在学习了C++的类和对象后,可以动手写一个日期类,以此来巩固和运用类的知识。下面是博主写的日期类,若自己在写的过程中有不懂的地方,可以参考参考
源代码
Date.h
#include <iostream>
using std::cout;
using std::endl;
class Date
{
public:
bool IsLeapYear(int year);
int GetMonthDay(int year, int month);
Date(int year = 2022, int month = 5, int day = 19);
Date(const Date& d);
bool operator==(const Date& d) const;
bool operator!=(const Date& d) const;
Date& operator=(const Date& d);
bool operator<(const Date& d) const;
bool operator>(const Date& d) const;
bool operator<=(const Date& d) const;
bool operator>=(const Date& d) const;
Date operator+(int day) const;
Date& operator+=(int day);
Date operator-(int day) const;
Date& operator-=(int day);
Date& operator++();
Date operator++(int);
Date& operator--();
Date operator--(int);
int operator-(const Date& d) const;
void Print() const;
Date* operator&();
const Date* operator&() const;
friend std::ostream& operator<<(std::ostream& out, const Date& d);
friend std::istream& operator>>(std::istream& in, Date& d);
private:
int _year;
int _month;
int _day;
};
Date.cpp
#define _CRT_SECURE_NO_WARNINGS 1
#include "Date.h"
bool Date::IsLeapYear(int year)
{
if ((year % 400 == 0) || (year % 4 == 0 && year % 100 != 0))
return true;
else
return false;
}
int Date::GetMonthDay(int year, int month)
{
static int monthday[13] = { 0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 };
if (month == 2 && Date::IsLeapYear(year))
return monthday[month] + 1;
return monthday[month];
}
Date::Date(int year, int month, int day)
{
if (year > 0\
&& month > 0 && month <= 12\
&& day > 0 && day <= Date::GetMonthDay(year, month))
{
_year = year;
_month = month;
_day = day;
}
else
cout << "数据不合法" << endl;
}
Date::Date(const Date& d)
{
_year = d._year;
_month = d._month;
_day = d._day;
}
bool Date::operator==(const Date& d) const
{
return _year == d._year\
&& _month == d._month\
&& _day == d._day;
}
bool Date::operator!=(const Date& d) const
{
return !(*this == d);
}
Date& Date::operator=(const Date& d)
{
if (this != &d)
{
_year = d._year;
_month = d._month;
_day = d._day;
}
return *this;
}
bool Date::operator<(const Date& d) const
{
if ((_year < d._year) ||
(_year == d._year && _month < d._month) ||
(_year == d._year && _month == d._month && _day < d._day))
return true;
else
return false;
}
bool Date::operator>(const Date& d) const
{
return (d < *this);
}
bool Date::operator<=(const Date& d) const
{
return !(*this > d);
}
bool Date::operator>=(const Date& d) const
{
return !(*this < d);
}
Date Date::operator+(int day) const
{
Date ret(*this);
ret += day;
return ret;
}
Date& Date::operator+=(int day)
{
if (day < 0)
return *this -= (-day);
_day += day;
while (_day > Date::GetMonthDay(_year, _month))
{
_day -= Date::GetMonthDay(_year, _month);
_month++;
if (_month > 12)
{
_month = 1;
_year++;
}
}
return *this;
}
Date Date::operator-(int day) const
{
Date ret(*this);
ret -= day;
return ret;
}
Date& Date::operator-=(int day)
{
if (day < 0)
return *this += (-day);
_day -= day;
while (_day <= 0)
{
_month--;
if (_month == 0)
{
_month = 12;
_year--;
}
_day += Date::GetMonthDay(_year, _month);
}
return *this;
}
Date& Date::operator++()
{
*this += 1;
return *this;
}
Date Date::operator++(int)
{
Date d(*this);
*this += 1;
return d;
}
Date& Date::operator--()
{
*this -= 1;
return *this;
}
Date Date::operator--(int)
{
Date d(*this);
*this -= 1;
return d;
}
int Date::operator-(const Date& d) const
{
Date max(*this);
Date min(d);
int flat = 1;
if (max < min)
{
flat = -1;
max = d;
min = *this;
