// dllmain.cpp : 定义 DLL 应用程序的入口点。
#include "pch.h"
BOOL APIENTRY DllMain( HMODULE hModule,
DWORD ul_reason_for_call,
LPVOID lpReserved
)
{
switch (ul_reason_for_call)
{
case DLL_PROCESS_ATTACH:
::MessageBox(NULL, L"if you see this, testDll.dll had been inject in this Process", L"hi", MB_ICONINFORMATION | MB_OK);
case DLL_THREAD_ATTACH:
case DLL_THREAD_DETACH:
case DLL_PROCESS_DETACH:
break;
}
return TRUE;
}
4. 注入代码
// RemoteInject.cpp : 定义应用程序的入口点。
//
#include "framework.h"
#include <shellapi.h>
#include <tlhelp32.h>
#include <string>
#include <thread>
DWORD processFind(LPCWSTR exeName)
{
HANDLE hProcess = CreateToolhelp32Snapshot(TH32CS_SNAPPROCESS, NULL);
if(!hProcess)
return 0;
PROCESSENTRY32 info;
info.dwSize = sizeof(PROCESSENTRY32);
if(!Process32First(hProcess, &info))
return 0;
while(true)
{
if(!wcscmp(info.szExeFile, exeName))
return info.th32ProcessID;
if(!Process32Next(hProcess, &info))
return 0;
}
return 0;
}
BOOL Inject(DWORD processId, LPCWSTR dllPath)
{
HANDLE hProcess = OpenProcess(PROCESS_ALL_ACCESS, TRUE, processId);
if(!hProcess)
return FALSE;
SIZE_T pathSize = (wcslen(dllPath) + 1) * sizeof(wchar_t);
LPVOID startAddr = VirtualAllocEx(hProcess, NULL, pathSize, MEM_COMMIT, PAGE_READWRITE);
if(!startAddr)
return FALSE;
if(!WriteProcessMemory(hProcess, startAddr, dllPath, pathSize, NULL))
return FALSE;
PTHREAD_START_ROUTINE pFuncStartAddr = (PTHREAD_START_ROUTINE)GetProcAddress(GetModuleHandle(L"kernel32.dll"), "LoadLibraryW");
if(!pFuncStartAddr)
return FALSE;
HANDLE hThread = CreateRemoteThreadEx(hProcess, NULL, NULL, pFuncStartAddr, startAddr, NULL, NULL, NULL);
if(!hThread)
return FALSE;
WaitForSingleObject(hThread, INFINITE);
CloseHandle(hThread);
CloseHandle(hProcess);
return TRUE;
}
int APIENTRY wWinMain(_In_ HINSTANCE hInstance,
_In_opt_ HINSTANCE hPrevInstance,
_In_ LPWSTR lpCmdLine,
_In_ int nCmdShow)
{
//运行windows自带的记事本程序
ShellExecute(0, L"open", L"notepad.exe", L"", L"", SW_SHOWNORMAL);
bool b = false;
std::thread th([&]() {
::Sleep(100);
DWORD id = processFind(L"notepad.exe");
if (!id)
{
b = true;
return;
}
wchar_t szPath[MAX_PATH];
if (!GetModuleFileNameW(NULL, szPath, MAX_PATH))
{
b = true;
return;
}
std::wstring str = szPath;
size_t pos = str.find_last_of(L"\\");
str = str.substr(0, pos + 1);
str += L"testDll.dll";
Inject(id, str.c_str());
b = true;
});
th.detach();
while (!b)
{
std::this_thread::yield();
}
return 0;
}
5. 界面显示
?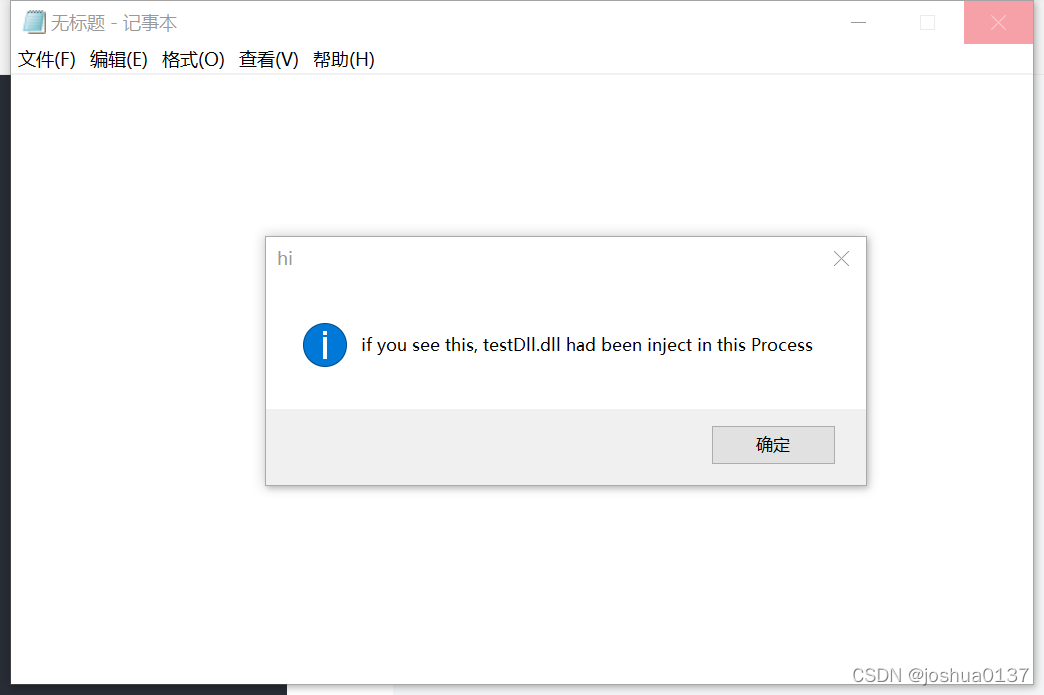
?