拷贝构造
class Object
{
private:
int value;
public:
Object(int x=0) :value(x)
{
cout << "Create Object:" << this << endl;
}
void Print()const
{
cout << value << endl;
}
~Object() {
cout << "Object::~Object" << this << endl;
}
};
int main()
{
int n;
cin >> n;
Object* s = new Object(n);
s->Print();
delete s;
Object* p = new Object[n];
for (int i = 0; i < n; ++i)
(p + i)->Print();
delete[]p;
return 0;
}
拷贝构造
在多种情况下会调用拷贝构造
class Object
{
private:
int value;
public:
Object(int x=0):value(x)
{
cout << "Create Object:" << this << endl;
}
void Print()const
{
cout << value << endl;
}
~Object() {
cout << "Object::~Object " << this << endl;
}
void SetValue(int x) { value = x; }
int GetValue() { return value; }
Object(const Object& obj) :value(obj.value)
{
cout << "Copy Creat " << this << endl;
}
};
Object fun(Object obj)
{
int val = obj.GetValue();
Object obja(val);
return obja;
}
int main() {
Object objx(0);
Object objy(0);
objy = fun(objx);
return 0;
}
构造析构对象过程
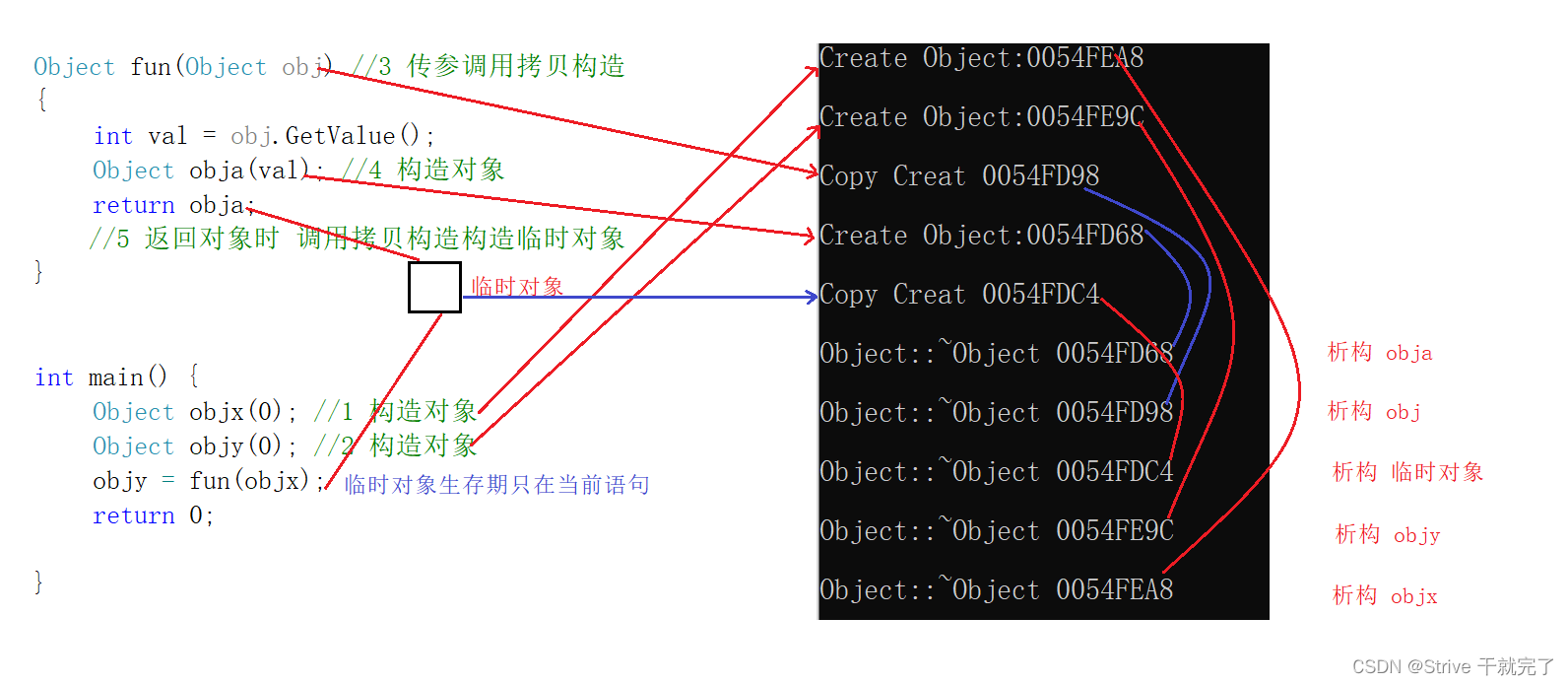
改造上面的代码 减少构建对象的次数
class Object
{
private:
int value;
public:
Object(int x=0):value(x)
{
cout << "Create Object:" << this << endl;
cout << endl;
}
void Print()const
{
cout << value << endl;
}
~Object() {
cout << "Object::~Object " << this << endl;
cout << endl;
}
int& Value()
{
return value;
}
const int& Value()const
{
return value;
}
Object(const Object& obj) :value(obj.value)
{
cout << "Copy Creat " << this << endl;
cout << endl;
}
};
Object fun(const Object &obj)
{
int val = obj.Value();
Object obja(val);
return obja;
}
int main() {
Object objx(0);
Object objy(0);
objy = fun(objx);
return 0;
}
深拷贝与浅拷贝
#define SEQ_INIT_SIZE 10
#define SEQ_INC_SIZE 2
class SeqList
{
int* data;
int maxsize;
int cursize;
public:
SeqList() :data(NULL), maxsize(SEQ_INIT_SIZE), cursize(0)
{
data = (int*)malloc(sizeof(int) * maxsize);
}
~SeqList()
{
free(data);
data = NULL;
}
SeqList(const SeqList& seq):maxsize(seq.maxsize),cursize(seq.cursize)
{
data = (int*)malloc(sizeof(int) * maxsize);
memcpy(data, seq.data, sizeof(data));
}
SeqList& operator=(const SeqList& seq)
{
if (this != &seq)
{
free(data);
maxsize = seq.maxsize;
cursize = seq.cursize;
data = (int*)malloc(sizeof(int) * maxsize);
memcpy(data, seq.data, sizeof(data));
}
return *this;
}
};
实现一个加法函数 尽量少构建对象