}
int day = 0;
while (max != min)
{
min++;
day++;
}
return day * flat;
}
void Date::Print() const
{
cout << "year - " << _year << " - month - " << _month << " - day - " << _day << endl;
}
void Date::Print() const
{
cout << "year - " << _year << " - month - " << _month << " - day - " << _day << endl;
}
Date* Date::operator&()
{
return this;
}
const Date* Date::operator&() const
{
return this;
}
std::ostream& operator<<(std::ostream& out, const Date& d)
{
out << d._year << "-" << d._month << "-" << d._day << endl;
return out;
}
std::istream& operator>>(std::istream& in, Date& d)
{
in >> d._year >> d._month >> d._day;
if (!(d._year > 0\
&& d._month > 0 && d._month <= 12\
&& d._day > 0 && d._day <= d.GetMonthDay(d._year, d._month)))
{
cout << "数据不合法" << endl;
exit(-1);
}
return in;
}
日期的构造函数
首先日期中三个成员变量:年,月,日。当创建一个日期类,要对日期的合法性进行判断,年要大于0,月要在1~12的范围中,天数要根据月份进行判断,还有平年闰年的2月天数不同。
这就有了if的判断条件,但麻烦的是天数最大值要怎么判断,我将其封装成一个函数,输入年和月就能得到该年该月的最大天数。
int Date::GetMonthDay(int year, int month)
{
static int monthday[13] = { 0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 };
if (month == 2 && Date::IsLeapYear(year))
return monthday[month] + 1;
return monthday[month];
}
bool Date::IsLeapYear(int year)
{
if ((year % 400 == 0) || (year % 4 == 0 && year % 100 != 0))
return true;
else
return false;
}
Date::Date(int year, int month, int day)
{
if (year > 0\
&& month > 0 && month <= 12\
&& day > 0 && day <= Date::GetMonthDay(year, month))
{
_year = year;
_month = month;
_day = day;
}
else
cout << "数据不合法" << endl;
}
两个日期之间的比较
比较的运算符有很多(像什么>,<,>=,==),但实际只要实现其中的两个运算符
bool Date::operator==(const Date& d)
{
return _year == d._year\
&& _month == d._month\
&& _day == d._day;
}
bool Date::operator<(const Date& d)
{
if ((_year < d._year) ||
(_year == d._year && _month < d._month) ||
(_year == d._year && _month == d._month && _day < d._day))
return true;
else
return false;
}
剩下的运算符重载复用这两个函数就行了,比如我要实现>的重载,>是<和=的逻辑反(一个数如果不大于并且不等于一个数,那么就是小于这个数)
bool Date::operator!=(const Date& d)
{
return !(*this == d);
}
bool Date::operator>(const Date& d)
{
return !(*this <= d);
}
bool Date::operator<=(const Date& d)
{
return (*this < d || *this == d);
}
bool Date::operator>=(const Date& d)
{
return !(*this < d);
}
bool Date::operator!=(const Date& d)
{
return !(*this == d);
}
赋值运算符的重载
这里提一下赋值与拷贝构造之间的区别,赋值是将一个存在对象的值赋值给另一个存在对象的值,而拷贝构造是用一个存在对象的值初始化一个不存在的对象
Date d1(2022, 5, 21);
Date d2(d1);
Date d2 = d1;
Date d3;
d3 = d1;
使用赋值的使用,经常会有这样的连等
int a = 10;
int b = 2;
int c = 3;
c = a = b;
=的优先级是从右到左,所以上面的连等是先将b赋值给a,再把a的值赋值给c,因此赋值运算符的重载函数的返回值一个是被赋值的对象(操作数左边的对象)。
Date& Date::operator=(const Date& d)
{
if (this != &d)
{
_year = year;
_month = month;
_day = day;
}
return *this;
}
没有复用的运算符可以看源代码
加减运算符
首先加减运算符也要支持连等,a = b + c + d,优先级是从右向左,所以返回的也是左操作数。还有就是+和+=不一样,+=的操作数只有一个,并且会改变操作数的值,而+的操作数有两个,不会改变操作数的值
Date& Date::operator+=(int day)
{
if (day < 0)
return *this -= (-day);
_day += day;
while (_day > GetMonthDay(_year, _month))
{
_day -= GetMonthDay(_year, _month);
_month++;
if (_month == 13)
{
_month = 1;
_year++;
}
}
return *this;
}
Date Date::operator+(int day)
{
Date ret(*this);
ret += day;
return ret;
}
Date& Date::operator-=(int day)
{
if (day < 0)
return *this += (-day);
_day -= day;
while (_day < 0)
{
_month--;
if (_month == 0)
{
_month = 12;
_year--;
}
_day -= GetMonthDay(_year, _month);
}
return *this;
}
Date Date::operator-(int day)
{
Date ret(*this);
ret -= day;
return ret;
}
++这样的运算符也是复用+=,但++分为前置和后置,重载的函数都没参数(++为单操作数,但类中的函数隐藏了this指针),要怎么区别前置和后置呢?
