Qt加载动态链接库
1、 直接在pro文件中添加
首先将.a(或者.lib)和.dll文件放入某一目录中,一般是放入程序目录下,例如在程序目录下放入了mydll.lab 和mydll.dll 文件,然后在.pro文件中添加:
LIBS += -L$$PWD/./ -lmydll
有的文章说mingw平台使用.a和.dll文件,msvc使用.lib和.dll,实测在mingw下使用.lib和.dll无任何问题
以上参数说明
-L"文件路径" -l"导入库文件名(不加扩展名)"
上述$$PWD指的是当前目录 即格式:LIBS+= -L地址 -l库名
另外也可以直接在工程上添加 ,如下:
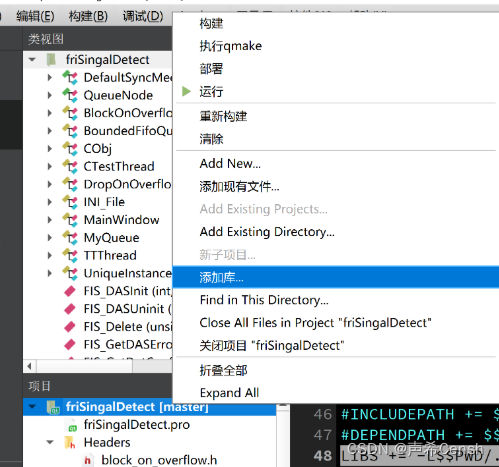
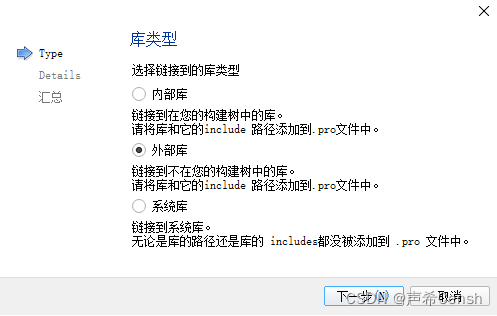
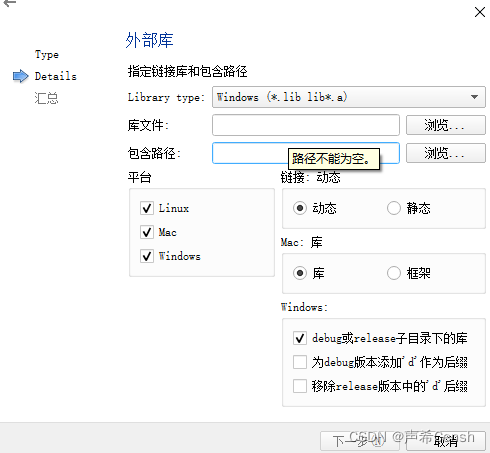
之后Qt会自动在pro文件中加入如下语句:
win32:CONFIG(release, debug|release): LIBS += -L$$PWD/./ -lmydll
else:win32:CONFIG(debug, debug|release): LIBS += -L$$PWD/./ -lmydll
else:unix: LIBS += -L$$PWD/./ -lmydll
#INCLUDEPATH += $$PWD/.
#DEPENDPATH += $$PWD/.
删除,只保留:
LIBS += -L$$PWD/./ -lmydll
2、LoadLibrary显示加载动态链接库(C++方法)
例如要加载当前目录下的DetTMex120T.dll文,该dll文件中包含了三个函数
这三个函数的原型为:
#define DT_API __declspec(dllimport)
DT_API void setData(void* callback);
DT_API int getData();
DT_API bool sendCmd(void * data, unsigned int len);
新建一个.h和.cpp,如下:
①.h文件
#include <Windows.h>
#include <QString>
#ifndef LOAD_DLL_FILE_H
#define LOAD_DLL_FILE_H
typedef void (__stdcall *FP_setData)(void*);
typedef int (__stdcall *FP_getData)(void);
typedef bool (*FP_sendCmd)(void * data, unsigned int len);
extern HINSTANCE gDLLModuleHandle;
extern FP_setData gsetData;
extern FP_getData ggetData;
extern FP_sendCmd gsendCmd;
bool __fastcall gGetFuncFromDLL();
bool loadDllFile();
bool loadDllFile(QString str);
#endif
②.cpp文件
#include "load_dll_file.h"
#include <qdebug.h>
#include <QLibrary>
#include <stringapiset.h>
#include <string>
HINSTANCE gDLLModuleHandle = NULL;
FP_setData gsetData = NULL;
FP_getData ggetData = NULL;
FP_sendCmd gsendCmd = NULL;
bool loadDllFile(QString str)
{
std::string s = str.toStdString();
LPCSTR str_DLL = s.c_str();
gDLLModuleHandle = LoadLibraryA(str_DLL);
if(gDLLModuleHandle)
{
qDebug() << u8"gDLLModuleHandle加载成功!" << endl;
return 1;
}
else
{
qDebug() << u8"gDLLModuleHandle加载失败!" << endl;
return 0;
}
}
bool loadDllFile()
{
gDLLModuleHandle = LoadLibrary(TEXT("DetTMex120T.dll"));
if(gDLLModuleHandle)
{
qDebug() << u8"gDLLModuleHandle加载成功!" << endl;
return 1;
}
else
{
qDebug() << u8"gDLLModuleHandle加载失败!" << endl;
return 0;
}
}
bool __fastcall gGetFuncFromDLL()
{
if(!gDLLModuleHandle) return false;
gsetData = (FP_setData)GetProcAddress(gDasModuleHandle,"setData")
ggetData = (FP_getData)GetProcAddress(gDasModuleHandle,"getData");
gsendCmd = (FP_sendCmd)GetProcAddress(gDasModuleHandle,"sendCmd");
if(!gsetData ||
!ggetData ||
!gsendCmd ||)
return false;
return true;
}
这样就可以使用了,例如在main.cpp中使用(需要包含头文件)
int main()
{
loadDllFile();
gGetFuncFromDLL();
if(gDLLModuleHandle)
{
int state = ggetData();
}
}
|