题目描述: 项目团队需要实现一个路由转发模块,转发模块的核心功能之一是根据功能号/接口名来 进行路由转发。路由匹配的基本原理是判断实际请求中的功能号/接口名是否与预先配置的转发规则匹配,转发规则支持’?‘和*‘通配符(’?‘表示匹配任意单个字符;’'表示匹配零个或多个任意字符)。比如转发规则串配置为“600???",当实际请求中的功能号是"600570”时就判定为匹配。现在请你编码实现一个函数来支持是否匹配的判断。 注意:要自己编码实现逻辑,不可以调用已有的实现函数。另外在函数编码之前可以用注释说明自己的逻辑思路。 函数原型及参数说明如下 l/入参string表示实际请求中的功能号/接口名; string可能为空l/入参 wild 表示预先定义的模式匹配串; wild可能为空 bool isMatch(char string, char* wild); 提示 1.实际请求中的功能号/接口名只包含从0-9的数字或者a-z的英文字母(大小写敏感)⒉预定义的模式匹配串只包含从0-9的数字,a-z的字母(大小写敏感)以及字符’?'和 1六1 示例输入: string = "aa"wild = "a"输出:false 解释: "a”无法匹配"aa"整个字符串。
#include<iostream>
using namespace std;
bool isMatch(char* string, char* wild);
int main()
{
char str[200] = { 'a','a'};
char w[200] = { 'a'};
bool result = false;
cout << "请输入请求功能号:" << endl;
cin >> str;
cout << "请输入模式匹配串:" << endl;
cin >> w;
result = isMatch(str,w);
cout << "输出匹配结果:" << endl;
cout << boolalpha;
cout << result<<endl;
return 0;
}
bool isMatch(char* string, char* wild)
{
int len_str = strlen(string);
int len_w = strlen(wild);
for (int i = 0; i <= len_w; i++)
{
if (wild[i] == '*')
{
if (wild[i+1]=='\0')
{
return true;
}
else
{
int j = 0, k = 1, count = 1;
for (j = i; j <= len_str; j++)
{
if (wild[i + 1] == string[j])
{
break;
}
else if (wild[i + 1] == '?')
{
while (wild[i + 1 + k] == '?')
{
count++;
k++;
}
for (; j <= len_str; j++)
{
if (string[j] == wild[i + 1 + k])
{
break;
}
}
j = j - count;
break;
}
}
if (j < len_str)
{
return isMatch(string + j, wild + i + 1);
}
else
{
return false;
}
}
}
else if (wild[i] == '?')
{
continue;
}
else if (wild[i] != string[i])
{
return false;
}
else
{
continue;
}
}
return true;
}
测试结果: 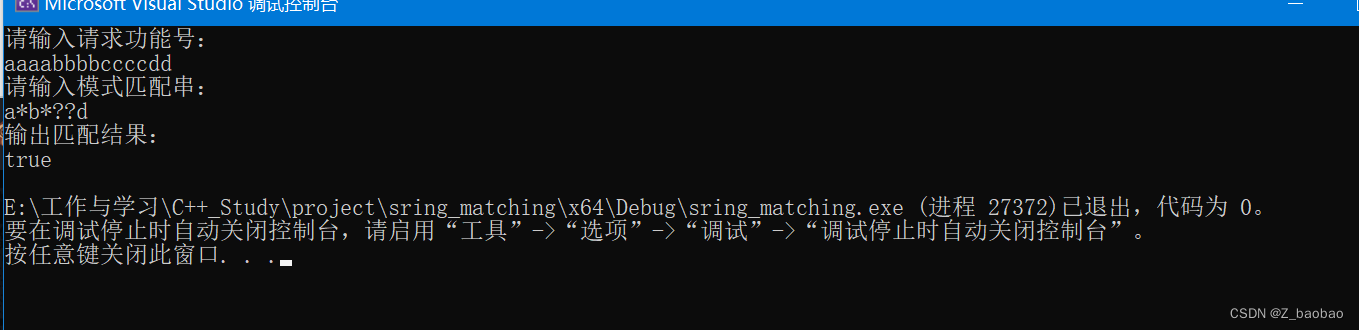
|