DisposeXMLFile.h
#include "tinyxml.h"
#include <string>
using namespace std;
class DisposeXMLFile
{
public:
DisposeXMLFile();
~DisposeXMLFile();
void createXMLFile();
void addXMLInfo();
void modifyNodeValue(TiXmlElement *pParentNodeElet, const string strChildNodeName, const string strValue);
void deleteNode(TiXmlElement *pParentNodeElet, const string strChildNodeName);
TiXmlElement *findNode(TiXmlElement *pNode, const string nodeName);
TiXmlDocument *getXmlDocument();
private:
TiXmlDocument *m_pDocument;
TiXmlDeclaration *m_pDeclaration;
};
DisposeXMLFile.cpp
#include "DisposeXMLFile.h"
#include <iostream>
#include <vector>
using namespace std;
const char *g_strXMLFolder = "plan.xml";
DisposeXMLFile::DisposeXMLFile()
{
m_pDocument = nullptr;
m_pDeclaration = nullptr;
}
DisposeXMLFile::~DisposeXMLFile()
{
if (nullptr != m_pDocument)
delete m_pDocument;
}
TiXmlDocument *DisposeXMLFile::getXmlDocument()
{
return m_pDocument;
}
创建XML文件
void DisposeXMLFile::createXMLFile()
{
m_pDocument = new TiXmlDocument(g_strXMLFolder);
if (nullptr == m_pDocument)
{
return;
}
m_pDeclaration = new TiXmlDeclaration("1.0", "UTF-8", "");
if (nullptr == m_pDeclaration)
{
return;
}
m_pDocument->LinkEndChild(m_pDeclaration);
}
添加XML内容
void DisposeXMLFile::addXMLInfo()
{
string strXMLName = g_strXMLFolder;
string strRootName = (strXMLName.substr(0, strXMLName.find(".")));
TiXmlElement *pRoot = new TiXmlElement(strRootName.c_str());
if (nullptr == pRoot)
{
return;
}
m_pDocument->LinkEndChild(pRoot);
TiXmlElement *pVersion = new TiXmlElement("VERSION");
TiXmlText *pVersionText = new TiXmlText("1.0.0.0");
pVersion->LinkEndChild(pVersionText);
TiXmlElement *pImagesetList = new TiXmlElement("IMAGESET_LIST");
TiXmlElement *pCount = new TiXmlElement("COUNT");
TiXmlText *pCountText = new TiXmlText("3");
pCount->LinkEndChild(pCountText);
TiXmlElement *pImageList = new TiXmlElement("IMAGE_LIST");
TiXmlElement *pImageOne = new TiXmlElement("IMAGE");
TiXmlText *pImageOneText = new TiXmlText("000000");
pImageOne->LinkEndChild(pImageOneText);
TiXmlElement *pImageTwo = new TiXmlElement("IMAGE");
TiXmlText *pImageTwoText = new TiXmlText("111111");
pImageTwo->LinkEndChild(pImageTwoText);
TiXmlElement *pImageThree = new TiXmlElement("IMAGE");
TiXmlText *pImageThreeText = new TiXmlText("222222");
pImageThree->LinkEndChild(pImageThreeText);
pImageList->LinkEndChild(pImageOne);
pImageList->LinkEndChild(pImageTwo);
pImageList->LinkEndChild(pImageThree);
pImagesetList->LinkEndChild(pCount);
pImagesetList->LinkEndChild(pImageList);
pRoot->LinkEndChild(pVersion);
pRoot->LinkEndChild(pImagesetList);
m_pDocument->SaveFile(g_strXMLFolder);
}
添加XML内容后效果
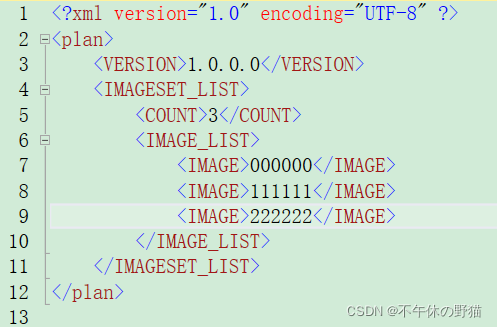
修改节点文本
void DisposeXMLFile::modifyNodeValue(TiXmlElement *pParentNodeElet, const string strChildNodeName, const string strValue)
{
TiXmlElement *pChildNode = findNode(pParentNodeElet, strChildNodeName);
if (nullptr == pChildNode)
{
return;
}
TiXmlText *pText = new TiXmlText(strValue.c_str());
pChildNode->Clear();
pChildNode->LinkEndChild(pText);
m_pDocument->SaveFile(g_strXMLFolder);
}
修改节点文本后效果
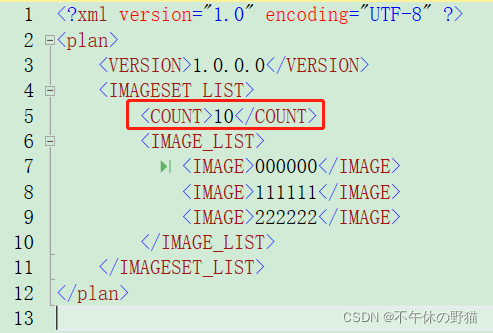
删除节点
void DisposeXMLFile::deleteNode(TiXmlElement *pParentNodeElet, const string strChildNodeName)
{
TiXmlElement *pChildNode = findNode(pParentNodeElet, strChildNodeName);
if (nullptr == pChildNode)
{
return;
}
TiXmlNode *parent = pChildNode->Parent();
if (nullptr == parent)
{
return;
}
TiXmlElement *parentElem = parent->ToElement();
if (nullptr == parentElem)
{
return;
}
parentElem->RemoveChild(pChildNode);
m_pDocument->SaveFile(g_strXMLFolder);
}
删除节点后效果
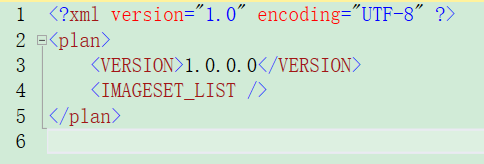
TiXmlElement *DisposeXMLFile::findNode(TiXmlElement *pNode, const string strNodeName)
{
TiXmlElement *p = pNode;
for (p = p->FirstChildElement(); p != nullptr; p = p->NextSiblingElement())
{
const char *value = p->Value();
if (strcmp(p->Value(), strNodeName.c_str()) == 0)
{
return p;
}
}
return nullptr;
}
int main()
{
DisposeXMLFile xmlFile;
xmlFile.createXMLFile();
xmlFile.addXMLInfo();
TiXmlDocument *pXMLDcument = xmlFile.getXmlDocument();
pXMLDcument->LoadFile(g_strXMLFolder);
TiXmlElement *xmlRoot = pXMLDcument->RootElement();
string strValue = "10";
TiXmlElement *nodeImagesetListElem = xmlRoot->FirstChildElement("IMAGESET_LIST");
xmlFile.modifyNodeValue(nodeImagesetListElem, "COUNT", strValue);
TiXmlElement* nodeImageListElem = xmlRoot->FirstChildElement("IMAGESET_LIST");
TiXmlElement* nodeCountElem = xmlRoot->FirstChildElement("IMAGESET_LIST");
xmlFile.deleteNode(nodeImageListElem,"IMAGE_LIST" );
xmlFile.deleteNode(nodeImageListElem, "COUNT");
return 0;
}
|