C++ 批量修改文件名
前言
在网上下一些学习资料,可是每个文件后带有一些其他无关的文字,形式如,某某某【某某某】.mp4,其中【】及其内容皆为无关内容,本文代码程序用于批量删除每个MP4文件后的【某某某】。
注意
文件名即为中文字符,不同于英文格式,所以以下代码中在需要的时候都使用了宽字符处理。
代码
#include <iostream>
#include <string>
#include <vector>
#include <io.h>
int main()
{
locale china("chs");
wcin.imbue(china);
wcout.imbue(china);
wstring dirpath = L"E:\\test\\";
_wfinddata_t file;
long lf;
wchar_t suffixs[] = L"*.mp4";
vector<wstring> fileNameList;
wchar_t* p;
int psize = dirpath.size() + 6;
p = new wchar_t[psize];
wcscpy(p, dirpath.c_str());
if ((lf = _wfindfirst(wcscat(p, suffixs), &file)) == -1l)
{
cout << "文件没有找到!\n";
} else
{
cout << "\n文件列表:\n";
do {
wstring str(file.name);
fileNameList.push_back(str);
wcout << str << endl;
} while (_wfindnext(lf, &file) == 0);
}
_findclose(lf);
delete[] p;
cout << "\n开始修改文件名:" << endl;
for (vector<wstring>::iterator iter = fileNameList.begin(); iter != fileNameList.end(); ++iter)
{
wstring oldName = dirpath + *iter;
auto pos = iter->find(L"【");
wstring newName = dirpath + iter->substr(0, pos);
newName += L".mp4";
wcout << "oldName:" << oldName << endl;
wcout << "newName:" << newName << endl;
wcout << "oldName size = " << oldName.size() << endl;
wcout << "newName size = " << newName.size() << endl;
int ret = _wrename(oldName.c_str(), newName.c_str());
if (ret != 0)
perror("rename error!");
cout << endl;
}
system("pause");
return 0;
}
读者可以根据自身需求修改代码。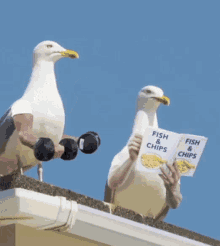
温馨提示
最好在使用程序前备用原资料,避免出现意外情况(比如我,在编写使用过程中忘记添加决定路径,导致原资料改名后跑到了项目路径下😑)。
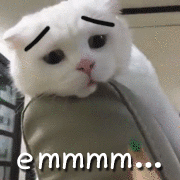
参考
https://blog.csdn.net/Dr_Myst/article/details/81463450
|