class Complex
{
private:
double Real, Image;
public:
Complex() :Real(0), Image(0) {}
Complex(double r, double i) :Real(r), Image(i) {}
~Complex() {}
Complex Add(const Complex& src)const {
double real = Real + src.Real;
double image = Image + src.Image;
return Complex(real, image);
}
void Print()const
{
cout << "Real=" << Real << endl;
cout << "Image=" << Image << endl;
}
};
int main()
{
Complex c1(1.2, 2.3);
Complex c2(4.5, 5.6);
Complex c3;
c3 = c1.Add(c2);
c3.Print();
}
1.以对象的引用返回不安全 不允许
Complex& Add(const Complex& src)const {
double real = Real + src.Real;
double image = Image + src.Image;
return Complex(real, image);
}
int main()
{
Complex c1(1.2, 2.3);
Complex c2(4.5, 5.6);
Complex c3;
c3 = c1.Add(c2);
c3.Print();
}
2.以对象返回
Complex Add(const Complex& src)const {
double real = Real + src.Real;
double image = Image + src.Image;
return Complex(real, image);
}
void Print()const
{
cout << "Real=" << Real << endl;
cout << "Image=" << Image << endl;
}
};
int main()
{
Complex c1(1.2, 2.3);
Complex c2(4.5, 5.6);
Complex c3;
c3 = c1.Add(c2);
c3.Print();
}
+运算符的重载
Complex operator+(const Complex& c)const {
double real = Real + c.Real;
double image = Image + c.Image;
return Complex(real, image);
}
void Print()const
{
cout << "Real=" << Real << endl;
cout << "Image=" << Image << endl;
}
};
int main()
{
Complex c1(1.2, 2.3);
Complex c2(4.5, 5.6);
Complex c3;
c3 = c1 + c2;
}
对于设计的任何一个类型 系统都会添加的6种缺省函数
class Object
{
public:
Object() {}
~Object() {}
Object(const Object& x) {}
Object& operator=(const Object& x)
{
return *this;
}
Object* operator&() { return this; }
const Object* operator&()const { return this; }
}
=运算符重载
class Object
{
private:
int value;
public:
Object(int x = 0) :value(x){cout << "Create Object:" << this << endl;}
~Object() { cout << "Object::~Object " << this << endl; }
Object(const Object& obj) :value(obj.value)
{
cout << "Copy Creat " << this << endl;
}
Object& operator=(const Object& obj) {
if (this != &obj) {
this->value = value;
}
return *this;
}
int& Value()
{
return value;
}
const int& Value()const
{
return value;
}
};
运算符重载
类和成员方法
class Int
{
private:
int value;
public:
Int(int x = 0) :value(x)
{
cout << "Creat Int: " << this << endl;
}
Int(const Int& it) :value(it.value)
{
cout << "Copy Creat Int:" << this << endl;
}
