首先先在此文件夹下创建data.txt
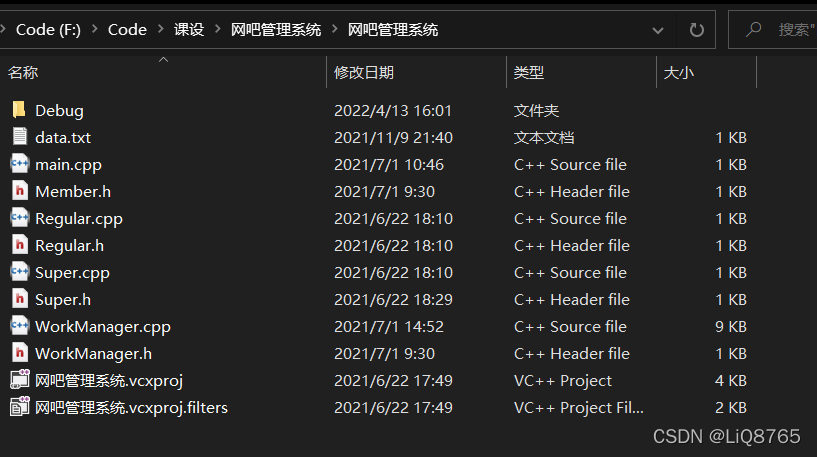
?
WorkManager.h
#pragma once
#include <iostream>
#include <Windows.h>
#include <fstream>
#include "Member.h"
#include "Regular.h"
#include "Super.h"
#define FILENAME "data.txt"
using namespace std;
class WorkManager
{
public:
WorkManager();
~WorkManager();
void Show_Menu();//展示菜单
void exitSystem();//退出系统
void Add_Mem();//添加会员
void save();//保存文件
int get_MemNum();//统计文件中人数
void init_Mem();//初始化会员
void Show_Mem();//显示会员
void Del_Mem();//删除会员
int IsExist(int number);//判断会员是否存在
void Mod_Mem();//修改会员
int m_MemNum;//记录会员人数
void Find_Mem();//查找会员
Member **m_MemArray;//会员数组指针
bool m_FileIsRmpty;//判断文件是否为空标志
};
WorkManager.cpp
#include "WorkManager.h"
WorkManager::WorkManager()
{
//1.文件不存在
ifstream ifs;
ifs.open(FILENAME, ios::in);//读文件
if (!ifs.is_open())
{
this->m_MemNum = 0;//初始化记录人数
this->m_MemArray = nullptr;//初始化数组指针
this->m_FileIsRmpty = true;//初始化文件是否为空
ifs.close();
return;
}
//2.文件存在 数据为空
char ch;
ifs >> ch;
if (ifs.eof())//代表文件为空
{
this->m_MemNum = 0;//初始化记录人数
this->m_MemArray = nullptr;//初始化指针数组
this->m_FileIsRmpty = true;//初始化文件是否为空
ifs.close();
return;
}
//3.文件存在 并且有数据记录
int num = this->get_MemNum();
this->m_MemNum = num;
this->m_MemArray = new Member*[this->m_MemNum];//开辟空间
this->init_Mem();//将文件中的数据保存到数组
}
WorkManager::~WorkManager()
{
if (this->m_MemArray != nullptr)
{
delete[]this->m_MemArray;
this->m_MemArray = nullptr;
}
}
void WorkManager::exitSystem()//退出系统
{
cout << "欢迎下次使用!" << endl;
system("pause");
exit(0);
}
void WorkManager::Add_Mem()//添加会员
{
cout << "请输入添加会员的数量:" << endl;
int addNum = 0;//保存会员的输入数量
cin >> addNum;
if (addNum > 0)
{
int newSize = this->m_MemNum + addNum;//新空间人数=原来记录人数+新增人数
Member **newSpace = new Member*[newSize];//开辟新空间
//将原来空间下的数据拷贝到新空间下
if (this->m_MemArray != nullptr)
{
for (int i = 0; i < this->m_MemNum; i++)
{
newSpace[i] = this->m_MemArray[i];
}
}
//批量增加新数据
for (int i = 0; i < addNum; i++)
{
int type;//会员类型
string name;//姓名
string sex;//性别
int age;//年龄
int number;//会员号
int integral;//积分
cout << "请输入第" << i + 1 << "个会员的姓名:" << endl;
cin >> name;
cout << "请输入第" << i + 1 << "个会员的性别:" << endl;
cin >> sex;
cout << "请输入第" << i + 1 << "个会员的年龄:" << endl;
cin >> age;
cout << "请输入第" << i + 1 << "个会员的会员号:" << endl;
cin >> number;
cout << "请输入第" << i + 1 << "个会员的积分:" << endl;
cin >> integral;
cout << "请选择该会员的类型:" << endl;
cout << "1.普通会员" << endl;
cout << "2.超级会员" << endl;
cin >> type;
Member *member = nullptr;
switch (type)
{
case 1:
member = new Regular(1, name, sex, age, number, integral);
break;
case 2:
member = new Super(2, name, sex, age, number, integral);
