学习网站:C语言网. C语言基础:C语言基础. 笔记及源码gitee地址:C++基础笔记及源码. 编译器:Red Panda Dev-C++.
1.异常概念
#include <iostream>
using namespace std;
int main(){
int num1,num2;
cout << "Please enter num1 and num2:" << endl;
cin >> num1 >> num2;
if(num2 == 0){
cout << "Exception.Divide 0!" << endl;
}
else{
cout << "num1 = " << num1 << endl;
cout << "num2 = " << num2 << endl;
cout << "num1 / num2 = " << num1 / num2 << endl;
}
return 0;
}
2.异常处理机制try-catch
#include <iostream>
using namespace std;
int main(){
int num1,num2;
cin >> num1 >> num2;
try{
if(num2 == 0){
throw "error!num2 == 0";
}
}
catch(const char *str){
cout << str << endl;
}
catch(int){
cout << "Throw int" << endl;
}
return 0;
}
3.标准异常exception处理类
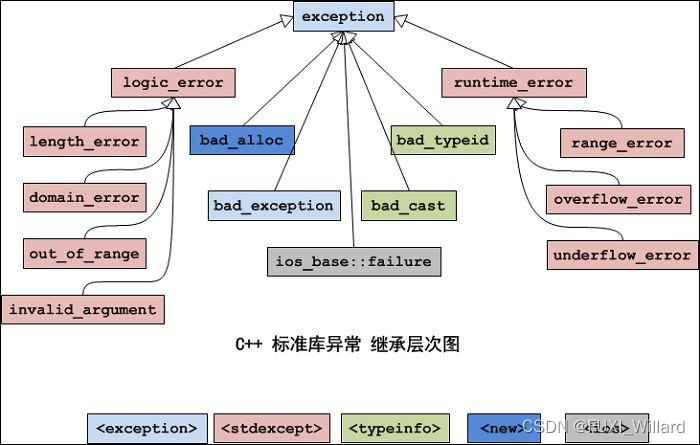
#include <iostream>
#include <new>
#include <stdexcept>
using namespace std;
int main(){
string *s;
try{
s = new string("www.fuxi.com");
cout << s->substr(15,5);
}
catch(bad_alloc &t){
cout << "Exception occurred!" << t.what() << endl;
}
catch(out_of_range &t){
cout << "Exception occurred!" << t.what() << endl;
}
return 0;
}
|