lambda 表达式简介
lambda表达式是C++11最重要也最常用的一个特性之一,其实在C#3.5中就引入了lambda,Java8中也引入了lambda表达式
lambda 来源于函数式编程的概念,也是现在编程语言的一个特点,有如下优点:
- 声明式编程风格:就地匿名定义目标函数或函数对象,不需要额外写一个命名函数或者对象,以更直接的方式去写程序,好的可读性和可维护性
- 简洁:不需要额外再写一个函数或者函数对象,避免了代码膨胀和功能分散,让开发者更加集中精力在手边的问题,同时也获取了更高的生产率
- 在需要的时间和地点实现功能闭包,使程序更灵活
lambda 表达式的基本概念和基本用法
lambda表达式定义了一个匿名函数,并且可以捕获一定范围内的变量,lambda表达式的语法形式可简单归纳如下:
[caoture] (params) opt -> ret {body;};
其中:capture是捕获列表;params是参数表;opt是函数选项;ret是返回值类型;body是函数体,因此一个完整的lambda表达式看起来就像这样:
int main()
{
auto f = [](int a) -> int {return a + 1; };
cout << f(1) << endl;
}
int main()
{
auto f = [](int a) {return a; };
auto f = [](int a) ->std::initializer_list<int> {return { a,1 }; };
}
lambda 表达式可以通过获取列表捕获一定范围内的变量:
- [] 不捕获任何变量
- [&] 捕获外部作用域中所有变量,并作为引用在函数体中使用(按引用捕获)
- [=] 捕获外部作用域中所有变量,并作为副本在函数体中使用(按值捕获)
- [=,&foo] 按值捕获外部作用域中所有变量,并按引用获取foo变量
- [bar] 按值捕获bar变量,同时不捕获其他变量
- [this] 捕获当前类中的this指针,让lambda表达式拥有和当前类成员同样的访问权限,如果已经使用了&或者=,就默认添加此选项,捕获this的目的是可以在lambda中使用当前类的成员函数和成员变量
int g_max = 10;
int add(int x, int y)
{
return x + y;
}
int fun(int x)
{
int sum = 10;
int num = 20;
auto f1 = [](int a) -> int {return a + g_max; };
auto f2 = [&](int a) -> int
{
x = a;
sum += 10;
num = a + x;
return num;
};
list<int> ar = { 12,23,34,45,56,67,78,89 };
ar.sort([&](int a, int b)->bool {return a < b; });
return 0;
}
int main()
{
list<int> ar = { 34,23,45,67,12,56,78,89 };
ar.sort([](int a, int b)->bool {return a > b; });
for (auto& x : ar)
{
cout << x << endl;
}
}
从下面代码,捕获方式的不同可以看出,编译时就进行了捕获
class F1
{
int& x;
public:
F1(int& a) :x(a) {}
};
class F2
{
int x;
public:
F2(int a) :x(a) {}
int operator()(int a)const
{
}
};
int main()
{
int x = 0;
auto f1 = [&](int a) -> int
{
x += 10;
cout << x << endl;
return x;
};
auto f2 = [=](int a)->int
{
return x;
};
x = 100;
cout << f1(0) << endl;
cout << f2(0) << endl;
return 0;
}
在下面的代码中,x2 按值进行捕获,可以对a,和g_max进行捕获,并且对this指针进行了捕获,继而可以去使用value
int g_max = 10;
class Object
{
int value;
public:
Object(int x = 0) :value(x) {}
void func(int a, int b)
{
auto x0 = []()->int {return g_max; };
auto x1 = []()->int {return a; };
auto x2 = [=]()->int
{
int x = value;
return x + a + g_max;
};
}
}
虽然我们上面说了,按值捕获在某种意义上是一种常量,但是此处捕获的实际为this指针,所以可以对value值进行修改
auto x3 = [=]() ->int
{
value += 10;
int x = value;
return x + a + g_max;
};
mutable
class Object
{
int value;
mutable int num;
public:
Object(int x = 0,int y = 0):value(x),num(y){}
int fun()const
{
num += 10;
return value + num;
}
};
int main()
{
int a = 0;
auto f1 = [=] {return a++; };
auto f2 = [=]() mutable {return a++; };
}
mutable关键字后,由于捕获为[=],所以lambda表达式中存放的是一个副本
class F1
{
int _a;
public:
F1(int x) :_a(x){}
int operator()() const
{
_a += 10;
return a;
}
};
int main()
{
int a = 10;
auto f1 = [=]()mutable->int
{
a += 10;
return a;
};
a += 100;
cout << "f1:" << f1() << endl;
cout << "a:" << a << endl;
}

函数,仿函数,lambda表达式
template<class _BI, class _Fn>
void my_for_each(_BI _first, _BI _last, _Fn _func)
{
cout << typeid(_Fn).name() << endl;
for (; _first != _last; ++_first)
{
_func(*_first);
}
}
void Print_Ar(int x)
{
printf(" %d ", x);
}
struct Print
{
void operator()(int x) const
{
printf(" %d ", x);
}
};
int main()
{
std::vector<int> ar = { 12,23,34,45,56,67,78 };
my_for_each(ar.begin(), ar.end(), Print_Ar);
cout << endl;
my_for_each(ar.begin(), ar.end(), Print());
cout << endl;
my_for_each(ar.begin(), ar.end(), [](int a)->void {printf(" %d ", a); });
return 0;
}
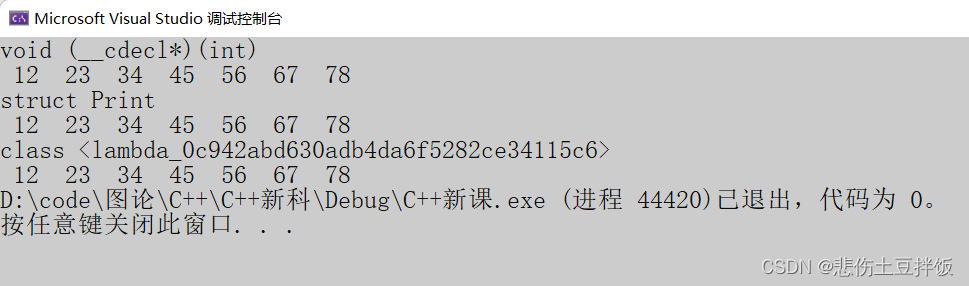 lambda表达式其实就是仿函数的语法糖
|