break;
default:
break;
}
//将创建会员类型保存到数组
newSpace[this->m_MemNum + i] = member;
}
delete[]this->m_MemArray;//释放原有的空间
this->m_MemArray = newSpace;//更改新空间的指向
this->m_MemNum = newSize;//更新新的会员人数
this->m_FileIsRmpty = false;//更新会员不为空标志
//成功添加后,保存到文件中
cout << "成功添加了:" << addNum << "名会员!" << endl;
this->save();//保存数据到文件中
}
else
{
cout << "输入数据有误!" << endl;
}
system("pause");
system("cls");
}
void WorkManager::save()//保存文件
{
ofstream ofs;
ofs.open(FILENAME, ios::out);//用写的方式打开文件(写文件)
//将每个人的数据写到文件中
for (int i = 0; i < this->m_MemNum; i++)
{
ofs << this->m_MemArray[i]->m_type << " "
<< this->m_MemArray[i]->m_name << " "
<< this->m_MemArray[i]->m_sex << " "
<< this->m_MemArray[i]->m_age << " "
<< this->m_MemArray[i]->m_number << " "
<< this->m_MemArray[i]->m_integral << endl;
}
ofs.close();//关闭文件
}
int WorkManager::get_MemNum()//统计文件中人数
{
ifstream ifs;
ifs.open(FILENAME, ios::in);//以读的方式打开文件
int type;//会员类型
string name;//姓名
string sex;//性别
int age;//年龄
int number;//会员号
int integral;//积分
int num = 0;
while (ifs >> type&&ifs >> name&&ifs >> sex&&ifs >> age&&ifs >> number&&ifs >> integral)
{
num++;
}
return num;
}
void WorkManager::init_Mem()//初始化会员
{
ifstream ifs;
ifs.open(FILENAME, ios::in);
int type;//会员类型
string name;//姓名
string sex;//性别
int age;//年龄
int number;//会员号
int integral;//积分
int index = 0;
while (ifs >> type&&ifs >> name&&ifs >> sex&&ifs >> age&&ifs >> number&&ifs >> integral)
{
Member *member = nullptr;
if (type == 1)//普通会员
{
member = new Regular(type, name, sex, age, number, integral);
}
else if (type == 2)
{
member = new Super(type, name, sex, age, number, integral);
}
this->m_MemArray[index] = member;
index++;
}
ifs.close();
}
void WorkManager::Show_Mem()//显示会员
{
if (this->m_FileIsRmpty)//判断文件是否为空
{
cout << "文件不存在或记录为空! " << endl;
}
else
{
for (int i = 0; i < m_MemNum; i++)
{
this->m_MemArray[i]->ShowInfo();//利用多态调用程序接口
}
}
system("pause");
system("cls");
}
int WorkManager::IsExist(int number)//判断会员是否存在
{
int index = -1;
for (int i = 0; i < this->m_MemNum; i++)
{
if (this->m_MemArray[i]->m_number == number)//找到会员
{
index = i;
break;
}
}
return index;
}
void WorkManager::Del_Mem()//删除会员
{
if (this->m_FileIsRmpty)
{
cout << "文件不存在或记录为空无法删除!" << endl;
}
else
{
cout << "请输入想要删除的会员号:" << endl;
int number;
cin >> number;
int index = this->IsExist(number);
if (index != -1)//说明会员存在,并且要删除掉index位置上的会员
{
for (int i = index; i < this->m_MemNum - 1; i++)
{
this->m_MemArray[i] = this->m_MemArray[i + 1];//数据前移
}
this->m_MemNum--;//更新数组中记录的人员个数
this->save();//同步更新到文件中
cout << "删除成功" << endl;
}
else
{
cout << "删除失败,未找到该职工!" << endl;