Date& Date::operator++()
{
return *this += 1;
}
Date Date::operator++(int)
{
Date ret(*this);
*this += 1;
return ret;
}
这里解释下为什么后置++的参数中有一个int:无论是前置还是后置,它们都是没有参数的,编译器为了区分前置后置,规定如果参数中出现了int则为后置,否则为前置(当形参中出现了类型但没有名字,表示该形参不重要,或者是接收了但不使用)。所以int是后置的一种标识符,供编译器区别调用。
Date& Date::operator--()
{
return *this -= 1;
}
Date Date::operator--(int)
{
Date ret(*this);
*this -= 1;
return ret;
}
但两者返回值不同,前置能返回引用,后置返回的是拷贝的局部变量,出了函数销毁不能返回引用。
两日期相减
要得到两个日期之间相差几天,就要知道两者之间有几个闰年,不仅如此还要知道大小月,实现起来有点复杂,这里提供一个简单的实现。知道两个日期中较小的,将较小的日期不断++,一边++一边计录天数,直到两者相等,记录的天数就是两这相差的天数
int Date::operator-(Date d)
{
Date max(*this);
Date min(d);
int flat = 1;
if (max < min)
{
max = d;
min = *this;
flat = -1;
}
int day = 0;
while (min != max)
{
min++;
day++;
}
return day * flat;
}
流提取和流插入的重载
首先要理解cout和cin是什么,两者都是一个对象,cout是ostream类的对象,cin则是istream类的对象,而cout和cin为什么支持任意类型数据的输入与输出(对于内置类型)?原因是ostream类和istream类中对<<和>>这两个运算符重载了,当输入一个任意类型的数据时,函数会自动匹配对应的类型 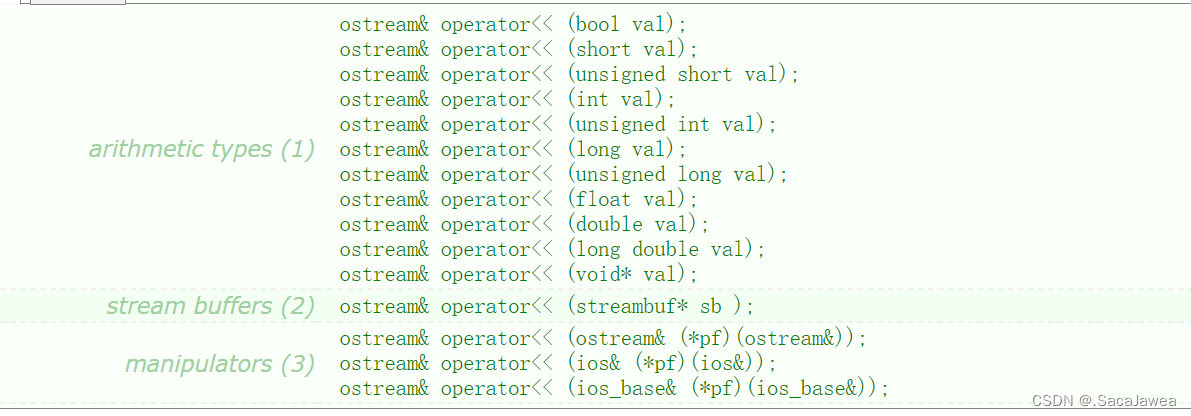 知道了cin和cout的类型,我们就能对<<和>>重载了。
void Date::operator<<(std::ostream& out)
{
out << _year << "-" << _month << "-" << _day << endl;
}
void Date::operator>>(std::istream& in)
{
in >> _year >> _month >> _day;
}
使用时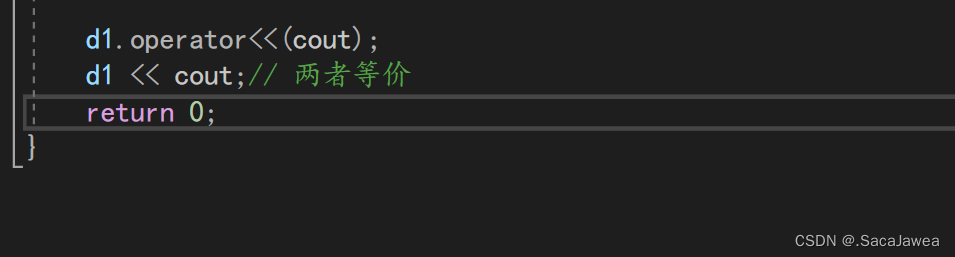 但有一个问题,用cout不都是cout << d1,这样的吗?怎么重载过后是d1 << cout,相反的?这样的重载看起来很奇怪,需要其他方式进行重载。