Int& operator=(const Int& it)
{
if (this != &it)
{
value = it.value;
}
return *this;
}
~Int()
{
cout << "Destroy Int:" << this << endl;
}
void Print()const
{
cout << value << endl;
}
};
&重载
Int* operator&()
{
return this;
}
---------------------------------------------------------
const Int* operator&()const
{
return this;
}
前置++和后置++重载
Int& operator++()
{
++value;
return *this;
}
---------------------------------------------------------
Int operator++(int)
{
Int old = *this;
++* this;
return old;
}
前置–和后置–重载
Int& operator--() {
this->value--;
return *this;
}
Int operator--(int)
{
Int old = *this;
--* this;
return old;
}
+重载
Int operator+(const Int& it)const
{
return Int(this->value + it.value);
}
c = a + 10;
c = 10 + a;
---------------------------------------------------------
Int operator+(const int x)const
{
return Int(this->value + x);
}
---------------------------------------------------------
Int operator+(const int x, const Int& it)
{
return it + x;
}
==与!=重载
bool operator==(const Int& it)const
{
return this->value == it.value;
}
---------------------------------------------------------
bool operator!=(const Int& it)const
{
return !(*this == it);
}
< ,>=, >, <=重载
bool operator<(const Int& it)const
{
return this->value < it.value;
}
---------------------------------------------------------
bool operator>=(const Int& it)const
{
return !(*this < it);
}
---------------------------------------------------------
bool operator>(const Int& it)const
{
return this->value > it.value;
}
---------------------------------------------------------
bool operator<=(const Int& it)const
{
return !(*this > it);
}
例子
int main()
{
int i = 0;
i = i++ + 1;
cout << i << endl;
Int a = 0;
a = a++ + 1;
a.Print();
}
补充:构造函数具有类型转换功能 内置类型强转为对象
构造函数具有此功能的前提是:构造函数只有单个参数 或者(有多个参数 但是除一个参数无默认值 其它参数都有默认值)
int main()
{
Int a(10);
int b=100;
a=b;
}
---------------------------------------------------------
explicit Int(int x = 0) :value(x)
{
cout << "Creat Int: " << this << endl;
}
int main()
{
Int a(10);
int b=100;
a=(Int)b;
}
---------------------------------------------------------
Int(int x,int y=0):value(x+y)
{
cout<<"Create Int: "<<this<<endl;
}
强转运算符重载 对象强转为内置类型
operator int()const
{
return value;
}
完整代码
class Int
{
private:
int value;
public:
explicit Int(int x = 0) :value(x)
{
cout << "Creat Int: " << this << endl;
}
Int(const Int& it) :value(it.value)
{
cout << "Copy Creat Int:" << this << endl;
}
Int& operator=(const Int& it)
{
if (this != &it)
{
value = it.value;
}
cout << this << "=" << &it << endl;
return *this;
}
~Int()
{
cout << "Destroy Int:" << this << endl;
}
void Print()const
{
cout << value << endl;
}
Int* operator&()
{ return this; }
const Int* operator&()const
{ return this; }
Int& operator++()
{
++value;
return *this;
}
Int operator++(int)
{
Int old = *this;
++* this;
return old;
}
Int& operator--() {
this->value--;
return *this;
}
Int operator--(int)
{
Int old = *this;
--* this;
return old;
}
Int operator+(const Int& it)const
{
return Int(this->value + it.value);
}
Int operator+(const int x)const
{
return Int(this->value + x);
}
bool operator==(const Int& it)const
{
return this->value == it.value;
}
bool operator!=(const Int& it)const
{
return !(*this == it);
}
bool operator<(const Int& it)const
{
return this->value < it.value;
}
bool operator>=(const Int& it)const
{
return !(*this < it);
}
bool operator>(const Int& it)const
{
return this->value > it.value;
}
bool operator<=(const Int& it)const
{
return !(*this > it);
}
operator int()const
{
return value;
}
};
Int operator+(const int x, const Int& it)
{
return it + x;
}
()运算符重载
class Add
{
private:
mutable int value;
public:
Add(int x = 0) :value(x) {}
int operator()(int a, int b)const
{
value = a + b;
return value;
}
};
int main()
{
int a = 10, b = 20, c = 0;
Add add;
c = add(a, b);
c = Add()(a, b);
return 0;
}
*与->重载
class Int
{
private:
int value;
public:
Int(int x=0) :value(x)
{
cout << "Creat Int: " << this << endl;
}
Int(const Int& it) :value(it.value)
{
cout << "Copy Creat Int:" << this << endl;
}
Int& operator=(const Int& it)
{
if (this != &it)
{
value = it.value;
}
cout << this << "=" << &it << endl;
return *this;
}
~Int()
{
cout << "Destroy Int:" << this << endl;
}
int& Value() { return value; }
const int& Value()const { return value; }
};
class Object
{
Int* ip;
public:
Object(Int* s = NULL) :ip(s) {}
~Object()
{
if (ip != NULL)
{
delete ip;
}
ip = NULL;
}
Int& operator*()
{
return *ip;
}
const Int& operator*()const
{
return *ip;
}
Int* operator->()
{
return &**this;
}
const Int* operator->()const
{
