C++/QT图形界面编程(GUI)
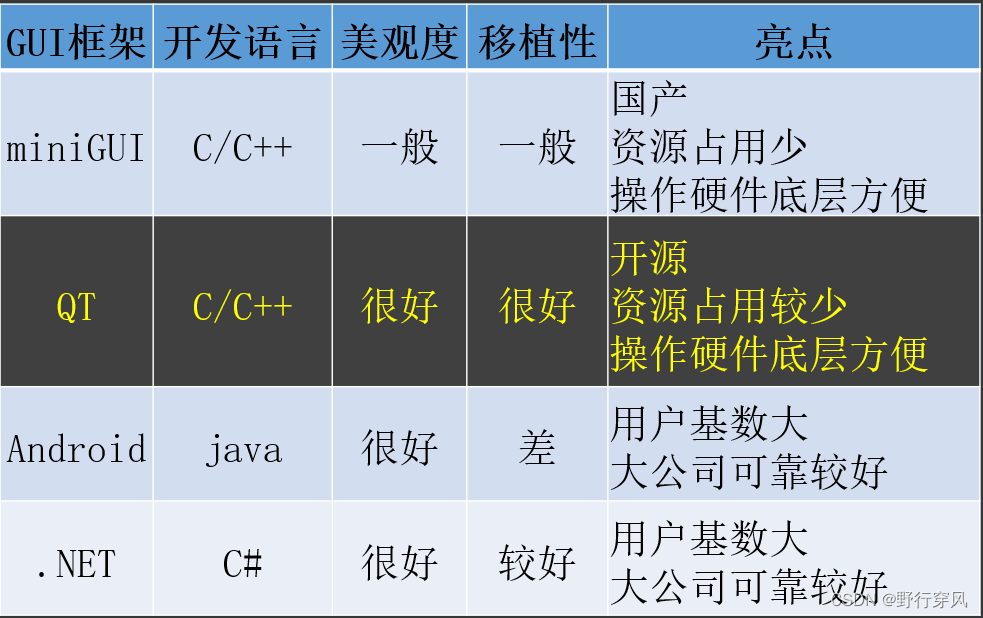
一、从C到C++
1.语法升级
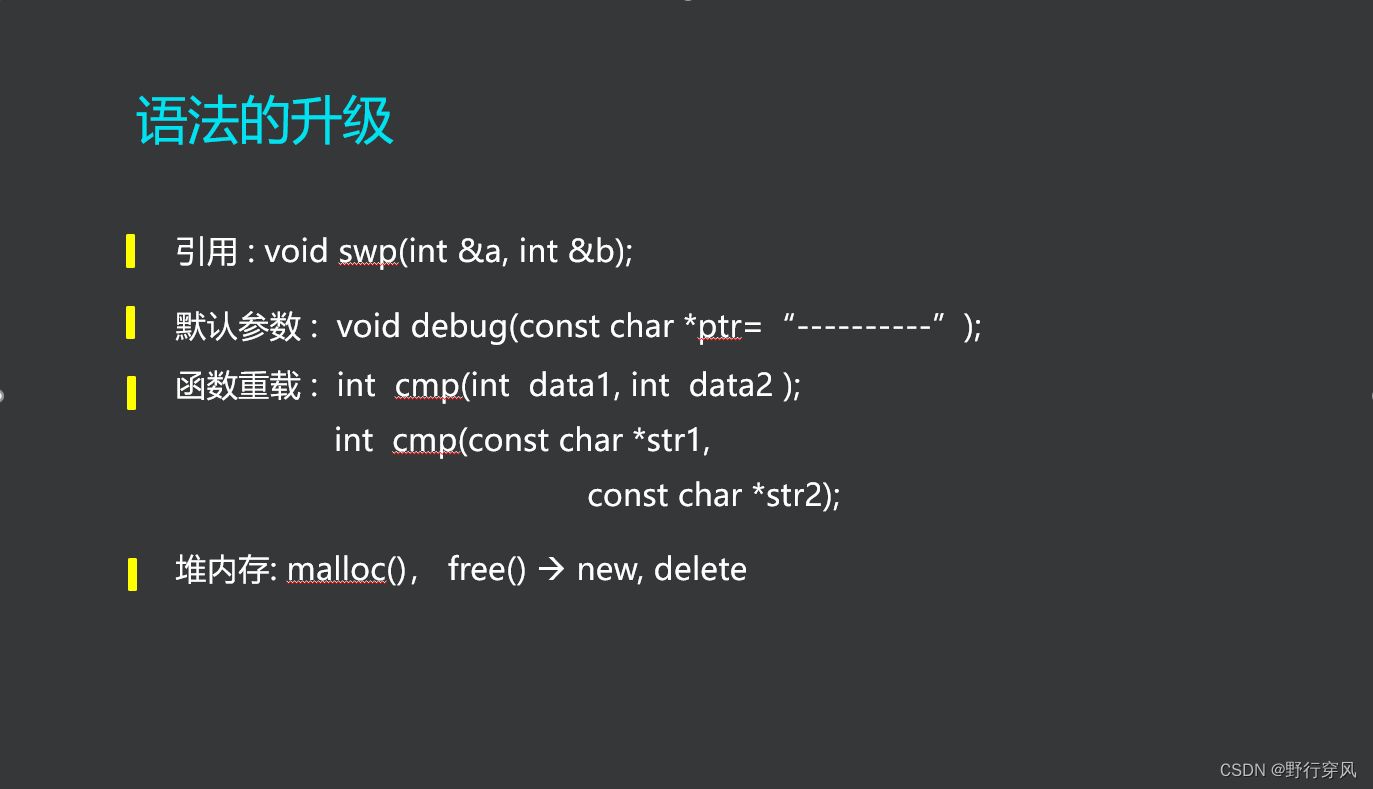
(1)引用
void swp(int &a, int &b);
#include <stdio.h>
int main()
{
int a = 100;
int &b = a;
printf("a = %d\n", a);
printf("b = %d\n", b);
printf("addr: a = %p\n", &a);
printf("addr: b = %p\n", &b);
return 0;
}
#include <stdio.h>
int swap(int &a, int &b)
{
a ^= b;
b ^= a;
a ^= b;
}
int main()
{
int a = 100;
int b = 10;
printf("a = %d, b = %d\n", a, b);
swap(a, b);
printf("a = %d, b = %d\n", a, b);
return 0;
}
(2)默认参数
void debug(const char *ptr=“----------”);
#include <stdio.h>
void debug(const char *ptr = "---------------")
{
printf("%s\n", ptr);
}
int main()
{
debug();
debug();
debug("hello");
debug("world");
return 0;
}
(3)函数重载
