#include <iostream>
using namespace std;
#include <string>
// 1.创建学生数据类型 学生包括(姓名,年龄,分数)
struct Student
{
string name;
int age;
int score;
}s3; // 顺便创建一个结构体变量
int main()
{
// 2.通过学生类型创建具体的学生
// struct 关键字可以省略
struct Student s1;
s1.name = "张三";
s1.age = 18;
s1.score = 88;
struct Student s2 = {"李四", 23, 98};
s3.name = "王五";
s3.age = 20;
s3.score = 89;
cout << "姓名: " << s1.name << " ";
cout << "年龄: " << s1.age << " ";
cout << "分数:" << s1.score << endl;
cout << "姓名: " << s2.name << " ";
cout << "年龄: " << s2.age << " ";
cout << "分数:" << s2.score << endl;
cout << "姓名: " << s3.name << " ";
cout << "年龄: " << s3.age << " ";
cout << "分数:" << s3.score << endl;
cout << endl;
system("pause");
return 0;
}
通过结构体创建变量有三种方式:
1. struct 结构体名 变量名; 然后通过"."进行赋值;
2. struct 结构体名 变量名 = {成员1的值,成员2的值,...};
3. 在结构体定义时,顺便创建一个结构体变量? // 这种方式不推荐
注意:定义结构体时,struct不可以省略;创建结构体变量时,struct可以省略。
结构体数组
#include <iostream>
using namespace std;
#include <string>
//定义结构体
struct Student
{
string name;
int age;
int score;
};
int main()
{
//创建结构体数组,并赋值
struct Student stuArray[3] = {
{"张三", 18, 100},
{"李四", 23, 87},
{"王五", 26, 97}
};
// 遍历结构体数组
for (int i = 0; i < 3; i++)
{
cout << stuArray[i].name << " ";
cout << stuArray[i].age << " ";
cout << stuArray[i].score << endl;
}
// 修改第三位同学的信息
stuArray[2].name = "赵六";
stuArray[2].age = 55;
stuArray[2].score = 88;
// 再次遍历输出
for (int i = 0; i < 3; i++)
{
cout << stuArray[i].name << " ";
cout << stuArray[i].age << " ";
cout << stuArray[i].score << endl;
}
cout << endl;
system("pause");
return 0;
}
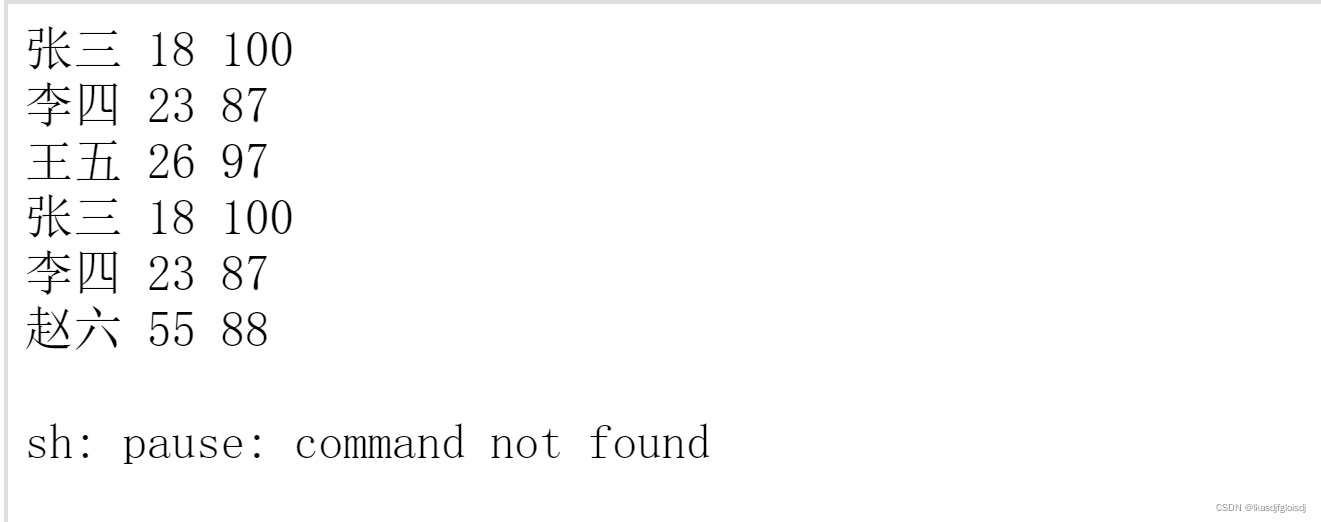
结构体指针
#include <iostream>
using namespace std;
#include <string>
struct Student
{
string name;
int age;
int score;
};
int main() {
// 创建学生结构体变量
struct Student s1 = {"张三", 18, 88};
// 通过指针指向结构体变量
struct Student * p = &s1;
// 通过指针访问结构体变量中的数据
// 通过结构体指针 访问结构体中的属性,需要利用'->'
cout << "姓名:" << p -> name << " ";
cout << "年龄: " << p -> age << " ";
cout << "成绩:" << p -> score << endl;
//system("pause");
return 0;
}
总结:结构体指针指向结构体,应定义为结构体类型;
? ? ? ? ? ?结构体指针可以通过 -> 操作符 来访问结构体中的成员;
结构体嵌套结构体
#include <iostream>