return &**this;
}
};
int main()
{
Object obj(new Int(10));
Int* p = new Int(1);
(*p).Value();
(*obj).Value();
p->Value();
obj->Value();
delete p;
}
类String << + 运算符重载
class String
{
char* str;
String(char* p, int)
{
str = p;
}
public:
String(const char* p = NULL) :str(NULL)
{
if (p != NULL)
{
str = new char[strlen(p) + 1];
strcpy_s(str, strlen(p) + 1,p);
}
else
{
str = new char[1];
*str = '\0';
}
}
~String()
{
if (str != NULL)
{
delete[]str;
}
str = NULL;
}
ostream& operator<<(ostream& out)const
{
if (str != NULL)
{
out << str;
}
return out;
}
String(const String& src):str(NULL)
{
str = new char[strlen(src.str) + 1];
strcpy_s(str, strlen(src.str) + 1, src.str);
}
String& operator=(const String& s)
{
if (this != &s)
{
delete[]str;
str = new char[strlen(s.str) + 1];
strcpy_s(str, strlen(s.str) + 1, s.str);
}
return *this;
}
String operator+(const String& s)const
{
char* p = new char[strlen(this->str) + strlen(s.str) + 1];
strcpy_s(p, strlen(str) + 1, str);
strcat_s(p, strlen(p) + strlen(s.str) + 1, s.str);
return String(p,1);
}
String operator+(const char* s)const
{
char* p = new char[strlen(this->str) + strlen(s) + 1];
strcpy_s(p, strlen(str) + 1, str);
strcat_s(p, strlen(p) + strlen(s) + 1, s);
return String(p, 1);
}
};
String operator+(const char* s, const String& src)
{
return String(s) + src;
}
ostream& operator<<(ostream& out, const String& s)
{
s << out;
return out;
}
int main()
{
String s1("yhping");
String s2("hello");
String s3;
s3 = s1 + s2;
s3 = s1 + "newdata";
s3 = "newdata" + s1;
cout << s3 << endl;
}
如何使得堆内空间多次利用 而不产生不良影响
左值右值的的定义
int main() {
int a = 10;
int& b = a;
int&& c=10;
String s1;
String& sx=s1;
String&& sy=String("hello");
}
使用 移动拷贝构造函数 和移动赋值函数
String(String&& s)
{
cout << "move copy constructor" << this << endl;
str = s.str;
s.str = NULL;
}
String& operator=(String&& s)
{
if (this != &s)
{
str = s.str;
s.str = NULL;
}
cout << "move operator=: " << &s << endl;
return *this;
}
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-qa6BJtMF-1650035516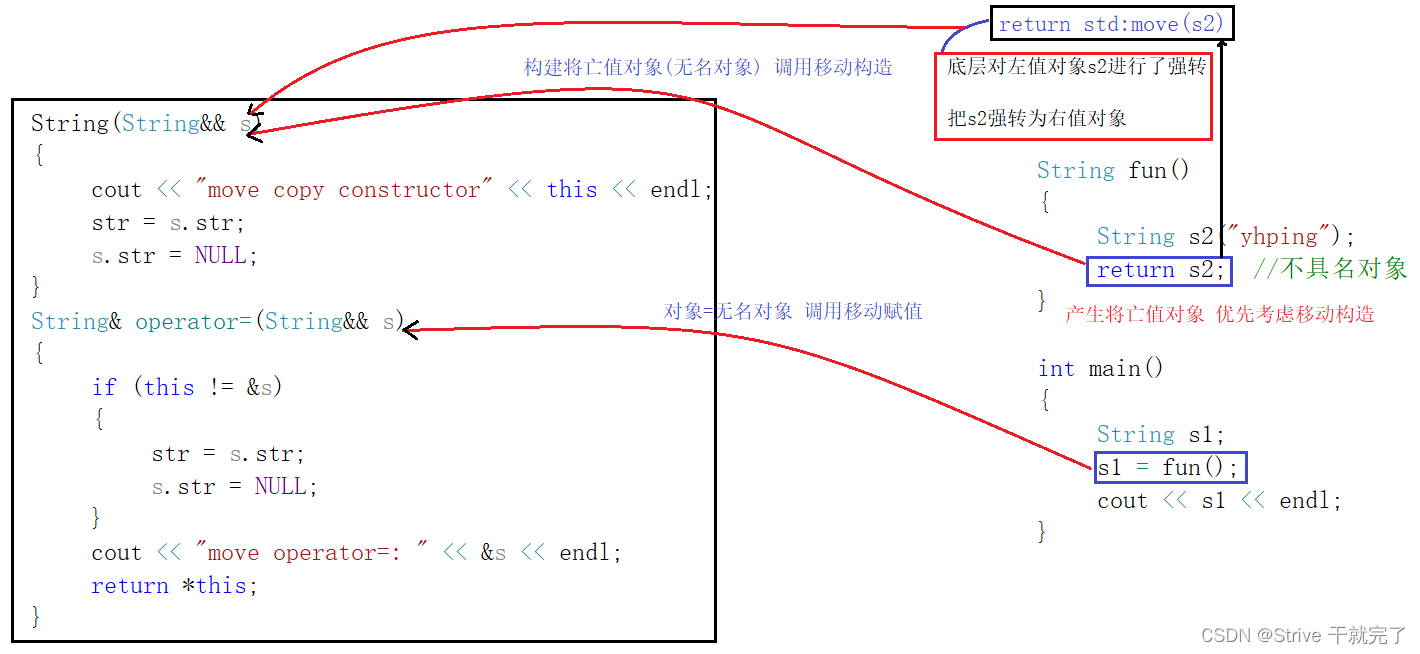 142)(image-20220125124203755.png)]
类String完整代码
class String
{
char* str;
String(char* p, int)
{
str = p;
}
public:
String(const char* p = NULL) :str(NULL)
{
if (p != NULL)
{
str = new char[strlen(p) + 1];
strcpy_s(str, strlen(p) + 1,p);
