一 打开目录,获取该目录的结构体指针
- 需要的头文件和函数原型
DIR *opendir(const char *name);
- 功能
打开一个文件目录 - 参数:
name: 目录名
-
返回值: 成功:返回指向该目录的结构体指针 失败:NULL -
代码
DIR *p = opendir("./");
if(NULL == p)
{
perror("opendir");
return 0;
}
二 读取目录下的文件或目录(调用一次只能读取一个文件)
- 需要的头文件和函数原型
struct dirent *readdir(DIR *dirp);
- 功能
读取目录,调用一次只能读取目录中一个文件或目录 - 参数:
dirp:opendir的返回值
-
返回值: 成功:dirent结构体指针 失败:NULL -
dirent结构体定义:
struct dirent {
ino_t d_ino; /* Inode number */
off_t d_off; /* Not an offset; see below */
unsigned short d_reclen; /* Length of this record */
unsigned char d_type; /* Type of file; not supported
by all filesystem types */
char d_name[256]; /* Null-terminated filename */
};
- dirent结构体中d_type的解析
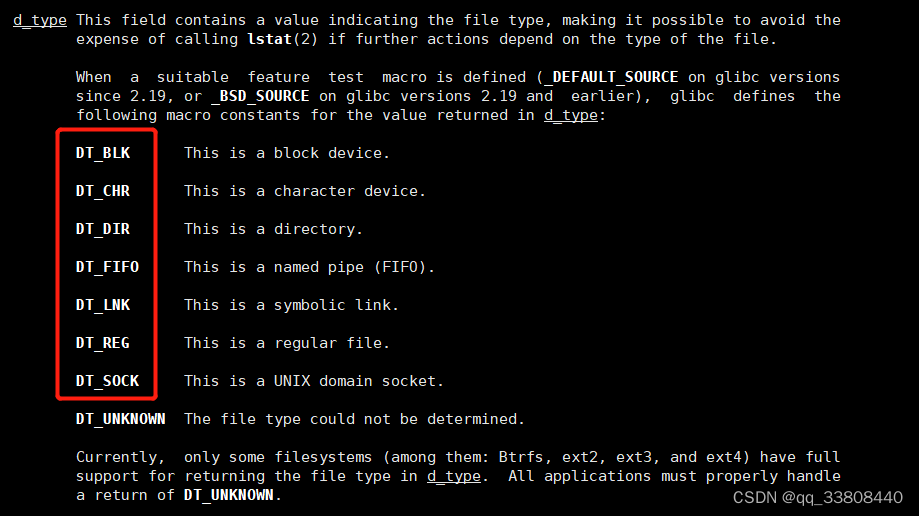 - 代码
struct dirent *dp = NULL;
while(dp = readdir(p))
{
if((dp->d_type & DT_REG) == DT_REG)
printf("%s是一个普通文件\n",dp->d_name);
else if((dp->d_type & DT_DIR) == DT_DIR)
printf("%s是一个目录\n",dp->d_name);
}
三 关闭目录
- 需要的头文件和函数原型
int closedir(DIR *dirp);
- 功能:关闭目录
- 参数:
dirp:opendir函数返回的指针
- 返回值
成功:0
失败:-1
- 代码
closedir(p);
四 递归扫描目录代码
[root@ansible9 ~]
void search_dir(const char* path)
{
DIR *dir = opendir(path);
if(NULL == dir)
{
perror("opendir");
printf("%s报错\n",path);
return;
}
struct dirent *res = NULL;
while(res = readdir(dir))
{
if((res->d_type & DT_REG) == DT_REG)
{
char filename[12800] = "";
strncpy(filename,res->d_name,strlen(res->d_name));
char filepath[12800] = "";
strncpy(filepath,path,strlen(path));
strcat(filepath,filename);
printf("%s是一个普通文件\n",filepath);
}
else if((res->d_type & DT_BLK) == DT_BLK)
{
char filename[12800] = "";
strncpy(filename,res->d_name,strlen(res->d_name));
char filepath[12800] = "";
strncpy(filepath,path,strlen(path));
strcat(filepath,filename);
printf("%s是一个块设备文件\n",filepath);
}
else if((res->d_type & DT_CHR) == DT_CHR)
{
char filename[12800] = "";
strncpy(filename,res->d_name,strlen(res->d_name));
char filepath[12800] = "";
strncpy(filepath,path,strlen(path));
strcat(filepath,filename);
printf("%s是一个字符设备文件\n",filepath);
}
else if((res->d_type & DT_FIFO) == DT_FIFO)
{
char filename[12800] = "";
strncpy(filename,res->d_name,strlen(res->d_name));
char filepath[12800] = "";
strncpy(filepath,path,strlen(path));
strcat(filepath,filename);
printf("%s是一个管道文件\n",filepath);
}
else if((res->d_type & DT_LNK) == DT_LNK)
{
char filename[12800] = "";
strncpy(filename,res->d_name,strlen(res->d_name));
char filepath[12800] = "";
strncpy(filepath,path,strlen(path));
strcat(filepath,filename);
printf("%s是一个链接文件\n",filepath);
}
else if((res->d_type & DT_SOCK) == DT_SOCK)
{
char filename[12800] = "";
strncpy(filename,res->d_name,strlen(res->d_name));
char filepath[12800] = "";
strncpy(filepath,path,strlen(path));
strcat(filepath,filename);
printf("%s是一个socket文件\n",filepath);
}
else if((res->d_type & DT_DIR) == DT_DIR)
{
char dirname[12800] = "";
strncpy(dirname,res->d_name,strlen(res->d_name));
char dirpath[12800] = "";
strncpy(dirpath,path,strlen(path));
//char dir_full_path[12800] = "";
//sprintf(dir_full_path,"%s/%s",dirpath,dirname);
strcat(dirpath,dirname);
printf("%s是一个目录=============================\n",dirpath);
if((strcmp(dirname,".") == 0) || (strcmp(dirname,"..") == 0))
{
printf("%s跳过=============================\n",dirpath);
}
else
{
strncat(dirpath,"/",1);
search_dir(dirpath);
}
}
else
{
char filename[12800] = "";
strncpy(filename,res->d_name,strlen(res->d_name));
char filepath[12800] = "";
strncpy(filepath,path,strlen(path));
strcat(filepath,filename);
printf("%s是一个未知类型文件\n",filepath);
}
}
closedir(dir);
return;
}
int main(int arg,char *args[])
{
search_dir("./");
}
|