如题 , 什么是函数顺序执行队列 ?
日常开发可能有这样的需求
软件打开 初始化
1.先初始化声音模块 2.初始化视频模块 3.初始化某某模块 等 顺序执行 ,而且初始化过程还有阻塞等,一个模块错误就退出报错 如果我们全部顺序写 会有很多重复的代码 比如 if(!initA()) { dosometh(); exit; } if(!initB()) { dosometh(); exit; } if(!initC()) { dosometh(); exit; }
可能c 还需要在线程中执行 等等 写起来不方便 不美观那么我们实现一个这样的队列 , 把函数都封装好 丢进去, 让他执行就好了很多功能都没有实现, 基本的函数顺序执行队列完成了由于函数的参数传递不等 我这里用的是个 void* , 你的参数如果是多个 就放到结构体里面 转为void*
下面是代码 比较精简:
#include <QCoreApplication>
#include <iostream>
#include <queue>
#include <functional>
class FunExeQueue
{
public:
typedef std::function<void(void *param)> function_;
struct FunStruct
{
function_ func = nullptr;
void* param = nullptr;
FunStruct();
FunStruct(const function_ & f, void* p)
:func(f), param(p){}
};
FunExeQueue(){}
~FunExeQueue(){}
void push(const FunStruct& funcStruct)
{
m_funStructQueue.push(funcStruct);
}
void run()
{
while(false == m_funStructQueue.empty())
{
auto funcStruct = m_funStructQueue.front();
m_funStructQueue.pop();
if(funcStruct.func)
{
funcStruct.func(funcStruct.param);
