overloaded operators? 重载几乎所有的运算符?
-
allows user-defined types to act like built in types -
another way to make a function call -
unary and binary operators can be overloaded: + - * / % ^(异或) & | ~
= < > += -= *= /= %=
^= &= |= << >> <<= >>= ==
!= <= >= ! && || ++ --
, ->* -> () [] operator new operator delete operator new[] operator delete[]
operators you can't overload 不能重载
. .* :: ?:
sizeof typeid
static_cast dynamic_cast const_cast
reinterpret_cast
Restrictions? ?限制条件
- only existing operators can be overloaded(you can't create a ** operator for exponentiation)? 已经存在的运算符可以被重载
- operators must be overloaded on a class or enumeration type??
- overloaded operators must:
- preserve number of operands? ?保持原有操作数的个数
- preserve precedence(优先级)? 保持优先级
c++ overloaded operator
-
just a function with an operator name!? 写一个函数
-
can be a member function? 成员函数
const String String::operator +(const String& that); //this + that -
can be a global (free) function? 全局函数
const String operator +(const String& r,const String& l);
how to overload
- an member function? ? 成员函数
- implicit first argument? ? this是第一个参数
- no type conversion performed on receiver(运算符左值)? ??
- must have access to class definition
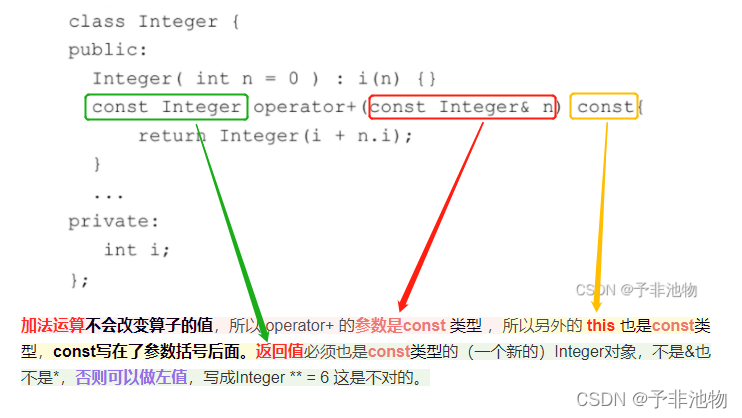
在编译的时候,能看到operator+的函数体默认变成内联函数,而且返回值只是为了能返回,在函数里并没有用,所以在这个函数里的拷贝构造是被优化的。
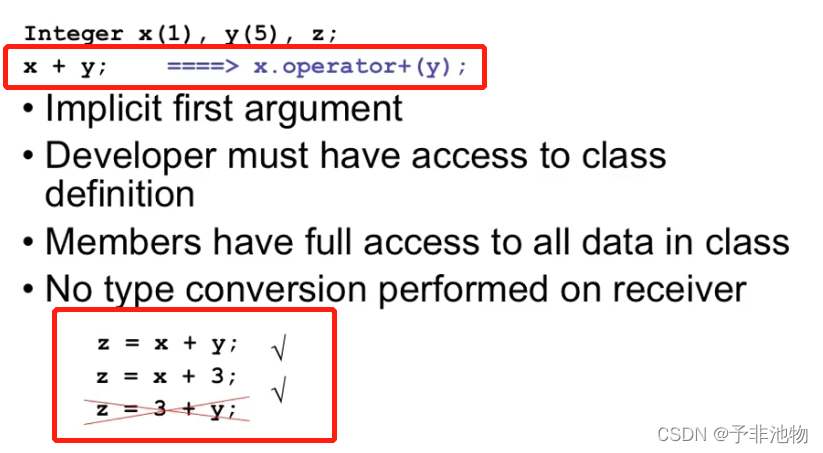
使用成员函数,编译器从左到右扫描,看用谁的运算符。
1. x + y, ? ? ? ?编译器从左到右扫描,用x的operator+,两个intrger类型;√
2. z = x + 3, ?编译器从左到右扫描,用x的operator+,integer里面有函数将int 3转化为integer的类型(构造函数);√
3. z = 3 + y, ? ?编译器从左到右扫描,用int 3的+,integer里面没有将nteger的类型转化为int类型的函数;×
4. z = x + 3.5 ,用x的operator+,double 到 int 不能自动转换,需要强制类型转换??×?
-
for binary operaters(+,-,*,etc) member functions require one argument.? 二元运算符需要一个参数 -
for unary operators (unary -,!,etc) member functions require no arguments:? ?一元不改自己,制造出来一个新的对象来 const Integer operator-() const{
return Integer(-i);
}
...
z=-x; //z.operator=(x.oprator-());
?operator- ,this仍然是const,不改自己,产生一个新的对象。
- as a global function? ?两个算子都要写上
- explicit first argument
- type conversions performed on both arguments
- can be made a friend
- type conversions performed on both arguments
global operators(friend)
class Integer{
friend const Integer operator+(const Integer&rhs,const Integer& lhs); //
...
}
const Integer operator+(const Integer&rhs,const Integer& lhs){ // 全局函数
return Integer(lhs.i,rhs.i);
}
- if you don't have access to private data members, then the global function must use the pubic interface
tips: members vs. free functions
-
unary operators should be members 单目的应该是成员的 -
= () -> ->* must be members 必须 -
assignment operators should be members -
all other binary operators as non-members 非成员的
|