1. 初识文件
1.1 文件流头文件
使用时,需要 include <fsteam>
1.2 文件类型
- 文本文件:以文本的ASCII码形式存储到计算机中;
- 二进制文件:二进制码格式存储。
1.3 文件操作
- 写入文件:ofsteam
- 读取文件:ifsteam
- 读写操作:fsteam
2. 写文件
- 包含头文件:
include <fsteam>
- 创建文件流对象:
ofsteam 对象名;
- 打开文件:
对象名.open(“文件路径”, ios :: out);
- 写数据:
对象名 << “写入的数据”;
- 关闭文件:
对象名.close();
3. 读文件、
- 头文件~
- ifsteam~
- 对象名.open(“文件路径”, ios :: in);
- 判断是否打开成功:
if(!对象名.is_open())
{
cout << "文件打开失败!" << endl;
return ;
}
- 四种读取文件方式
5.1 文件对象名 >> 字符数组变量名;
- 从文件中读取一行数据
- 读取到换行符就停止读入,并舍弃末尾换行符
- 读取到空(即文件末尾)时,返回假(?可能描述不准确)
5.2 文件对象名.getline(字符数组变量名, 读取字节数):
- 同上;
5.3 getline(文件对象名, string变量名); - 同上;
5.4 文件变量名.get(); - 每次读取一个字符
- 读到换行符就舍弃
- 读取到文件结束返回EOF
- 关闭文件
4. 测试代码
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
char filename[] = "test.txt";
bool fout()
{
char phone[] = "0123456789";
ofstream ofs;
ofs.open(filename, ios::out);
if (!ofs.is_open()) {
return false;
}
ofs << "姓名:张三" << endl;
ofs << "性别:男" << endl;
ofs << "\t" << endl;
ofs << "电话:" << phone << endl;
ofs.close();
return true;
}
bool fin1()
{
ifstream ifs;
char buf[1024];
ifs.open(filename, ios::in);
if (!ifs.is_open()) {
return false;
}
int count = 0;
while (count++, ifs >> buf)
{
cout << buf << endl;
}
cout << count << endl;
ifs.close();
return true;
}
