游戏破解器与文件加解密
Clion输出中文乱码终极解决方案: https://blog.csdn.net/qq_43302195/article/details/109009784
1. 游戏作弊器
工具的使用: 打开附件提供的修改器(Cheat Engine.exe) => 文件,打开进程,找到并选择正在运行的目标exe => 调试进程 => 输入值(eg:97) => 首次扫描,找到97对应的地址,右键修改其所对应的值,完成修改.
#include <stdio.h>
#include <windows.h>
int main() {
printf("游戏倒计时开始了....\n");
int i;
for (int i = 100; i > 0 ; i--) {
printf("还剩下多少秒:%d, 内存地址是:%p\n", i, &i);
Sleep(2000);
}
printf("恭喜,你已经赢得了游戏最高等级,碉堡了!\n");
getchar();
return 0;
}
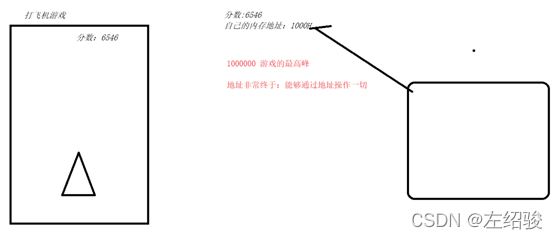
2. 文件的读
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
char * fileNameStr = "H:\\00C\\0C06\\Leo.txt";
FILE * file = fopen(fileNameStr, "r");
if (!file) {
printf("文件打开失败,请你个货检测:路径为%s路径的文件,看看有什么问题\n", fileNameStr);
exit(0);
}
char buffer[10];
while (fgets(buffer, 10, file)) {
printf("%s\n", buffer);
}
fclose(file);
return 0;
}
3. 文件的写
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
char * fileNameStr = "H:\\00C\\0C06\\LeoW.txt";
FILE * file = fopen(fileNameStr, "w");
if (!file) {
printf("文件打开失败,请你个货检测:路径为%s路径的文件,看看有什么问题\n", fileNameStr);
exit(0);
}
fputs("ni hao", file);
fclose(file);
return 0;
}
4. 文件的复制
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
char * fileNameStr = "H:\\00C\\0C06\\Leo.txt";
char * fileNameStrCopy = "H:\\00C\\0C06\\LeoCopy.txt";
FILE * file = fopen(fileNameStr, "rb");
FILE * fileCopy = fopen(fileNameStrCopy, "wb");
if (!file || !fileCopy) {
printf("文件打开失败,请你个货检测:路径为%s路径的文件,看看有什么问题\n", fileNameStr);
exit(0);
}
int buffer[512];
int len;
while ((len = fread(buffer, sizeof(int), 514, file)) != 0) {
fwrite(buffer, sizeof(int), len, fileCopy);
}
fclose(file);
fclose(fileCopy);
return 0;
}
5. 文件大小的获取
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
char * fileNameStr = "H:\\00C\\0C06\\Leo.txt";
FILE * file = fopen(fileNameStr, "rb");
if (!file) {
printf("文件打开失败,请你个货检测:路径为%s路径的文件,看看有什么问题\n", fileNameStr);
exit(0);
}
fseek(file, SEEK_SET, SEEK_END);
long file_size = ftell(file);
printf("%s文件的字节大小是:%ld\n", fileNameStr, file_size);
fclose(file);
return 0;
}
6. 文件加密和文件解密
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
char * fileNameStr = "H:\\00C\\0C06\\Leo.png";
char * fileNameStrEncode = "H:\\00C\\0C06\\LeoEncode.png";
FILE * file = fopen(fileNameStr, "rb");
FILE * fileEncode = fopen(fileNameStrEncode, "wb");
if (!file || !fileEncode) {
printf("文件打开失败,请你个货检测:路径为%s路径的文件,看看有什么问题\n", fileNameStr);
exit(0);
}
int c;
while ((c = fgetc(file)) != EOF) {
fputc(c ^ 5, fileEncode);
}
fclose(file);
fclose(fileEncode);
}
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
char * fileNameStr = "H:\\00C\\0C06\\LeoEncode.png";
char * fileNameStrDecode = "H:\\00C\\0C06\\LeoDecode.png";
FILE * file = fopen(fileNameStr, "rb");
FILE * fileEncode = fopen(fileNameStrDecode, "wb");
if (!file || !fileEncode) {
printf("文件打开失败,请你个货检测:路径为%s路径的文件,看看有什么问题\n", fileNameStr);