上面函数重载奇怪的原因是:类中成员函数的第一个形参是this指针(第一个永远都是this指针),所以调用重载时第一个实参也只能是Date类的对象。要解决这个问题,只能将重载定义在类外,使函数的第一个参数不是this指针 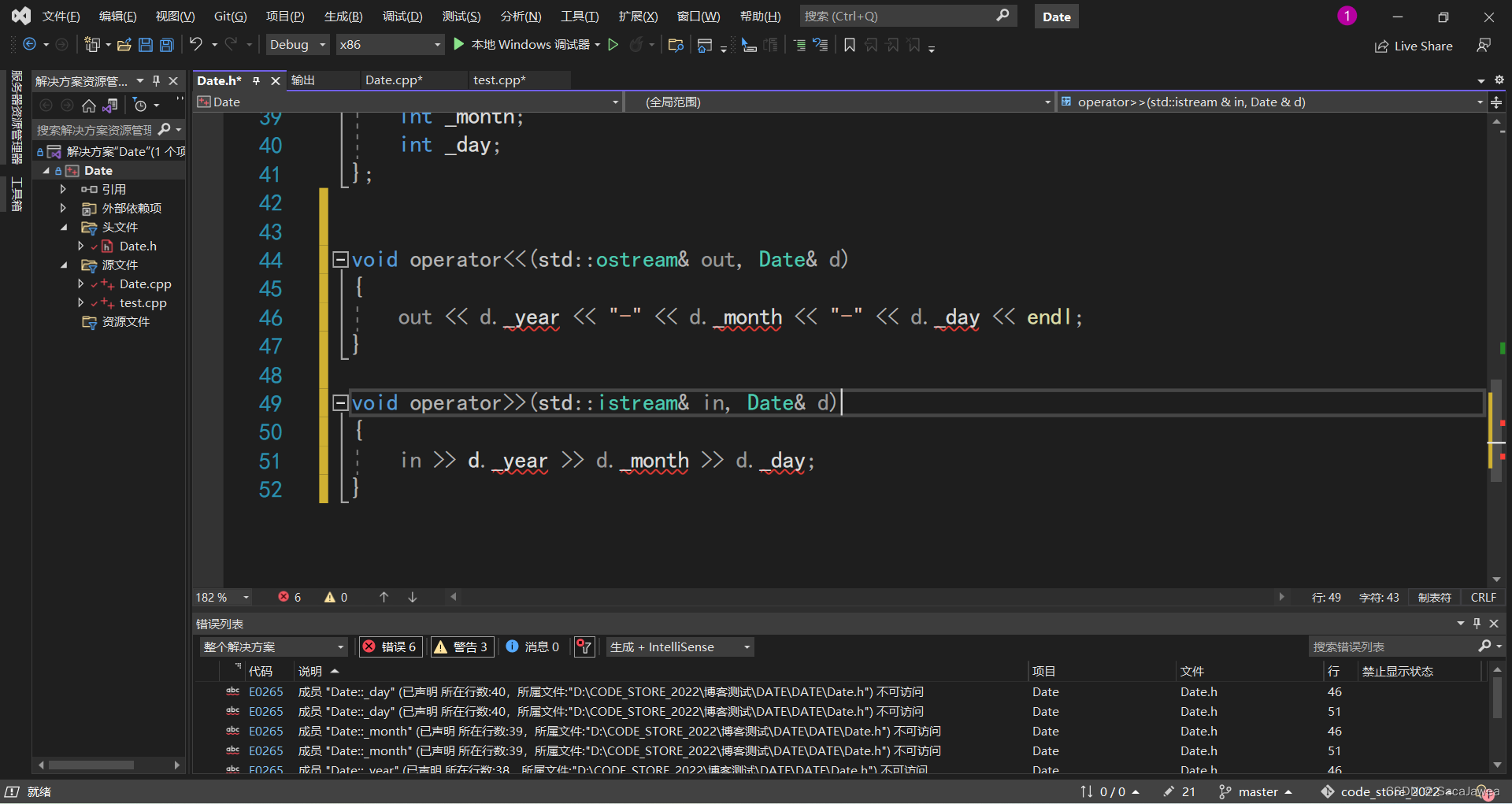 定义在类外后,又有一个问题,Date的私有成员不能访问,因此需要将这两个重载声明成Date类的友元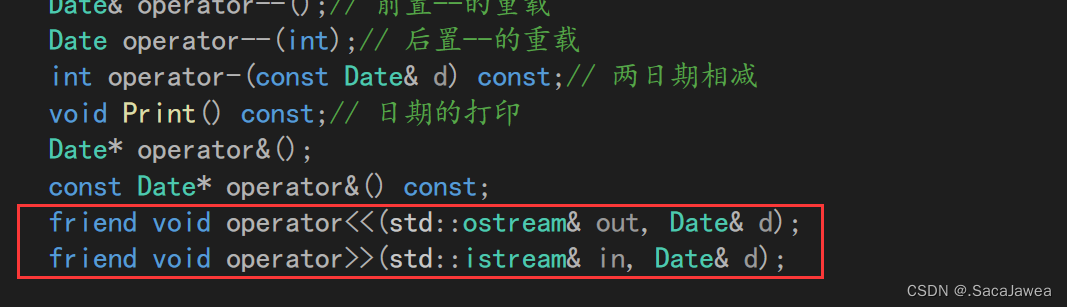 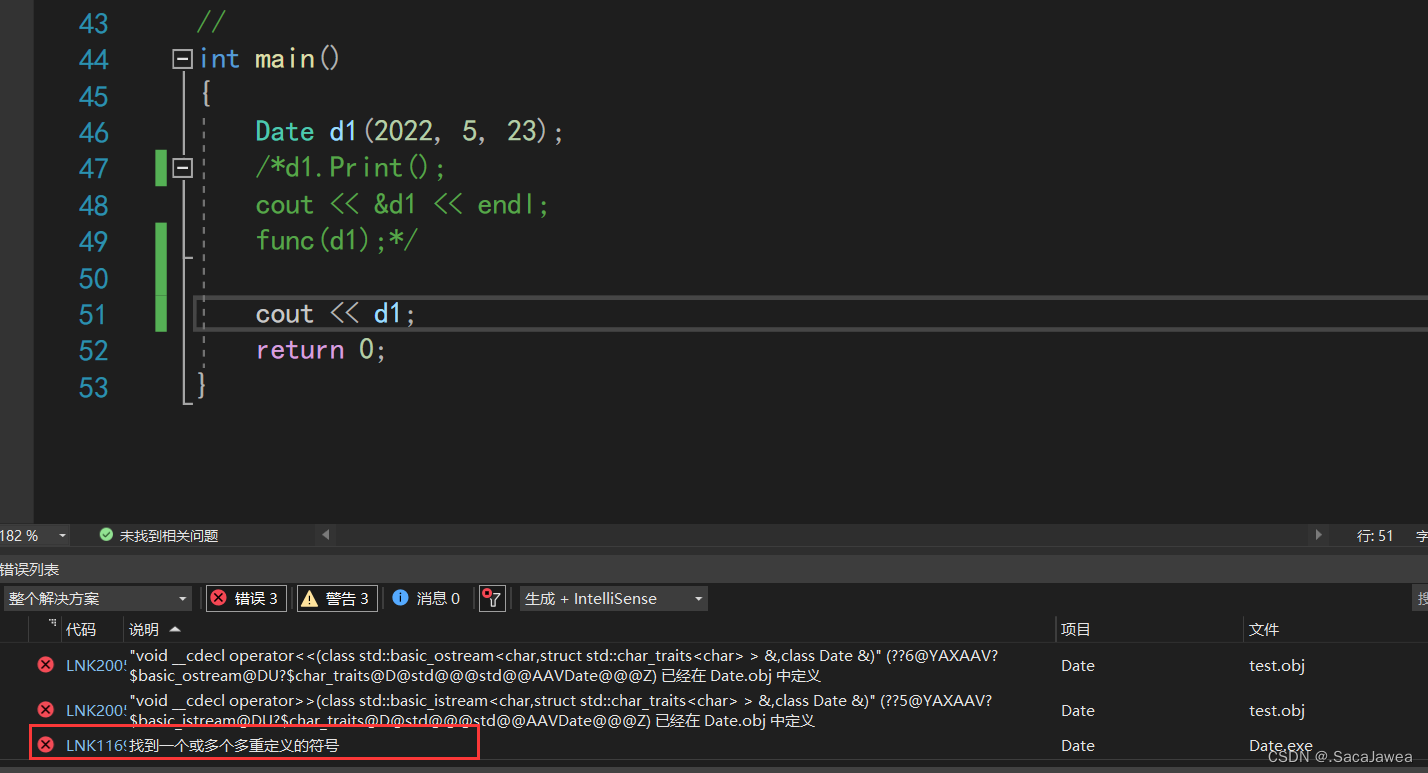 此时再调用的话又有一个链接错误,“找到一个或多个多重定义的符号”,原因是:我将两个函数的重载定义在头文件中,头文件会在两个源文件中展开,源文件合并后这两个重载函数就会出现两次,属于重定义。解决方法就是将函数定义在Date.cpp源文件中,声明在Date.h头文件中
这样的话,这两个函数就值剩下一个问题,就是连续的使用。比如输入多个Date对象,cout << d1 << d2 << d3 << endl,由于重载的函数没有返回值,程序会报错(运算符优先级为从左到右,先输出d1),所以重载的函数需要返回cout,也就是ostream的对象。最后的函数是
std::ostream& operator<<(std::ostream& out, const Date& d)
{
out << d._year << "-" << d._month << "-" << d._day << endl;
return out;
}
std::istream& operator>>(std::istream& in, Date& d)
