一回顾
上一节说明了在栈区定义的变量 在回收时就会出现错误(不共享资源)
只有在堆区才会正常。(共享资源)
二 线程同步
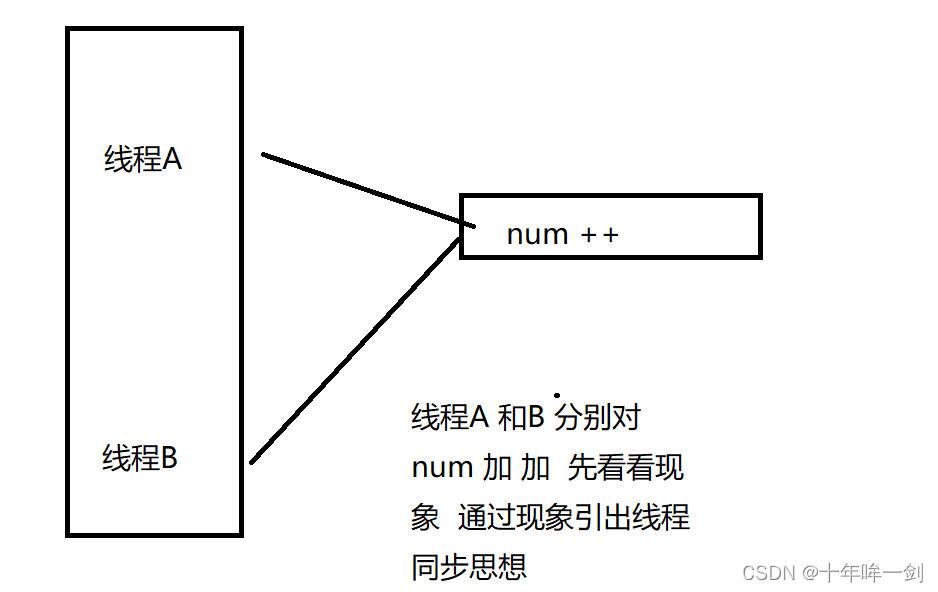
还是一样的流程 1 创建 线程 2 父线程回收子线程 3 写对应的子线程函数
#include<pthread.h>
#include<stdio.h>
#include <string.h>
#include<stdlib.h>
int res;
void *mychlidA(void *arg)
{
for(int i=0;i<10000;i++)
{
int usart=res;
unsart++;
res=usart;
printf("********I=====%d\n",res);
usleep(10);
}
}
void *mychlidB(void *arg)
{
for(int i=0;i<10000;i++)
{
int usart=res;
unsart++;
res=usart;
printf("&&&&I=====%d\n",res);
usleep(10);
}
}
int main()
{
pthread_t pthidA,pthidB;
pthread_create(&pthidA,NULL,mychlidA,NULL);
pthread_create(&pthidB,NULL,mychlidB,NULL);
pthread_join(pthidA,NULL);
pthread_join(pthidB,NULL);
return 0;
}
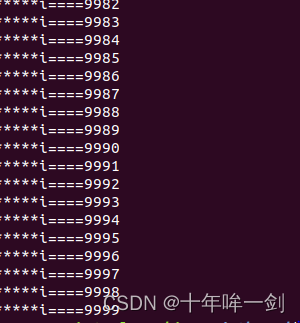
没有打印完
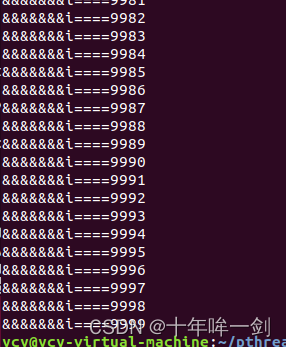 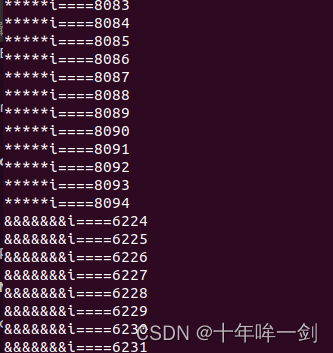
还会发生抢占