int cmp(int data1, int data2 ); int cmp(const char *str1, const char *str2);
#include <stdio.h>
#include <string.h>
int cmp(int a, int b)
{
return a-b;
}
int cmp(const char *str1, const char *str2)
{
return strcmp(str1, str2);
}
int main()
{
printf("%d, %d\n", cmp(1, 1), cmp(1,2));
printf("%d, %d\n", cmp("hhh", "hhh"), cmp("hhh", "aaa"));
return 0;
}
(4)堆内存
malloc(),free() -> new, delete
#include <stdio.h>
#include <malloc.h>
#include <string.h>
int main()
{
int *intp = new int;
*intp = 100;
printf("*intp = %d\n", *intp);
delete intp;
char *p = new char[10];
strcpy(p, "hello");
printf("p = %s\n", p);
delete [] p;
return 0;
}
2.概念升级
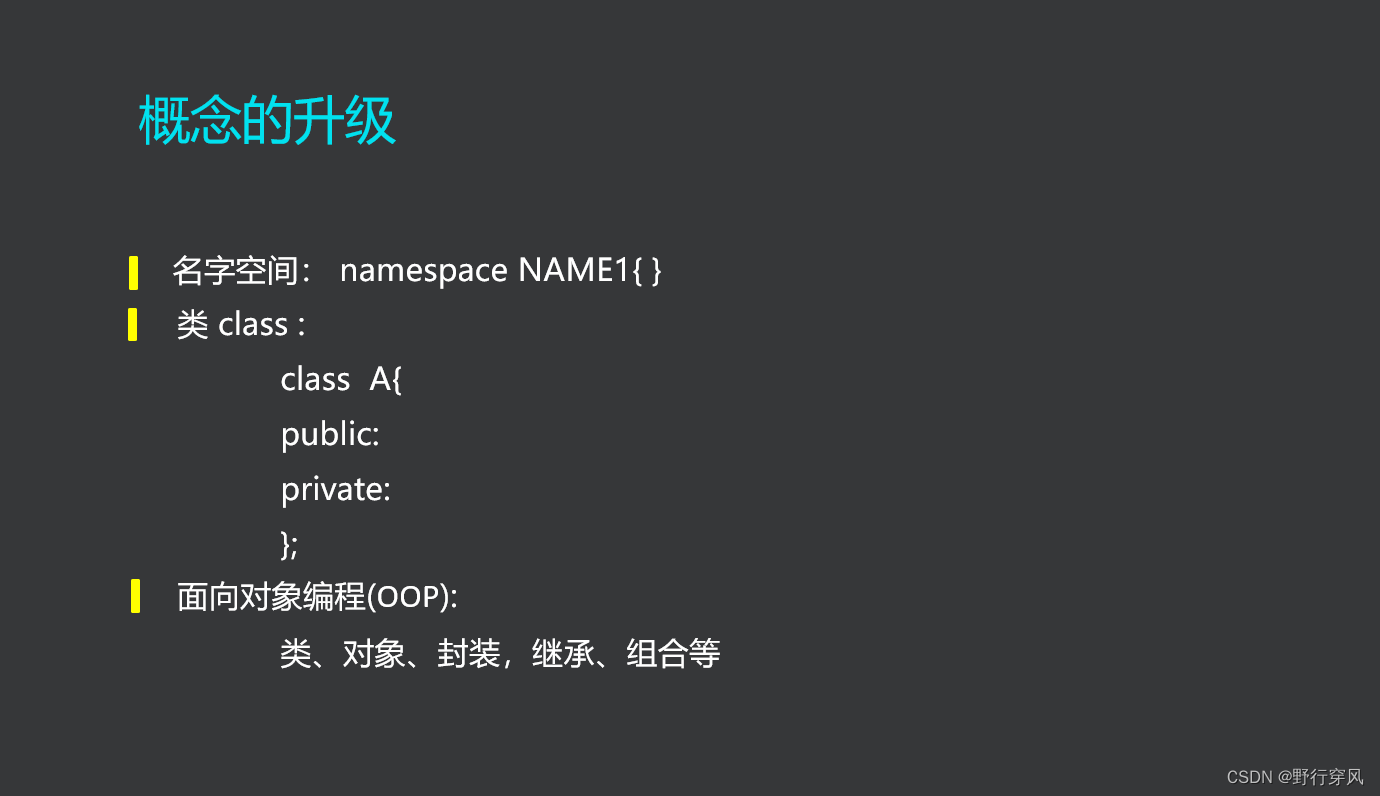
(1)名字空间
namespace NAME1{ };
#include <stdio.h>
namespace A{
int add(int a, int b)
{
return a+b;
}
};
namespace B{
