0.前提(文件内容)
(1)test.h
#pragma once
class Test{
public:
Test();
~Test();
void Func(int i);
};
(2)test.cpp
#include <iostream>
#include "test.h"
using namespace std;
Test::Test(){
cout << __func__ << "()" << endl;
}
Test::~Test(){
cout << __func__ << "()" << endl;
}
void Test::Func(int i){
cout << __func__ << "(" << i << ")" << endl;
}
(3)main.cpp
#include "test.h"
int main(){
Test t;
t.Func(100);
}
1、静态库的制作与使用
1.1 创建
(1)编译源文件
g++ -c -o test.o test.cpp
(2)生成静态库
ar -rcs libtest.a test.o
1.2 使用
(1)链接静态库
g++ -o main main.cpp -L. -ltest
或者
g++ -o main main.cpp ./libtest.a
注意: 库一定要放在命令行的末尾
(2)测试
./main
(3)结果
Test()
Func(100)
~Test()
尝试把test.cpp修改如下,重现编译libtest.a,再次执行./main查看结果
void Test::Func(int i){
cout << __func__ << "(" << i*100 << ")" << endl;
}
2、动态库的制作
2.1 创建
(1)编译目标文件
g++ -c -fPIC test.cpp -o test.o
(2)生成静态库
g++ -shared test.o -o libtest.so
可以合并为
g++ -shared -fPIC -o libtest.so test.cpp
2.2 使用
(1)生成可执行文件
g++ -o main main.cpp -L. -ltest
或者
g++ -o main main.cpp ./libtest.so
(2)测试
指定动态链接库位置
export LD_LIBRARY_PATH=动态链接库位置
执行
./main
(3)结果
Test()
Func(100)
~Test()
尝试把test.cpp修改如下,重现编译libtest.a,再次执行./main查看结果
void Test::Func(int i){
cout << __func__ << "(" << i*100 << ")" << endl;
}
3、动态链接库的制作
类的动态链接库是利用多态性动态加载类,只能用于C++调用,不能用于C。 具体实现步骤如下: 1、定义一个抽象类,提供纯虚函数接口 2、具体实现类继承抽象类 3、提供抽象类对象的创建和销毁的接口
3.1 创建
(1)修改test.h
#pragma once
class ITest{
public:
virtual void Func(int i) = 0;
virtual ~ITest(){}
};
class Test : public ITest{
public:
Test();
~Test();
void Func(int i);
};
extern "C" {
ITest* create(void);
void destroy(ITest *p);
typedef ITest* create_t(void);
typedef void destory_t(ITest*);
}
(2)修改test.cpp
#include <iostream>
#include "test.h"
using namespace std;
Test::Test(){
cout << __func__ << "()" << endl;
}
Test::~Test(){
cout << __func__ << "()" << endl;
}
void Test::Func(int i){
cout << __func__ << "(" << i << ")" << endl;
}
ITest* create(void){
return new Test;
}
void destroy(ITest *p){
if(NULL != p){
delete p;
}
}
(3)修改main.cpp
#include <iostream>
#include <cstdlib>
#include <dlfcn.h>
#include "test.h"
using namespace std;
int main(){
void *so_handle = dlopen("./libtest.so", RTLD_LAZY);
if (!so_handle) {
cerr << "Error: load so failed." << endl;
exit(-1);
}
create_t *create = (create_t*) dlsym(so_handle, "create");
char *err = dlerror();
if (NULL != err) {
cerr << err;
exit(-1);
}
ITest *pt = create();
pt->Func(100);
destory_t *destroy = (destory_t*) dlsym(so_handle, "destroy");
err = dlerror();
if (NULL != err) {
cerr << err;
exit(-1);
}
destroy(pt);
pt = NULL;
dlclose(so_handle);
return 0;
}
(4)编译动态加载库
g++ -shared -fPIC -o libtest.so test.cpp
3.2 使用
(1)编译主程序
g++ -o main -ldl main.cpp
(2)测试
./main
(3)结果
Test()
Func(100)
~Test()
4、总结

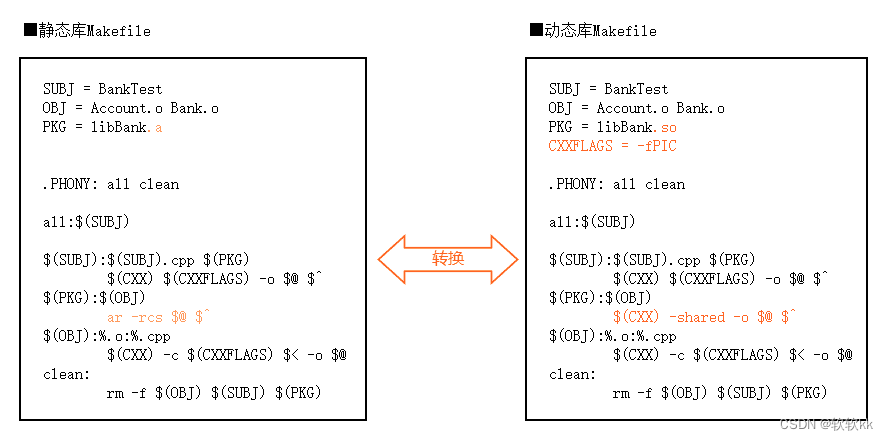
|