单点时限:?2.0 sec
内存限制:?256 MB
There is a database,partychen want you to sort the database’s data in the order from the least up to the greatest element,then do the query: “Which element is i-th by its value?”- with i being a natural number in a range from 1 to N.
It should be able to process quickly queries like this.
输入格式
The standard input of the problem consists of two parts. At first, a database is written, and then there’s a sequence of queries. The format of database is very simple: in the first line there’s a number?N(1?N?100000), in the next N lines there are numbers of the database one in each line in an arbitrary order. A sequence of queries is written simply as well: in the first line of the sequence a number of queries?K(1?K?100)?is written, and in the next?K?lines there are queries one in each line. The query “Which element is i-th by its value?” is coded by the number i.
输出格式
The output should consist of K lines. In each line there should be an answer to the corresponding query. The answer to the query “i” is an element from the database, which is i-th by its value (in the order from the least up to the greatest element).
样例
input
5
7
121
123
7
121
3
3
2
5
output
121
7
123
提示
Hint After we sort the data we get 7 7 121 121 123,so the postion 3 is 121,the postion 2 is 7 and the position 5 is 123 etc.
代码如下:
#include<iostream>
using namespace std;
int partition(int A[], int low, int high)
{
int i = low, j = high + 1;
int p = A[low];
while (true) {
while (A[++i] < p && i < high);//从左到右找大的
while (A[--j] > p);//从右到左找小的
if (i >= j)break;
swap(A[i], A[j]);
}
A[low] = A[j];
A[j] = p;
return j;
}
void quickSort(int A[], int low, int high)
{
if (low < high) {
int j = partition(A, low, high);
quickSort(A, low, j - 1);
quickSort(A, j + 1, high);
}
}
int main()
{
int n;
cin >> n;
int A[100001];
for (int i = 1; i <= n; i++)
cin >> A[i];
int queries;
cin >> queries;
int q[100];
for (int i = 0; i < queries; i++)
cin >> q[i];
quickSort(A, 1, n);
for (int i = 0; i < queries; i++)
cout << A[q[i]] << endl;
return 0;
}
运行结果如下:
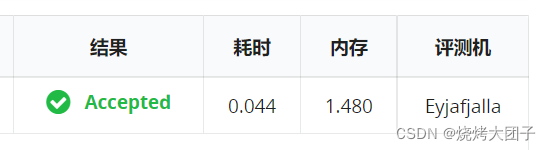
?
|