}
}
}
private:
std::queue<FunStruct> m_funStructQueue;
};
void fun1(void* p)
{
std::cout << "i am fun1()" << std::endl;
}
void fun2(void* p)
{
std::cout << "i am fun2()" << std::endl;
}
struct CustomParam
{
int a = 10;
int b = 29;
};
void c(void* p)
{
CustomParam* param = static_cast<CustomParam*>(p);
if(param)
{
std::cout << "i am c()" << std::endl;
std::cout << param->a << std::endl;
std::cout << param->b << std::endl;
}
}
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
FunExeQueue funQueue;
funQueue.push(FunExeQueue::FunStruct(&fun1, nullptr));
funQueue.push(FunExeQueue::FunStruct(&fun2, nullptr));
QScopedPointer<CustomParam> param;
param.reset(new CustomParam());
param->a = 200;
param->b = 400;
funQueue.push(FunExeQueue::FunStruct(&c, static_cast<CustomParam*>(param.data())));
funQueue.run();
return a.exec();
}
?运行结果如下:
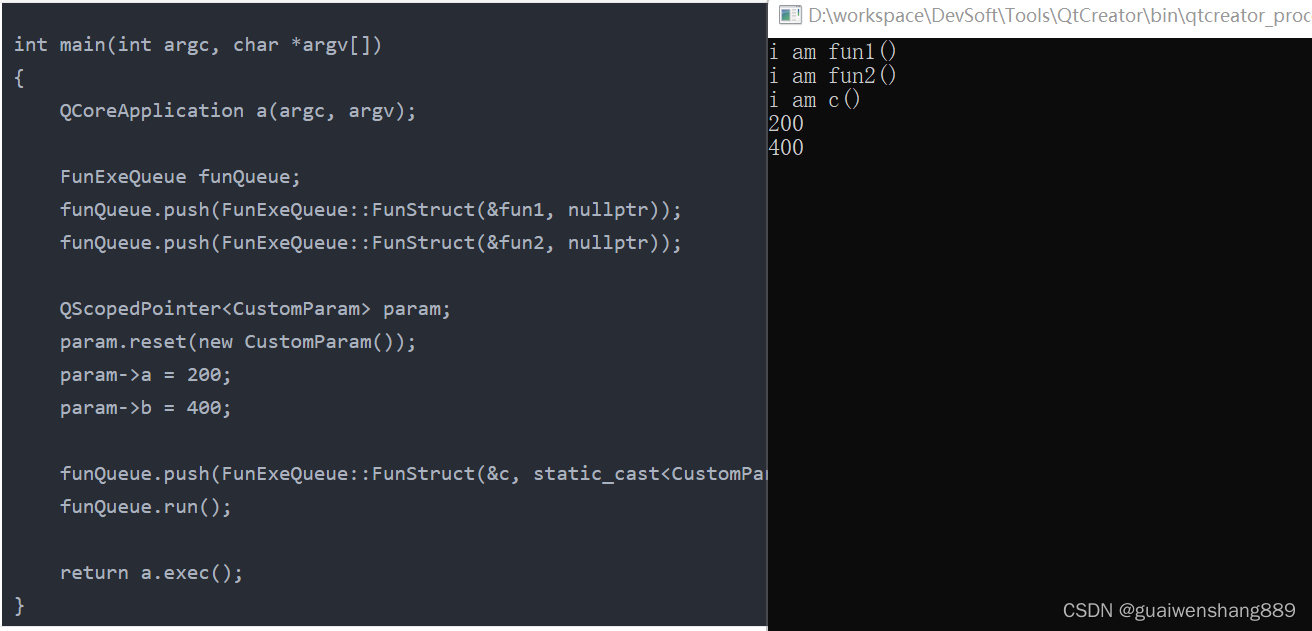
代码思路:
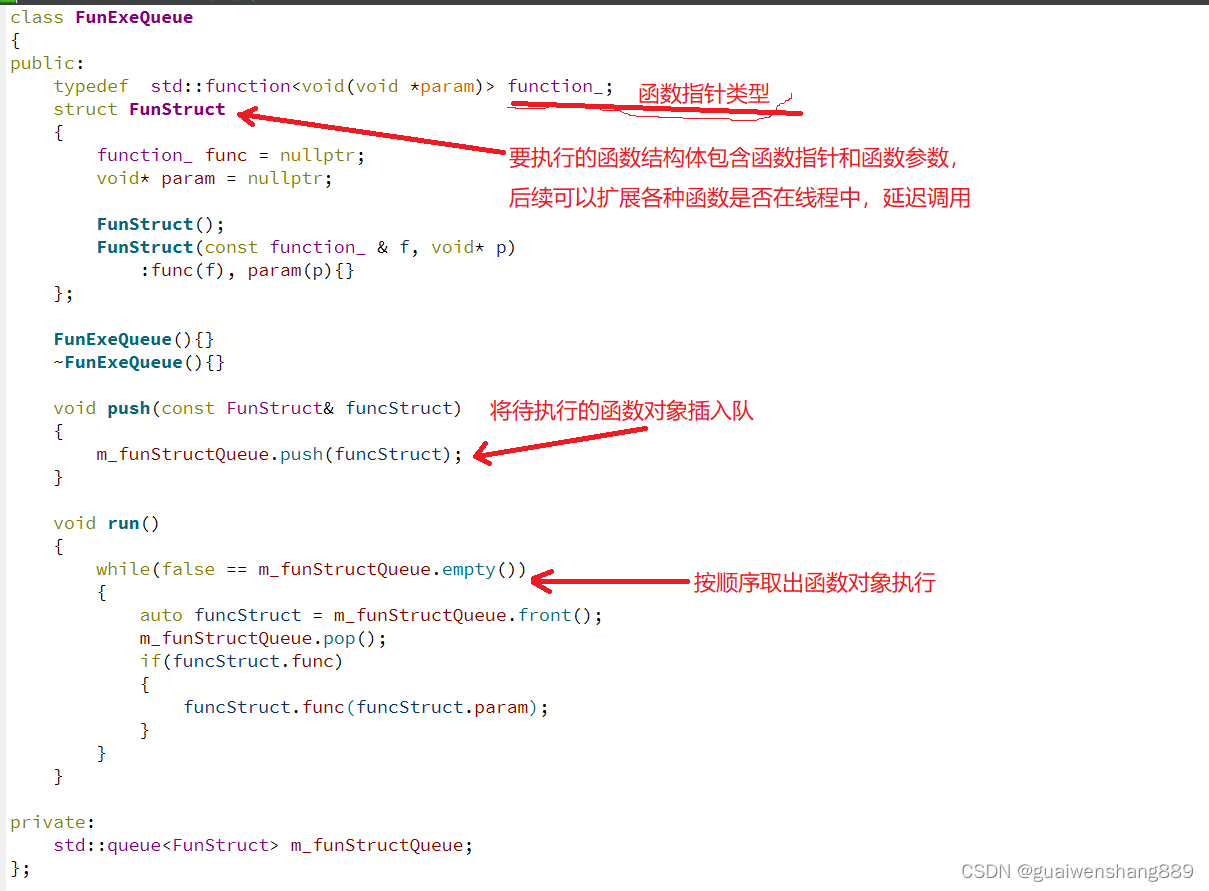
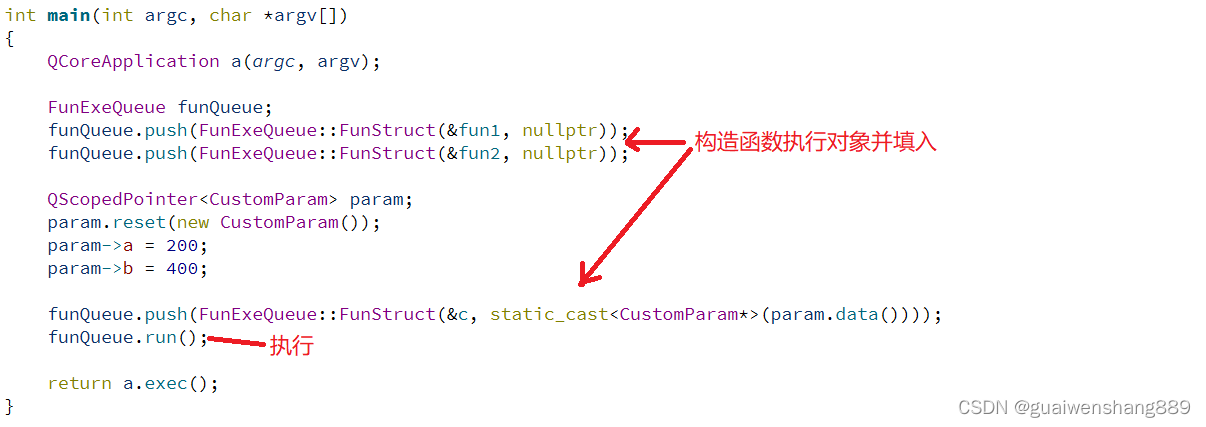
以上就是函数执行队列的实现过程
|