课题目标
- 使用C语言和卷积运算实现N点移动平均滤波器程序。
输入信号如下所示:
(
1
)
????
x
[
n
]
=
s
i
n
(
π
n
/
64
)
(1)\;\;x[n]=sin(\pi n/64)
(1)x[n]=sin(πn/64)
(
2
)
????
x
[
n
]
=
s
i
n
(
π
n
/
64
)
+
0.2
s
i
n
(
10
π
n
/
64
)
+
0.1
s
i
n
(
20
π
n
/
64
)
(2)\;\;x[n]=sin(\pi n/64)+0.2sin(10\pi n/64)+0.1sin(20\pi n/64)
(2)x[n]=sin(πn/64)+0.2sin(10πn/64)+0.1sin(20πn/64)
(
3
)
????
在
x
[
n
]
=
s
i
n
(
π
n
/
64
)
中
添
加
功
率
为
0.2
的
噪
声
(3)\;\;在x[n]=sin(\pi n/64)中添加功率为0.2的噪声
(3)在x[n]=sin(πn/64)中添加功率为0.2的噪声 N点分别设定为3,6,12,画出波形图。
一、首先实现功率为1的白噪声
白噪声通过一个头文件和源文件实现,代码如下: rnd.h
#ifndef ___RND_H
#define ___RND_H
class UnitRand{
protected:
unsigned int RandKernel;
double RandMax;
public:
void ChangeKernel(unsigned int);
double Urand(void);
UnitRand();
};
class NormRand : public UnitRand{
private:
int Norm_f;
double Norm_k,
Norm_q;
public:
double Nrand(void);
NormRand();
};
#endif
rnd.cpp
#include "rnd.h"
#include <stdio.h>
#include <math.h>
#include <limits.h>
#include <time.h>
#define DOUBLE_PI (atan(1.0) * 4.0)
UnitRand::UnitRand(){
RandKernel = (unsigned int)time(NULL);
RandMax = (double)UINT_MAX+1.0;
}
void UnitRand::ChangeKernel(unsigned int NewKernel){
RandKernel = NewKernel;
}
double UnitRand::Urand(void){
RandKernel *= 0x5D21DB81U;
RandKernel++;
return((double)RandKernel/RandMax);
}
NormRand::NormRand() : UnitRand(){
Norm_f = 0;
}
double NormRand::Nrand(void){
if( Norm_f ){
Norm_f = 0;
return( Norm_k*sin(Norm_q) );
}
Norm_f = 1;
Norm_k = sqrt(-2.0*log(1.0-Urand()));
Norm_q = 2*DOUBLE_PI*Urand();
return( Norm_k*cos(Norm_q) );
}
main.cpp
#include<stdio.h>
#include<math.h>
#include<D:\DOCUMENT\T1\Project2\rnd.h>
#include<stdlib.h>
int main()
{
NormRand sig;
sig.ChangeKernel(2);
int x = 0, i = 0;
for (x = 0; x < 256; ++x)
{
double a = sig.Nrand();
printf("%f\n", a);
}
}
结果使用matlab画图: 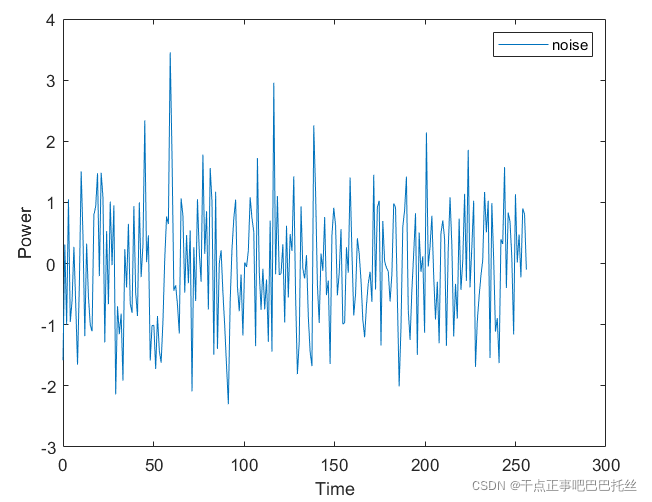
t1x=xlsread('noise.xlsx');
t1 = linspace(0,256,256);
y1=t1x(:,1);
plot(t1,y1);
xlabel('Time');
ylabel('Power');
legend('noise');
set(0,'defaultfigurecolor','w')
|