C++Primer第五版 ——— (ch1)课后习题参考答案
练习 1.3
编写程序,在标准输出上打印Hello, World。
#include <iostream>
using namespace std;
int main()
{
char arr[10] = { 0 };
char arr0[10] = { 0 };
char arr1[] = "hello, world";
cin >> arr;
cin >> arr0;
cout << arr << " " << arr0 << endl;
cout << arr1 << endl;
return 0;
}
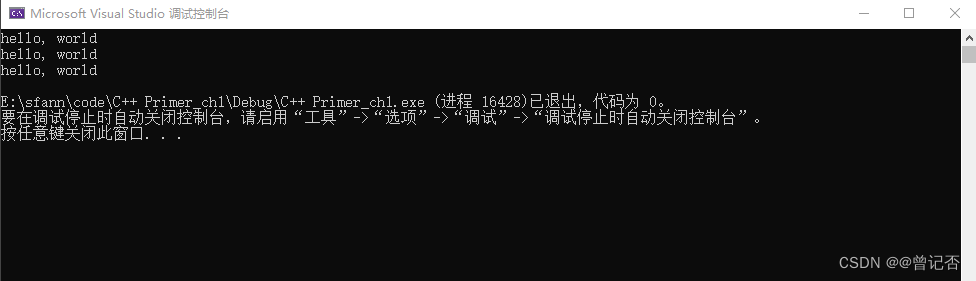
练习 1.4
我们的程序使用加法运算符+来将两个数相加。编写程序使用惩罚运算符*,来打印两个数的积。
#include <iostream>
using namespace std;
int main()
{
int a = 0, b = 0;
cout << "please enter two number:";
cin >> a >> b;
cout << "the sum of " << a << " and " << b << " is " << a + b << endl;
cout << "the multiply of " << a << " and " << b << " is " << a * b << endl;
return 0;
}
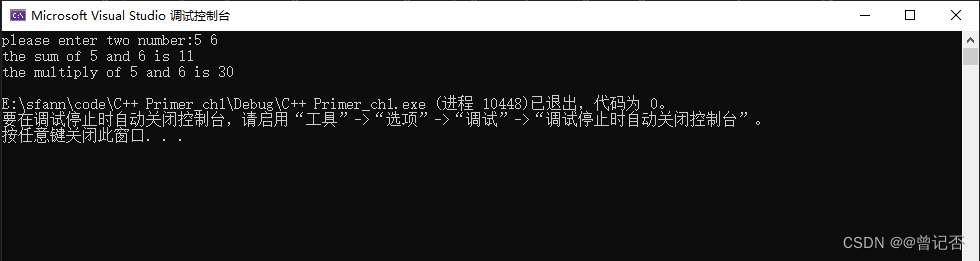
练习1.5
我们将所有输出放在一条很长的语句中。重写程序,将每个运算对象的打印操作放在一条独立的语句中。
#include <iostream>
using namespace std;
int main()
{
int a = 0;
int b = 0;
cout << "please enter two number:";
cin >> a;
cin >> b;
cout << "the sum of ";
cout << a;
cout << " and ";
