C_lesson6
- 1.循环 while
-
- 2.for循环
- 2.1 break:1,2,3,4
- 2.2 continue:1,2,3,4,6,7,8,9
- 2.3 for循环变种
- 2.4 for循环中的陷阱,注意是== 还是 =
- 3.do...while循环
-
- 4.练习
-
- 5.goto语句
-
1.循环 while
(1)while循环定义:先判定后执行
while(表达式){循环语句;更新条件};
组成:条件初始化+条件判定+条件更新
(2)break:
在循环中只要遇到break,就停止后期的所有的循环,直接终止循环。
while中的break是用于永久终止循环的。
(3)continue:
continue是用于终止本次循环的,也就是本次循环中continue后边的代码不会再执行,
而是直接跳转到while语句的判断部分,进行下一次循环的入口判断。
(4)break:从控制台死循环获取字符,如何退出死循环。
continue的例子:从输入的字符中,获取0-9数
1.1 while循环
#if 0
#include <stdio.h>
#include <windows.h>
int main()
{
int flag = 0;
while(flag<10)
{
printf("%d",flag);
flag++;
}
printf("\n");
system("pause");
return 0;
}
#endif
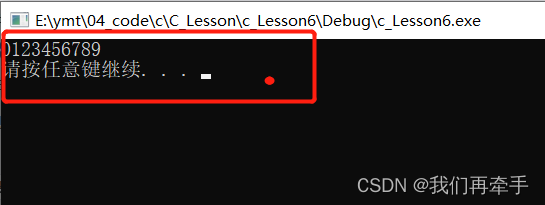
1.2 break:结束循环
#if 1
#include <stdio.h>
#include <windows.h>
int main()
{
int flag = 0;
while(flag<10)
{
printf("%d ",flag);
flag++;
if(flag>5)
{
break;
}
}
printf("\n");
system("pause");
return 0;
}
#endif
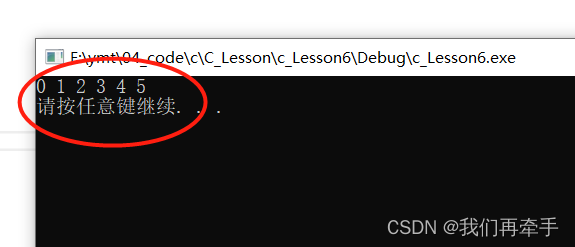
1.3 continue:这里一直6进行死循环,因为continue退出了本次循环,进入下一次循环。没有条件更新。
#if 1
#include <stdio.h>
#include <windows.h>
int main()
{
int flag = 0;
while(flag<10)
{
if(flag > 5)
{
printf("%d ",flag);
continue;
}
printf("%d ",flag);
flag++;
}
printf("\n");
system("pause");
return 0;
}
#endif
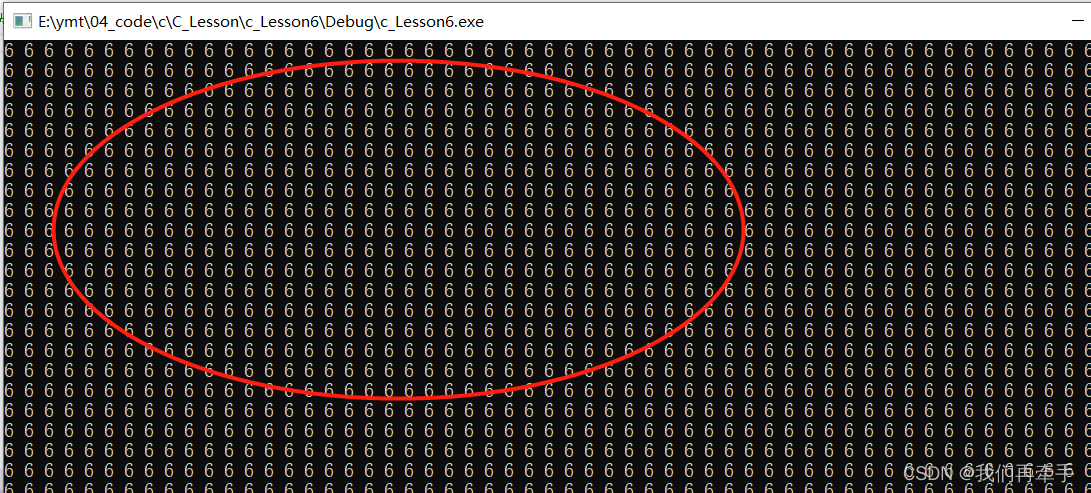
1.4 解决continue因为无条件更新造成了死循环。因为continue结束了5的循环,所以运行结果是0,1,2,3,4,6,7,8,9
#if 1
#include <stdio.h>
#include <windows.h>
int main()
{
int flag = 0;
while(flag<10)
{
if(flag == 5)
{
flag++;
continue;
}
printf("%d ",flag);
flag++;
}
printf("\n");
system("pause");
return 0;
}
#endif
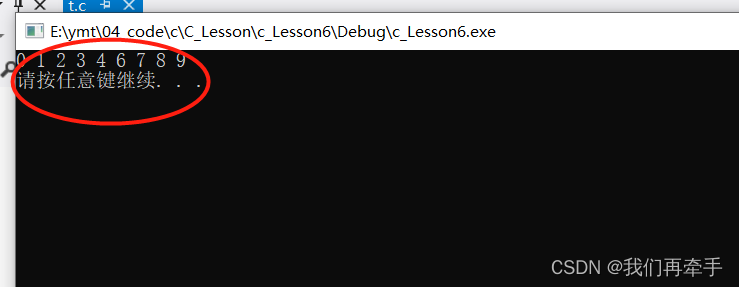
1.5 break例子:从控制台死循环获取字符,如何退出死循环。
#if 1
#include <stdio.h>
#include <windows.h>
int main()
{
int ch = 0;
