一.strlwr与strupr;大小写转换函数 strlwr与strupr函数的格式: char *strlwr(char *s1); char *strupr(char *s1); 对strlwr函数进行封装:
char *strlwr_s(char *src)
{
int i;
while(src[i]!='\0')
{
if(src[i]>='A'&&src[i]<='Z')
{
src[i] += 32;
}
i++;
}
return src;
}
对strupr函数进行封装:
char *strupr_s(char *src)
{
int i;
while(src[i]!='\0')
{
if(src[i]>='a'&&src[i]<='z')
{
src[i]-=32;
}
i++;
}
return src;
}
代码展示:
#include<stdio.h>
#include<string.h>
char *strlwr_s(char *src);
char *strupr_s(char *src);
int main()
{
char str[20] = "saacdsCWDFDdsc";
char *p = strlwr(str);
printf("%s\n",p);
char *p1 = strlwr_s(str);
printf("%s",p1);
char *p2 = strupr(str);
printf("%s\n",p2);
char *p3 = strupr_s(str);
printf("%s\n",p3);
return 0;
}
运行结果:
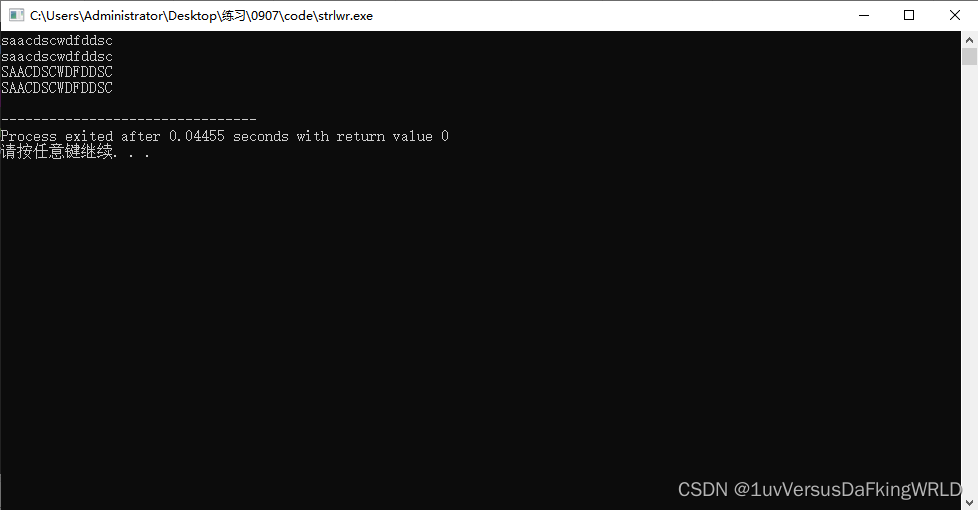
?
二.atoi与atol&atof;字符串转换函数(tips:这三者仅适用纯数字字符串)
atoi与atol&atof函数的格式: int _cdecl atoi(const char *str);
long _cdecl atol(const char *str);
double_cdecl atof(const char *str);
代码展示:
#include<stdio.h>
#include<string.h>
#include<stdlib.h>
int main()
{
char str[64] = "dvdsvds";
char *pch = NULL;
int ret = atoi(str);
printf("%d\n",ret);
long ret1 = atol(str);
printf("%ld\n",ret1);
double ret2 = atof(str);
printf("%f\n",ret2);
运行结果:
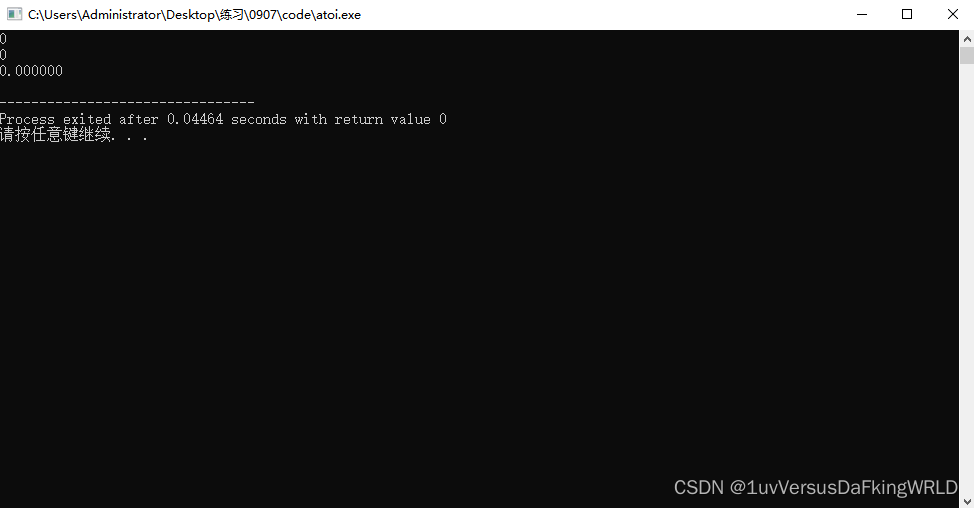
?