cout << b;
cout << " is ";
cout << a + b;
cout << endl;
cout << "the multiply of ";
cout << a;
cout << " and ";
cout << b;
cout << " is ";
cout << a * b;
cout << endl;
return 0;
}
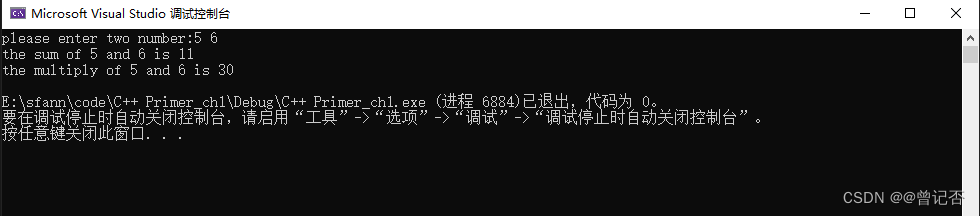
练习1.6
解释下面的程序片段是否合法。
std::cout << "The sum of " << v1;
<< "and " << v2;
<< " is " << v1+v2 << std::endl;
不合法。前两行的末尾有分号,代表语句结束,第2、3两行为两条新的语句。而这两条语句在“<<”之前缺少了输出流,应在“<<”之前加上"cout "。 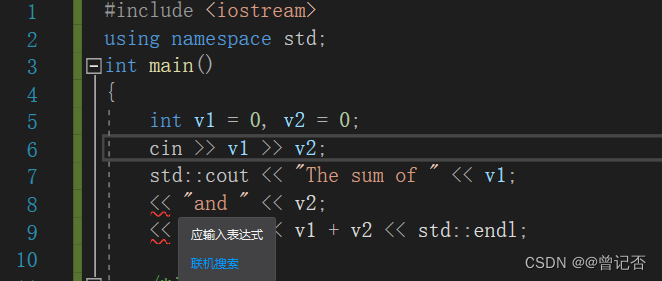 改为: 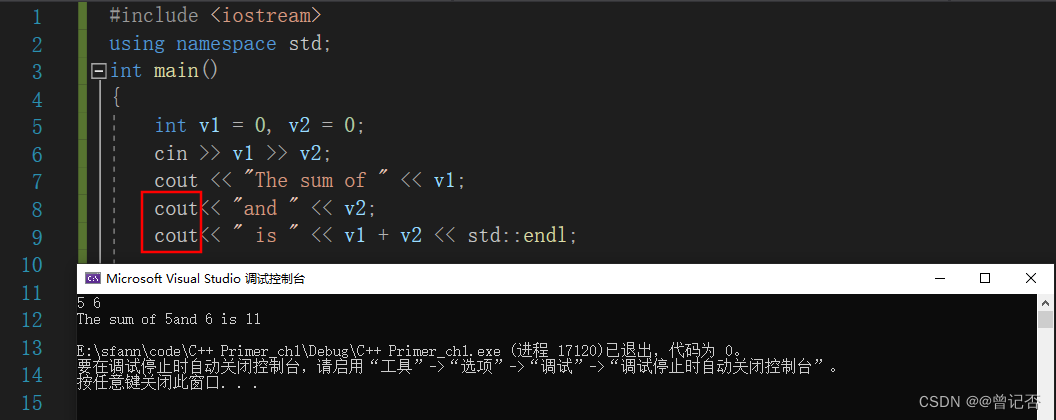
练习1.7
编译一个包含不正确的嵌套注释的程序,观察编译器返回的错误信息。
#include <iostream>
不能嵌套
* “不能嵌套”几个字会被认为是源码,像剩余程序一样处理
*/
int main (void)
{
return 0;
}

练习1.8
指出下列哪些输出语句是合法的(如果有的话)。
std::cout << "/*";
std::cout << "*/";
std::cout << " */;
std::cout << " /* ";
预测编译这些语句会产生什么样的结果,实际编译这些语句来验证你的答案(编写一个小程序,每次将上述一条语句作为其主体),改正每个编译错误。
第一条和第二条语句是合法的,第三条语句的第一个双引号和第四个双引号左引号被注释掉了,第二个双引号和第三个双引号之间的“*/”被认为是字符串的文字内容。 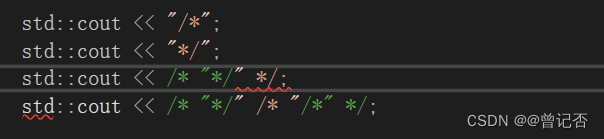
练习1.9
编写程序,使用while循环将50到100的整数相加。
