二叉树结构体,代码:
struct node{
int data;
struct node* lchild;
struct node* rchild;
};
写结构体的时候,脑子里要浮现这张图:
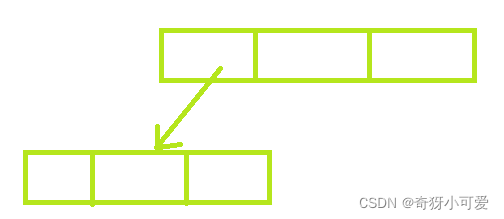
中间的那个是data,放树里面的数据,struct node*,其中*表示指向,struct node表示同样的node结构。
然后是用先序遍历创建二叉树:
void pre_cre(tree &bt){
char a;
a=getchar();
if(a!='#'){
bt = (tree)malloc(sizeof(tree));
bt->data=a;
pre_cre(bt->lchild);
pre_cre(bt->rchild);
}
else{
bt=NULL;
}
}
先回顾一下getchar()的知识点。
①getchar()读取字符
#include<bits/stdc++.h>
using namespace std;
int main(){
char c=getchar();
printf("%c",c);
}
结果:
?
②getchar()读取字符串。
第一次getchar()的时候人工输入字符串,以后每次读取的时候会直接从缓存区读取。
#include<bits/stdc++.h>
using namespace std;
int main(){
char c=getchar();
printf("%c",c);
printf("\n");
char b=getchar();
printf("%c",b);
printf("\n");
c=getchar();
printf("%c",c);
}
结果:
?
?二叉树的前中后序遍历代码:
void xianxu(tree bt){
if(bt==NULL) return;
cout<<bt->data<<" ";
xianxu(bt->lchild);
xianxu(bt->rchild);
}
void zhongxu(tree bt){
if(bt==NULL) return;
zhongxu(bt->lchild);
cout<<bt->data<<" ";
zhongxu(bt->rchild);
}
void houxu(tree bt){
if(bt==NULL) return;
houxu(bt->lchild);
houxu(bt->rchild);
cout<<bt->data<<" ";
}
完整代码:
#include<bits/stdc++.h>
using namespace std;
struct node{
char data;
struct node* lchild;
struct node* rchild;
};
typedef struct node btnode;
typedef struct node* tree;
void pre_cre(tree &bt){
char a;
a=getchar();
if(a!='#'){
bt = (tree)malloc(sizeof(tree));
bt->data=a;
pre_cre(bt->lchild);
pre_cre(bt->rchild);
}
else{
bt=NULL;
}
}
void xianxu(tree bt){
if(bt==NULL) return;
cout<<bt->data<<" ";
xianxu(bt->lchild);
xianxu(bt->rchild);
}
void zhongxu(tree bt){
if(bt==NULL) return;
zhongxu(bt->lchild);
cout<<bt->data<<" ";
zhongxu(bt->rchild);
}
void houxu(tree bt){
if(bt==NULL) return;
houxu(bt->lchild);
houxu(bt->rchild);
cout<<bt->data<<" ";
}
int main(void){
tree root=NULL;
pre_cre(root);
cout<<endl;
xianxu(root);
cout<<endl;
zhongxu(root);
cout<<endl;
houxu(root);
cout<<endl;
}
|