1.内存布局
在 C++ 中,我们把内存详细分为 5 个区域:
- 栈:一般函数内的局部变量都会放在这里,由编译器自动分配和释放。
- 堆:程序员通过 malloc()/free() 或 new/delete 来分配和释放。
- 全局区/静态存储区:存放全局变量和静态变量,程序结束时由系统释放。
- 常量存储区
- 程序代码区
堆和栈的区别:
- 栈:空间是有限的,分配速度快,程序员控制不了。
- 堆:只要不超出实际拥有的物理内存,同时也在操作系统允许能够分配的最大内存大小之内,都可以分配成功。分配速度比栈慢,程序员可以随时用 malloc()/free() 或 new/delete 来分配和释放,非常灵活。
2.malloc()/free() 的用法
在程序运行过程,为了能够自由控制内存的生命周期、大小,会经常使用堆空间的内存。
在 C 语言中,用 malloc() 和 free() 从堆中分配和释放内存,malloc() 和 free() 是函数。
一般使用格式:
void *malloc(int NumBytes) ,其中 NumBytes 表示要分配的字节数。分配成功则返回指向被分配内存的指针,分配失败则返回 NULL。当分配的这段内存不再使用的时候,应该用 free() 函数将内存释放掉,供其他地方使用。
void free(void *FirstByte) ,将之前用 malloc() 分配的内存空间还给程序(操作系统),也就是说释放了这块内存,这样这块内存就被系统回收并在需要的时候由系统分配出去再给其他地方使用。
举例1:
#include<iostream>
using namespace std;
int main()
{
int* p = NULL;
p = (int*)malloc(sizeof(int));
if (p != NULL)
{
*p = 10;
cout << *p << endl;
free(p);
}
return 0;
}
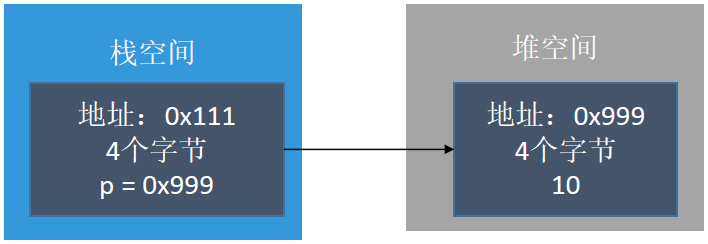
举例2:
#include<iostream>
using namespace std;
int main()
{
int* p = (int*)malloc(100 * sizeof(int));
if (p != NULL)
{
int* q = p;
*q++ = 1;
*q++ = 5;
cout << *p << endl;
cout << *(p + 1) << endl;
free(p);
}
return 0;
}
初始化:
memset() 函数是将较大的数据结构(比如对象、数组等)内存清零的比较快的方法。
#include <iostream>
using namespace std;
int main()
{
int size = sizeof(int) * 10;
int* p = (int*)malloc(size);
memset(p, 1, size);
cout << *p << endl;
return 0;
}
注意:通过 malloc()/free() 分配和释放的对象不会调用其构造函数和析构函数。
#include <iostream>
using namespace std;
struct Person
{
int m_age;
Person()
{
cout << "Person::Person()" << endl;
}
~Person()
{
cout << "Person::~Person()" << endl;
}
void run()
{
cout << "Person::run() - " << m_age << endl;
}
};
int main()
{
Person* p = (Person*)malloc(sizeof(Person));
p->m_age = 10;
p->run();
free(p);
return 0;
}
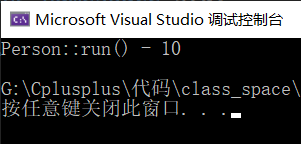