#include <iostream>
using namespace std;
int main()
{
int a = 50;
int cut = 0;
while (a <= 100)
{
cut += a;
++a;
}
cout << "sum is " << cut << endl;
return 0;
}
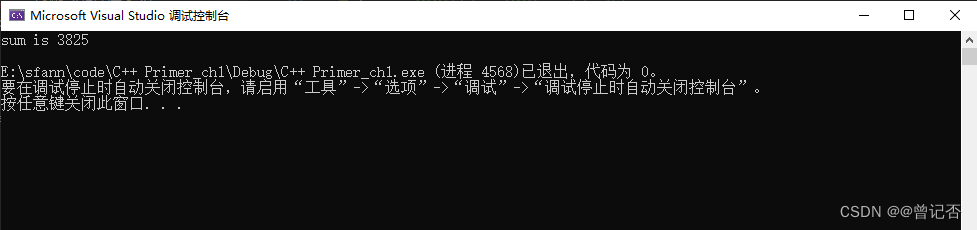
练习1.10
除了++运算符将运算对象的值增加1以外,还有一个递减运算符(–)实现将值减少1。编写程序,使用递减运算符在循环中按递减序打印出10到0之间的整数。
#include <iostream>
using namespace std;
void main()
{
int i=10;
while (i >= 0)
{
cout << i << endl;
--i;
}
}
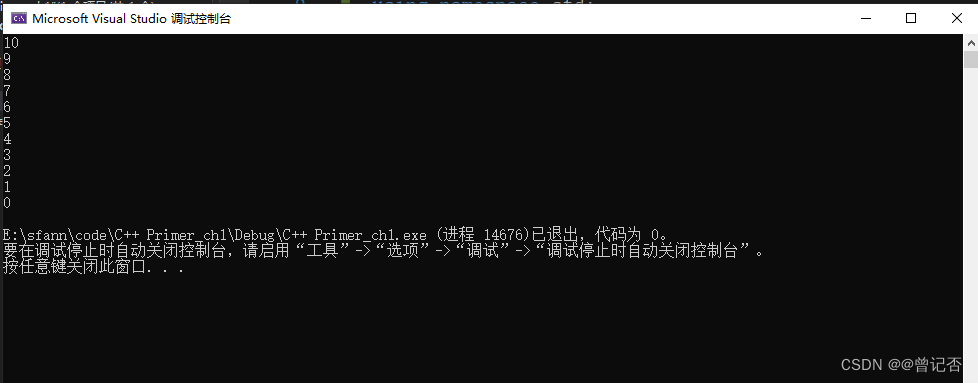
练习1.11
编写程序,提示用户输入两个整数,打印出这两个整数所指定的范围内的所有整数。
#include <iostream>
using namespace std;
int main()
{
int a = 0;
int b = 0;
cout << "输入两个整数:";
cin >> a >> b;
if (a > b)
{
int t = 0;
t = a;
a = b;
b = t;
}
while (a <= b)
{
cout << a << " ";
++a;
}
return 0;
}
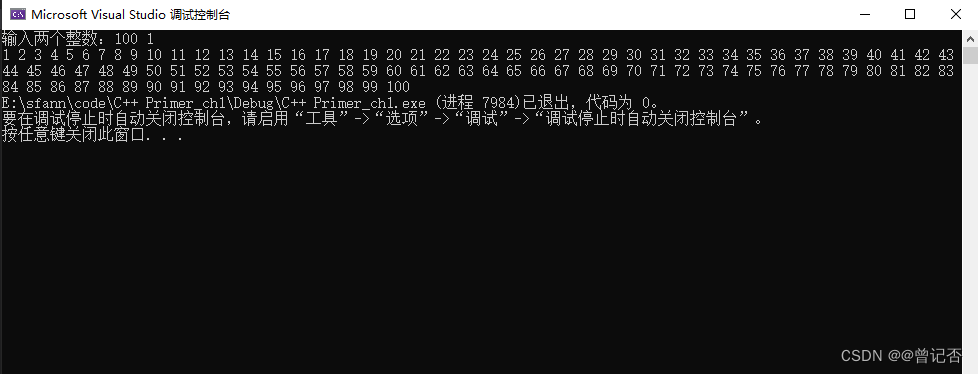
练习1.12
下面的for循环完成了什么功能?sum的终值是多少?
int sum = 0;
for (int i = -100; i <= 100; ++i)
sum += i;
-100到100之间(包含-100和100)的整数相加,sum的终值为0。
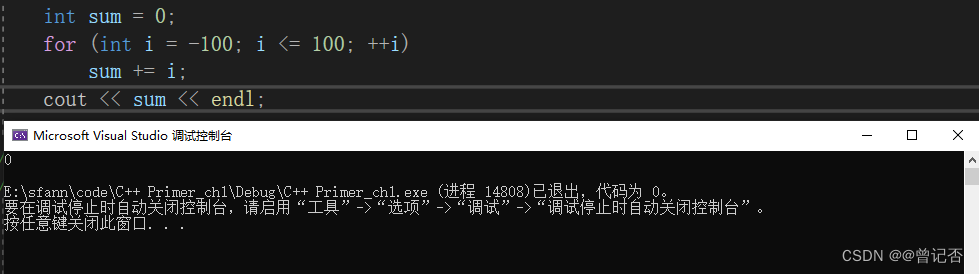
练习1.14
对比for循环和while循环,两种形式的优缺点各是什么?