while ((ch = getchar()) != EOF)
{
putchar(ch);
}
system("pause");
return 0;
}
#endif
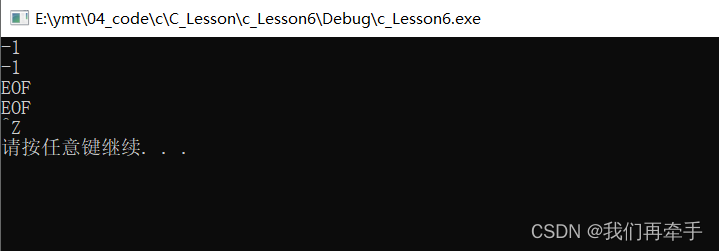
int ch = getchar();获得一个字符 putchar(ch);输出一个字 #define EOF -1 注意: (1)break也能退出死循环 (2)ctrl+z,在对话框中输入完毕 (3)EOF -1均不能使下面程序退出死循环
#if 1
#include <stdio.h>
#include <windows.h>
int main()
{
while(1)
{
int ch = getchar();
if (ch == EOF)
{
printf("EOF=%d ch = %d\n",EOF,ch);
break;
}
putchar(ch);
}
system("pause");
return 0;
}
#endif
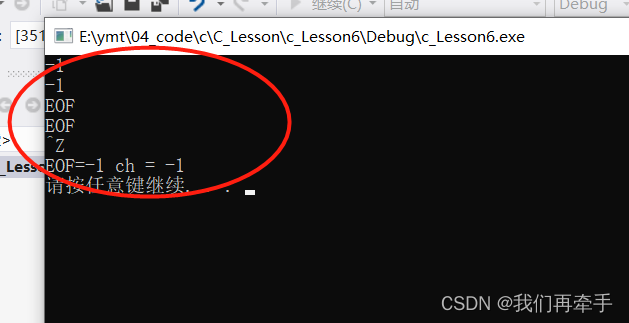
1.6 continue实例:从输入的一堆字符中,获取0-9数
0,‘0’, '\0’三个的区别,ASCII码表是数据和图形的一种映射关系。 0 --> 0 ‘0’ --> 48 ‘\0’–> 0
#if 1
#include <stdio.h>
#include <windows.h>
int main()
{
int ch = 0;
while ((ch = getchar()) != EOF)
{
if((ch<'0')|| (ch>'9'))
{
continue;
}
putchar(ch);
}
system("pause");
return 0;
}
#endif
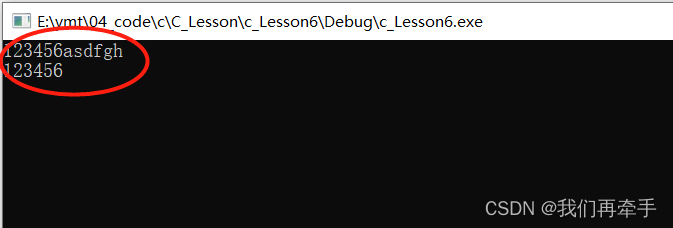
2.for循环
(1)for循环定义:先判定后执行
for(表达式1;表达式2;表达式3){循环语句;}
表达式1 表达式1为初始化部分,用于初始化循环变量的。
表达式2 表达式2为条件判断部分,用于判断循环时候终止。
表达式3 表达式3为调整部分,用于循环条件的调整。
注:
1.不可在for循环体内修改循环变量,防止 for 循环越界,而失去控制。
2.建议for语句的循环控制变量的取值采用“前闭后开区间”写法。
3.for死循环:for(;;){语句;}
(2)break:结束循环
(3)continue:结束本次循环,进入下一次循环
注:for循环中的break、continue和while循环类似,只不过for循环continue时不存在死循环,由于条件更新已经存在。
(4)for循环变种。
2.1 break:1,2,3,4
#if 0
#include <stdio.h>
#include <windows.h>
int main()
{
int i = 0;
for(i=1; i<=10; i++)
{
if(i == 5)
{
break;
}
printf("%d ",i);
}
printf("\n");
system("pause");
return 0;
}
#endif
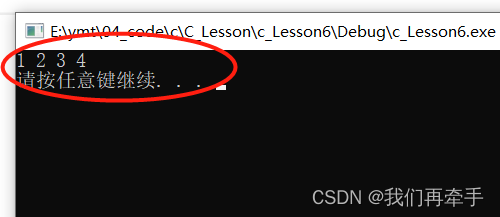
2.2 continue:1,2,3,4,6,7,8,9
#if 1
#include <stdio.h>
#include <windows.h>
int main()
{
int i = 0;
for(i=1; i<=10; i++)
{
if(i == 5)
{
continue;
}
printf("%d ",i);
}
printf("\n");
system("pause");
return 0;
}
#endif
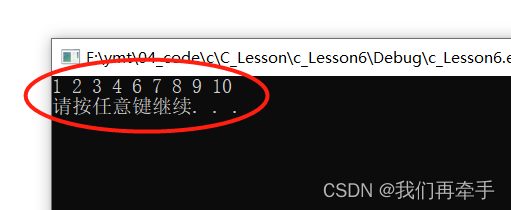
