c++ Primer 第三章:字符串、向量和数组 练习答案记录
下面练习程序别忘记开头都加上这一语句
#include<iostream>
练习3.1(1) 使用恰当的using声明重做1.4.1节(第11页)的练习
using std::cin;
using std::cout;
using std::endl;
int main()
{
int i = 0;
int sum = 0, j = 50;
cout << "请输入所要进入的练习:" << endl;
cin >> i;
switch (i) {
case 1:
cout << "练习1.9 编写程序,使用while循环将50到100的整数相加\n" << endl;
while (j<= 100) {
sum += j;
j++;
}
cout << "50到100的整数相加结果:" << sum << endl;
break;
case 2:
cout << "练习1.10 编写程序,使用递减运算符在循环中按递减顺序打印出10到0之间的整数\n" << endl;
for (int a = 10; a >= 0; a--) {
cout << a << " " << endl;
}
break;
case 3:
cout << "练习1.11 提示用户输入两个整数,打印出这两整数所指定的范围内的所有整数\n" << endl;
cout << "输入两个整数,打印出这两整数所指定的范围内的所有整数:" << endl;
cin >> i >> j;
if (i < j) {
for (i; i <= j; i++) {
cout << i << endl;
}
}
else {
if (i == j) {
cout << "所输入两整数相等,无范围" << endl;
}
else {
for (i; i >= j; i--) {
cout << i << endl;
}
}
}
break;
}
}
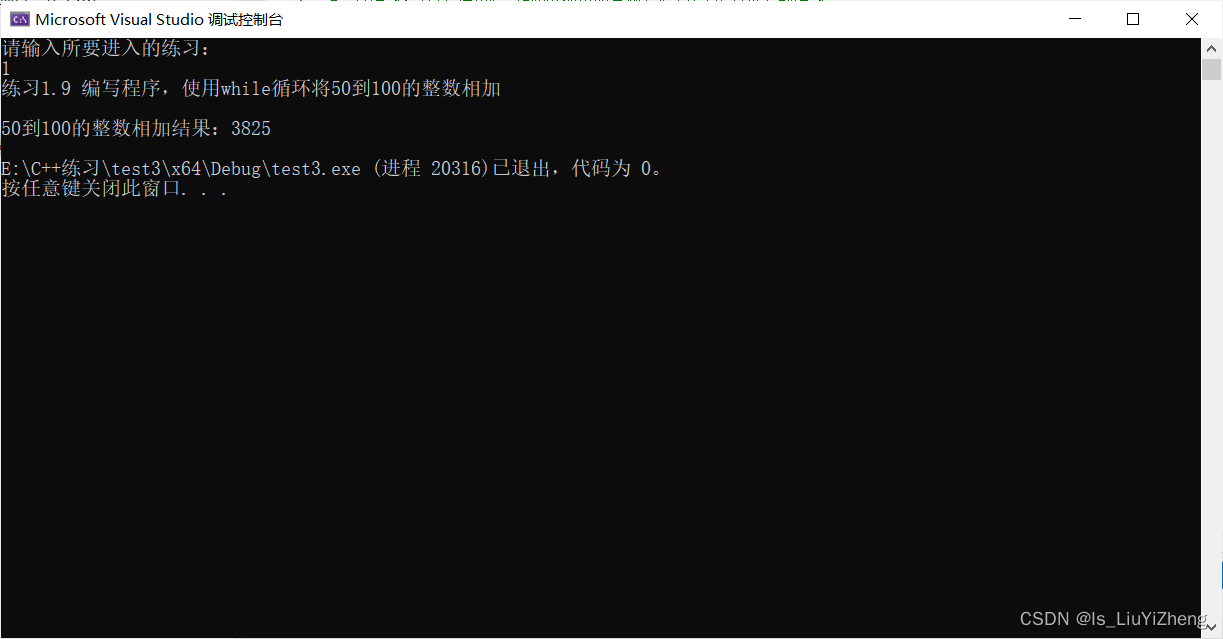 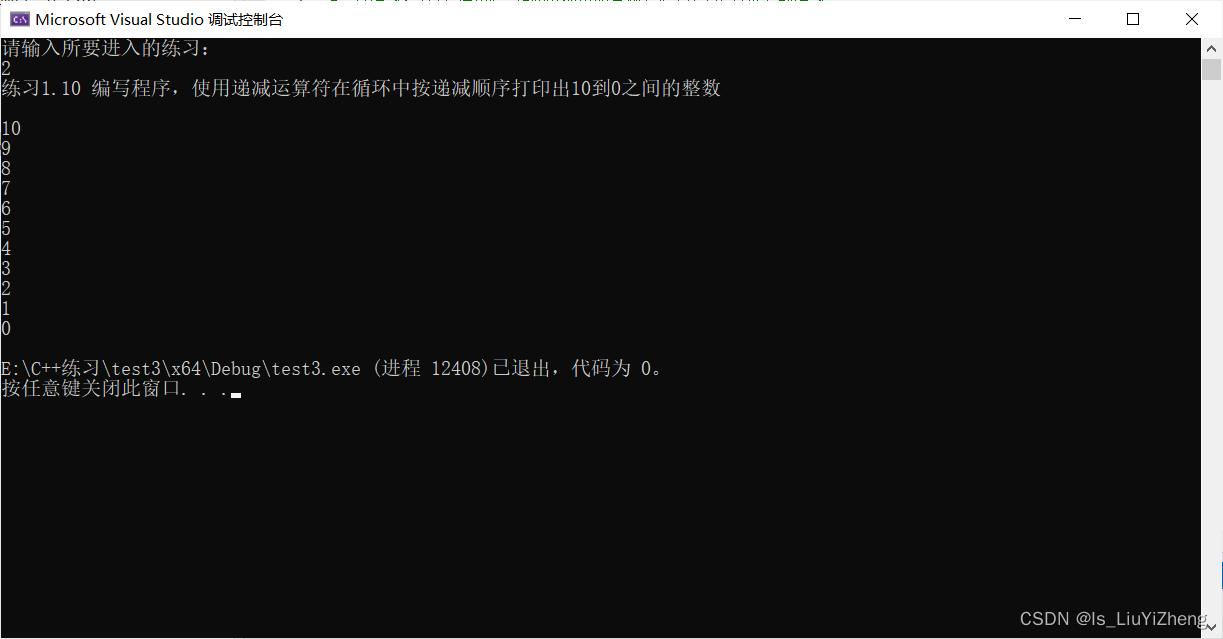 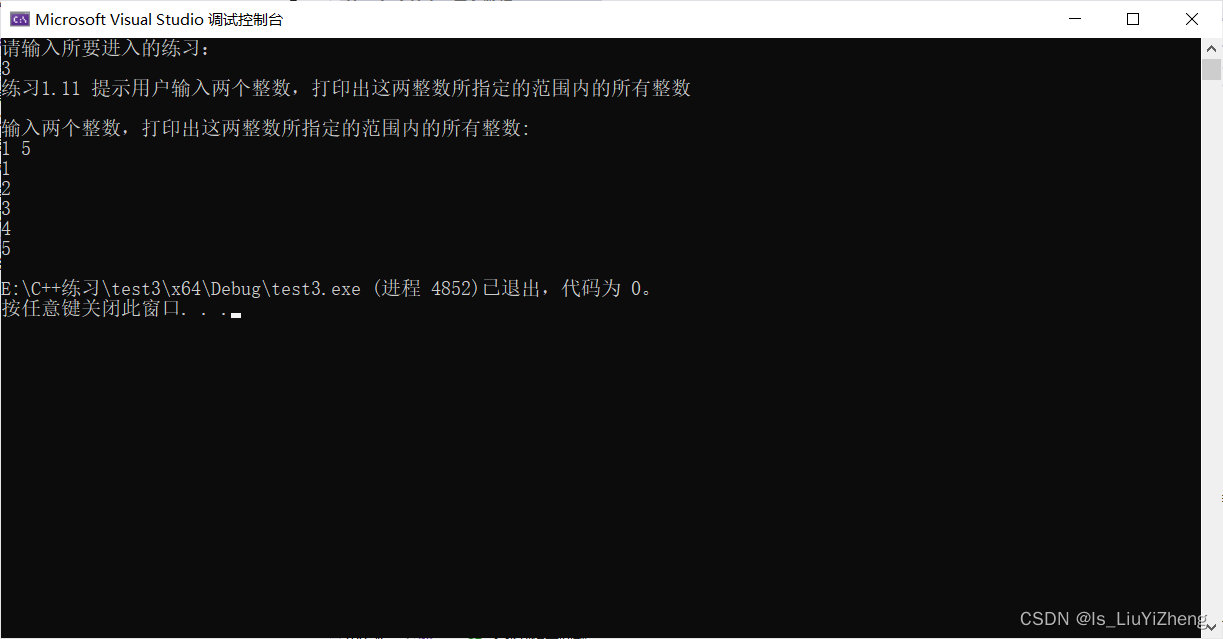
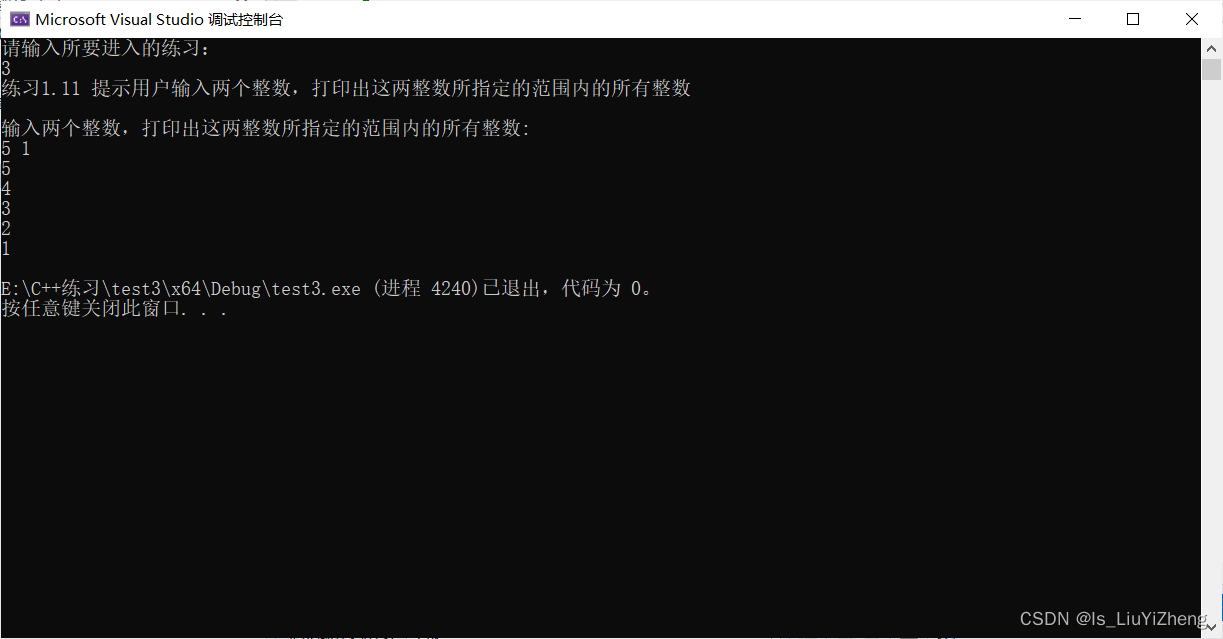 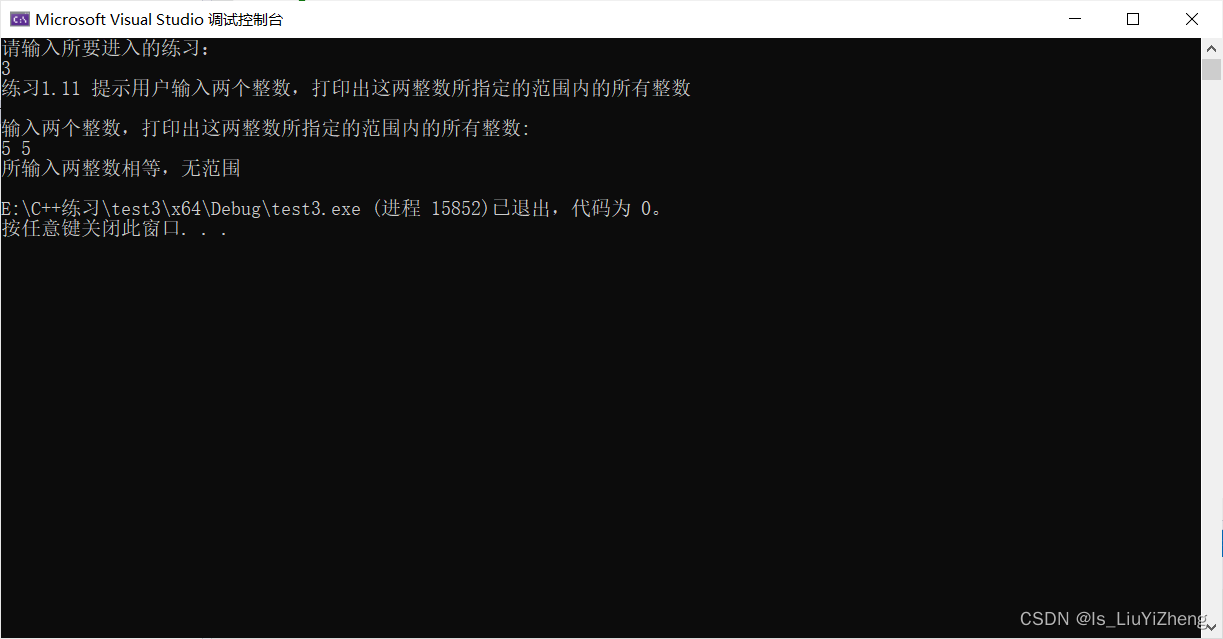
练习3.1(1) 使用恰当的using声明重做2.6.2节(第67页)的练习
练习2.41 使用你自己的Sales_data类重写1.5.1节(第20页)、1.5.2节(21页)和1.6节(22页)的练习
using std::cin;
using std::cout;
