前言
🎬本文章是 【C++笔记】 专栏的文章,主要是C++黑马的笔记、自己的实验与课设 🔗C++笔记 传送门 💻提示:源码已在github整理
一、要求
【项目】定义Student类和Score类,输出一个学生的成绩单(包括学号、姓名、高数、英语、政治、C++成绩)
【要求:】使用友元的不同形式加以实现
形式1:非成员函数作为友元函数
形式2:成员函数作为友元函数
形式3:友元类
形式4:类的组合
二、分析
设计Student和Score类
1.分别在Student和Score类中声明friend void show(Student student,Score score);,通过调用show函数实现非成员函数作为友元函数,之后通过main函数调用
2.设计Show2类,分别在Student和Score类中声明friend void Show2::visitinfo(Student student, Score score);,在Show2类中编写输出函数visitinfo,之后在main函数中调用
3.设计Show3类,分别在Student和Score类中声明friend class Show3;,在Show3中编写void visit(Student student,Score score)函数,之后通过Show3新建对象,调用该函数
4.设计Show4类,利用组合类在成员函数中定义Student stu与Score sc,之后通过Show4的构造函数调用Student类和Score类中的print函数
三、代码
💻提示:所有实验源码已在github整理
#include<iostream>
using namespace std;
#include<string>
class Student;
class Score;
class Show2
{
public:
void visitinfo(Student student,Score score);
};
class Student
{
friend void show(Student student,Score score);
friend void Show2::visitinfo(Student student, Score score);
friend class Show3;
public:
Student(string stu_id, string stu_name)
{
this->stu_id = stu_id;
this->stu_name = stu_name;
}
private:
string stu_id;
string stu_name;
};
class Score
{
friend void show(Student student,Score score);
friend void Show2::visitinfo(Student student, Score score);
friend class Show3;
public:
Score(double sc_math, double sc_english, double sc_politics, double sc_cpp)
{
this->sc_math = sc_math;
this->sc_english = sc_english;
this->sc_politics = sc_politics;
this->sc_cpp = sc_cpp;
}
private:
double sc_math;
double sc_english;
double sc_politics;
double sc_cpp;
};
void Show2::visitinfo(Student student,Score score)
{
cout << "2.成员函数作为友元函数" << endl;
cout << "学号 " << student.stu_id << " 姓名 " << student.stu_name << endl;
cout << "高数 " << score.sc_math << " 英语 " << score.sc_english << " 政治 " << score.sc_politics << " C++ " << score.sc_cpp << endl << endl;
}
class Show3
{
public:
void visit(Student student,Score score)
{
cout << "3.友元类" << endl;
cout << "学号 " << student.stu_id << " 姓名 " << student.stu_name << endl;
cout << "高数 " << score.sc_math << " 英语 " << score.sc_english << " 政治 " << score.sc_politics << " C++ " << score.sc_cpp << endl << endl;
}
};
void show(Student student,Score score)
{
cout << "1.非成员函数作为友元函数" << endl;
cout << "学号 "<<student.stu_id<<" 姓名 "<<student.stu_name<< endl;
cout << "高数 " << score.sc_math << " 英语 " << score.sc_english << " 政治 " << score.sc_politics << " C++ " << score.sc_cpp << endl << endl;
}
int main()
{
Student s1("192062116", "张帆");
Score ss1(80, 81, 82, 83);
show(s1,ss1);
Show2 s2;
s2.visitinfo(s1,ss1);
Show3 sh;
sh.visit(s1,ss1);
system("pause");
return 0;
}
#include <iostream>
#include <string>
using namespace std;
class Student
{
public:
Student() {};
Student(string stu_id, string stu_name)
{
this->stu_id = stu_id;
this->stu_name = stu_name;
}
void print()
{
cout << "4.组合类" << endl;
cout << "学号 " << stu_id << " 姓名 " << stu_name << endl;
}
private:
string stu_id;
string stu_name;
};
class Score
{
public:
Score() {};
Score(double sc_math, double sc_english, double sc_politics, double sc_cpp)
{
this->sc_math = sc_math;
this->sc_english = sc_english;
this->sc_politics = sc_politics;
this->sc_cpp = sc_cpp;
}
void print()
{
cout << "高数 " << sc_math << " 英语 " << sc_english << " 政治 " << sc_politics << " C++ " << sc_cpp << endl << endl;
}
protected:
double sc_math;
double sc_english;
double sc_politics;
double sc_cpp;
};
class Show
{
public:
Show(Student stu,Score sc)
{
stu.print();
sc.print();
}
private:
Student stu;
Score sc;
};
int main()
{
Student stu("192062116", "张帆");
Score sc( 80, 81, 82, 83);
Show(stu, sc);
system("pause");
return 0;
}
四、 结果
通过本次实验,学习了四种编写友元的方法。在成员函数作为友元函数时,有如下报错,Show2不能访问Student类中的私有成员。这里的格式不同其它三种,必须在开头提前说明Student和Score类以及visitinfo函数(把类的成员函数声明为友元函数,但不能访问私有成员的原因和解决办法_秃草是真的的博客-CSDN博客_声明了友元函数不能访问)

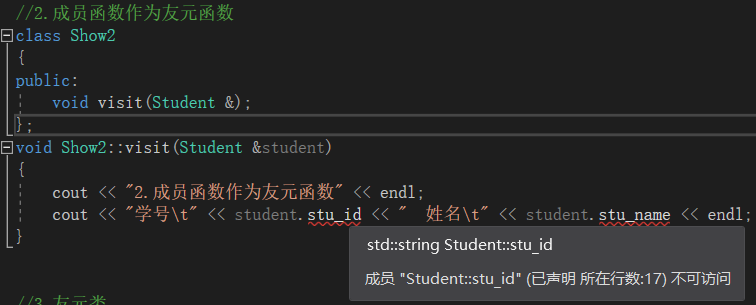
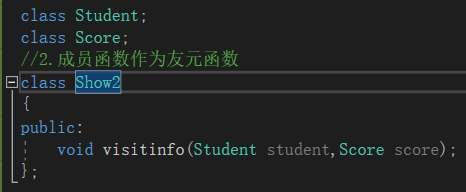
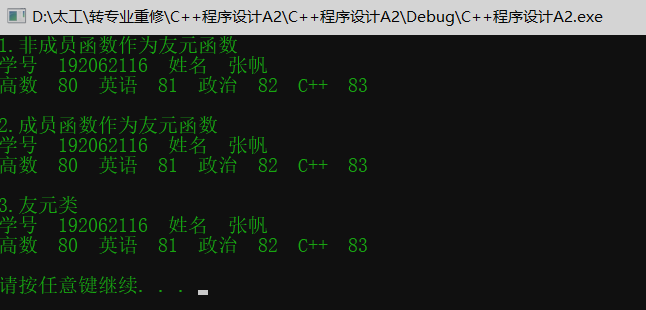
|