bool fin2()
{
ifstream ifs;
char buf[1024];
ifs.open(filename, ios::in);
if (!ifs.is_open()) {
return false;
}
int count = 0;
while (count++, ifs.getline(buf, 1024))
{
cout << buf << endl;
}
cout << count << endl;
ifs.close();
return true;
}
bool fin3()
{
ifstream ifs;
string buf;
ifs.open(filename, ios::in);
if (!ifs.is_open()) {
return false;
}
int count = 0;
while (count++, getline(ifs, buf))
{
cout << buf << endl;
}
cout << count << endl;
ifs.close();
return true;
}
bool fin4()
{
ifstream ifs;
char buf;
ifs.open(filename, ios::in);
if (!ifs.is_open()) {
return false;
}
int count = 0;
while (count++, (buf = ifs.get()) != EOF)
{
cout << buf;
}
cout << count << endl;
ifs.close();
return true;
}
int main()
{
if (!fout()) {
cout << "文件建立失败!" << endl;
}
cout << "文件建立成功!" << endl;
if (!fin4()) {
cout << "文件读取失败!" << endl;
}
cout << "文件读取成功!" << endl;
system("pause");
return 0;
}
5. 文件打开方式
打开方式 | 解释 | c语言 |
---|
in | 只读方式打开 | “r” | out | 只写方式打开 | “w” | ate | 文件指针移动到文件末尾打开 | — | app | 以追加写方式打开 | “a” | trunc | 覆盖原文件并打开新文件 | — | binary | 以二进制方式打开 | “b–” |
文件打开方式可以配合使用,利用"|"操作符,如ios :: app | ios :: ate
6. 二进制形式读写文件
6.1 写入数据
语法: 文件对象名.write((const char *)变量地址, 写入字符串的长度)
如:
#include <fstream>
void WriteBinary()
{
