPort::Data7);
break;
case 2:
serialPort->setDataBits(QSerialPort::Data6);
break;
case 3:
serialPort->setDataBits(QSerialPort::Data5);
break;
default:
break;
}
serialPort->setStopBits(QSerialPort::OneStop);
serialPort->setParity(QSerialPort::NoParity);
connect(serialPort,&QSerialPort::readyRead,this, &MySerial::readToHex);
ui->btn_open_close->setText(tr("关闭串口"));
}
else
{
QMessageBox::warning(NULL , "提示", "串口打开失败,资源未创建或串口可能被占用!");
return;
}
}
else
{
serialPort->clear();
serialPort->close();
serialPort->deleteLater();
ui->btn_open_close->setText(tr("打开串口"));
}
}
void MySerial::readToHex()
{
qDebug() << "触发信号";
emit this->has_data();
QByteArray buf = serialPort->readAll();
QString rece_str = "";
QDataStream out(&buf,QIODevice::ReadOnly);
while (!out.atEnd())
{
qint8 outChar = 0;
out >> outChar;
QString str = QString("%1").arg(outChar&0xFF,2,16,QLatin1Char('0'));
rece_str.append(str.toUpper());
rece_str.append("");
}
MyValue * myval = new MyValue(this);
myval->set_ans_value(buf);
}
void MySerial::tx_dianliu_code()
{
myCom = new MyCommand(this);
sendData = myCom->command_dianliu_code();
serialPort->write(sendData);
}
void MySerial::tx_dianya_code()
{
myCom = new MyCommand(this);
sendData = myCom->command_dianya_code();
serialPort->write(sendData);
}
void MySerial::tx_yali_code()
{
myCom = new MyCommand(this);
sendData = myCom->command_yali_code();
serialPort->write(sendData);
}
三、命令管理文件
#include "mycommand.h"
#include <QDebug>
MyCommand::MyCommand(QWidget *parent) : QMainWindow(parent)
{
}
MyCommand::~MyCommand()
{
}
QByteArray MyCommand::command_dianliu_code()
{
QString str;
str = "21 01 22";
return StringToHex(str);
}
QByteArray MyCommand::command_dianya_code()
{
QString str;
str = "21 02 23";
return StringToHex(str);
}
QByteArray MyCommand::command_yali_code()
{
QString str;
str = "21 03 24";
return StringToHex(str);
}
QByteArray MyCommand::StringToHex(QString str)
{
QByteArray senddata;
int hexdata,lowhexdata;
int hexdatalen = 0;
int len = str.length();
senddata.resize(len/2);
char lstr,hstr;
for(int i=0; i<len; )
{
hstr=str[i].toLatin1();
if(hstr == ' ')
{
i++;
continue;
}
i++;
if(i >= len)
break;
lstr = str[i].toLatin1();
hexdata = ConvertHexChart(hstr);
lowhexdata = ConvertHexChart(lstr);
if((hexdata == 16) || (lowhexdata == 16))
break;
else
hexdata = hexdata*16+lowhexdata;
i++;
senddata[hexdatalen] = (char)hexdata;
hexdatalen++;
}
senddata.resize(hexdatalen);
return senddata;
}
char MyCommand::ConvertHexChart(char ch)
{
if((ch >= '0') && (ch <= '9'))
{
return ch-0x30;
}
else if((ch >= 'A') && (ch <= 'F'))
{
return ch-'A'+10;
}
else if((ch >= 'a') && (ch <= 'f'))
{
return ch-'a'+10;
}
else
{
return -1;
}
}
等等
总结
以上就是今天要讲的内容,具体工程文件可通过下面链接下载!
https://download.csdn.net/download/goujimin/20667219
|