运算符重载一般格式:
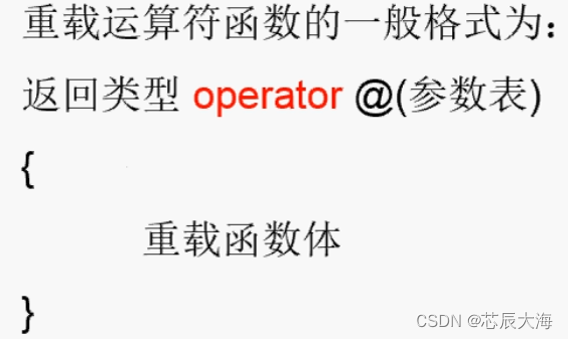
@表示要重载的运算符;若是重载"="则@用“=”替换;若是重载"+"则@用“+”替换;
参数表根据运算符的操作数个数决定;单目or双目;
c++中可重载运算符如下:
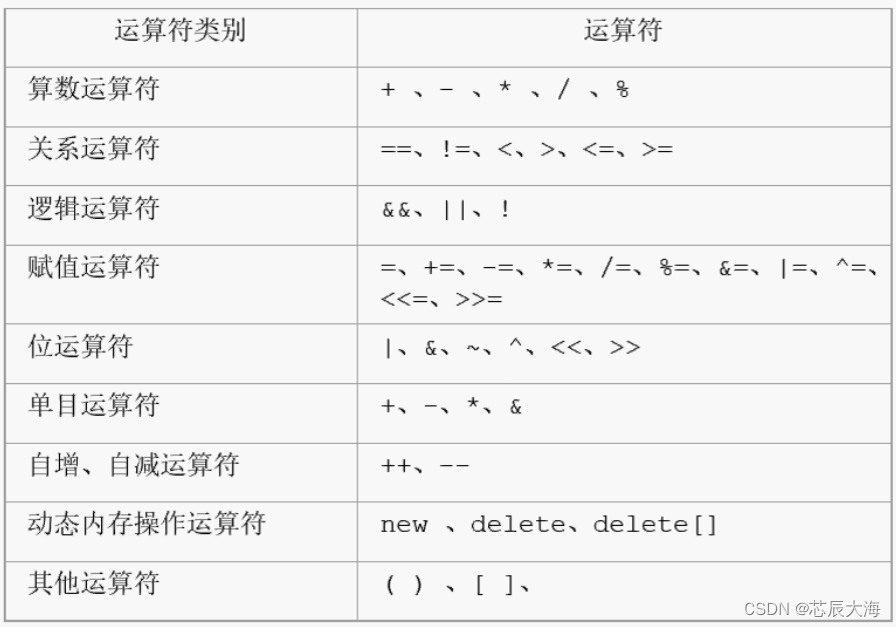
c++中有下列5个运算符不可重载:
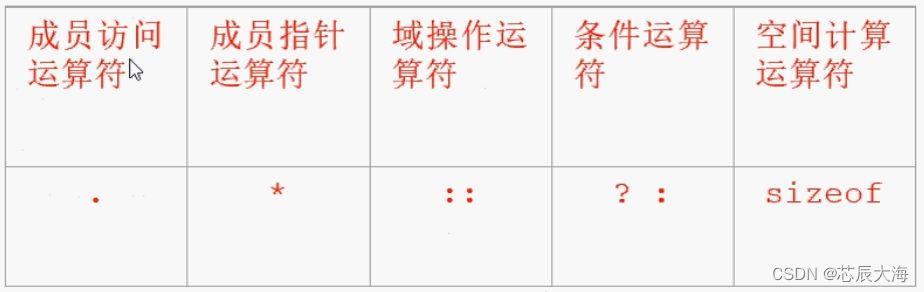
重载运算符使用规则:
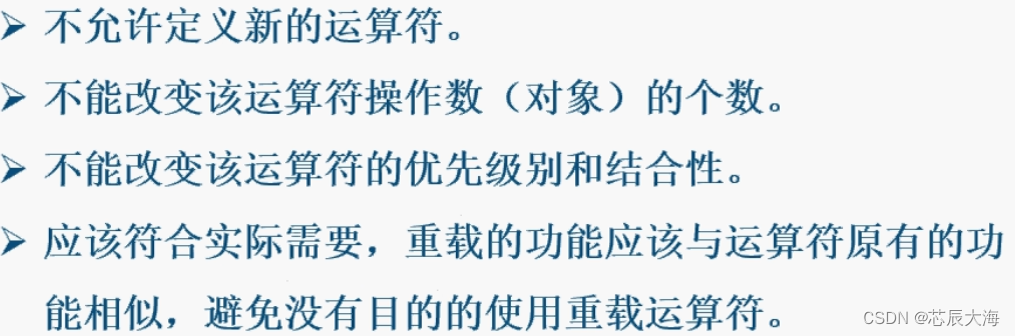
运算符重载两种形式:
成员运算符函数;友元运算符函数;
对于同一运算符,要么定义为成员,要么定义为友元;不可二者都定义;
运算符重载为友元函数:
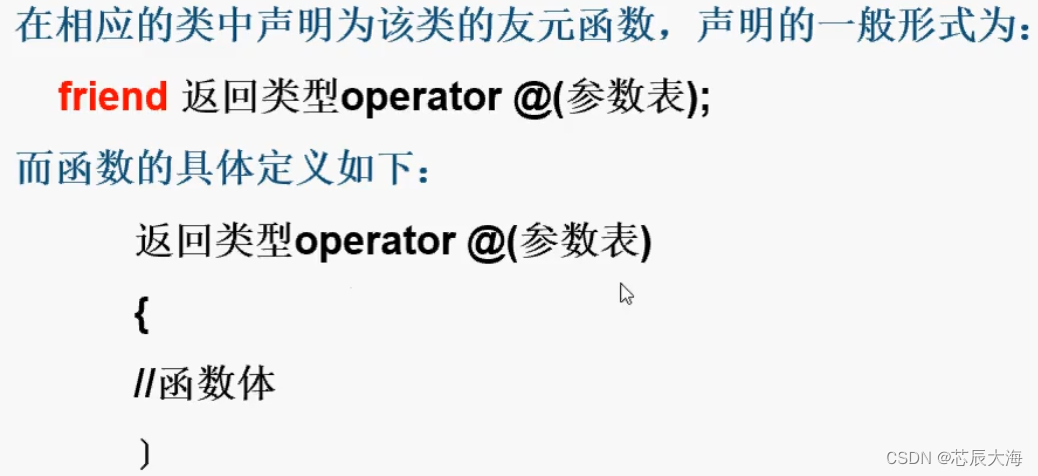
友元才能访问类的私有属性;
案例1:友元方式重载运算符“+”、“==”
#include<iostream>
using namespace std;
class Add {
double real, image;
public:
Add(double r = 0, double i = 0)
{
real = r;
image = i;
}
void show()
{
if (image > 0)
{
if (image == 1)
cout << real << "+" << "i" << endl; //虚部系数为1,如:3+i
else
{
cout << real << "+" << image << "i" << endl; //虚部系数为其它正数,如:3+5i
}
}
else if (image < 0) {
if (image == -1)
cout << real << "-" << "i" << endl; //虚部系数为-1情况;如:2-i
else
{