{
in >> d._year >> d._month >> d._day;
return in;
}
关于const
 当构造函数和拷贝构造函数都没加const时  为什么会报错?这里涉及到权限放大的问题 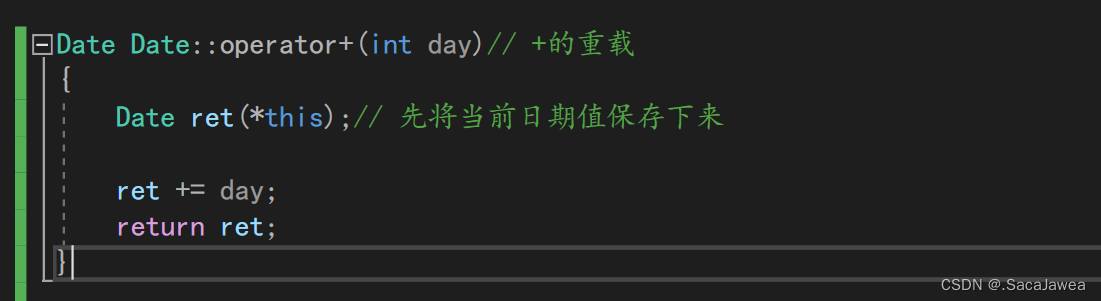 +的重载函数返回ret的值,由于ret是局部变量,函数在返回前拷贝ret的值到临时变量中,而临时变量具有常性,可以理解为是const修饰的,不能修改,d1 + 1得到的返回值是const修饰的临时变量,而Date d3 = d1 + 1是一个拷贝构造函数,该表达式等价于Date d3(d1 + 1) 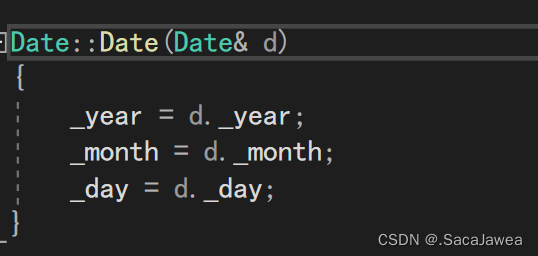 d1 + 1是一个临时变量,作为拷贝构造函数的参数,而该拷贝构造函数的形参为Date&,当引用没有const修饰,实体却有const修饰,属于权限放大,程序当然报错。
同理赋值重载的形参也没加const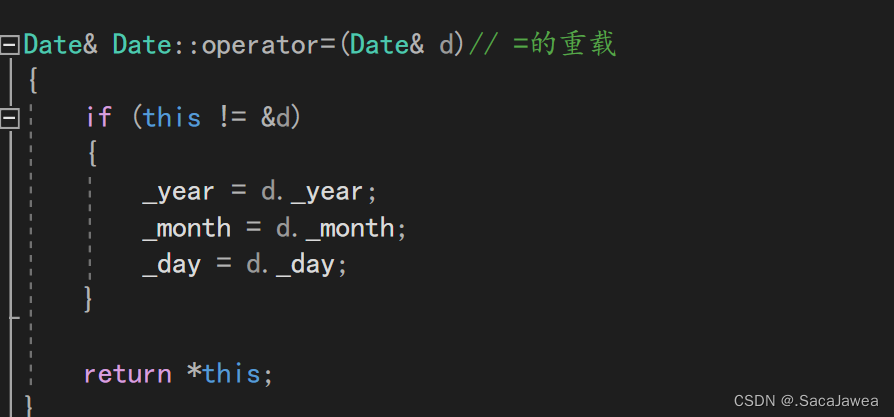 在加上const后程序不再报错
之前一直说当函数不改变对象时,需要对形参的类型加上const表示只读以保护变量的值。比如打印函数,void Print(const int* i),打印一个整形的值,不需要对它进行改变,所以加上const,但看下面的程序,为什么会报错呢? 