多线程单例创建对象时,需要加锁保护。
其示例代码如下:
#include <atomic>
#include <chrono>
#include <iostream>
#include <mutex>
#include <thread>
class TSingleton
{
public:
static TSingleton* ins()
{
if (__instance != NULL)
return __instance;
locker.lock();
if (__instance == NULL)
__instance = new TSingleton; //注意没有指定atomic,可能导致CPU乱序执行导致异常
locker.unlock();
return __instance;
}
static void release()
{
if (__instance == NULL)
return;
locker.lock();
if (__instance != NULL)
{
delete __instance;
__instance = NULL;
}
locker.unlock();
}
void speak()
{
std::cout << "Welcome to Shanghai !" << std::endl;
}
void cry()
{
std::cout << "oh, I am seeking you !" << std::endl;
}
private:
TSingleton() {};
static std::atomic<TSingleton*> __instance;
static std::mutex locker;
};
std::atomic<TSingleton*> TSingleton::__instance = NULL;
std::mutex TSingleton::locker;
bool bExit = false;
int main()
{
std::thread thr1([=]()
{
while (!bExit)
{
TSingleton::ins()->speak();
std::this_thread::sleep_for(std::chrono::seconds(1));
}
});
std::thread thr2([=]()
{
while (!bExit)
{
TSingleton::ins()->cry();
std::this_thread::sleep_for(std::chrono::seconds(2));
}
});
thr1.detach();
thr2.detach();
char c;
std::cin >> c;
bExit = true;
std::this_thread::sleep_for(std::chrono::seconds(3));
TSingleton::release();
return 0;
}
运行效果如下:
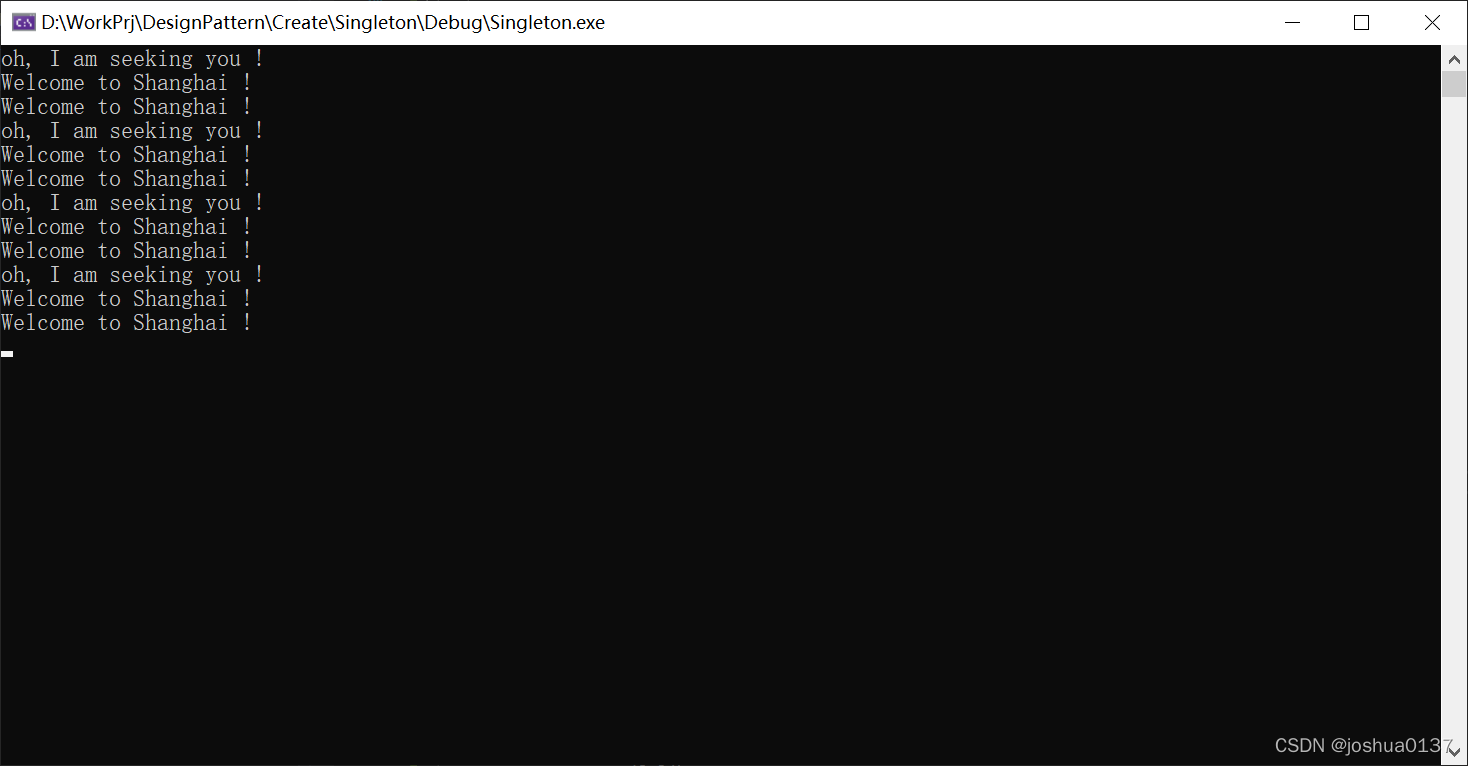
|