三. strtod(转换为实型函数),strtol(转换为long型函数),strtoul(转换为无符号long类型函数)?
strtod的定义格式:double strtod(const char *str, const char **endptr);
strtol的定义格式:long?strtol(const char *str, const char **endptr,int radix);
strtoul的定义格式:unsigned long?strtoul(const char *str, const char **endptr,int radix);
代码展示:
#include<stdio.h>
#include<string.h>
#include<stdlib.h>
int main()
{
char str[10] = "802345671";
char *pch =NULL;
printf("%p\n",str);
double ret3 = strtod(str,&pch);
printf("%p\n",pch);
printf("%f\n",ret3);
printf("%p\n",str);
long ret4 = strtol(str,&pch,10);
printf("%p\n",pch);
printf("%ld\n",ret4);
unsigned long ret5 = strtoul(str,&pch,10);
printf("%p\n",pch);
printf("%lu\n",ret5);
return 0;
}
运行结果:
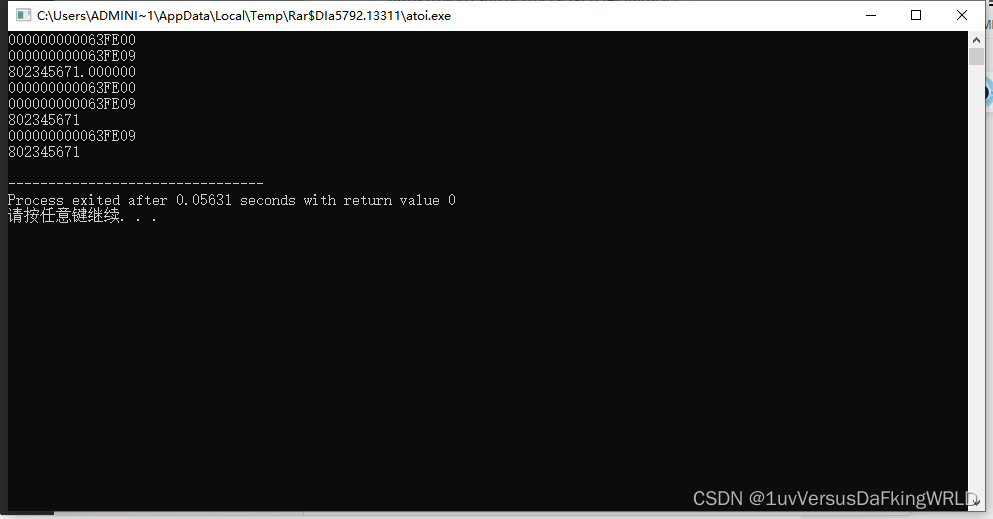
四:malloc:
用法以及代码展示:
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
int main()
{
char *pa = NULL;
char *pb = NULL;
pa = (char *)malloc(sizeof(char)*20);
pb = (char *)malloc(sizeof(char)*30);
memset(pa,0,sizeof(char)*20);
memset(pb,0,sizeof(char)*30);
// int i;
// for(i=0;i<10;i++)
// {
// pa[i] = 'a'+i;
// }
//
// for(i=0;i<20;i++)
// {
// pb[i] = 'A'+i;
// }
// pa = "hello world";
// pb = "BSP2208class";
strcpy(pa,"hello world");
strcpy(pb,"BSP2208class");
printf("%s\n",pa);
printf("%s\n",pb);
// strcpy(pb,pa);
memcpy(pb,pa,sizeof(char)*10);
printf("%s\n",pb);
// for(i=0;i<30;i++)
// {
// printf("%d\n",pb[i]);
// }
free(pa);
free(pb);
pa = NULL;
pb = NULL;
return 0;
}
输出结果:
?