2.3 for循环变种
第一次:x = 0,y = 0 hehe 第二次:x = 1,y = 1 hehe 第三次:x = 2,y = 2;x不成立,退出循环
#if 0
#include <stdio.h>
#include <windows.h>
int main()
{
int x, y;
for (x = 0, y = 0; x<2 && y<5; ++x, y++)
{
printf("hehe\n");
}
system("pause");
return 0;
}
#endif
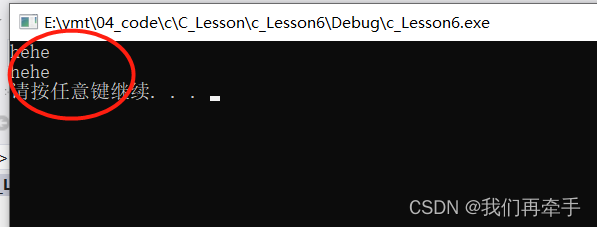
2.4 for循环中的陷阱,注意是== 还是 =
第一次:i = 0;k = 0 第二次:i = 1;k = 2; 2 = 0相当于k为0,所以退出循环,k = 0
#if 0
#include <stdio.h>
#include <windows.h>
int main()
{
int i = 0;
int k = 0;
for(i =0,k=0; k=0; i++,k++)
{
k++;
}
printf("%d\n",k);
system("pause");
return 0;
}
#endif
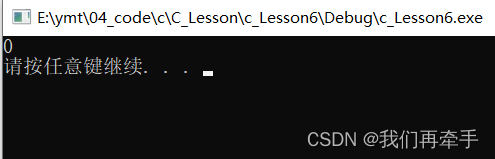
3.do…while循环
(1)do...while循环的定义
do{循环语句;更新;}while(判定条件);
(2)break、continue和while循环一样
(3)不管条件为真还是为假,都会先执行一次。即先执行,后判定。
3.1 do…while
#if 1
#include <stdio.h>
#include <windows.h>
int main()
{
int i = 0;
do{
printf("%d ",i);
i++;
}while(i<10);
printf("\n");
system("pause");
return 0;
}
#endif
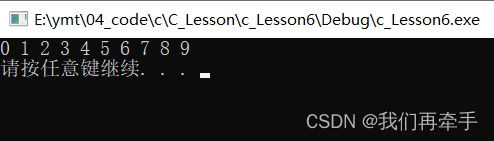
3.2 break
#if 1
#include <stdio.h>
#include <windows.h>
int main()
{
int i = 0;
do{
i++;
if(i == 5)
{
break;
}
printf("%d ",i);
}while(i<10);
printf("\n");
system("pause");
return 0;
}
#endif
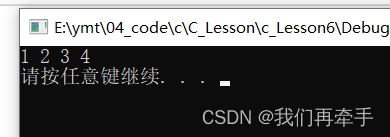
3.3 continue:这块和while循环一样,要注意防止死循环,更新条件一定要写在前面。
#if 1
#include <stdio.h>
#include <windows.h>
int main()
{
int i = 0;
do{
i++;
if(i == 5)
{
continue;
}
printf("%d ",i);
}while(i<10);
printf("\n");
system("pause");
return 0;
}
#endif
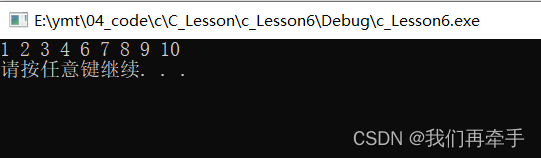
3.4 先执行,后判定。
#if 1
#include <stdio.h>
#include <windows.h>
int main()
{
int i = 0;
do{
i++;
printf("%d ",i);
}while(0);
printf("\n");
system("pause");
return 0;
}
#endif
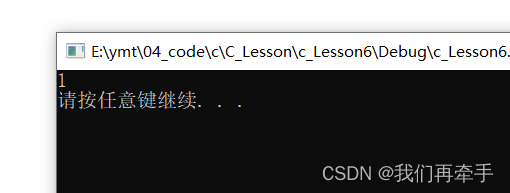
4.练习
(1)1.计算 n的阶乘。
(2)计算 1!+2!+3!+……+10!