循环次数已知的情况下,for循环更为简洁;无法预知循环次数是,while循环更合适。
练习1.16
编写程序,从cin读取一组数,输出其和。
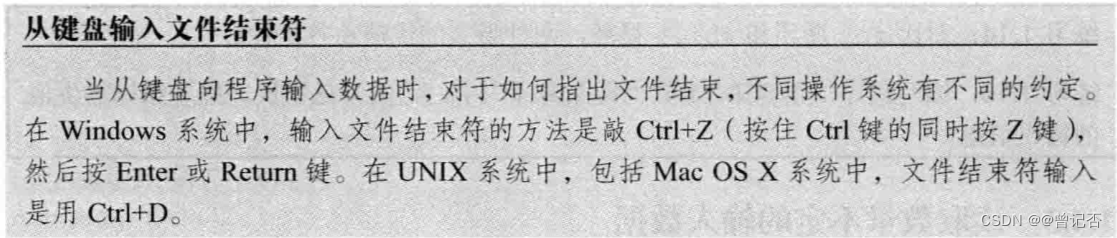 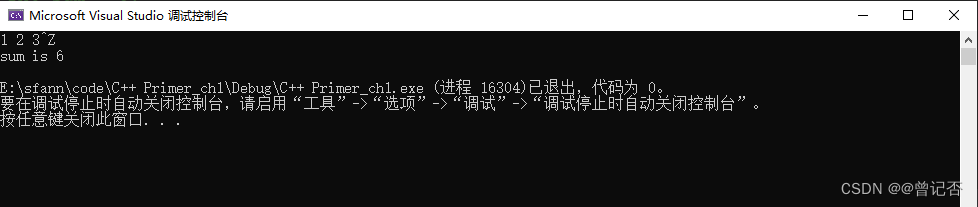
练习1.18
编译并运行本节的程序,给它输入全都相等的值。再次运行程序,输入没有重复的值。
#include <iostream>
using namespace std;
int main()
{
int a = 0;
int b = 0;
if (cin >> a)
{
int cut = 1;
while (cin >> b)
{
if (a == b)
{
++cut;
}
else
{
cout << a << "连续重复个数为:" << cut << "个" << endl;
cut = 0;
a = b;
}
}
cout << a << "连续重复个数为:" << cut << "个" << endl;
}
return 0;
}
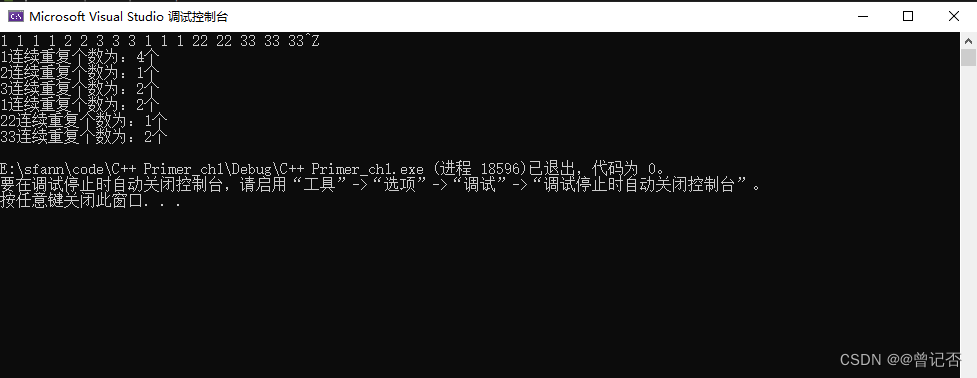 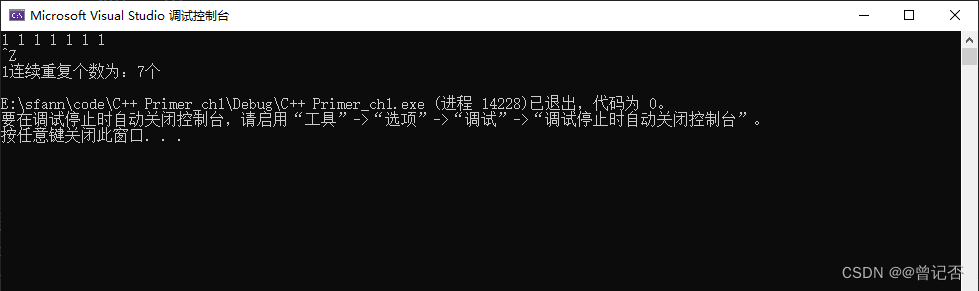 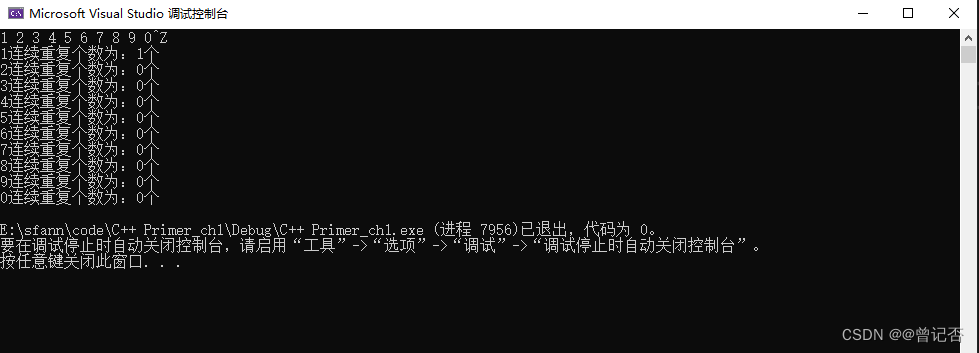
练习1.20
在网站http://www.informit.com/title/0321714113上,第1章的代码目录汇总包含了头文件Sales_item.h。将它拷贝到你自己的工作目录中。用它编写一个程序,读取一组书籍销售记录,将每条记录打印到标准输出上。
Sales_item.h
#ifndef SALESITEM_H
#define SALESITEM_H
#include <iostream>
#include <string>
class Sales_item {
friend std::istream& operator>>(std::istream&, Sales_item&);
friend std::ostream& operator<<(std::ostream&, const Sales_item&);
friend bool operator<(const Sales_item&, const Sales_item&);
friend bool
operator==(const Sales_item&, const Sales_item&);
public:
Sales_item() = default;
Sales_item(const std::string &book): bookNo(book) { }
Sales_item(std::istream &is) { is >> *this; }
public:
Sales_item& operator+=(const Sales_item&);
std::string isbn() const { return bookNo; }
double avg_price() const;
private:
std::string bookNo;
unsigned units_sold = 0;
