1.软件安装目录保存在注册表中
离线程序自动更新,其本质是替换原来的.dll,.exe等文件,并保持文件名不变,或者新增部分文件。 以下是以同名文件替换为例: 1.首先需要知道软件的安装路径,这个可以在软件运行时,自动保存软件安装路径到注册表指定位置; 2.更新软件读取注册表对应的安装路径; 3.文件的删除与替换。
保存安装路径到注册表
QString path = QCoreApplication::applicationFilePath();
path.replace("/", "\\");
QString readPath;
QSettings* settings = new QSettings(Reg_MyExe_Path, QSettings::NativeFormat);
QVariant p = settings->value(exeName);
if (p.toString().isEmpty())
{
settings->setValue(exeName, path);
}
delete settings;
读取注册表软件安装目录
QString exePth;
QSettings* settings = new QSettings(Reg_MyExe_Path, QSettings::NativeFormat);
exePth = settings->value(exeName).toString();
if (exePth.isEmpty())
{
QMessageBox::warning(this, "Warning", "软件更新失败!");
return;
}
delete settings;
qDebug() << "-----file path: " << exePth;
QString oldFile, newFile,fname = "123.txt";
oldFile = QFileInfo(exePth).absolutePath().append("%1%2").arg("/").arg(fname);
newFile = QFileInfo("testFile/" + fname).absoluteFilePath();
autoReplaceFile(oldFile, newFile);
测试demo界面
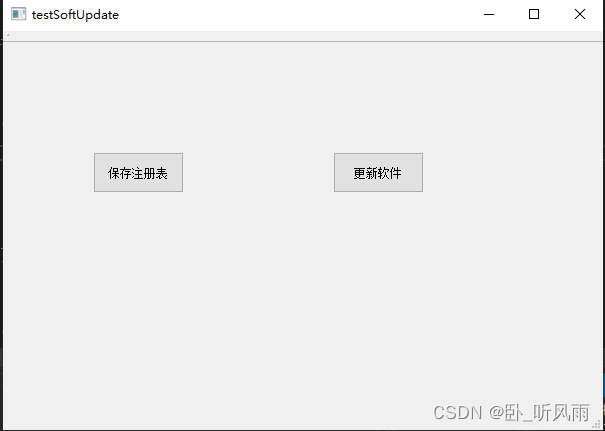
测试demo.h
#pragma once
#include <QtWidgets/QMainWindow>
#include "ui_testSoftUpdate.h"
#include <qsettings.h>
#include <QFile>
#include <qfileinfo.h>
#include <qmessagebox.h>
#define Reg_MyExe_Path "HKEY_LOCAL_MACHINE\\SOFTWARE\\MyCompany"
#if _MSC_VER >= 1600
#pragma execution_character_set("utf-8")
#endif
class testSoftUpdate : public QMainWindow
{
Q_OBJECT
public:
testSoftUpdate(QWidget *parent = Q_NULLPTR);
private slots:
void on_btnSave_clicked();
void on_btnUpdate_clicked();
private:
Ui::testSoftUpdateClass ui;
QString exeName = "MyExe";
void autoReplaceFile(const QString& oldfile, const QString& newfile);
};
测试demo.cpp
#include "testSoftUpdate.h"
#include <qdebug.h>
testSoftUpdate::testSoftUpdate(QWidget *parent)
: QMainWindow(parent)
{
ui.setupUi(this);
}
void testSoftUpdate::on_btnSave_clicked()
{
QString path = QCoreApplication::applicationFilePath();
path.replace("/", "\\");
QString readPath;
QSettings* settings = new QSettings(Reg_MyExe_Path, QSettings::NativeFormat);
QVariant p = settings->value(exeName);
if (p.toString().isEmpty())
{
settings->setValue(exeName, path);
}
delete settings;
}
void testSoftUpdate::on_btnUpdate_clicked()
{
QString exePth;
QSettings* settings = new QSettings(Reg_MyExe_Path, QSettings::NativeFormat);
exePth = settings->value(exeName).toString();
if (exePth.isEmpty())
{
QMessageBox::warning(this, "Warning", "软件更新失败!");
return;
}
delete settings;
qDebug() << "-----file path: " << exePth;
QString oldFile, newFile,fname = "123.txt";
oldFile = QFileInfo(exePth).absolutePath().append("%1%2").arg("/").arg(fname);
newFile = QFileInfo("testFile/" + fname).absoluteFilePath();
autoReplaceFile(oldFile, newFile);
}
void testSoftUpdate::autoReplaceFile(const QString& oldfile, const QString& newfile)
{
qDebug() << "-----oldfile path: " << oldfile << ", newfile path : " << newfile;
QFile f;
if (f.remove(oldfile))
{
QMessageBox::warning(this, "Warning", "请先关闭软件!");
return;
}
_sleep(20);
if (f.copy(newfile, oldfile))
{
qDebug() << "-----copy succeed ! --------";
QMessageBox::information(this, "通知", "软件已更新完成!");
}
else
{
QMessageBox::warning(this, "Warning", "请先关闭软件!");
}
}
|