原因在哪里呢
1 比如 线程A 原本0-100 是要输出的 但是到了100 就被线程B 抢占cpu占用权
导致了100 却没有显示
2 数据必须是写入内存才算是 真正存在的
3 因为是对堆区的变量进行操作
下面引出一个线程同步思想 来解决 这个问题
三 线程同步思想
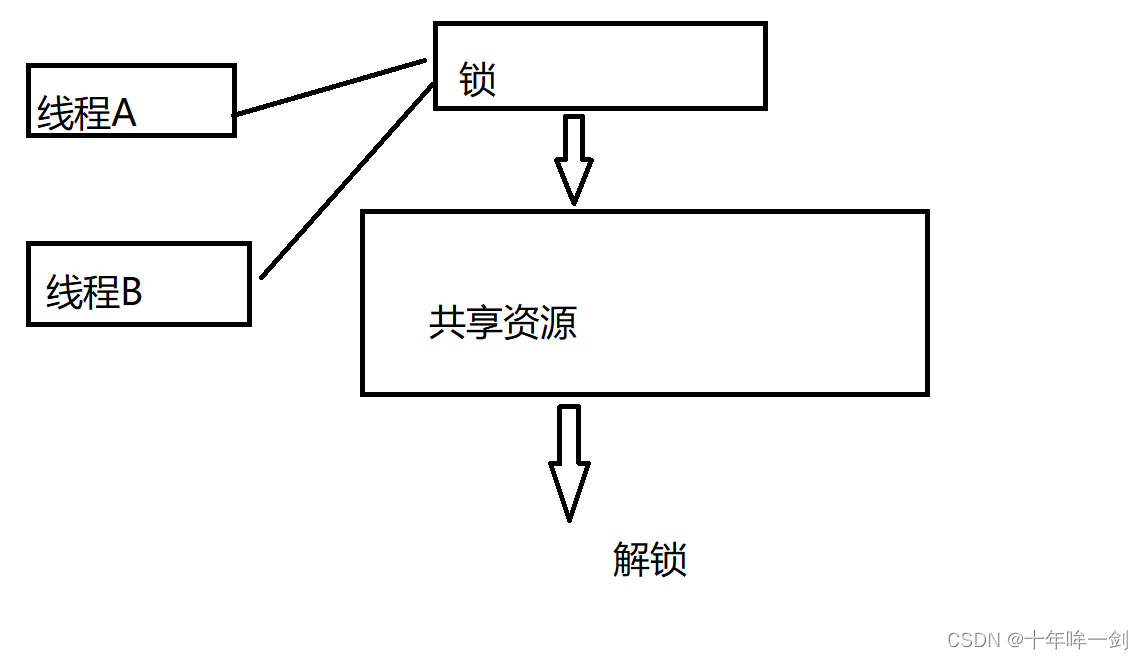
引出一个 锁 的 思想
上面加锁之后 就出现下面三种情况
1 线程A------锁开了-----共享资源-----开锁
2 锁关闭---线程阻塞 阻塞在锁上
3 线程本来是可以并行的 但是共享资源只能一个人使用,通过加锁的方式 并行的情况变成了
串行 (轮流执行)
四 互斥量 (互斥锁)实现同步思想
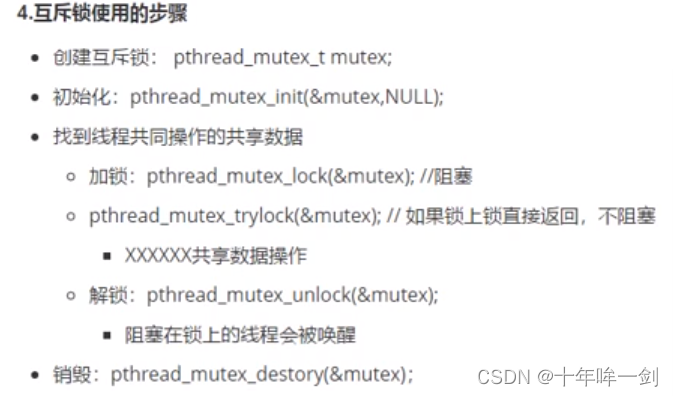
1 创建互斥量 2 初始化 3 加锁 4 找到资源 5解锁 6销毁(没有用到就销毁)
1 创建互斥量只有一个定义 很好理解
2 初始化函数
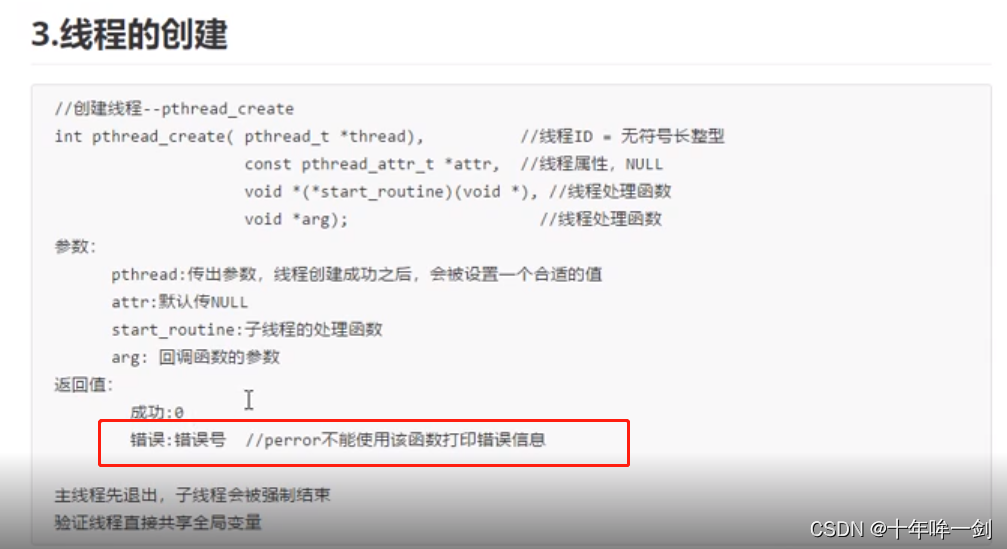 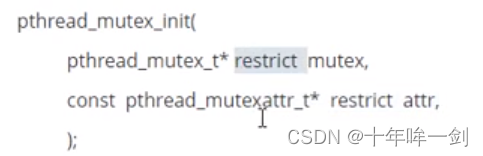
第一个参数:一个pthread_mutex * restrict mutex (互斥量赋值)
中restrict 是一个关键字 什么意思呢
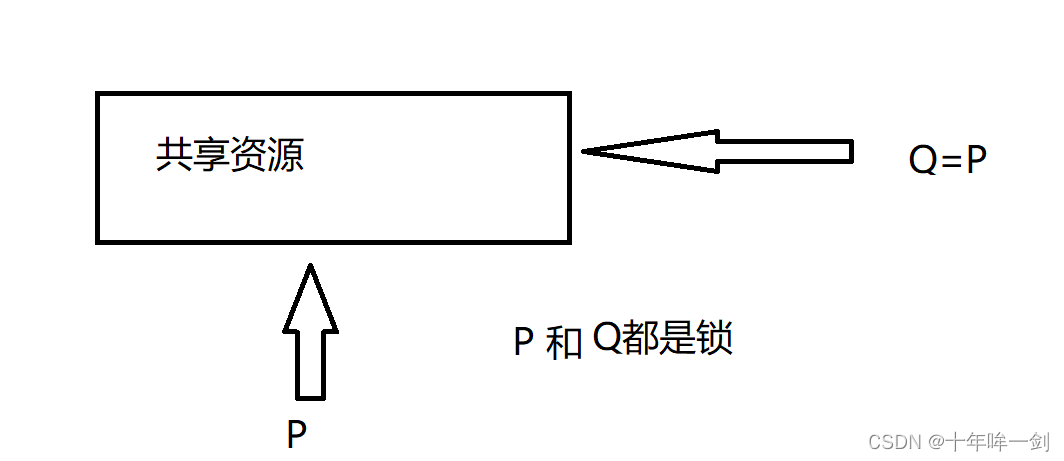
有了restrict的关键字 p赋值和Q
Q是不可以用的 restrict作用就体现出来了。
第二个参数:是和线程创建的第二个参数相关量 锁无需属性 为NULL
3 加锁
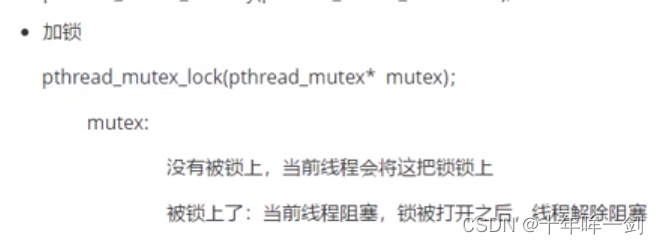
函数只有一个参数
参数解释
没有锁上 就锁 锁上了 就为阻塞 开锁了 就是不阻塞
4 找到资源 :找到要执行的程序
5 解锁 :阻塞被唤醒 就是不阻塞

