代码
WindowsUtils.hpp
#ifndef __WGUI_WINDOWSUTILS_HPP__
#define __WGUI_WINDOWSUTILS_HPP__
#include <windows.h>
#include <iostream>
#include <sstream>
#include <map>
namespace WUtils
{
typedef LRESULT CALLBACK (*WNDPROC)(HWND hwnd, UINT Message, WPARAM wParam, LPARAM lParam);
void check(HWND hwnd)
{
if (hwnd == NULL)
{
int error = GetLastError();
std::cout << "Error:" << error << std::endl;
std::stringstream str;
str << "Window Creation Failed!\nError:" << error;
MessageBox(NULL, TEXT(str.str().data()), TEXT("Error!"), MB_ICONEXCLAMATION | MB_OK);
}
}
bool registerClass(LPCTSTR className, HINSTANCE hInstance, WNDPROC WndProc)
{
WNDCLASSEX wc;
memset(&wc, 0, sizeof(wc));
wc.cbSize = sizeof(WNDCLASSEX);
wc.lpfnWndProc = WndProc;
wc.hInstance = hInstance;
wc.hCursor = LoadCursor(NULL, IDC_ARROW);
wc.hbrBackground = (HBRUSH)(COLOR_WINDOW + 1);
wc.lpszClassName = className;
wc.hIcon = LoadIcon(NULL, IDI_APPLICATION);
wc.hIconSm = LoadIcon(NULL, IDI_APPLICATION);
if (!RegisterClassEx(&wc))
{
int error = GetLastError();
std::cout << "Error:" << error << std::endl;
std::stringstream str;
str << "Window Registration Failed!\nError:" << error;
MessageBox(NULL, TEXT(str.str().data()), TEXT("Error!"), MB_ICONEXCLAMATION | MB_OK);
return false;
}
return true;
}
typedef void (*CW_CB)(HWND wndhwnd,HWND thishwnd, UINT Message, WPARAM wParam, LPARAM lParam);
struct ChildWindow
{
HWND hwnd;
CW_CB cb;
};
typedef std::map<int, ChildWindow> ChildMap;
void ProcessEvent(ChildMap cm, HWND hwnd, UINT Message, WPARAM wParam, LPARAM lParam)
{
int id = LOWORD(wParam);
int event = HIWORD(wParam);
std::cout << id << std::endl;
std::cout << cm.size() << std::endl;
ChildWindow cw = cm.at(id);
(*cw.cb)(hwnd,cw.hwnd,Message,wParam,lParam);
}
void addChild(ChildMap& cm,HWND hwnd,CW_CB cb,int id)
{
check(hwnd);
ChildWindow child;
memset(&child,0,sizeof(ChildWindow));
child.hwnd = hwnd;
child.cb = cb;
cm.insert(std::pair<int,ChildWindow>(id,child));
}
}
#endif
test.cpp
#include <windows.h>
#include "../WindowsUtils.hpp"
using namespace WUtils;
ChildMap childs;
HINSTANCE instanceHandle;
HWND window;
LRESULT CALLBACK WndProc(HWND hwnd, UINT Message, WPARAM wParam, LPARAM lParam);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow)
{
instanceHandle = hInstance;
registerClass(TEXT("WindowClass"),hInstance,WndProc);
window = CreateWindowEx(WS_EX_CLIENTEDGE,TEXT("WindowClass"),TEXT("Window"),WS_VISIBLE|WS_OVERLAPPEDWINDOW,0,0,640,480,NULL,NULL,hInstance,NULL);
check(window);
MSG msg;
while (GetMessage(&msg, NULL, 0, 0) > 0)
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
void cb(HWND wndhwnd,HWND thishwnd, UINT Message, WPARAM wParam, LPARAM lParam)
{
MessageBox(thishwnd,TEXT(""),TEXT(""),MB_OK);
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT Message, WPARAM wParam, LPARAM lParam)
{
switch(Message)
{
case WM_CREATE:
{
int id = 1;
HWND btn = CreateWindowEx(WS_EX_WINDOWEDGE,TEXT("button"),TEXT("按钮"),WS_VISIBLE|WS_BORDER|WS_CHILD|BS_CENTER,0,0,100,100,hwnd,(HMENU)id,instanceHandle,NULL);
addChild(childs,btn,cb,id);
break;
}
case WM_DESTROY:
{
PostQuitMessage(0);
break;
}
case WM_COMMAND:
{
ProcessEvent(childs,hwnd,Message,wParam,lParam);
DefWindowProc(hwnd, Message, wParam, lParam);
}
default:
return DefWindowProc(hwnd, Message, wParam, lParam);
}
return 0;
}
效果图
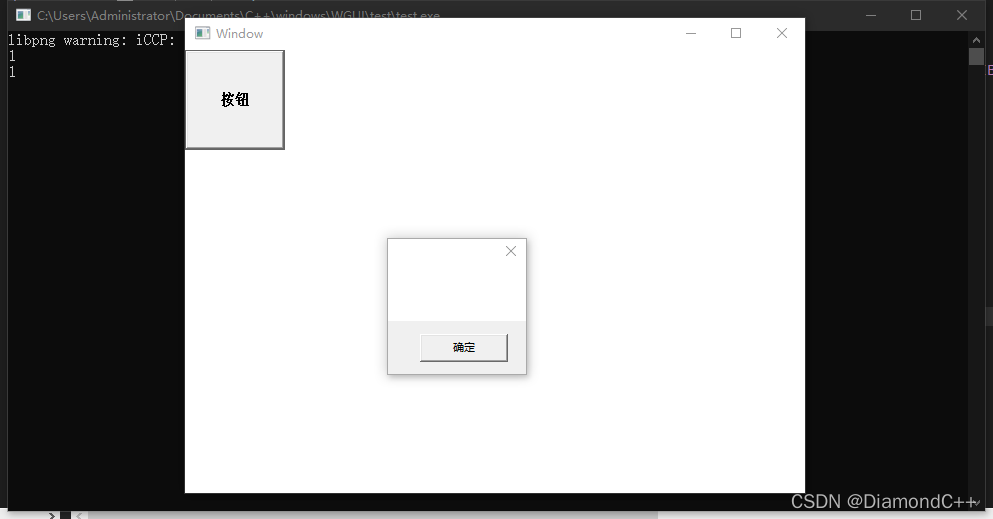
|