int add(int a, int b)
{
return 2*a+b;
}
};
using namespace A;
int main()
{
printf("%d\n", A::add(1, 2) );
printf("%d\n", B::add(1, 2) );
printf("%d\n", add(1, 2) );
return 0;
}
(2)类:class
class 类名 { private: 私有的数据和成员函数; public: 公用的数据和成员函数; protected: 保护的数据和成员函数 };
(3)面向对象编程(oop)
类、对象、封装,继承、组合等
C实现尾部插入(结构体)
test.h
#ifndef _TEST_H_
#define _TEST_H_
typedef struct arr_t{
int data[10];
int last;
}ARR;
void init(ARR *arr);
void addtail(ARR *arr, int data);
void show(ARR *arr);
#endif
test.c
#include "test.h"
#include <stdio.h>
void init(ARR *arr)
{
arr->last = -1;
}
void addtail(ARR *arr, int data)
{
arr->last++;
arr->data[arr->last] = data;
}
void show(ARR *arr)
{
int i;
for (i = 0; i <= arr->last; i++)
printf("%d, ", arr->data[i]);
puts("");
}
main.c
#include "test.h"
int main()
{
ARR arr;
init(&arr);
int i = 5;
for (; i > 0; i--) {
addtail(&arr, i);
}
show(&arr);
return 0;
}
C实现尾部插入(面向对象思想、结构体、函数指针)
test.h
#ifndef _TEST_H_
#define _TEST_H_
typedef struct arr_t{
int data[10];
int last;
void (*addtail)(struct arr_t *arr, int data);
void (*show)(struct arr_t *arr);
}ARR;
void init(ARR *arr);
#endif
test.c
#include "test.h"
#include <stdio.h>
static void addtail(struct arr_t *arr, int data)
{
arr->last++;
arr->data[arr->last] = data;
}
static void show(struct arr_t *arr)
{
int i;
for (i = 0; i <= arr->last; i++)
printf("%d, ", arr->data[i]);
puts("");
}
void init(ARR *arr)
{
arr->last = -1;
arr->addtail = addtail;
arr->show = show;
}
main.c
#include "test.h"
int main()
{
ARR arr;
init(&arr);
int i = 5;
for (; i > 0; i--) {
