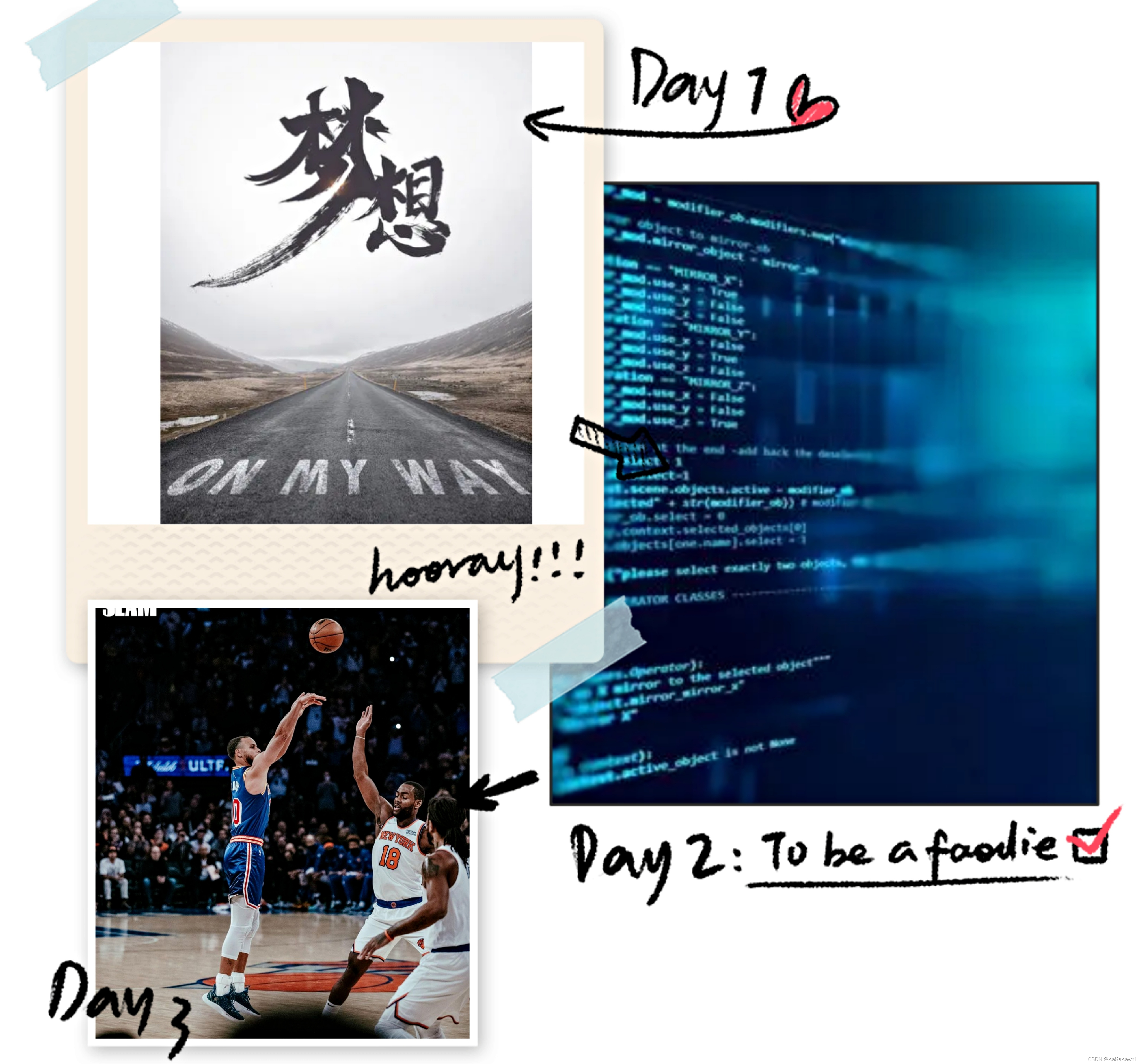
前言
本文总结学习动态栈的c++实现。
一、动态栈头文件Stack.h
本节主要包含:类的创建,部分成员函数声明,部分成员函数定义,成员变量的声明,头文件包含
#pragma once
#include <iostream>
using std::cout;
using std::cin;
using std::endl;
typedef int STDataType;
class Stack
{
public:
Stack(int capacity)
:_a(new STDataType[capacity])
,_capacity(capacity)
,_top(0)
{}
~Stack()
{
delete[] _a;
_a = nullptr;
_capacity = 0;
_top = 0;
}
void Print()const
{
if (0 == this->_top)
{
cout << "空栈";
}
for (int i = 0; i < this->_top; i++)
{
cout << this->_a[i] << " ";
}
cout << endl;
}
void Push(STDataType x);
void Pop();
STDataType Top();
int Size();
bool IsEmpty();
private:
STDataType* _a;
int _capacity;
int _top;
};
二、动态栈各接口具体实现Stack.c
本节主要包含:部分成员函数的定义
2.1 入栈
void Stack::Push(STDataType x)
{
if (this->_capacity == this->_top)
{
int newcapacity = (this->_capacity) * 2;
this->_a = (STDataType*)realloc(this->_a, newcapacity * sizeof(STDataType));
this->_capacity = newcapacity;
}
(this->_a)[this->_top] = x;
(this->_top)++;
}
2.3 出栈
void Stack::Pop()
{
if (this->IsEmpty())
{
cout << "空栈无法出栈" << endl;
}
(this->_top)--;
}
2.4 获取栈顶元素
STDataType Stack::Top()
{
if (this->IsEmpty())
{
cout << "空栈无法获取栈顶元素" << endl;
}
return (this->_a)[(this->_top) - 1];
}
2.5 获取栈中有效元素个数
int Stack::Size()
{
return this->_top;
}
2.6 检测栈是否为空
bool Stack::IsEmpty()
{
return 0 == this->_top;
}
总结
这里对文章进行总结: 以上就是今天总结的内容,本文包括了动态栈的c++实现,分享给大家。 真💙欢迎各位给予我更好的建议,欢迎访问!!!小编创作不易,觉得有用可以一键三连哦,感谢大家。peace 希望大家一起坚持学习,共同进步。梦想一旦被付诸行动,就会变得神圣。
欢迎各位大佬批评建议,分享更好的方法!!!🙊🙊🙊
|