(3)遍历查找数组元素
(4)在一个有序数组中查找具体的某个数字n。
编写int binsearch(int x, int v[], int n);
功能:在v[0]<=v[1]<=v[2]<= ….<=v[n-1]的数组中查找x.
(5)编写代码,演示多个字符从两端移动,向中间汇聚。
(6)编写代码实现,模拟用户登录情景,并且只能登录三次。
(只允许输入三次密码,如果密码正确则提示登录成,如果三次均输入错误,则退出程序。
(7)玩数字游戏
4.1 计算 n的阶乘。
1.控制台/键盘输入的数字,比如10,其实就是字符,一个是1,一个是0. 2.而scanf(“%d”,&x);其实就是格式化输入,将1和0两个字符转为十进制数字10,然后赋值给n。则这里n里面放的就是格式化后的值。
#if 1
#include <stdio.h>
#include <windows.h>
int Factorial(int _x)
{
int ret = 1;
for(; _x>0; _x--)
{
if(_x == 1)
{
printf("%d",_x);
}
else
{
printf("%d*",_x);
}
ret *= _x;
}
return ret;
}
int main()
{
int x = 0;
int result = 0;
printf("请输入一个数:");
scanf("%d",&x);
printf("%d! = ",x);
result = Factorial(x);
printf("= %d\n", result);
system("pause");
return 0;
}
#endif
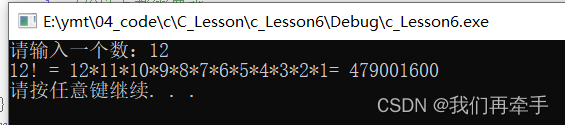
4.2 计算 1!+2!+3!+……+10!
#if 1
#include <stdio.h>
#include <windows.h>
int Factorial(int _x)
{
int ret = 1;
printf("%d! = ",_x);
for(; _x>0; _x--)
{
if(_x == 1)
{
printf("%d",_x);
}
else
{
printf("%d*",_x);
}
ret *= _x;
}
printf(" = %d\n", ret);
return ret;
}
int main()
{
int i = 1;
int result = 0;
for(;i<10;i++){
result += Factorial(i);
}
printf("result = %d\n", result);
system("pause");
return 0;
}
#endif
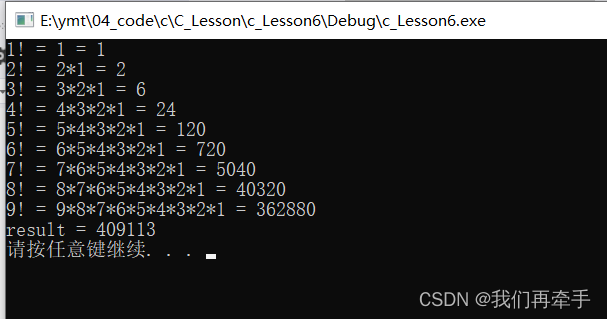
4.3 遍历查找数组元素
#if 0
#include <stdio.h>
#include <windows.h>
int Find(int _arr[],int _num,int _index)
{
int i = 0;
for(;i<=_num;i++){
if(_arr[i] == _index){
return i;
}
}
return -1;
}
int main()
{
int arr[] = {1,2,3,4,5,6,7,8,9,10};
int num = sizeof(arr)/sizeof(arr[0]);
int index = 0;
printf("请输入要查找的数:");
scanf("%d",&index);
printf("%d\n",Find(arr, num, index));
system("pause");
return 0;
}
#endif
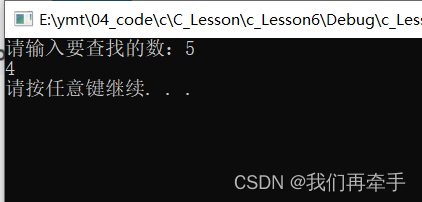
4.4 二分查找法:查找的过程就是排出的过程。
思想:
1.定义一个有序数组
2.计算出数组元素的个数
3.输入你要查找的数
4.二分查找函数:
a.传参:数组名,元素个数,查找的数
b.二分查找,则就是对半查找,即求出mid,那就需要定义start和end
c.如果你查找的数大于中间元素,则end不变,start=mid+1;
如果你查找的数小于中间元素,则start不变,end=mid-1;
如果查找的数等于中间元素,那就返回中间元素下标。
没有找到就返回-1.
