前言:更多内容请看总纲《嵌入式C/C++学习路》
1.C++的结构
声明或定义结构型变量,可以省略struct关键字
#include <iostream>
using namespace std;
struct Student
{
char name[256];
int age;
};
int main(void)
{
Student s1 = {"张飞", 25};
cout << s1.name << ' ' << s1.age << endl;
Student *ps = &s1;
cout << ps->name << ' ' << ps->age << endl;
return 0;
}

可以定义成员函数,在结构体的成员函数中可以直接访问该结构体的成员变量,无需通过点” . “ 或者” -> “ 调用成员变量
#include <iostream>
using namespace std;
struct Student
{
char name[256];
int age;
void who(void){
cout << "我是" << name << ",今年" << age << "岁了。" << endl;
}
};
int main(void)
{
Student s1 = {"张飞", 25},s2 = {"赵云",22};
cout << s1.name << ' ' << s1.age << endl;
Student *ps = &s1;
cout << ps->name << ' ' << ps->age << endl;
s1.who();
ps->who();
s2.who();
ps = &s2;
ps->who();
return 0;
}
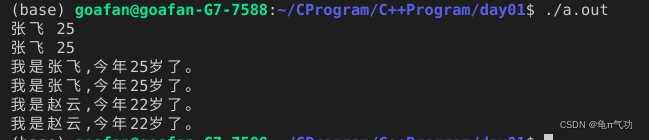
2. C++的联合
声明或定义联合型变量,可以省略union关键字
支持匿名联合
#include <iostream>
#include <cstdio>
using namespace std;
int main(void)
{
union
{
int i;
char c[4];
};
i = 0x12345678;
printf("%x %x %x %x\n", c[0], c[1], c[2], c[3]);
return 0;
}
 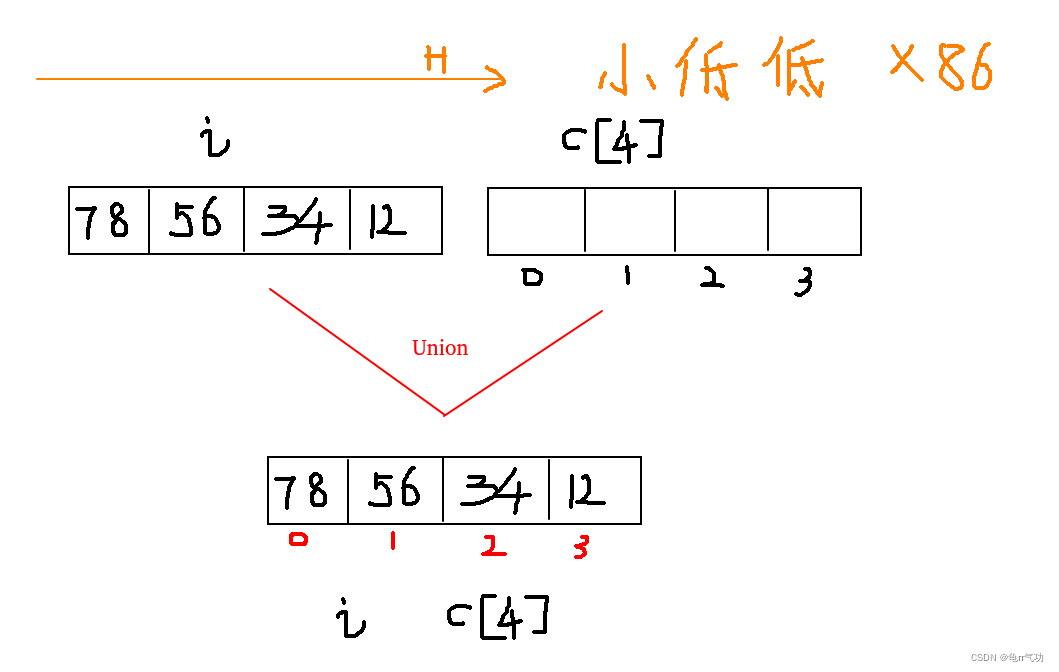
3. C++的枚举
声明或定义枚举类型变量,可以省略enum关键字 独立的类型,和整型之间不能隐式相互转换
#include <iostream>
using namespace std;
int main(void)
{
enum
{
RED,
GREEN,
BLUE
} color;
color = RED;
int i = RED, j = BLUE;
cout << i << endl << j << endl;
return 0;
}

4. 布尔类型
表示布尔量的数据-bool 布尔类型的字面值常量 -true 表示真 -false表示假 布尔类型的本质-单字节整数,用1和0表示真和假 任何基本类型都可以被隐式转换为布尔类型 -非0为真 -0为假
#include <iostream>
using namespace std;
int main()
{
cout << sizeof(bool) << endl;
bool b = true;
cout << b << endl;
b = false;
cout << b << endl;
return 0;
}

|