学习网站:C语言网. 编译器:Red Panda Dev-C++
1.位运算符表
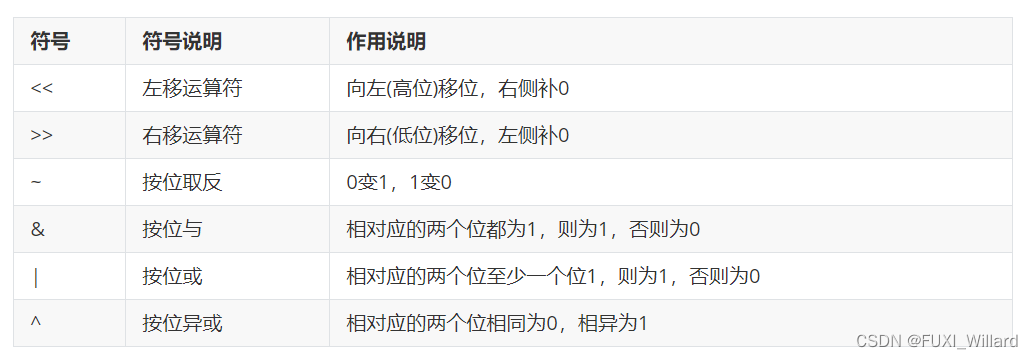
#include <stdio.h>
int main(){
int numberOne,numberTwo;
numberOne = 13 << 2;
numberTwo = 25 >> 3;
printf("numberOne = %d,numberTwo = %d\n", numberOne, numberTwo);
return 0;
}
2.按位与&运算符
#include <stdio.h>
int main(){
int numberOne;
int numberTwo;
int numberThree;
numberOne = 3 & 5;
numberTwo = 0 & 1;
numberThree = 5 & 5;
printf("The result of 3 & 5 = %d\n", numberOne);
printf("The result of 0 & 1 = %d\n", numberTwo);
printf("The result of 5 & 5 = %d\n", numberThree);
return 0;
}
3.按位或|运算符
#include <stdio.h>
int main(){
int numberOne;
int numberTwo;
int numberThree;
numberOne = 8 | 7;
numberTwo = 8 | 0;
numberThree = 8 | 15;
printf("The result of 8 | 7 = %d\n", numberOne);
printf("The result of 8 | 0 = %d\n", numberTwo);
printf("The result of 8 | 15 = %d\n", numberThree);
return 0;
}
4.按位异或^运算符
#include <stdio.h>
int main(){
int numberOne;
int numberTwo;
numberOne = 15 ^ 16;
numberTwo = 15 ^ 0;
printf("The result of 15 ^ 16 = %d\n", numberOne);
printf("The result of 15 ^ 0 = %d\n", numberTwo);
return 0;
}
#include <stdio.h>
int swap(int *a,int *b){
*a = *a ^ *b;
*b = *b ^ *a;
*a = *a ^ *b;
}
int main(){
int a = 3;
int b = 5;
swap(&a, &b);
printf("a = %d b = %d\n",a,b);
return 0;
}
5.按位取反~运算符
#include <stdio.h>
int main(){
unsigned int numberOne = 1;
int numberTwo = 1;
int numberThree = 0;
int numberFour = 10;
printf("~1 = %u\n", ~numberOne);
printf("~1 = %d\n", ~numberTwo);
printf("~0 = %d\n", ~numberThree);
printf("~10 = %d\n", ~numberFour);
return 0;
}
|