}
else
{
str = new char[1];
*str = '\0';
}
}
~String()
{
if (str != NULL)
{
delete[]str;
}
str = NULL;
}
ostream& operator<<(ostream& out)const
{
if (str != NULL)
{
out << str;
}
return out;
}
String(const String& src):str(NULL)
{
str = new char[strlen(src.str) + 1];
strcpy_s(str, strlen(src.str) + 1, src.str);
}
String& operator=(const String& s)
{
if (this != &s)
{
delete[]str;
str = new char[strlen(s.str) + 1];
strcpy_s(str, strlen(s.str) + 1, s.str);
}
return *this;
}
String operator+(const String& s)const
{
char* p = new char[strlen(this->str) + strlen(s.str) + 1];
strcpy_s(p, strlen(str) + 1, str);
strcat_s(p, strlen(p) + strlen(s.str) + 1, s.str);
return String(p,1);
}
String operator+(const char* s)const
{
char* p = new char[strlen(this->str) + strlen(s) + 1];
strcpy_s(p, strlen(str) + 1, str);
strcat_s(p, strlen(p) + strlen(s) + 1, s);
return String(p, 1);
}
String(String&& s)
{
cout << "move copy constructor" << this << endl;
str = s.str;
s.str = NULL;
}
String& operator=(String&& s)
{
if (this != &s)
{
delete[]str;
str = s.str;
s.str = NULL;
}
cout << "move operator=: " << &s << endl;
return *this;
}
};
String operator+(const char* s, const String& src)
{
return String(s) + src;
}
ostream& operator<<(ostream& out, const String& s)
{
s << out;
return out;
}
String fun()
{
String s2("yhping");
return s2;
}
int main()
{
String s1;
s1 = fun();
cout << s1 << endl;
}
类成员中有其它类对象 它们之间构造和析构的调用顺序
对象中成员的构建顺序和声明的顺序保持一致,与参数列表无关
1.在函数体中对类的成员进行赋值
class Int
{
private:
int value;
public:
Int(int x=0) :value(x)
{
cout << "Creat Int: " << this << endl;
}
Int(const Int& it) :value(it.value)
{
cout << "Copy Creat Int:" << this << endl;
}
Int& operator=(const Int& it)
{
if (this != &it)
{
value = it.value;
}
cout << this << "=" << &it << endl;
return *this;
}
~Int()
{
cout << "Destroy Int:" << this << endl;
}
};
class Object
{
private:
int num;
Int val;
public:
Object(int x, int y)
{
cout << "Creat Object: " << this << endl;
num = x;
val = y;
}
~Object()
{
cout << "Destroy Object: " << this << endl;
}
};
int main()
{
Object obj(1, 2);
}
运行结果
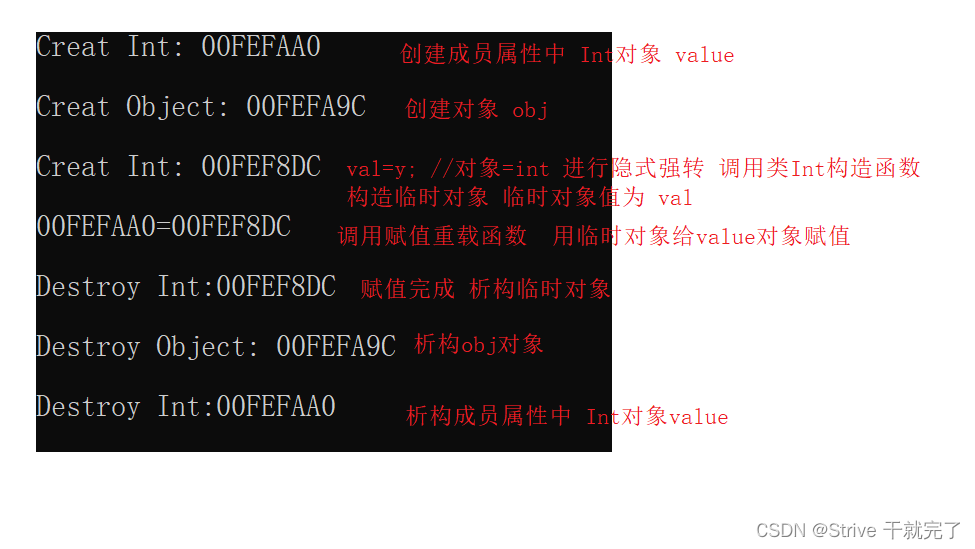
2.在初始化列表中对类的成员进行赋值
Object(int x, int y):num(x),val(y)
{
cout << "Creat Object: " << this << endl;
}
运行结果
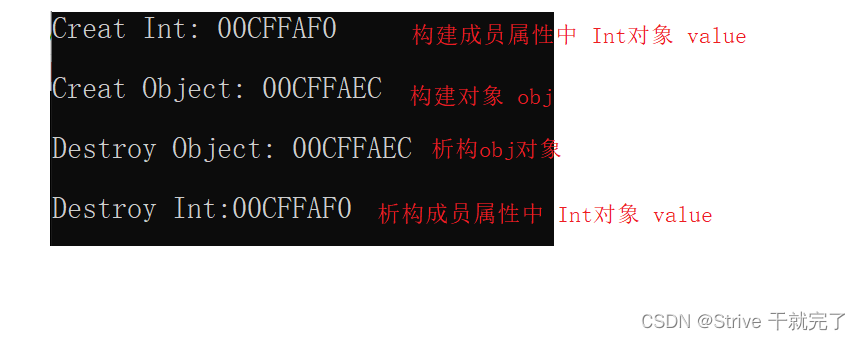
|