3.new/delete 的用法
new/delete 是关键字或运算符,不是函数。在 C++ 中使用 new 和 delete 在堆中分配和释放内存,不再使用 malloc() 和 free() 来分配和释放内存。
malloc()/free() 和 new/delete 这两对都用于动态地在堆中分配和释放内存,但是 new/delete 比 malloc()/free() 干了更多的事情。new/delete 具备对堆上所分配内存进行初始化和释放的能力,而这些能力是 malloc()/free() 所不具备的。
一般使用格式:
(1) 指针变量名 = new 类型标识符;
(2) 指针变量名 = new 类型标识符(初始值);
(3) 指针变量名 = new 类型标识符[内存单元个数];
初始化:
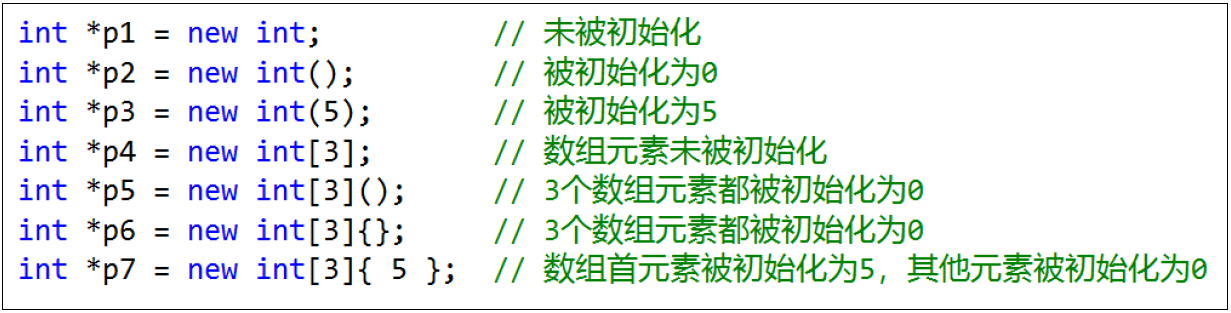
举例1:
#include<iostream>
using namespace std;
int main()
{
int* p = new int(18);
if (p != NULL)
{
cout << *p << endl;
delete p;
}
return 0;
}
举例2:
#include<iostream>
using namespace std;
int main()
{
int* pa = new int[100];
if (pa != NULL)
{
int* q = pa;
*q++ = 12;
*q++ = 18;
cout << *pa << endl;
cout << *(pa + 1) << endl;
delete[] pa;
}
return 0;
}
注意:delete 回收堆空间内存,表示这块堆空间内存可以重新被别人使用,但这块堆空间并不会被清零,指针也不会被置成 NULL。
#include <iostream>
using namespace std;
int main()
{
int* p = new int;
*p = 10;
delete p;
p = nullptr;
return 0;
}
注意:通过 new/delete 分配和释放的对象会调用其构造函数和析构函数。
#include <iostream>
using namespace std;
struct Person
{
int m_age;
Person()
{
cout << "Person::Person()" << endl;
}
~Person()
{
cout << "Person::~Person()" << endl;
}
void run()
{
cout << "Person::run() - " << m_age << endl;
}
};
int main()
{
Person* p = new Person;
p->m_age = 10;
p->run();
delete p;
return 0;
}
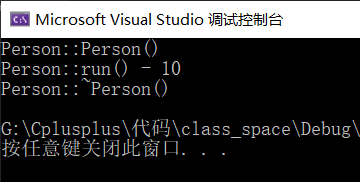
4.new/delete 探秘
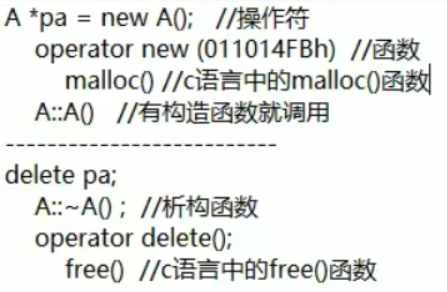
4.1 operator new() 和 operator delete()
operator new() 和 operator delete() 是函数。
new 干了两个事情:
- 在堆中分配内存:通过 operator new() 来分配内存
- 调用构造函数来初始化内存
delete 也干了两个事:
- 调用析构函数
- 释放内存:调用 operator delete() 来释放内存
4.2 new 记录分配的内存大小供 delete 使用
new 如何记录分配的内存大小供 delete 使用?
答:不同的编译器,new 内部有不同的实现方式。
int *p = new int;
delete p;
删除的时候,编译器怎么知道要回收的是 4 字节?