using std::endl;
using std::string;
struct Sales_data {
string book_name;
unsigned book_sales = 0;
double unit_price = 0;
double book_revenue = 0;
};
int main()
{
Sales_data data, data1, data2;
int i = 0, sum = 1;
cout << "请输入所需要进入的练习程序中:" << std::endl;
cin >> i;
switch (i) {
case 1:
cout << "练习1.23 编写程序,读取多条销售记录,并统计每个ISBN(每本书)有几条销售记录" << endl;
if (cin >> data1.book_name) {
if (data1.book_name == "over")
break;
cin >> data1.book_sales >> data1.unit_price;
}
while (cin >> data2.book_name) {
if (data2.book_name == "over")
break;
cin >> data2.book_sales >> data2.unit_price;
if (data1.book_name == data2.book_name) {
sum++;
}
else {
cout << data1.book_name << "卖出了:" << sum << "本" << endl;
data1.book_name = data2.book_name;
data1.book_sales = data2.book_sales;
data1.unit_price = data2.unit_price;
sum = 1;
}
}
break;
case 2:
cout << "练习1.24 输入表示多个ISBN的多条销售记录来测试上一个程序,每个ISBN的记录应该聚在一起" << endl;
break;
}
}
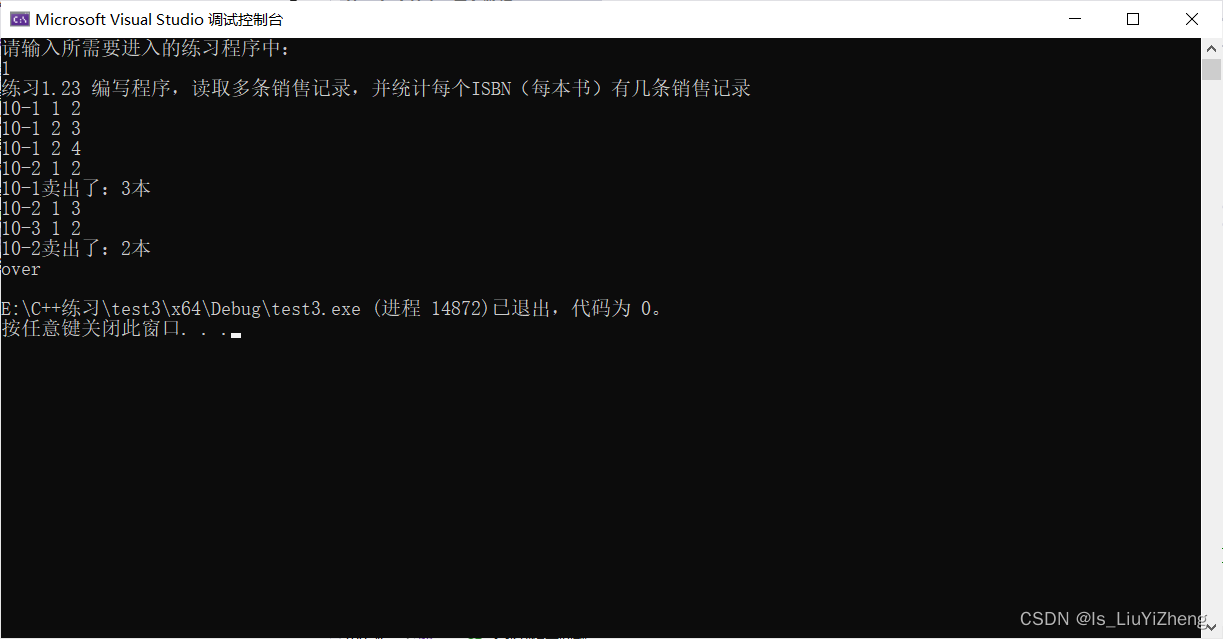
练习3.2 编写一段程序从标准输入中一次读入一整行,然后修改该程序使其一次读入一个词
#include<string>
using namespace std;
int main()
{
string input;
unsigned int i = 0;
cout << "从标准输入中一次读入一整行按1,一次读入一个词按2" << endl;
cin >> i;
switch (i) {
case 1:
cout << "从标准输入中一次读入一整行:" << endl;