double revenue = 0.0;
};
inline
bool compareIsbn(const Sales_item &lhs, const Sales_item &rhs)
{ return lhs.isbn() == rhs.isbn(); }
Sales_item operator+(const Sales_item&, const Sales_item&);
inline bool
operator==(const Sales_item &lhs, const Sales_item &rhs)
{
return lhs.units_sold == rhs.units_sold &&
lhs.revenue == rhs.revenue &&
lhs.isbn() == rhs.isbn();
}
inline bool
operator!=(const Sales_item &lhs, const Sales_item &rhs)
{
return !(lhs == rhs);
}
Sales_item& Sales_item::operator+=(const Sales_item& rhs)
{
units_sold += rhs.units_sold;
revenue += rhs.revenue;
return *this;
}
Sales_item
operator+(const Sales_item& lhs, const Sales_item& rhs)
{
Sales_item ret(lhs);
ret += rhs;
return ret;
}
std::istream&
operator>>(std::istream& in, Sales_item& s)
{
double price;
in >> s.bookNo >> s.units_sold >> price;
if (in)
s.revenue = s.units_sold * price;
else
s = Sales_item();
return in;
}
std::ostream&
operator<<(std::ostream& out, const Sales_item& s)
{
out << s.isbn() << " " << s.units_sold << " "
<< s.revenue << " " << s.avg_price();
return out;
}
double Sales_item::avg_price() const
{
if (units_sold)
return revenue/units_sold;
else
return 0;
}
#endif
#include <iostream>
#include "Sales_item.h"
using namespace std;
int main (void)
{
Sales_item book;
cout << "请输入销售记录:" << endl;
while (cin >> book)
cout << "ISBN、销售额和平均售价为 " << book << endl;
return 0;
}
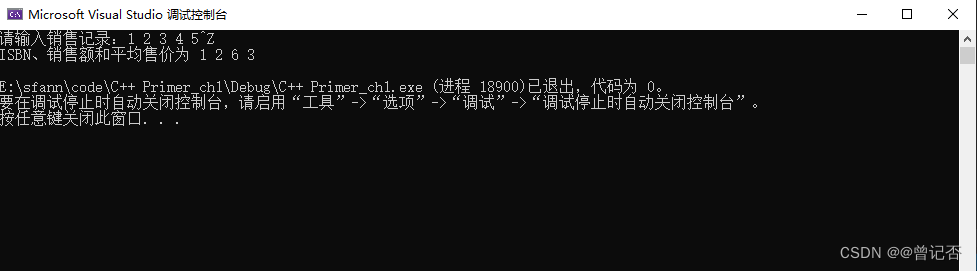
|