d.你并不是一次就能查找到,需要多次二分查找才能找到索要查找的数,因此需要循环,而循环的条件就是start<=end.
5.二分查找相比于遍历查找的优势:
遍历每次排除一个元素;而二分查找每次排除一半元素,大大提高了效率。
#if 1
#include <stdio.h>
#include <windows.h>
int BinaryLookup(int _arr[],int _num,int _index)
{
int end = _num -1;
int start = 0;
while(start<=end)
{
int mid = end + start/2;
if(_index<_arr[mid]){
end = mid - 1;
}else if(_index>_arr[mid]){
start = mid + 1;
}else{
return mid;
}
}
return -1;
}
int main()
{
int arr[] = {1,2,3,4,5,6,7,8,9,10};
int num = sizeof(arr)/sizeof(arr[0]);
int index = 0;
printf("请输入要查找的数:");
scanf("%d",&index);
printf("%d\n",BinaryLookup(arr, num, index));
system("pause");
return 0;
}
#endif
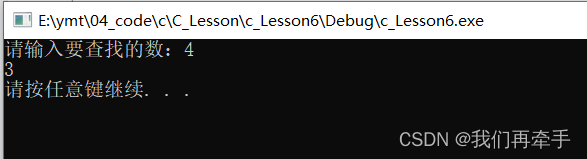
4.5 编写代码,演示多个字符从两端移动,向中间汇聚。
思想:
1.定义两个相同空间的数组。
2.字符汇聚显示函数:
a.传参:两个数组名
b.既然要向中间汇聚,即需要知道start和end,start=0,end=strlen(arr1)-1;
c.字符汇聚需要多次汇聚,因此需要循环来实现,而判定条件就是start<=end
d.两个数组进行对应替换就ok;
e:显示替换后的数组,要想看过程使用\n,要向看动态显示,即使用\r。并使用Sleep()函数。
\n:回车+换行,而不仅仅是换行,printf("%s\n",_arr2);
\r:回车,一个数字在同一个位置进行更新,printf("%s\r",_arr2);
#if 1
#include <stdio.h>
#include <windows.h>
void CharacterConvergenceShow(char _arr1[], char _arr2[])
{
int start = 0;
int end = strlen(_arr1) - 1;
for(; start<=end; start++,end--)
{
_arr2[start] = _arr1[start];
_arr2[end] = _arr1[end];
printf("%s\r",_arr2);
Sleep(1000);
}
printf("\n");
}
int main()
{
char arr1[] ="hello world!";
char arr2[] ="************";
CharacterConvergenceShow(arr1,arr2);
system("pause");
return 0;
}
#endif
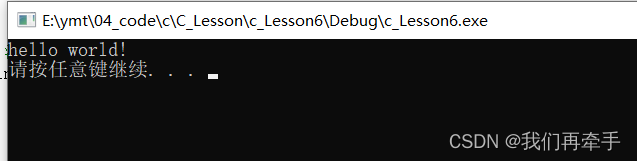
4.6 补充4.5,动态显示。
思想:
1.定义一个数组,将元素放入里面。
2.死循环让其输出每个元素个数。初始化;while(1){语句;更新条件;}
a.语句:将元素依次打印出来;因为了让其在一个位置动态显示,则\r,而不是\n.
b.更新条件就是下标的i++;但要注意,你一直死循环的是4个元素,所以要i = i%4
c.为了看其动态,则Sleep()函数。
#if 1
#include <stdio.h>
#include <windows.h>
void show()
{
const char arr1[] ="|/-\\";
int i = 0;
while(1)
{
printf("%c\r",arr1[i]);
i++;
Sleep(100);
i = i%4;
}
}
int main()
{
show();
system("pause");
return 0;
}
#endif
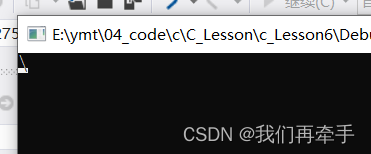
4.7 编写代码实现,模拟用户登录情景,并且只能登录三次。(只允许输入三次密码,如果密码正确则提示登录成,如果三次均输入错误,则退出程序。
思想:
1.宏定义正确的登录名和密码
2.定义两个数组,来存放所要输入的登录名和密码。
3.登录三次,那就必须用循环。
4.循环语句:
a.首先要实现键盘输入登录名和密码;
b.其次判断登录名、密码和你输入的登录名、密码是否一致。
strcmp ----》int strcmp ( const char * str1, const char * str2 );返回值为0时,登陆成功。
如果一致,登陆成功;
如果不一致,那登录失败,然后登录次数也相应减1.