arr.addtail(&arr, i);
}
arr.show(&arr);
return 0;
}
C++实现尾部插入和倒序(面向对象编程、类、友元函数)
test.h
#ifndef _TEST_H_
#define _TEST_H_
class ARR{
public:
ARR():last(-1){
}
void addtail(int data);
void show();
friend void rev(ARR &arr);
private:
int data[10];
int last;
};
#endif
test.cpp
#include "test.h"
#include <stdio.h>
void ARR::addtail(int data)
{
this->data[++last] = data;
}
void ARR::show()
{
int i = 0;
for (; i <= last; i++)
printf("%d, ", data[i]);
puts("");
}
main.cpp
#include "test.h"
void rev(ARR &arr)
{
int i = 0;
for (; i < arr.last/2; i++) {
int temp = arr.data[i];
arr.data[i] = arr.data[arr.last-i];
arr.data[arr.last-i] = temp;
}
}
int main()
{
ARR arr;
int i = 0;
for (; i < 5; i++)
arr.addtail(i);
arr.show();
rev(arr);
arr.show();
return 0;
}
3.思维升级
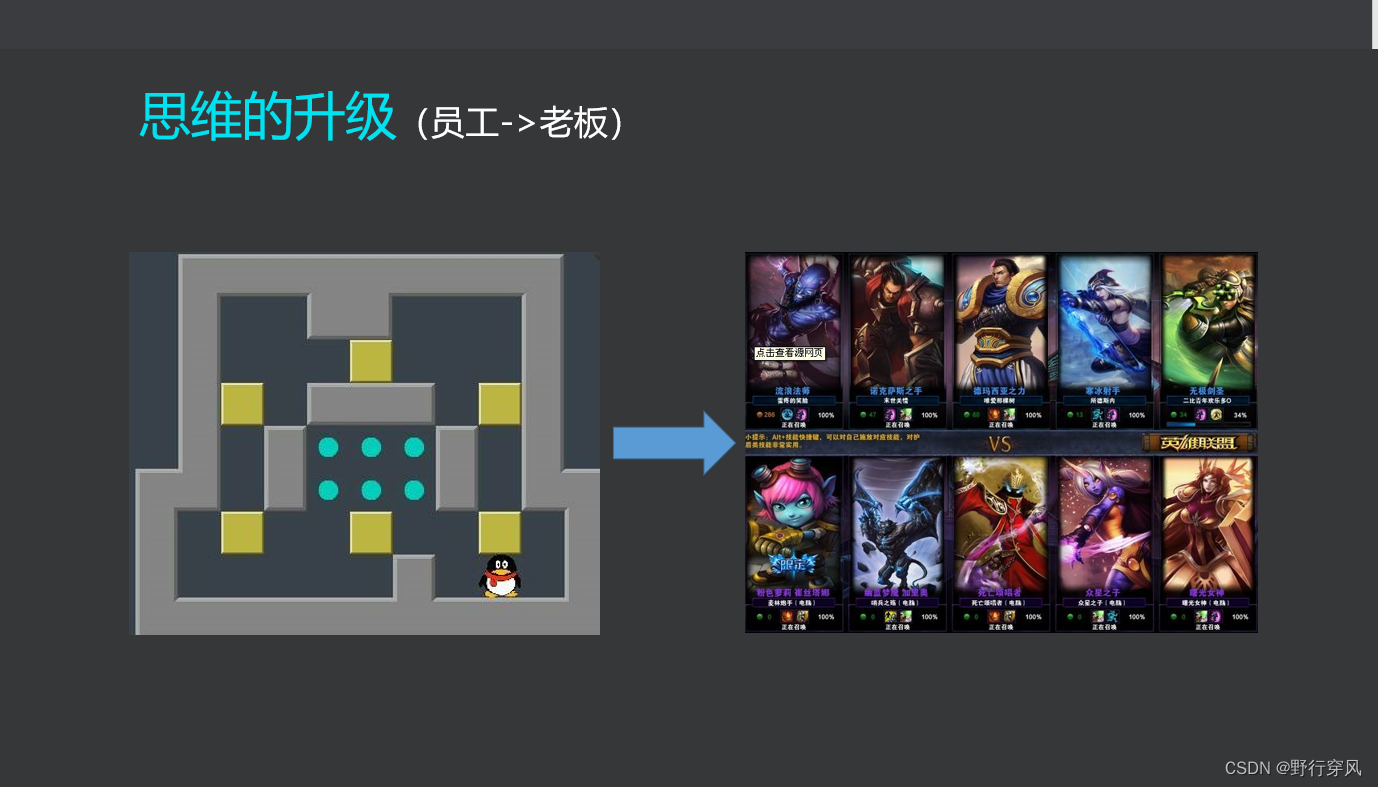
二、类与对象
1.类的声明
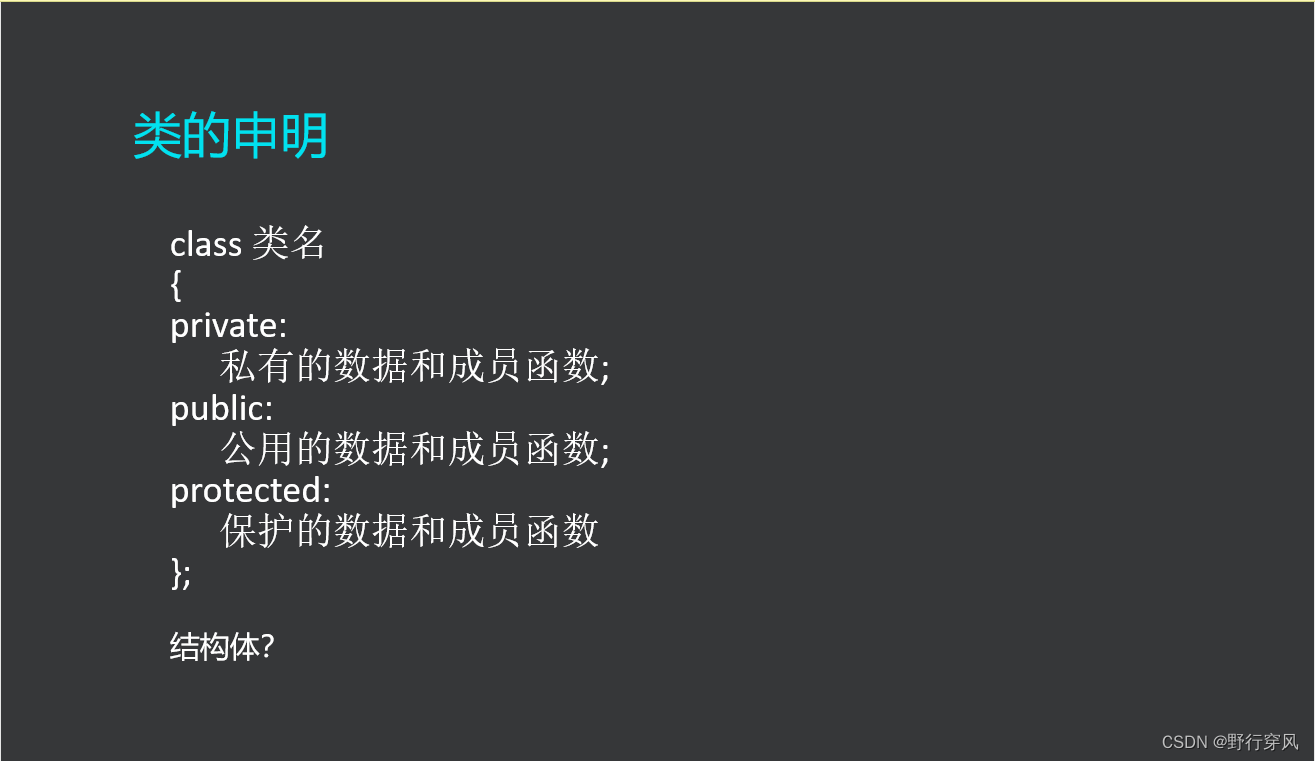
class.cpp
类中成员数据、成员函数的声明和使用。
#include <stdio.h>
class A{
public:
A()
{
printf("construct!\n");
a = 0;
}
A(int data)
{
a = data;
}
~A()
{
printf("ddddddddddd\n");
}
void show()
{
printf("xxxxxxxxxxx\n");
}
void setdata(int data)
{
a = data;
}
int getdata(void);
private:
int a;
};
int A::getdata(void)
{
return a;
}
int main()
{
A x(100);
printf("%d\n", x.getdata() );
x.show();
}
2.类的成员函数(类内、类外实现)
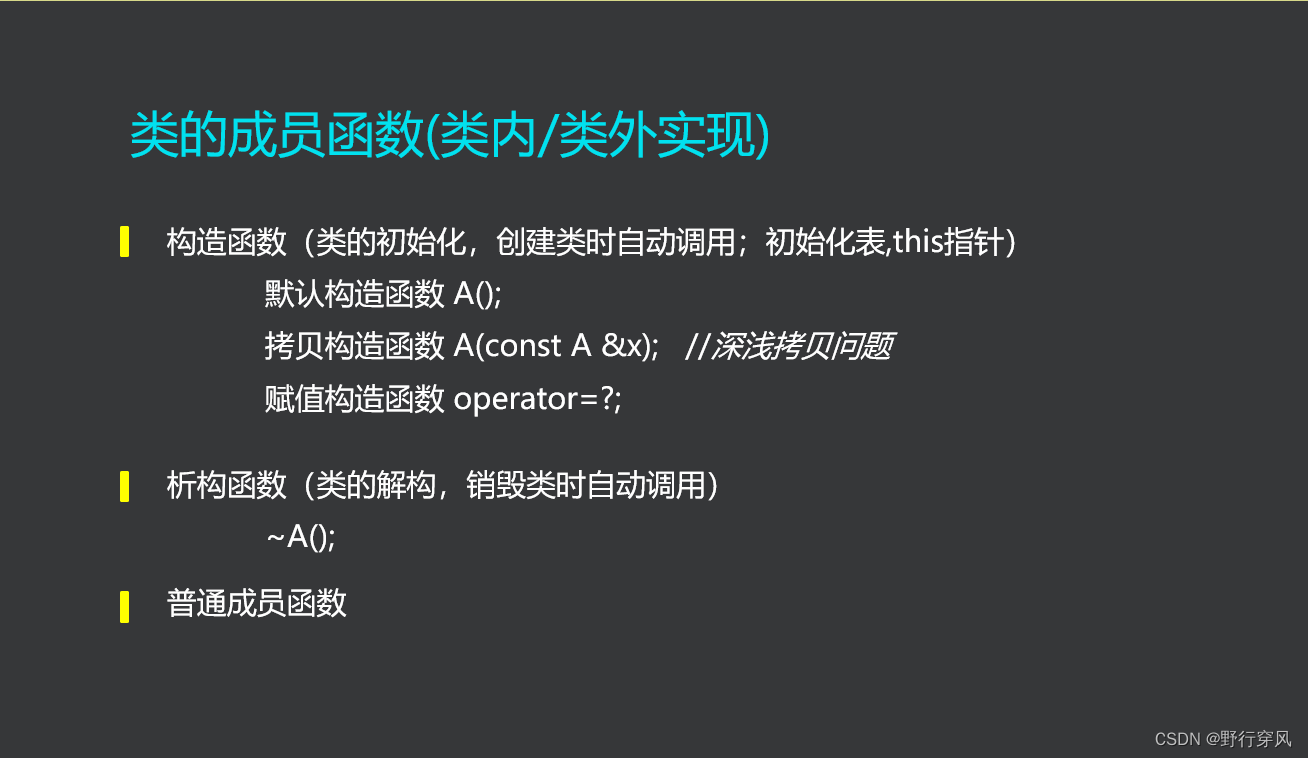
class_construct.cpp
当使用类创建新对象时的会调用的函数。
#include <stdio.h>
class A{
public:
A()
{
printf("A()\n");
}
A(int data)
{
printf("A(int data)\n");
}
~A(){
printf("~A()\n");
}
};
int main()
{
A *p = new A(1000);
A x;
A m(100);
A y = 10;
A z = y;
}
class_copy.cpp