exit(0);
}
int c;
while ((c = fgetc(file)) != EOF) {
fputc(c ^ 5, fileEncode);
}
fclose(file);
fclose(fileEncode);
}
7. 字符串密码加密和解密
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int mainT9() {
char * fileNameStr = "H:\\00C\\0C06\\Leo.png";
char * fileNameStrEncode = "H:\\00C\\0C06\\LeoEncode.png";
char * password = "123456";
FILE * file = fopen(fileNameStr, "rb");
FILE * fileEncode = fopen(fileNameStrEncode, "wb");
if (!file || !fileEncode) {
printf("文件打开失败,请你个货检测:路径为%s路径的文件,看看有什么问题\n", fileNameStr);
exit(0);
}
int c;
int index = 0;
int pass_len = strlen(password);
while ((c = fgetc(file)) != EOF) {
char item = password[index%pass_len];
printf("item:%c\n", item);
fputc(c ^ item, fileEncode);
index ++;
}
fclose(file);
fclose(fileEncode);
return 0;
}
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
char * fileNameStr = "H:\\00C\\0C06\\LeoEncode.png";
char * fileNameStrDecode = "H:\\00C\\0C06\\LeoDecode.png";
FILE * file = fopen(fileNameStr, "rb");
FILE * fileDecode = fopen(fileNameStrDecode, "wb");
if (!file || !fileDecode) {
printf("文件打开失败,请你个货检测:路径为%s路径的文件,看看有什么问题\n", fileNameStr);
exit(0);
}
char * password = "123456";
int c;
int index = 0;
int pass_len = strlen(password);
while ((c = fgetc(file)) != EOF) {
fputc(c ^ password[index%pass_len], fileDecode);
index++;
}
fclose(file);
fclose(fileDecode);
return 0;
}
8. 上节课遗留知识点
#include <stdio.h>
void substrAction3(char * result, char * str, int start, int end) {
for (int i = start; i < end; ++i) {
*(result++) = *(str + i);
}
}
int mainT11() {
char * str = "Derry is";
char result[100] = "ABC";
substrAction3(result, str, 3, 5);
printf("%s\n", result);
char * arry = "21";
char arry3[10] = "12";
return 0;
}
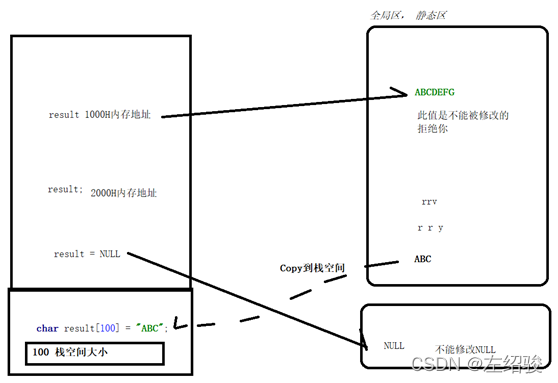
9. 额外练习题
#include "stdio.h"
void MySort(int* array, int arraySize);
int main() {
int array[10] = {23,325,3,656,1,-23,235,666,90,879};
int length = sizeof(array) / sizeof(int);
MySort(array, length);
for (int i = 0; i < length; ++i) {
printf("%d ", array[i]);
}
}
void MySort(int *array, int arraySize) {
int m,i,j;
for (i = 0; i < arraySize - 1; ++i) {
for (j = 0; j < arraySize - 1 - i; ++j) {
if (array[j] > array[j + 1]) {
m = array[j];
array[j] = array[j + 1];
array[j + 1] = m;
}
}
}
}
#include "stdio.h"
void UpperCase(char str[]) {
for(size_t i = 0; i < sizeof(str)/sizeof(str[0]); ++i) {
if ('a' <= str[i] && str[i] <= 'z') {
str[i] -= ('a' - str[i] + 'A');
}
}
}
int main() {
char str[] = "aBcDe";
UpperCase(str);
printf("%c ", str);
}
#include <stdio.h>
int climbStairs(int n) {
if (n <= 2) {
return n;
} else {
int num1 = 1;
int num2 = 2;
int temp = 0;
for (int i = 2; i < n; ++i) {
temp = num1 + num2;
num1 = num2;
num2 = temp;
}
return temp;
}
}
int main() {
int n = 0;
scanf("%d", &n);
printf("%d ", climbStairs(n));
}
|