c.登录失败三次后,让等到一定时间再次尝试输入。并且实时刷新经过了多长时间。
注意:一定要在后面给count赋值,要不进不去循环。
#if 1
#include <stdio.h>
#include <windows.h>
#pragma warning(disable:4996)
#define SIZE 128
#define LOGINCOUNT 3
#define DEF_NAME "admin"
#define DEF_PASSWORD "123456"
void Login()
{
char admin[SIZE];
char password[SIZE];
int count = LOGINCOUNT;
while(count>0)
{
printf("请输入登录名:");
scanf("%s",admin);
printf("请输入密码:");
scanf("%s",password);
if((strcmp(admin,DEF_NAME) == 0)&& (strcmp(password,DEF_PASSWORD) == 0))
{
printf("登录成功\n");
break;
}else{
count--;
printf("登录失败,你还剩余 %d 次\n",count);
}
if(count == 0)
{
int _time = 1;
printf("等待60s重新登录\n");
while(_time<=60)
{
printf("经过了%ds\r",_time);
Sleep(1000);
_time++;
}
count = LOGINCOUNT;
}
}
}
int main()
{
Login();
system("pause");
return 0;
}
#endif
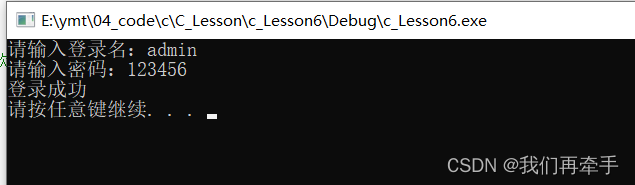
4.8 玩数字游戏
思想:
1.菜单函数:来解释规则。
2.玩游戏函数:
a.系统随机生成一个1-100的数;
srand((unsigned int)time(NULL));
int data = rand() % 100 + 1;
b.因为猜数字的过程是一个循环过程,因此需要使用循环语句。
c.键盘输入你要猜的值。
d.键盘输入的值和系统生成的值进行对比。(猜大了、猜小了、猜中了。)猜中后就break跳出死循环。
3.主函数:
a.调用菜单函数,告诉玩家你的游戏规则;
b.玩家输入1或者2,来确定自己是玩游戏还是退出游戏;
输入1,调用game函数;输入2,则退出游戏;输入其他数字,输入错误,重新输入。
c.为了让玩家多玩几把,可以通过循环来实现。这里使用quit来决定退出,还是继续游戏。
4.注意:VS2012,一般要把初始化写在前面,要不就会报错。
#if 1
#include <stdio.h>
#include <windows.h>
#include <stdlib.h>
#include <time.h>
void Menu()
{
printf("************************************\n");
printf("****1:猜游戏 2:退出游戏****\n");
printf("************************************\n");
}
void Game()
{
int data = 0;
srand((unsigned int)time(NULL));
data = rand() % 100 + 1;
printf("游戏开始\n");
for(;;)
{
int num = 0;
printf("输入1-100之间的数字:\n");
scanf("%d",&num);