cout << real << "-" << image << "i" << endl; //虚部系数为其它负数,如:3-5i
}
}
else
{
cout << real << endl;
}
}
friend Add operator + (const Add& c1, const Add& c2); //类中声明是友元,否则重载运算符的函数不可访问类私有属性
friend bool operator ==(const Add& c1, const Add& c2);
};
//类外重载运算符"+"
Add operator + (const Add& c1, const Add& c2) //引用方式传参,保证传参不变加const,返回值数据类型Add
{
Add temp;
temp.real = c1.real + c2.real;
temp.image = c1.image + c2.image;
return temp;
}
//类外重载运算符"=="
bool operator ==(const Add& c1, const Add& c2) {
if (c1.real == c2.real && c1.image == c2.image)
return true;
else
return false;
}
int main()
{
Add a1(10, 5), a2(2, -5);
a1.show();
a2.show();
Add a3 = a1 + a2; //隐式调用,两个自定义数据类型相加
// Add a3=operator+(a1,a2); //显式调用
a3.show();
if(a1==a2)
cout << "两个复数相等" << endl;
else
{
cout << "两个复数不相等" << endl;
}
return 0;
}
输出结果:
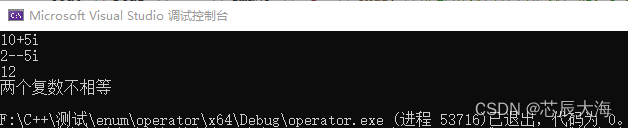
?运算符重载为成员函数:
|