使用A z = y语句时,会调用系统的拷贝函数,当类A的构造函数中有开辟堆空间、析构函数会释放空间时,z和y调用析构函数释放空间时会是同一个空间,会导致double free。因此应该自己创建一个拷贝构造函数。
#include <stdio.h>
#include <string.h>
class A{
public:
A()
{
printf("A()\n");
p = new char[10];
strcpy(p, "hello");
}
A(const A &x)
{
printf("A(const A &x)\n");
p = new char[10];
strcpy(p, x.p);
}
~A()
{
printf("~A()\n");
delete [] p;
}
private:
char *p;
};
int main()
{
A x;
A y = x;
}
3.常成员、常函数(C++推荐const而不用#define,mutable )
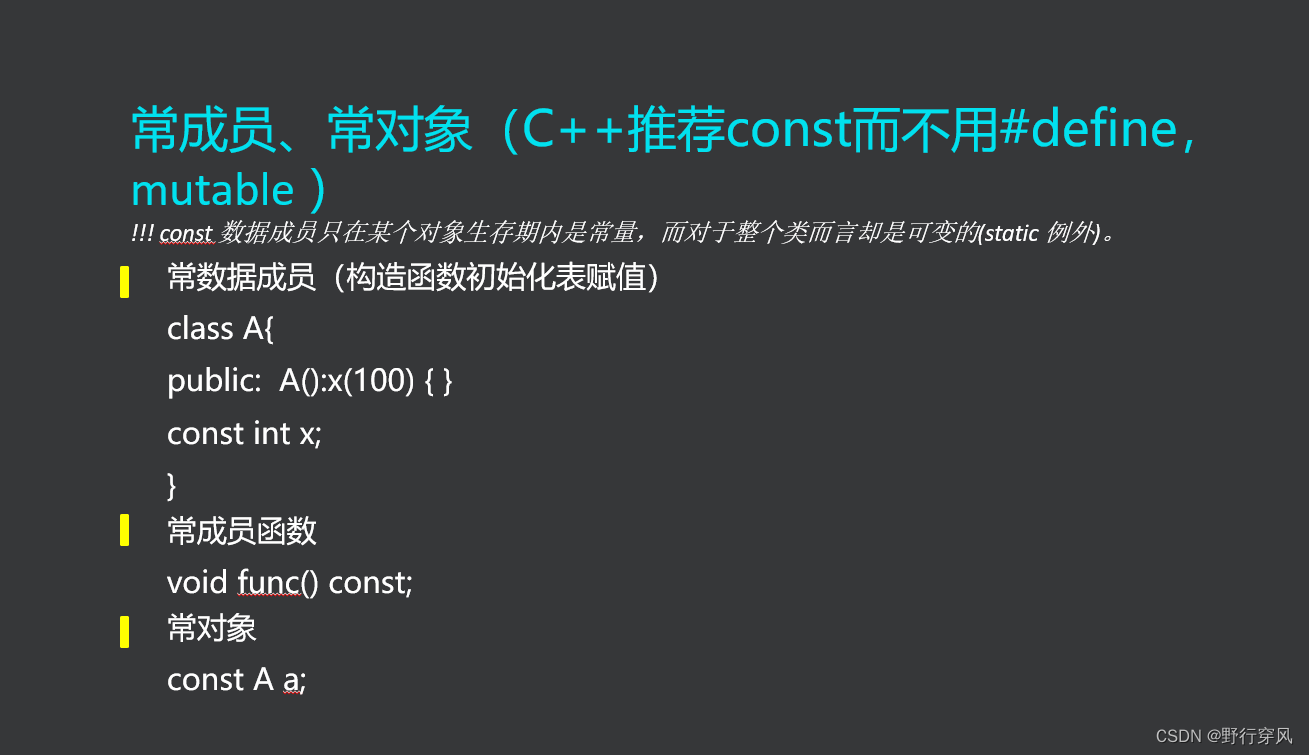
const.cpp
常成员数据、常成员函数的声明和使用。
#include <stdio.h>
class A{
public:
A(int a = 50, int data = 1000):b(data){
this->a = a;
printf("AAAAAAAAA\n");
}
~A(){
printf("~~~~~~~~~\n");
}
void show(void) const
{
printf("a = %d\n", a);
printf("b = %d\n", b);
}
private:
int a;
const int b;
};
int main()