答:new 内部有记录机制,记录了分配出去多少内存。
4.3 new[]/delete[] 申请和释放一个数组
基本数据类型(内置类型)
举例1:
#include <iostream>
using namespace std;
int main()
{
int* p = new int(100);
delete p;
return 0;
}
举例2:
#include <iostream>
using namespace std;
int main()
{
int* p = new int[2];
delete[] p;
return 0;
}
自定义类型(类类型)
举例1:
#include <iostream>
using namespace std;
class A
{
public:
A()
{
cout << "A::A()" << endl;
}
~A()
{
cout << "A::~A()" << endl;
}
};
int main()
{
A* p = new A();
delete p;
return 0;
}
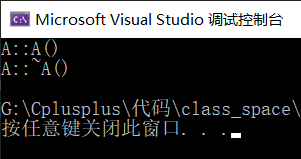
#include <iostream>
using namespace std;
class A
{
public:
A()
{
cout << "A::A()" << endl;
}
~A()
{
cout << "A::~A()" << endl;
}
};
int main()
{
A* p = new A[2]();
delete[] p;
return 0;
}
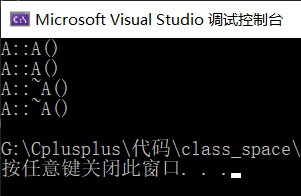
疑问:为什么给类型 A 对象数组动态分配内存时多出来 4 个字节,而给内置类型 int 数组动态分配内存时并没有多出来 4 字节?
在上面的程序中,对于类类型 A,调用了两次构造函数、两次析构函数,delete一个数组时,要为每一个数组元素调用析构函数。
但是,对于 delete 表达式,它并不知道数组的元素个数,只有 operator new() 函数和 operator delete() 函数知道。因此,必须有一种手段来告诉 delete 表达式的数组大小是多少。
那么,一种可行的方式就是,多分配一个大小为 4 字节的空间来记录数组大小,同时可以约定前 4 字节来记录大小。那么,由 operator new() 函数分配的地址与 new 表达式返回的地址应该相差 4 个字节。
当然,对于非类类型数组和不需要调用析构函数的类类型数组,这多余的 4 字节就不需要了。
4.4 new/delete、new[]/delete[] 要配对使用
举例1:
#include <iostream>
using namespace std;
int main()
{
int* pi = new int[3];
delete[] pi;
return 0;
}
举例2:
#include<iostream>
using namespace std;
class A
{
public:
A()
{
cout << "A::A()" << endl;
}
};
int main()
{
A* pa = new A[2]();
delete[] pa;
return 0;
}
举例3:
#include <iostream>
using namespace std;
class A
{
public:
A()
{
cout << "A::A()" << endl;
}
~A()
{
cout << "A::~A()" << endl;
}
};
int main()
{
A* pa = new A[2]();
delete[] pa;
return 0;
}
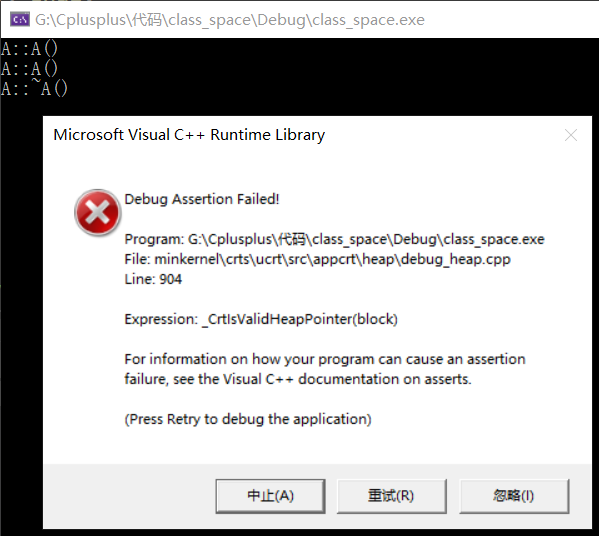
为什么自己一提供析构函数,不用 delete[] 来释放 new[] 出来的内存就报异常呢?
首先只调用了 1 次 A 的析构函数而不是 2 次,表示肯定有内存泄漏。真正释放内存的是 operator delete() 函数,而多出来的 4 个字节导致释放内存空间错乱。
结论:如果一个对象(内置对象、类对象),使用 new[] 来分配内存,却用单独的 delete (而不是 delete[] )来释放内存,那么这个对象需要满足的条件是:对象的类型要么是内置类型或者无自定义的析构函数的类类型。
|