if(num > data)
{
printf("猜大了!\n");
}else if(num < data)
{
printf("猜小了!\n");
}else
{
printf("猜中了!数字是%d \n",num);
break;
}
}
printf("游戏结束\n");
}
int main()
{
int quit = 0;
Menu();
while(!quit)
{
int select = 0;
printf("请输入数字(1:猜游戏 2:退出游戏):\n");
scanf("%d",&select);
switch(select)
{
case 1:
Game();
printf("再玩一把?\n");
break;
case 2:
quit = 1;
printf("退出游戏\n");
break;
default:
printf("输入错误,请重新输入\n");
break;
}
}
system("pause");
return 0;
}
#endif
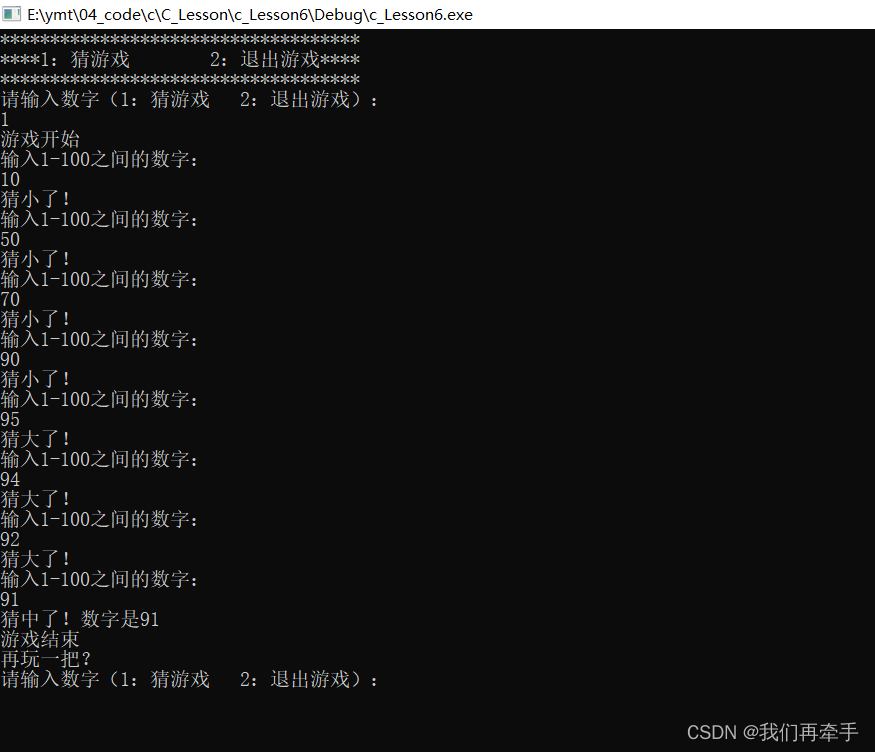
5.goto语句
(1)goto语句:终止程序在某些深度嵌套的结构的处理过程,例如一次跳出两层或多层循环。
这种情况使用break是达不到目的的。break只能从最内层循环退出到上一层的循环。
(2)goto向上跳转:
start:
goto start;
(3)goto向下跳转:
goto end;
end:
(4)goto语句实现电脑关机
5.1 goto向上跳转,下面代码死循环输出hello world。
#if 1
#include <stdio.h>
#include <windows.h>
int main()
{
int flag = 1;
START:
printf("hello world\n");
if(flag == 1)
{
goto START;
}
system("pause");
return 0;
}
#endif
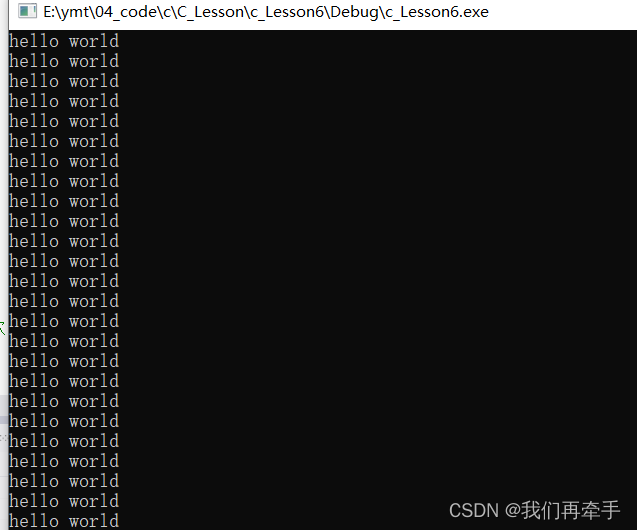
5.2 goto,模拟用户登录情景,并且只能登录三次。
#if 1
#include <stdio.h>
#include <windows.h>
#pragma warning(disable:4996)
#define SIZE 128
#define LOGINCOUNT 3
#define DEF_NAME "admin"
#define DEF_PASSWORD "123456"
void Login()
{
char admin[SIZE];
char password[SIZE];
int count = LOGINCOUNT;;
START:
while(count>0)
{
printf("请输入登录名:");
scanf("%s",admin);
printf("请输入密码:");
scanf("%s",password);
if((strcmp(admin,DEF_NAME) == 0)&& (strcmp(password,DEF_PASSWORD) == 0))
{
printf("登录成功\n");
break;
}else{
count--;
printf("登录失败,你还剩余 %d 次\n",count);
}
if(count == 0)
{
int _time = 1;
printf("等待60s重新登录\n");
while(_time<=60)
{
printf("经过了%ds\r",_time);
Sleep(100);
_time++;
}
count = LOGINCOUNT;
goto START;
}
}