char str[] = "I'm a string!";
ofstream ofs(filename, ios::out|ios::binary);
ofs.write((const char*)&str, sizeof(str));
ofs.close();
}
6.2 读入文件
语法: 文件对象名.read(变量指针, 读入字符串的长度)
如:
#include <fstream>
void ReadBinary()
{
char str[24];
ifstream ifs(filename, ios::in|ios::binary);
ifs.read(str, sizeof(str));
ifs.close();
}
7. 测试代码
#include <iostream>
#include <fstream>
using namespace std;
char filename[] = "Binary.bat";
class Person
{
public:
char name[16];
int age;
char sex[8];
};
void WriteBinary()
{
Person temp[2] = {
{"张三", 18, "男"} ,
{"李四", 19, "女"} };
ofstream ofs(filename, ios::out | ios::binary);
ofs.write((const char*)&temp, sizeof(temp));
ofs.close();
}
void ReadBinary()
{
Person temp[2];
ifstream ifs(filename, ios::in | ios::binary);
ifs.read((char*)&temp, sizeof(temp));
cout << temp[0].name << " " << temp[0].age << " " << temp[0].sex << endl;
cout << temp[1].name << " " << temp[1].age << " " << temp[1].sex << endl;
ifs.close();
}
void test()
{
WriteBinary();
cout << "写入成功!" << endl;
ReadBinary();
cout << "读取成功!" << endl;
}
int main()
{
test();
system("pause");
return 0;
}
运行截图: 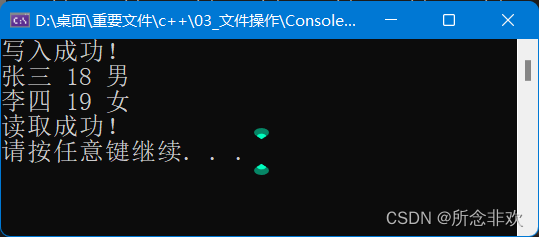
8. 放松时刻
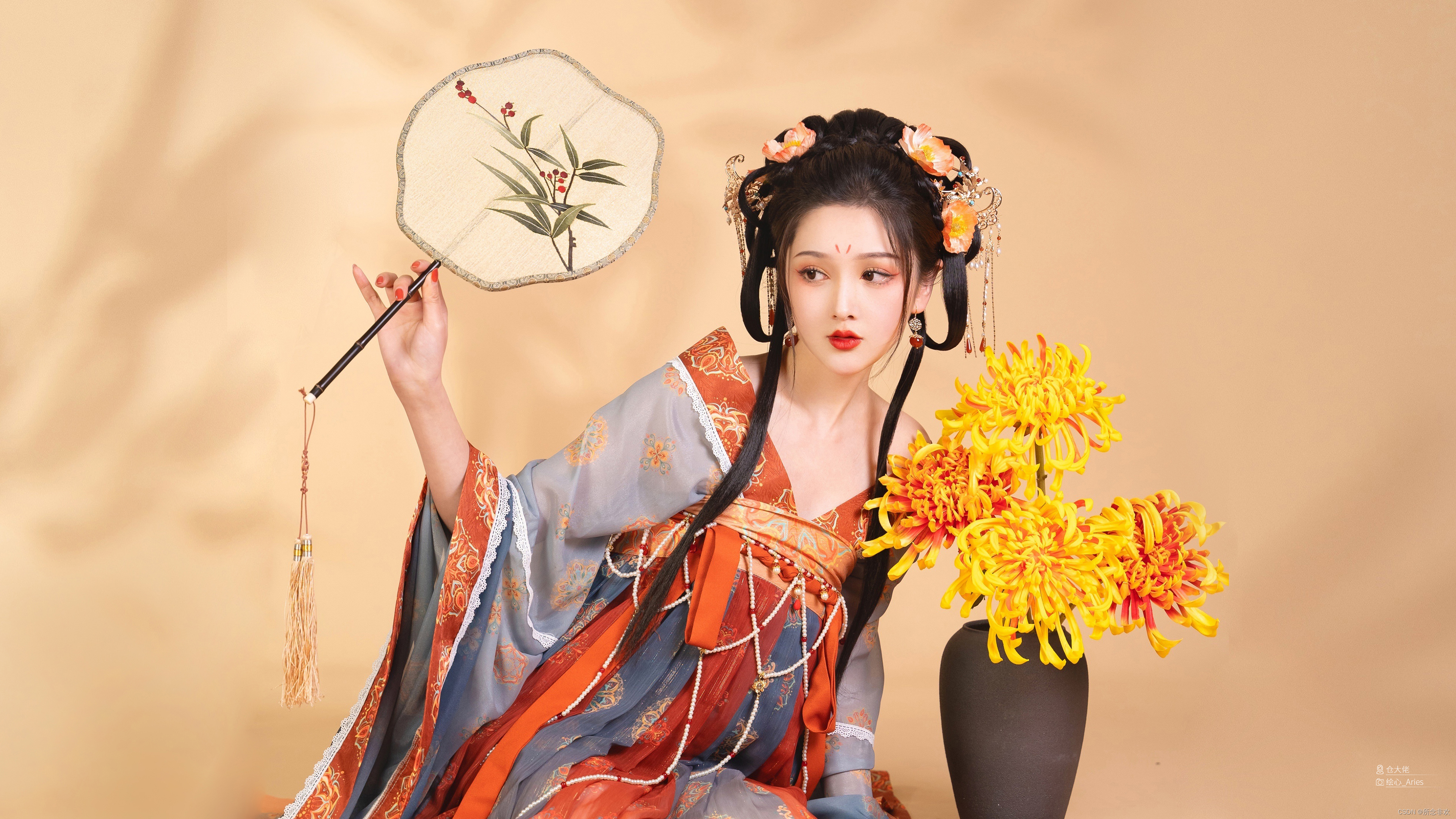 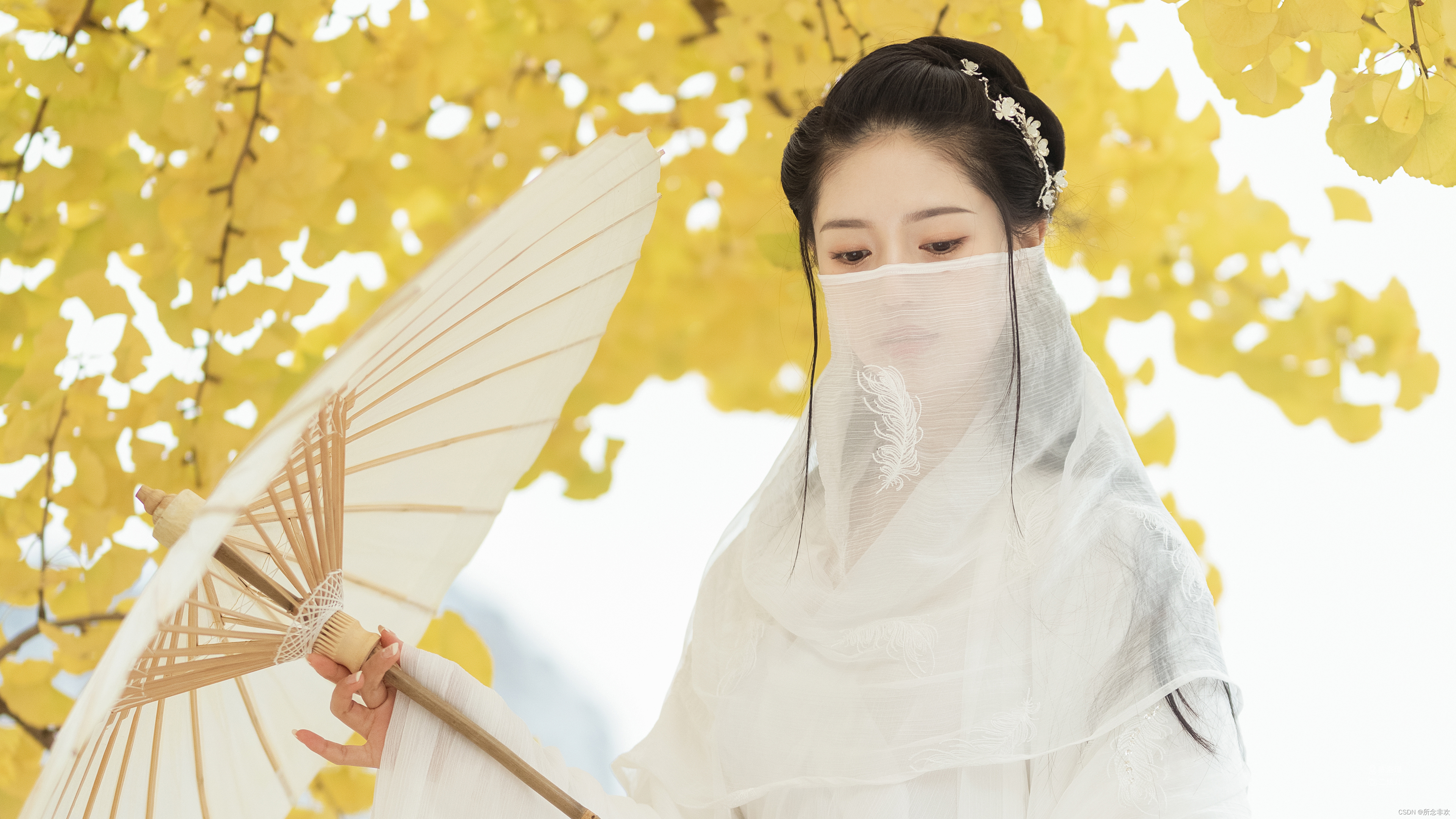
|