五:memcpy:
格式: void *memcp(void *dest,const void *source,size_t n);
可以除了字符型拷贝其他数据类型 ,strcpy只能copy字符型
代码展示:
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
int main()
{
// char src[20] = "hello world";
// char dest[30] = "BSP2208calss";
int arr[10] = {1,2,3,4,5,6,7,8,9,10};
int brr[20] = {11,21,31,41,51,61,71,81,91,110,111,112,113};
// strcpy(dest,src);
// printf("%s\n",dest);
// memcpy(dest,src,10);
// printf("%s\n",dest);
memcpy(brr,arr,sizeof(int)*10);
int i=0;
for(i=0;i<20;i++)
{
printf("%d ",brr[i]);
}
return 0;
}
运行结果:
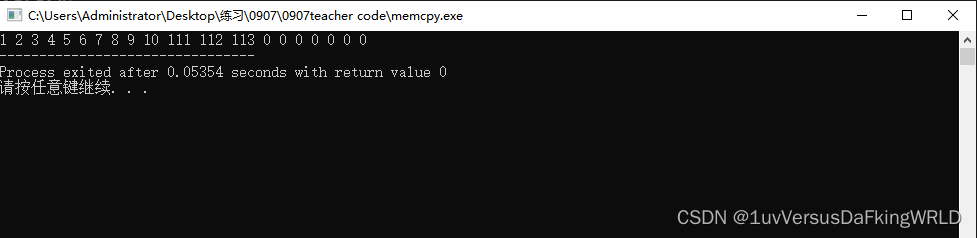
?
六:memmove:
?格式:void *memmove(void *dest,const void *src, size_t n);
代码展示:
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
int main()
{
char *pa = NULL;
char *pb = NULL;
pa = malloc(sizeof(char)*20);
pb = realloc(pa,sizeof(char)*50);
printf("%p\n",pa);
printf("%p\n",pb);
strcpy(pa,"hello world");
strcpy(pb,"BSP2208class");
memmove(pb,pa,sizeof(char)*10);
printf("%s\n",pb);
free(pb);
pb = NULL;
return 0;
}
运行结果:
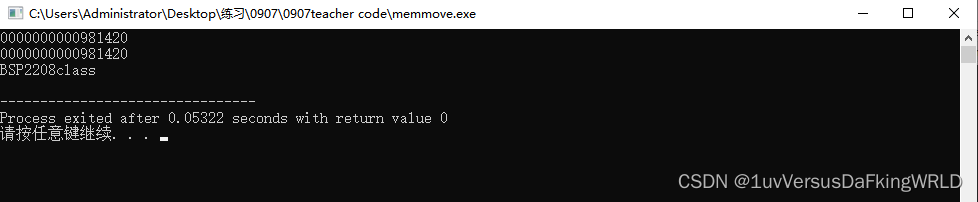
七:memcmp;
?
格式:int memcmp(const void *ptrl,const void* ptr2,size_t num);
代码展示:
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
int main()
{
int *pa = NULL;
int *pb = NULL;
pa = malloc(sizeof(int)*10);
pb = malloc(sizeof(int)*20);
memset(pa,0,10);
memset(pb,0,10);
// strcpy(pa,"BSP220llo world");
// strcpy(pb,"BSP22e8");
int i;
for(i=0;i<10;i++)
{
pa[i] = i+10;
}
for(i=0;i<20;i++)
{
pb[i] = i+20;
}
int ret = memcmp(pa,pb,6);
printf("%d\n",ret);
free(pa);
free(pb);
pa = NULL;
pb = NULL;
return 0;
}
运行结果:

?
?
|