}
int main()
{
Login();
system("pause");
return 0;
}
#endif
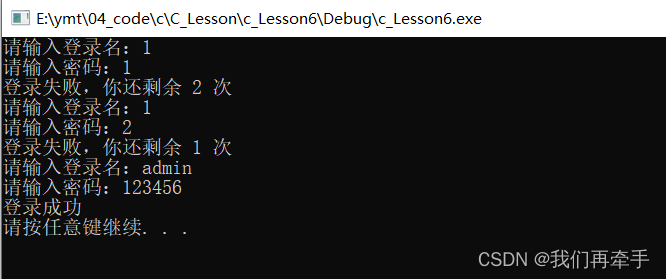
5.3 goto语句向下跳转,下面代码直接跳转至END打印hello world,不输出xxxxxxxxxxxxxxxxxxxxx
#if 1
#include <stdio.h>
#include <windows.h>
int main()
{
int flag = 1;
if(flag == 1)
{
goto END;
}
printf("xxxxxxxxxxxxxxxxxxxxx\n");
END:
printf("hello world\n");
system("pause");
return 0;
}
#endif
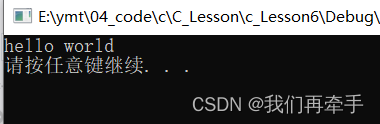
5.4 goto语句的实例,实现电脑关机
1.关机命令:shutdown -s -t 180(三分钟后系统将关机)
取消关机命令:shutdown -a
注:dos命令:system("");来实现;比如systeminfo(电脑硬件信息);calc(计算器);osk(键盘)
2.电脑关机:使用关机指令后,要取消关机指令,输入“取消”二字,则取消关机;如果输入错误,我再给你一次机会,直到时间截止。
3.定义一个数组,存放你输入的字符;
然后用strcmp()函数对输入的字符和“取消”进行比较,相等则执行取消关机指令,如果失败,再给你一次机会。
4.要向实现这个过程,就是用死循环来实现。这里使用goto和while(1)
(1)while循环实现
#if 1
#include <stdio.h>
#include <windows.h>
#define SIZE 128
int main()
{
system("shutdown -s -t 600");
printf("电脑在600s后即将关机,输入“取消”,则取消关机。\n");
while(1)
{
char intput[SIZE];
printf("输入intput:");
scanf("%s",intput);
if((strcmp(intput,"取消")) == 0)
{
system("shutdown -a");
printf("关机程序已经取消\n");
break;
}else{
printf("输入错误,再给你一次机会,请输入“取消”\n");
}
}
system("pause");
return 0;
}
#endif
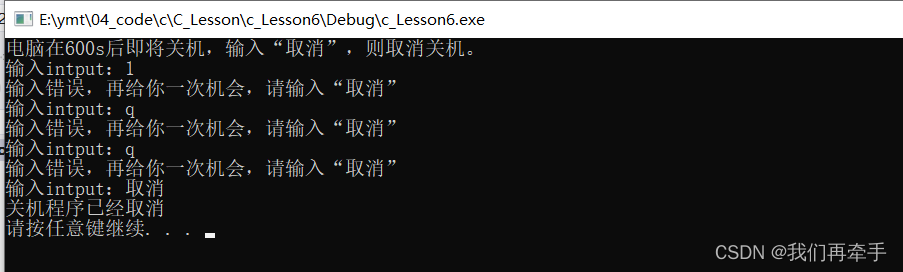 (2)goto语句实现
#if 1
#include <stdio.h>
#include <windows.h>
#define SIZE 128
int main()
{
char intput[SIZE];
system("shutdown -s -t 600");
printf("电脑在600s后即将关机,输入“取消”,则取消关机。\n");
START:
printf("输入intput:");
scanf("%s",intput);
if((strcmp(intput,"取消")) == 0)
{
system("shutdown -a");
printf("关机程序已经取消\n");
}else{
printf("输入错误,再给你一次机会,请输入“取消”\n");
goto START;
}
system("pause");
return 0;
}
#endif
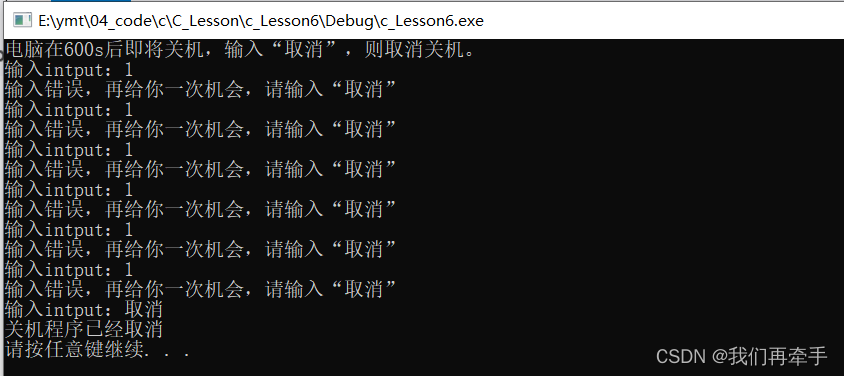
|