using namespace std;
#include <string>
// 定义学生结构体
struct Student
{
string name; //学生姓名
int age; // 学生年龄
int score; // 学生分数
};
//定义教师结构体
struct Teacher
{
int id; // 教师编号
string name; // 教师姓名
int age; // 教师年龄
struct Student stu; // 辅导的学生
};
int main() {
// 结构体嵌套结构体
// 创建教师结构体变量
struct Teacher t;
t.id = 1000;
t.name = "王刚";
t.age = 50;
t.stu.name = "小明";
t.stu.age = 18;
t.stu.score = 88;
cout << "教师姓名: " << t.name << " ";
cout << "教师编号: " << t.id <<" ";
cout << "教师年龄: " << t.age << endl;
cout << "教师所带学生姓名: " << t.stu.name << " ";
cout << "教师所带学生年龄: " << t.stu.age << " ";
cout << "教师所带学生成绩: " << t.stu.score << endl;
system("pause");
return 0;
}
总结:在结构体中可以嵌套另一个结构体,用来解决实际问题。
结构体做函数参数
#include <iostream>
using namespace std;
#include <string>
// 定义学生结构体
struct Student
{
string name;
int age;
int score;
};
void PrintStudent1(struct Student stu)
{
stu.age = 100;
cout << "子函数1中 姓名:" << stu.name << " ";
cout << "年龄: " << stu.age << " ";
cout << "分数:" << stu.score << endl;
}
void PrintStudent2(struct Student * p)
{
p -> age = 200;
cout << "子函数2中 姓名:" << p -> name << " ";
cout << "年龄: " << p -> age << " ";
cout << "分数:" << p -> score << endl;
}
int main() {
// 结构体做函数参数
// 创建结构体变量
struct Student stu;
stu.name = "张三";
stu.age = 24;
stu.score = 89;
// 值传递
PrintStudent1(stu);
// 地址传递
PrintStudent2(&stu);
cout << "main函数中 姓名:" << stu.name << " ";
cout << "年龄: " << stu.age << " ";
cout << "分数:" << stu.score << endl;
system("pause");
return 0;
}
值传递:形参不能修改实参;
地址传递:形参可以修改实参;
如果不想修改主函数中的数据,则用值传递;反之用地址传递。
结构体中const使用场景
#include <iostream>
using namespace std;
#include <string>
// 定义学生结构体
struct Student
{
string name;
int age;
int score;
};
// 将函数中的形参改为指针,可以减少内存空间,而且不会复制新的副本出来
void PrintStudent(const struct Student *stu)
{
// stu -> age = 150; // 加入const后,一旦有修改的操作就会报错,可以防止误操作
cout << "姓名:" << stu -> name << " ";
cout << "年龄: " << stu -> age << " ";
cout << "分数:" << stu -> score << endl;
}
int main() {
// 结构体中const使用场景
struct Student s = {"张三", 18, 78};
// 地址传递
PrintStudent(&s);
cout << "main 函数中张三的年龄:" << s.age << endl;
system("pause");
return 0;
}
|