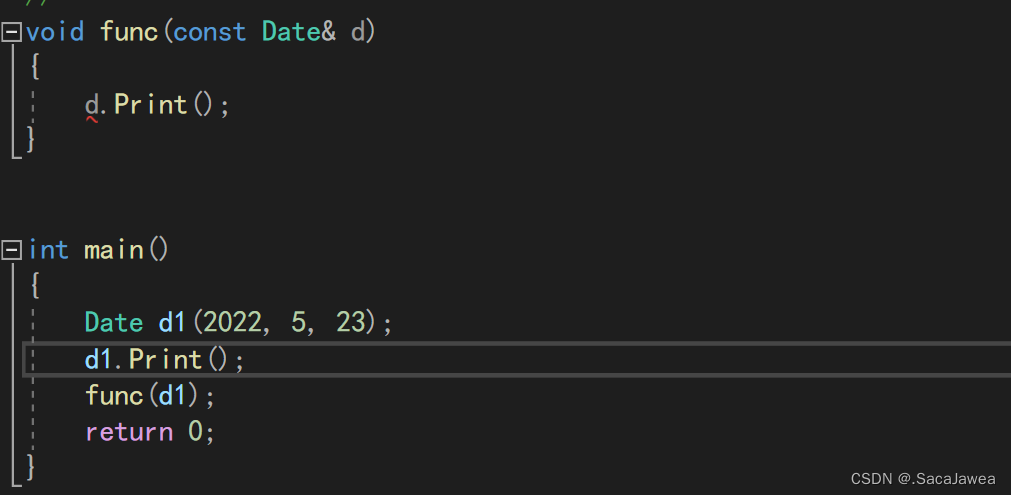   这里给出Print函数的定义,可以看到由于this指针是隐藏在形参中的,所以定义打印这样的只有类对象一个参数的函数时,是不用写参数的。将this显式的写出是这样的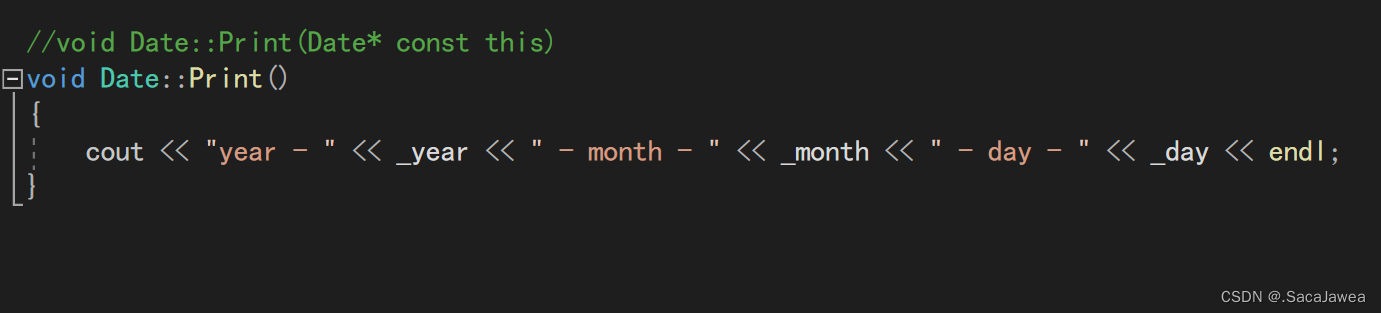 在类的那篇博客中有提到,编译器默认this指针的类型是* const,const在*的右边表示this指针的值不能改变,但能改变this指针指向的对象。
调用d1.Print()时,编译器处理后将d1的地址作为函数形参传给this指针,d1.Print(&d1)。这时的&d1类型是Date *,但调用func函数时,将d1作为形参传给一个引用,该引用用const修饰表示只读,func函数中调用d.Print()时,函数参数也是&d,但由于d是一个const引用,所以这个&d的类型是const Date *,表示这个指针指向对象不能修改,是只读属性。而Date类的Print定义时,编译器默认this的类型是Date * const,const只保护了this没有保护this指针指向的对象,所以将const Date *传给Date * const是一个权限放大的行为,权限放大会引起安全问题,程序报错。
要解决这个问题就必须给this加上const,而this指针是被隐藏的,不能显式的写出,那要怎么加呢? 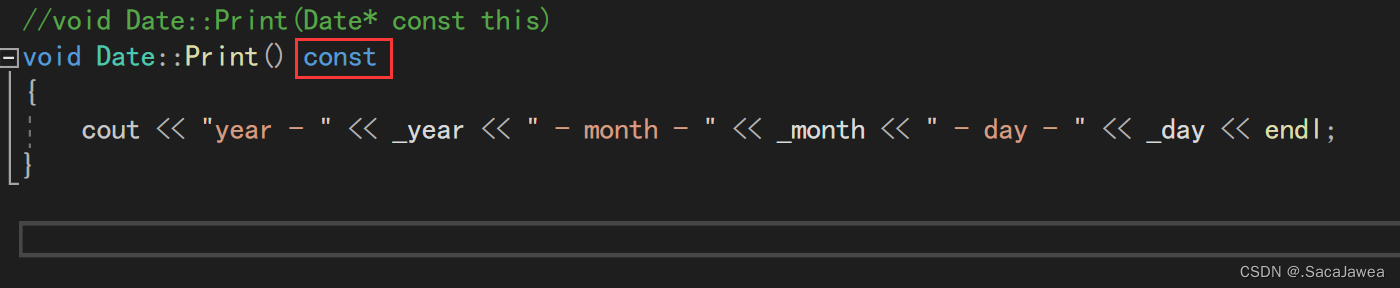 在整个函数的后面加上const就表示this指针指向的对象为只读类型,不能修改 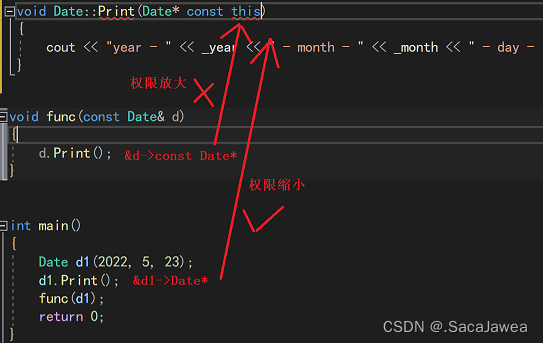 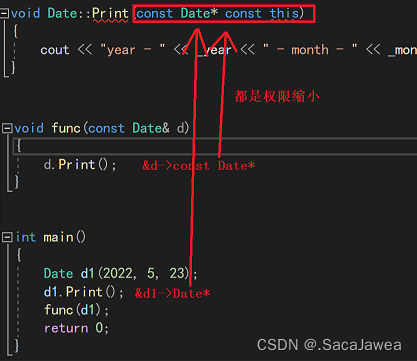