{
A x(10,10);
x.show();
A y = 100;
y.show();
A z;
z.show();
}
4.静态成员(属于类不属于对象)
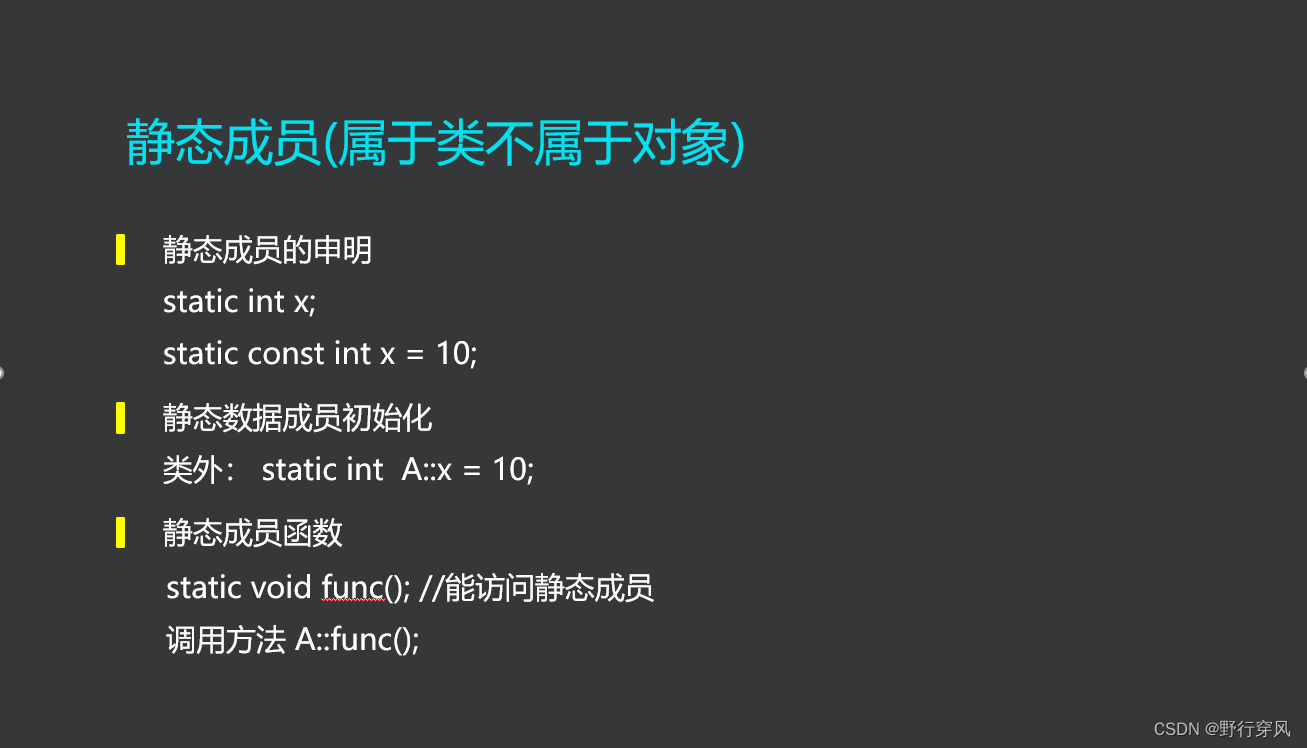
static.cpp
静态成员数据、静态成员函数的声明和使用。
#include <stdio.h>
class A{
public:
static void func(void)
{
printf("xxxxxxxxx\n");
}
static int data;
};
int A::data = 10;
int main()
{
A a;
a.func();
A::func();
A x;
x.data = 100;
printf("x.data = %d\n", x.data);
A::data = 1000;
printf("x.data = %d\n", x.data);
}
5.友元(破坏封装)
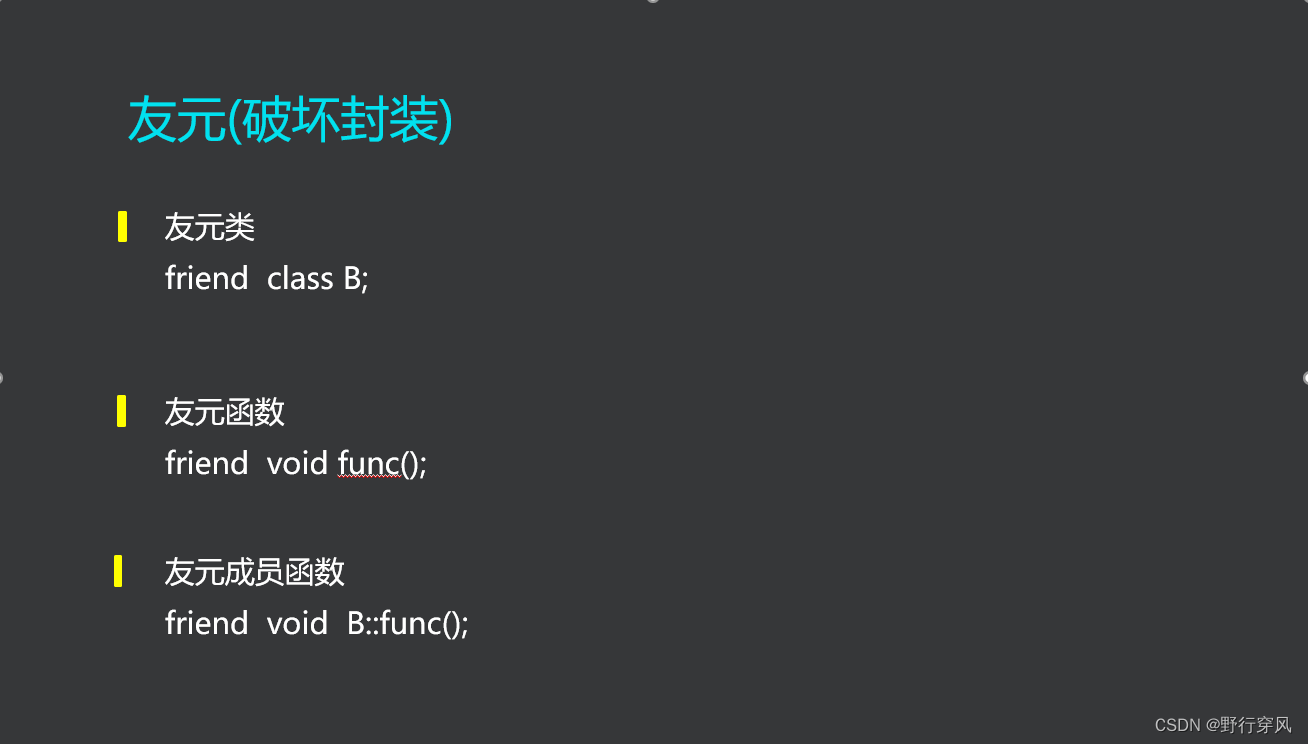
friend.cpp
友元类、友元成员函数的声明和使用。(友元函数的使用在一、2.(3)中使用过)
#include <stdio.h>
class A;
class B{
public:
void printfA(A &c);
void show() {}
};
class A{
public:
A()
{
x = 100;
}
friend void B::printfA(A &x);
private:
int x;
};
void B::printfA(A &c)
{
printf("%d\n", c.x);
}
int main()
{
A a;
B b;
b.printfA(a);
}
|