cin.ignore();
getline(cin, input);
cout << input << endl;
break;
case 2:
cout << "一次读入一个词" << endl;
while (cin >> input) {
if (input == "over")
break;
cout << input << endl;
}
break;
}
}
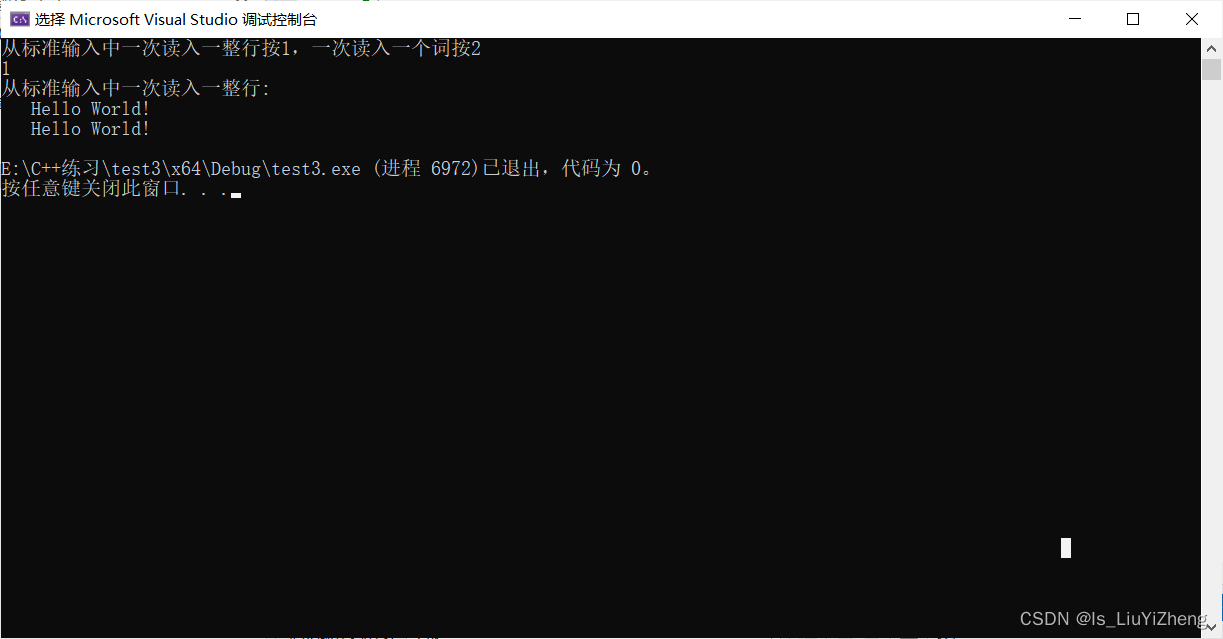 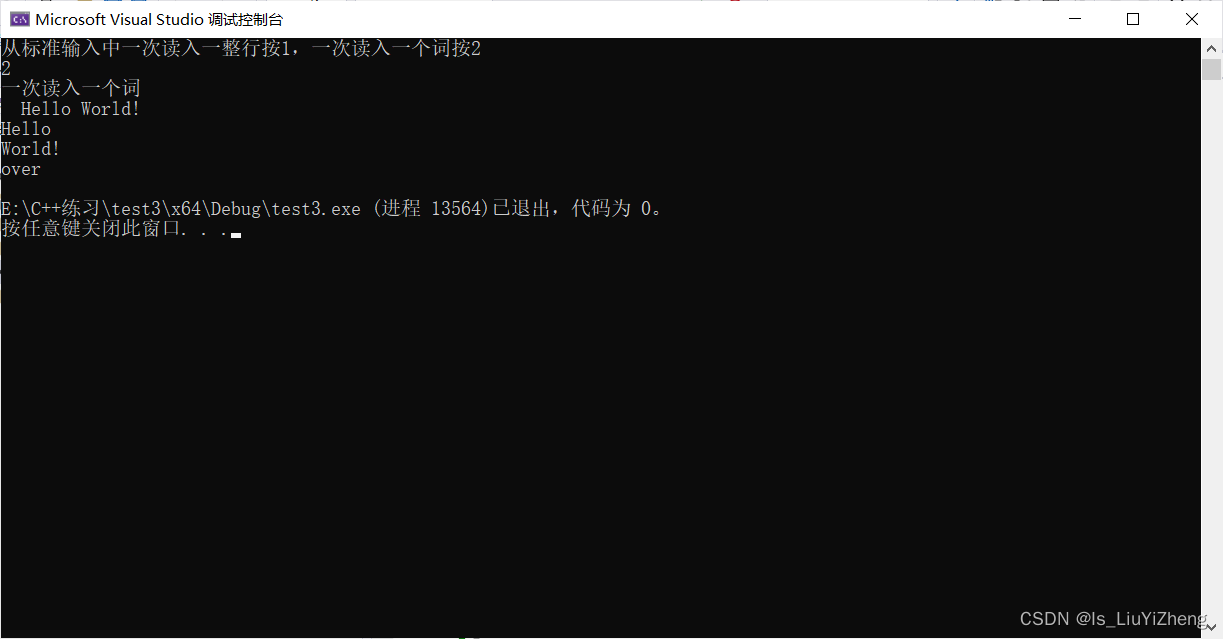
练习3.4 编写一段程序读入两个字符串,比较其是否相等并输出结果。结果不相等,输出较大的那个字符串。改写上述程序,比较输入的两个字符串是否等长,如果不等长,输出长度较大的那个字符串
#include<string>
using namespace std;
int main()
{
string input1,input2;
unsigned i = 0;
cout << "两个字符串,结果不相等,输出较大的那个字符串按1,比较输入的两个字符串是否等长,如果不等长,输出长度较大的那个字符串按2" << endl;
cin >> i;
switch (i) {
case 1:
cout << "比较两个字符串,结果不相等,输出较大的那个字符串" << endl;
