1 函数基本知识
要使用C++函数,必须完成如下工作:
如下例子用一个简单的示例演示了这三个步骤:
//函数原型
void simple();
int main(){
using namespace std;
cout<<"main() will call the simple() function:\n";
//函数调用
simple();
cout<<"main() is finished with the simple() function.\n";
return 0;
}
//函数定义
void simple(){
using namespace std;
cout<<"I'm but a simple function.\n";
}
程序输出:
main() will call the simple() function:
I'm but a simple function.
main() is finished with the simple() function.
执行函数simple() 时,将暂停执行main() 中的代码;等函数simple() 执行完毕后,继续执行main() 中的代码。在每个函数定义中,都使用了一条using 编译指令,因为每个函数都使用了cout 。另一种方法是,在函数定义之前放置一条using 编译指令或在函数中使用std::cout
1.1 定义函数
可以将函数分成两类:没有返回值的函数和有返回值的函数。没有返回值的函数被称为void函数,其通用格式如下: 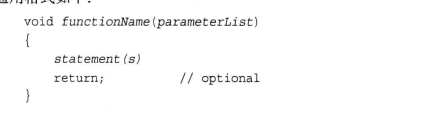 可选的返回语句标记了函数的结尾;否则,函数将在右花括号处结束。
有返回值的函数将生成一个值,并将它返回给调用函数。这种函数的类型被声明为返回值的类型,其通用格式如下:    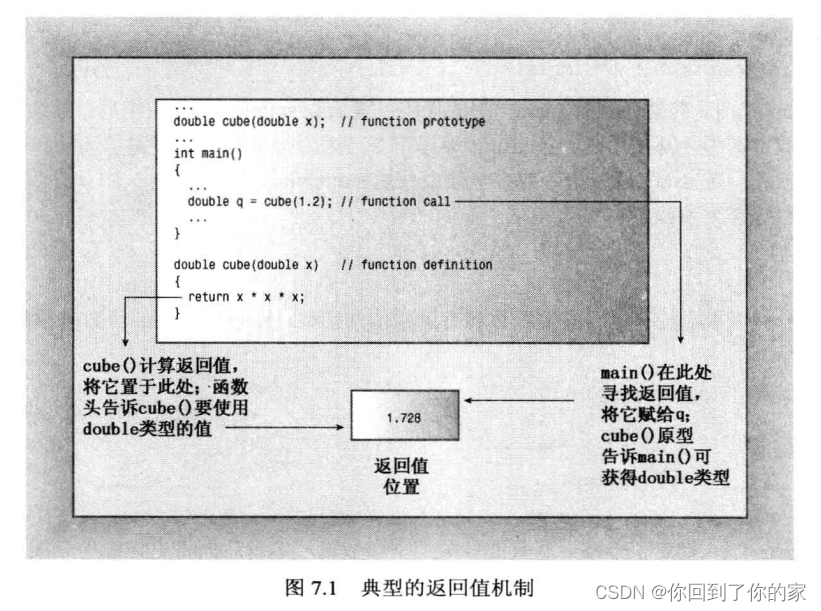 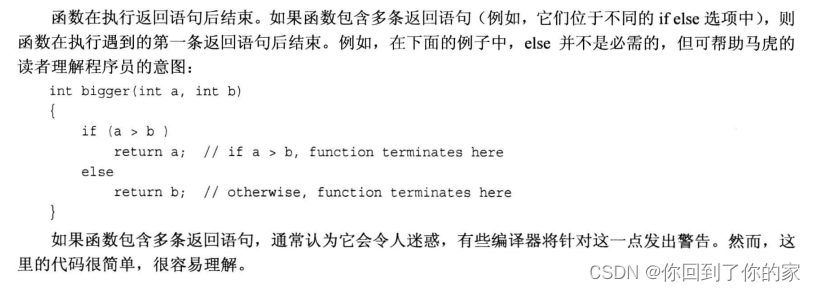
1.2 函数原型和函数调用
下面的例子在一个程序中使用了函数cheer() 和cube() ,留意其中的函数原型:
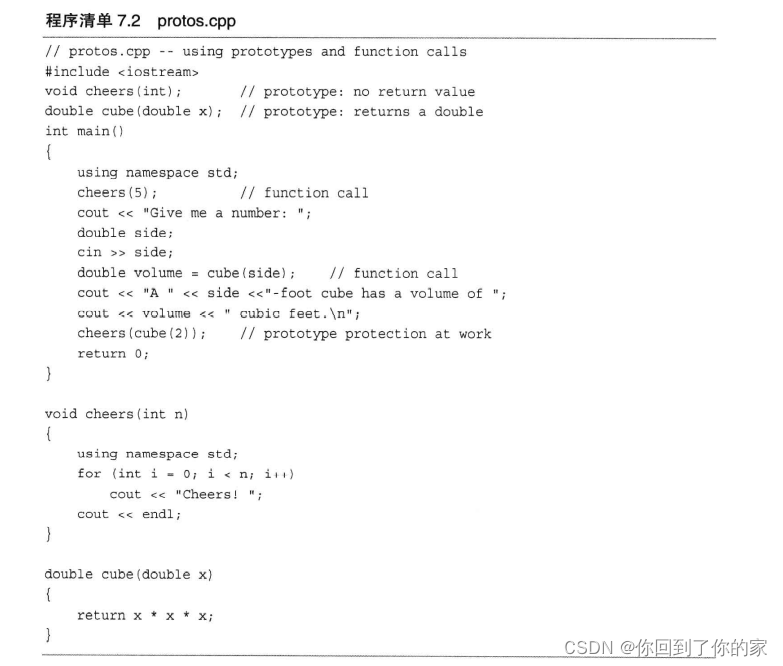   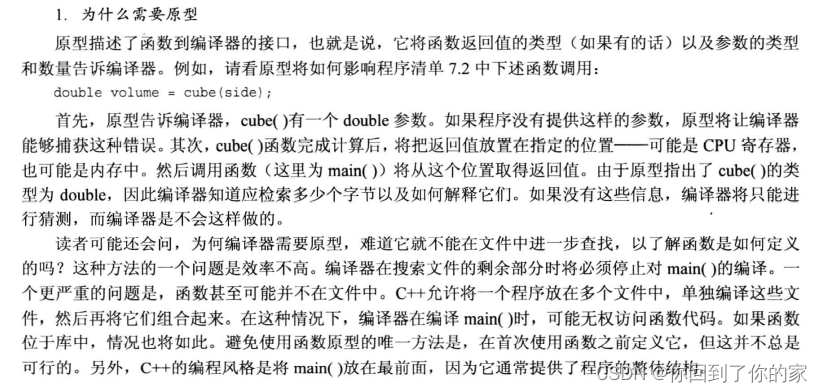 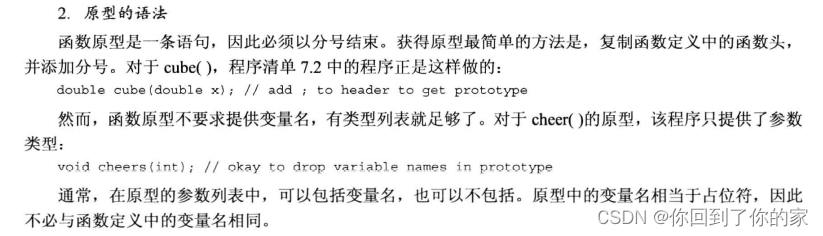  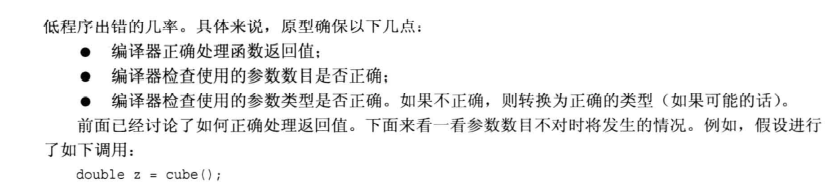 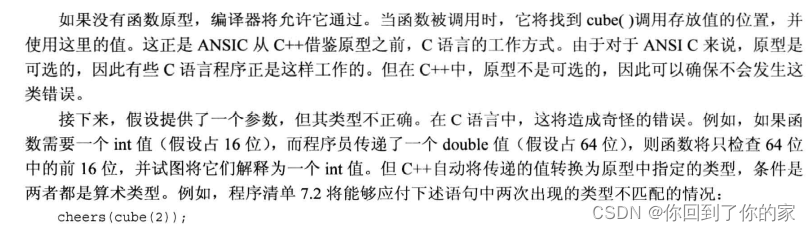 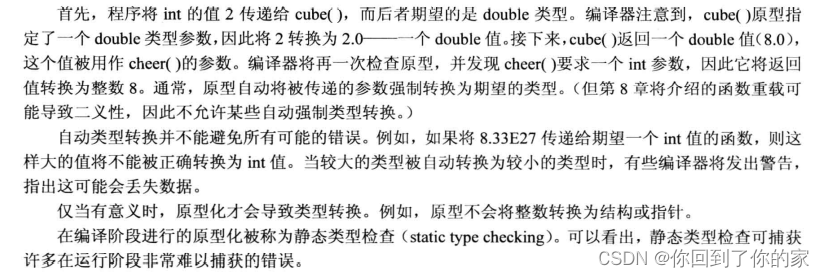
2 函数参数和按值传递
C++通常按值传递参数,这意味着将数值参数传递给函数,而后者将其赋给一个新的变量。例如,程序7.2包含下面的函数调用:
double volume=cube(side);
其中,side 是一个变量,在前面的程序运行中,其值为5.cube() 的函数头如下:
double cube(double x)
被调用时,该函数将创建一个新的名为x 的double变量,并将其初始化为5.这样,cube() 执行的操作将不会影响main() 中的数据,因为cube() 使用的是side的副本,而不是原来的数据。用于接收传递值的变量被称为形参,传递给函数的值被称为实参。出于简化的目的,C++标准使用参数(argument)来表示实参,使用参量(parameter)来表示形参,因此参数传递将参量付给参数,如图7.2所示:
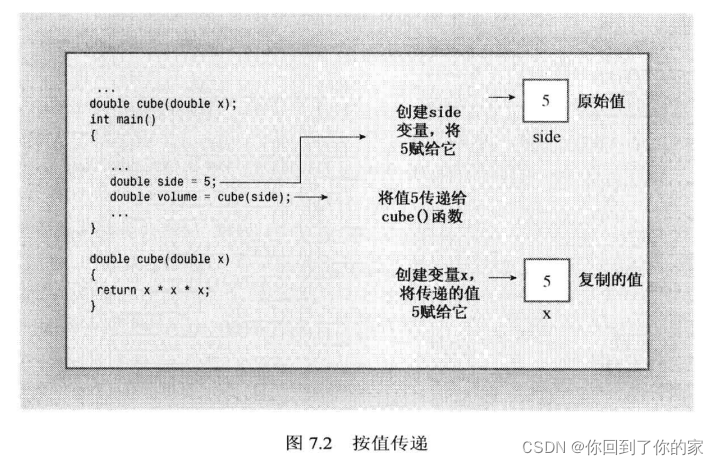 待补充 224
|