}
}
system("pause");
system("cls");
}
void WorkManager::Mod_Mem()//修改会员
{
if (this->m_FileIsRmpty)
{
cout << "文件不存在或记录为空!" << endl;
}
else
{
cout << "请输入要修改的会员号:" << endl;
int number;
cin >> number;
int ret = this->IsExist(number);
if (ret != -1)
{
delete this->m_MemArray[ret];
int newType;//会员类型
string newName;//姓名
string newSex;//性别
int newAge;//年龄
int newNumber;//会员号
int newIntegral;//积分
int dSelect = 0;
cout << "查找到会员号为:" << number << "的会员,请输入新的会员号:" << endl;
cin >> newNumber;
cout << "请输入新的姓名:" << endl;
cin >> newName;
cout << "请输入新的性别:" << endl;
cin >> newSex;
cout << "请输入新的年龄:" << endl;
cin >> newAge;
cout << "请输入新的积分:" << endl;
cin >> newIntegral;
cout << "请输入新的会员类型:" << endl;
cout << "1.普通会员" << endl;
cout << "2.超级会员" << endl;
cin >> dSelect;
Member *member = nullptr;
switch (dSelect)
{
case 1:
member = new Regular(1, newName, newSex, newAge, newNumber, newIntegral);
break;
case 2:
member = new Super(2, newName, newSex, newAge, newNumber, newIntegral);
break;
default:
break;
}
this->m_MemArray[ret] = member;//更新数据
cout << "修改成功!" << endl;
this->save();//保存到文件中
}
else
{
cout << "修改失败,查无此人!" << endl;
}
system("pause");
system("cls");
}
}
void WorkManager::Find_Mem()//查找会员
{
if (this->m_FileIsRmpty)
{
cout << "文件不存在或记录为空!" << endl;
}
else
{
cout << "请输入查找的方式:" << endl;
cout << "1.按会员号查找:" << endl;
cout << "2.按会员姓名查找:" << endl;
int select = 0;
cin >> select;
if (select == 1)//按照会员号查找
{
int number;
cout << "请输入查找的会员号:" << endl;
cin >> number;
int ret = IsExist(number);
if (ret != -1)
{
cout << "查找成功!该会员信息如下:" << endl;
this->m_MemArray[ret]->ShowInfo();
}
else
{
cout << "查找失败,查无此人" << endl;
}
}
else if (select == 2)
{
string name;
cout << "请输入查找的会员姓名:" << endl;
cin >> name;
bool flag = false;//加入判断是否查到的标志 默认为未找到
for (int i = 0; i < m_MemNum; i++)
{
if (this->m_MemArray[i]->m_name == name)
{
cout << "查找成功,会员号为:"
<< this->m_MemArray[i]->m_number
<< "会员信息如下:" << endl;
flag = true;
this->m_MemArray[i]->ShowInfo();//调用显示信息接口
}
}
if (flag == false)
{
cout << "查找失败,查无此人!" << endl;
}
}
else//输入有误
{
cout << "输入选项有误!" << endl;
}
}
system("pause");
system("cls");
}
void WorkManager::Show_Menu()//展示菜单
{
cout << "****************************************" << endl;
cout << "******** 欢迎使用网吧管理系统! *******" << endl;
cout << "*********** 0.退出管理程序 ***********" << endl;
cout << "*********** 1.增加会员信息 ***********" << endl;
cout << "*********** 2.显示会员信息 ***********" << endl;
cout << "*********** 3.删除会员信息 ***********" << endl;
cout << "*********** 4.修改会员信息 ***********" << endl;
cout << "*********** 5.查找会员信息 ***********" << endl;