cin.ignore();
getline(cin, input1);
getline(cin, input2);
if (input1 == input2) {
cout << "两字符串相同" << endl;
}
else {
if (input1 > input2) {
cout <<"第一个字符串比较大:" << input1 << endl;
}
else {
cout <<"第二个字符串比较大:" << input2 << endl;
}
}
break;
case 2:
cout << "比较输入的两个字符串是否等长,如果不等长,输出长度较大的那个字符串" << endl;
cin.ignore();
getline(cin, input1);
getline(cin, input2);
if (input1.size() == input2.size()) {
cout << "两字符串等长" << endl;
}
else {
if (input1.size() > input2.size()) {
cout << "第一个字符串长:" <<input1<< endl;
}
else {
cout << "第二个字符串长:"<<input2 << endl;
}
}
break;
}
}
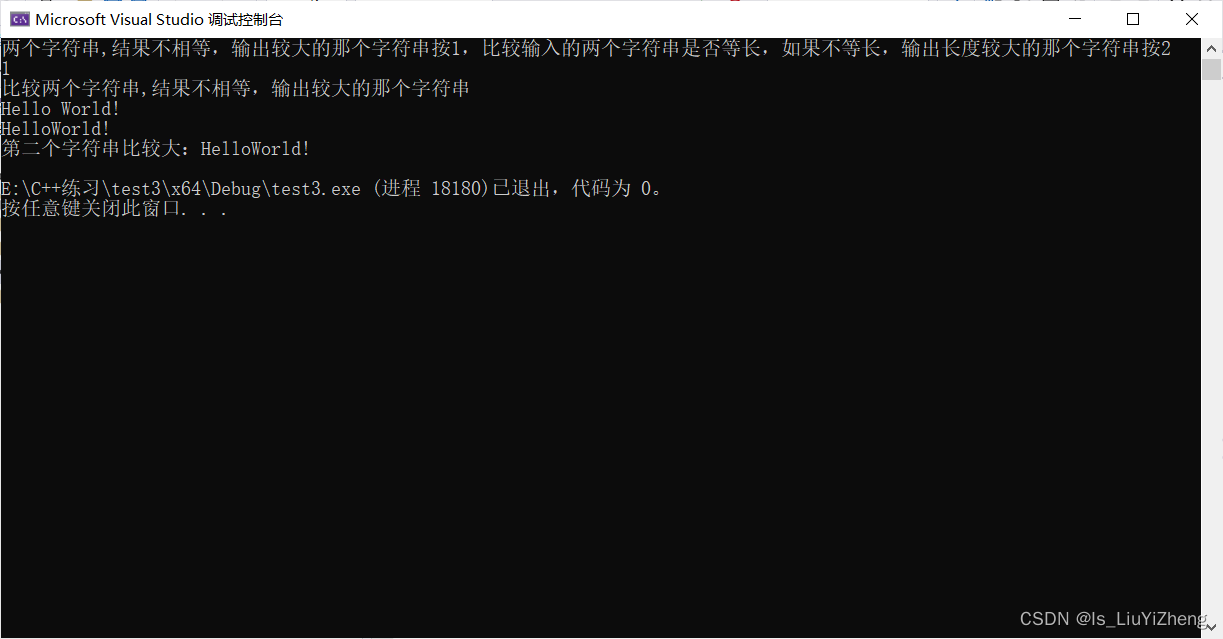 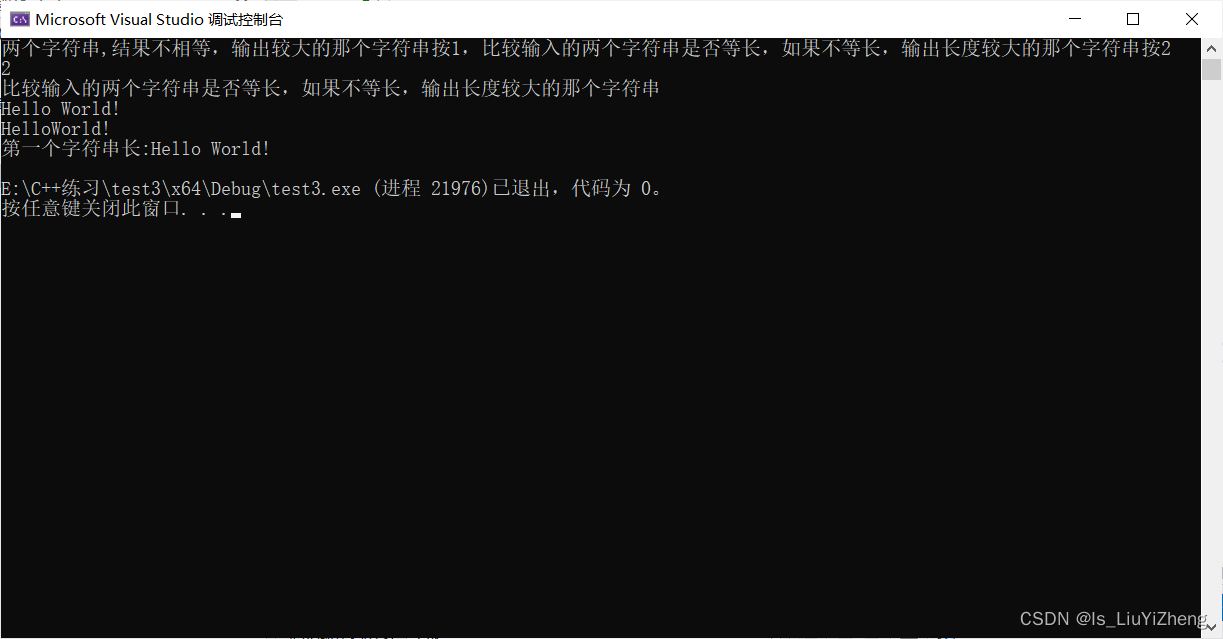
练习3.5 编写一段程序从标准输入中读入多个字符串并将它们连接在一起,输出链接成的大字符串。然后修改上述程序,用空格把输入的多个字符串分隔开来
#include<string>
using namespace std;
int main()
{
string input,output;
cout << "请输入字符串,如若结束输入则输入over" << endl;
while (getline(cin,input)) {
if (input == "over")
break;
cout << "请继续输入字符串,如若结束输入则输入over" << endl;
output = output + input;
}
cout << "入多个字符串并将它们连接在一起,输出链接成的大字符串:" << output << endl;
}
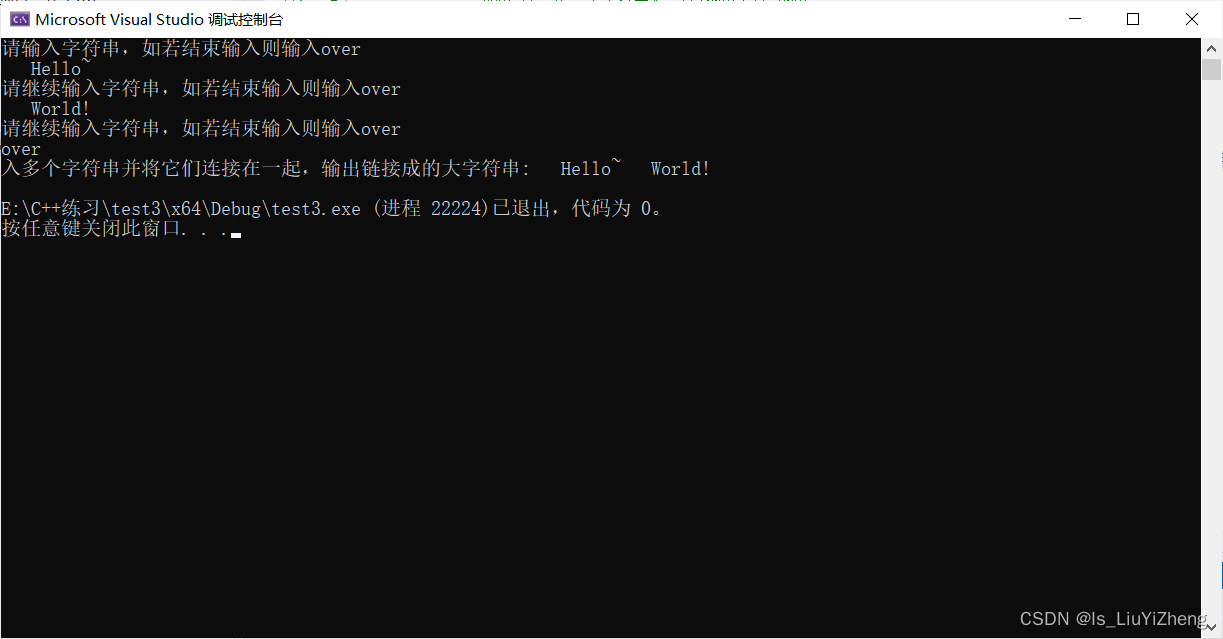
练习3.6 编写一段程序,使用范围for语句将字符串内的所有字符用X代替 法一
#include<string>
using namespace std;
int main()
{
string str = "Hello World!";