6 销毁 :不用就销毁

#include<pthread.h>
#include<stdio.h>
#include <string.h>
#include<stdlib.h>
int res;
pthread_mutex_t mutex;
void *mychlidA(void *arg)
{
for(int i=0;i<10000;i++)
{
pthread_mutex_lock(&mutex);
int usart=res;
unsart++;
res=usart;
printf("********I=====%d\n",res);
pthread_mutex_unlock(&mutex);
usleep(11);
}
}
void *mychlidB(void *arg)
{
for(int i=0;i<10000;i++)
{
pthread_mutex_lock(&mutex);
int usart=res;
unsart++;
res=usart;
printf("&&&&I=====%d\n",res);
pthread_mutex_unlock(&mutex);
usleep(11);
}
}
int main()
{
pthread_t pthidA,pthidB;
pthread_mutex_init(&mutex,NULL);
pthread_create(&pthidA,NULL,mychlidA,NULL);
pthread_create(&pthidB,NULL,mychlidB,NULL);
pthread_join(pthidA,NULL);
pthread_join(pthidB,NULL);
pthread_mutex_destroy(&mutex);
return 0;
}
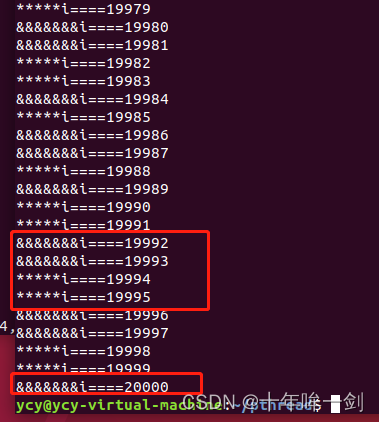
都是按顺序 共享资源 并且会打印到2 00 00
|