所以对于不修改成员变量的成员7函数,我们都要尽量加上const,这样普通对象和const对象都可以调用该成员函数
还有一种情况
int main()
{
Date d1(2022, 5, 24);
(d1 + 10).Print();
}
d1 + 10是+的重载,重载函数的返回值是日期类,调用Print去打印这个日期,看上去没有什么问题。但+的重载函数返回的是一个临时变量 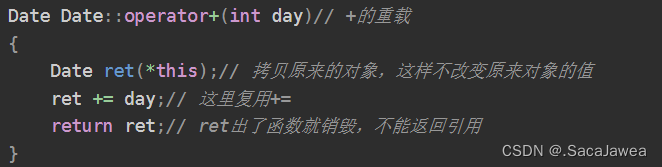 由于ret要销毁,为了返回ret的值,将ret的值拷贝到一个临时变量中,该临时变量具有常属性,也就是const修饰,调用Print时,Print函数会传该临时变量的地址,地址类型为const Date*,若Print函数的this指针没用const修饰,属于权限放大,理论上程序会报错(但可能编译器对其优化,使得地址的类型也为const Date*,在vs中程序不会报错)。
虽然这种情况不会报错,但也验证了对于不修改参数的函数,需要用const修饰参数(当const修饰this指针时,const写在函数体的最后)。
几个问题
1.const对象能调用非const成员函数吗?
2.非const对象能调用const成员函数吗?
3.cosnt成员函数能调用非const成员函数吗?
4.非const成员函数能调用const成员函数吗?
|