意图
原型模式(Prototype)是一种创建型设计模式, 使你能够复制已有对象, 而又无需使代码依赖它们所属的类。
结构
原型式的结构包含以下几个角色:
- 抽象原型类(AbstractPrototype):声明克隆clone自身的接口
- 具体原型类(ConcretePrototype):实现clone接口
- 客户端(Client):客户端中声明一个抽象原型类,根据客户需求clone具体原型类对象实例
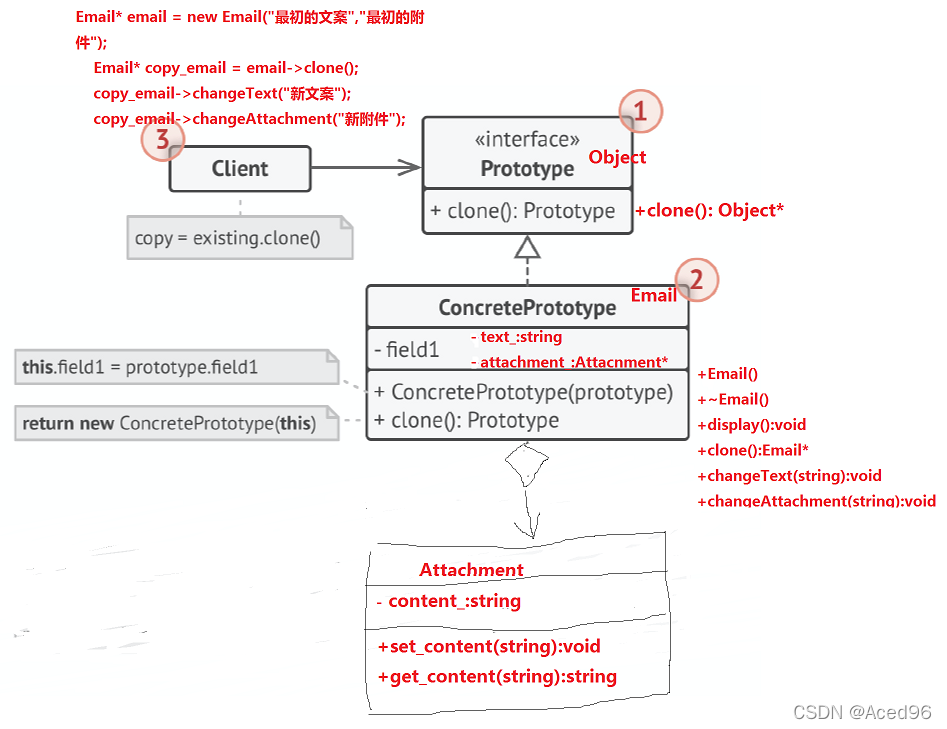
代码
Prototype.h:
#ifndef PROTOTYPE_H_
#define PROTOTYPE_H_
class Object
{
public:
virtual Object* clone() = 0;
};
#endif
ConcretePrototype.h:
#ifndef CONCRETE_PROTOTYPE_H_
#define CONCRETE_PROTOTYPE_H_
#include<iostream>
#include<string>
#include"Prototype.h"
class Attachment
{
public:
void set_content(std::string content){ content_ = content; }
std::string get_content(){ return content_; }
private:
std::string content_;
};
class Email : public Object
{
public:
Email(){}
Email(std::string text, std::string attachment_content):text_(text),attachment_(new Attachment())
{
attachment_->set_content(attachment_content);
}
~Email()
{
if(attachment_ != nullptr)
{
delete attachment_;
attachment_ = nullptr;
}
}
void display()
{
std::cout << "------------查看邮件------------" << std::endl;
std::cout << "正文: " << text_ << std::endl;
std::cout << "邮件: " << attachment_->get_content() << std::endl;
std::cout << "------------查看完毕--------" << std::endl;
}
Email* clone() override
{
return new Email(this->text_, this->attachment_->get_content());
}
void changeText(std::string new_text)
{
text_ = new_text;
}
void changeAttachment(std::string content)
{
attachment_->set_content(content);
}
private:
std::string text_;
Attachment* attachment_ = nullptr;
};
#endif
main.cpp:
#include<iostream>
#include"ConcretePrototype.h"
int main()
{
Email* email = new Email("最初的文案","最初的附件");
Email* copy_email = email->clone();
copy_email->changeText("新文案");
copy_email->changeAttachment("新附件");
std::cout<<"copy email:"<<std::endl;
copy_email->display();
delete email;
delete copy_email;
return 0;
}
|