#include <iostream>
#include <vector>
#include <io.h>
using namespace std;
bool get_filelist_from_dir(std::string path, std::vector<std::string>& files)
{
long long hFile = 0;
//_findnext()第一个参数”路径句柄”,返回的类型为intptr_t(long long),
//如果定义为long,在win7中是没有问题,但是在win10中就要改为long long或者intptr_t
struct _finddata_t fileinfo;
files.clear();
if ((hFile = _findfirst(path.c_str(), &fileinfo)) != -1)
{
do
{
cout << fileinfo.name << endl;
if (!(fileinfo.attrib & _A_SUBDIR))
{
files.push_back(fileinfo.name);
}
} while (_findnext(hFile, &fileinfo) == 0);
_findclose(hFile);
return true;
}
else
{
return false;
}
//另一种写法
//struct _finddata_t fileinfo;
//long long handle;
_findnext()第一个参数”路径句柄”,返回的类型为intptr_t(long long),
如果定义为long,在win7中是没有问题,但是在win10中就要改为long long或者intptr_t
//handle = _findfirst(path.c_str(), &fileinfo); //返回文件句柄<br>
//if (handle == -1)
//{
// cout << "fail..." << endl;
// return false;
//}
//else
//{
// cout << fileinfo.name << endl;
// files.push_back(fileinfo.name);
//}
//cout << "#### 1 ####" << endl;
//while (!_findnext(handle, &fileinfo))
//{
// cout << "#### 2 ####" << endl;
// cout << fileinfo.name << endl;
//}
//_findclose(handle);
//return true;
}
vector<string> getImageList(std::string file_path, std::string suffix)
{
//string file_path = "D:\\image\\";
//string suffix = "*.png";
string search_path = file_path + suffix;
vector<string> file_list;//保存遍历到的文件name
if (!get_filelist_from_dir(search_path, file_list))
cout << "open file error!" << endl;
vector<string> image_path_list;//保存文件绝对路径
for (int i = 0; i < file_list.size(); i++)
{
image_path_list.push_back(file_path + file_list[i]);
//Mat image = imread(image_path);
//这里可以对图像进行处理
}
return image_path_list;
}
int main()
{
vector<string> output;
string filepath = "D:\\image\\";
string suffix = "*.*";//后缀名
output = getImageList(filepath, suffix);
return 0;
}
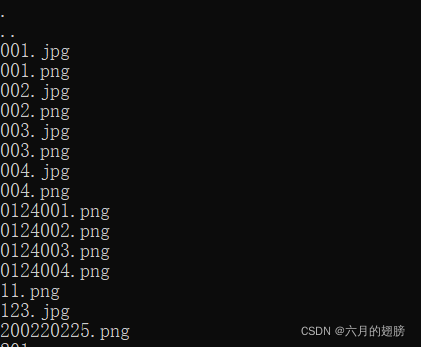
涉及到了一个结构体,需要用的是name属性,当然假如需要文件大小的话也可以把size提取出来
#define _finddata_t _finddata64i32_t
struct _finddata64i32_t
{
unsigned attrib;
__time64_t time_create; // -1 for FAT file systems
__time64_t time_access; // -1 for FAT file systems
__time64_t time_write;
_fsize_t size;
char name[260];
};
?
|