#include <iostream>
#include <string>
using namespace std;
class Animal
{
public:
virtual void speaking()
{
cout<<"动物在叫"<<endl;
}
Animal(){
cout<<"Animal 构造函数"<<endl;
}
virtual ~Animal(){//不加上关键字就无法是放掉cat子类在堆内存分配的空间
cout<<"Animal 析构函数"<<endl;
}
};
class Cat:public Animal
{
public:
Cat(string name)
{
cout<<"cat 构造函数"<<endl;
m_name = new string(name);
}
~Cat()
{
if(m_name != nullptr){
cout<<"cat 析构函数"<<endl;
delete m_name;
m_name = nullptr;
}
}
void speaking()
{
cout<<*m_name<<"小猫在叫"<<endl;
}
string *m_name;
};
class Dog:public Animal
{
public:
void speaking()
{
cout<<"小狗在叫"<<endl;
}
};
void doSpeaking(Animal *animal)
{
animal->speaking();
delete animal;
}
void test()
{
// Animal *animal = new Cat("tom");
// animal->speaking()
// delete animal;
doSpeaking(new Cat("tom"));
}
void test01()
{
// Dog dog;
// doSpeaking(dog);
}
int main()
{
test();
return 0;
}
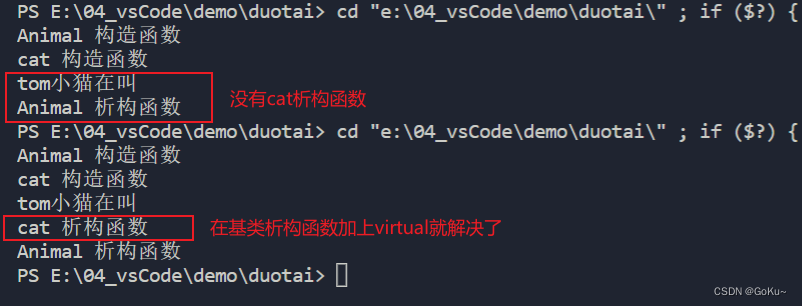
|