cout << "****************************************" << endl;
cout << endl;
}
Super.h
#pragma once
#include <iostream>
#include "Member.h"
using namespace std;
class Super:public Member//超级会员
{
public:
Super(int type,string name, string sex, int age, int number, int integral);
virtual void ShowInfo();//显示个人信息
virtual string getType();//获取会员类型
};
Super.cpp
#include "Super.h"
Super::Super(int type, string name, string sex, int age, int number, int integral)
{
this->m_type = type;
this->m_name = name;
this->m_sex = sex;
this->m_age = age;
this->m_number = number;
this->m_integral = integral;
}
void Super::ShowInfo()//显示个人信息
{
cout << "会员类型:" << this->getType()
<< "会员名:" << this->m_name
<< "\t性别:" << this->m_sex
<< "\t年龄:" << this->m_age
<< "\t会员号:" << this->m_number
<< "\t积分:" << this->m_integral << endl;
}
string Super::getType()
{
return string("超级会员");
}
Regular.h
#pragma once
#include <iostream>
#include "Member.h"
using namespace std;
class Regular:public Member//普通会员
{
public:
Regular(int type,string name, string sex, int age, int number, int integral);
virtual void ShowInfo();//显示个人信息
virtual string getType();//获取会员类型
};
Regular.cpp
#include "Regular.h"
Regular::Regular(int type, string name, string sex, int age, int number, int integral)
{
this->m_type = type;
this->m_name = name;
this->m_sex = sex;
this->m_age = age;
this->m_number = number;
this->m_integral = integral;
}
void Regular::ShowInfo()//显示个人信息
{
cout << "会员类型:" << this->getType()
<< "会员名:" << this->m_name
<< "\t性别:" << this->m_sex
<< "\t年龄:" << this->m_age
<< "\t会员号:" << this->m_number
<< "\t积分:" << this->m_integral << endl;
}
string Regular::getType()
{
return string("普通会员");
}
Member.h
#pragma once
#include <string>
using namespace std;
class Member//会员
{
public:
virtual void ShowInfo() = 0;//显示个人信息
virtual string getType() = 0;//获取会员类型
int m_type;//会员类型
string m_name;//姓名
string m_sex;//性别
int m_age;//年龄
int m_number;//会员号
int m_integral;//积分
};
Main.cpp
#include <iostream>
#include <Windows.h>
#include "WorkManager.h"
#include "Member.h"
#include "Regular.h"
#include "Super.h"
using namespace std;
int main(void)
{
WorkManager wm;
int choice = 0;
while (1)
{
wm.Show_Menu();
cout << "请输入您的选择:" << endl;
cin >> choice;//接受用户的选项
switch (choice)
{
case 0://退出系统
wm.exitSystem();
break;
case 1://添加会员
wm.Add_Mem();
break;
case 2://显示会员
wm.Show_Mem();
break;
case 3://删除会员
wm.Del_Mem();
break;
case 4://修改会员
wm.Mod_Mem();
break;
case 5://查找会员
wm.Find_Mem();
break;
default:
system("cls");
break;
}
}
system("pause");
return 0;
}
运行结果:
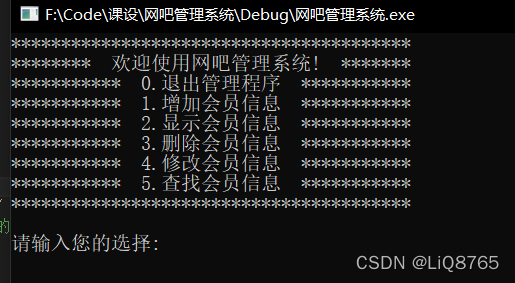
?实现增删改查功能
|