const char* a = "X";
for (auto& n : str) {
n = *a;
}
cout << str << endl;
}
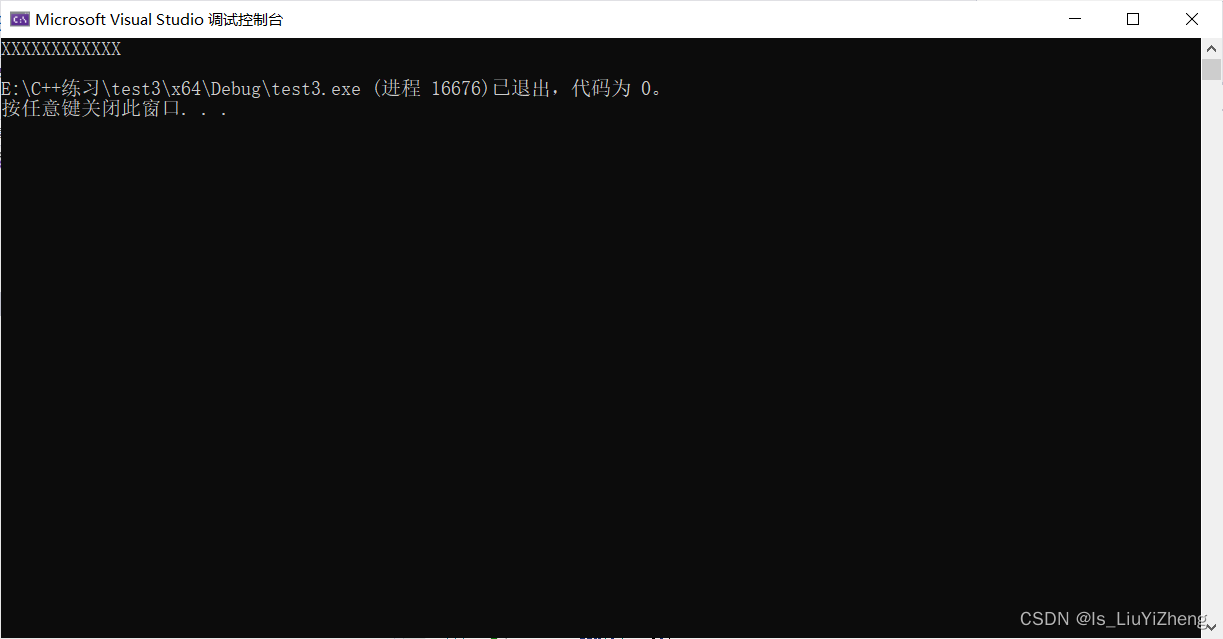
练习3.6 编写一段程序,使用范围for语句将字符串内的所有字符用X代替 法二
#include<string>
using namespace std;
int main()
{
string str = "Hello World!";
const char* a = "X";
for (int i = 0; i <= str.size();i++) {
str[i] = *a;
}
cout << str << endl;
}
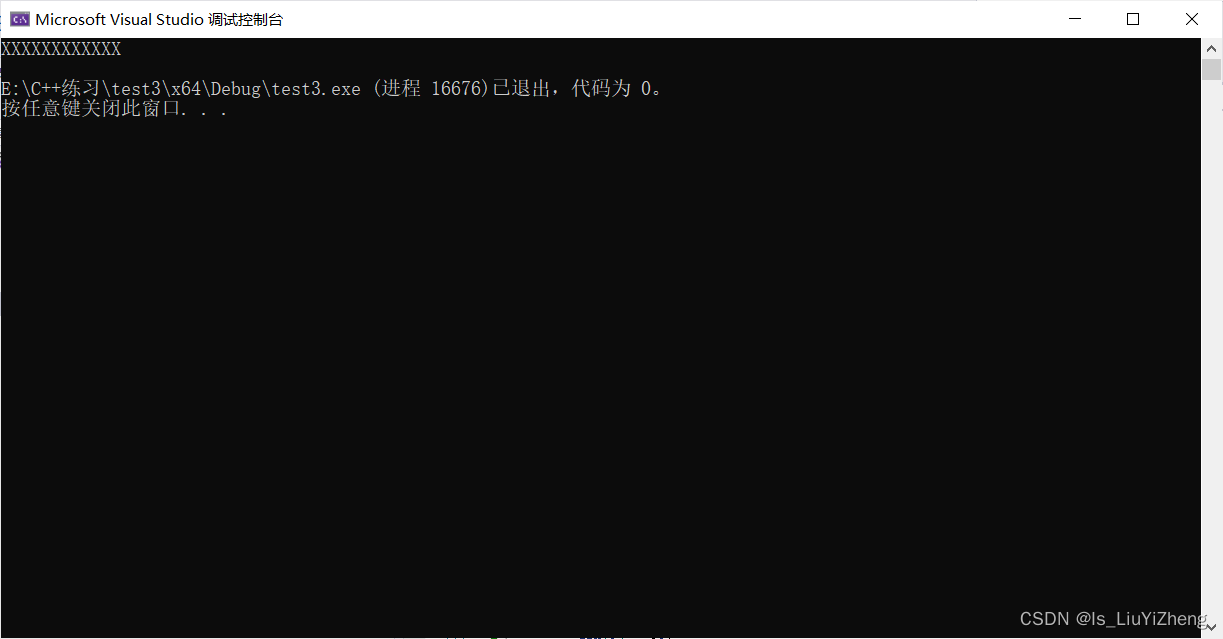
练习3.6 编写一段程序,使用范围for语句将字符串内的所有字符用X代替 法三
#include<string>
using namespace std;
int main()
{
string str = "Hello World!";
const char* a = "x";
for (int i = 0; i <= str.size();i++) {
str[i] = toupper(*a);
}
cout << str << endl;
}
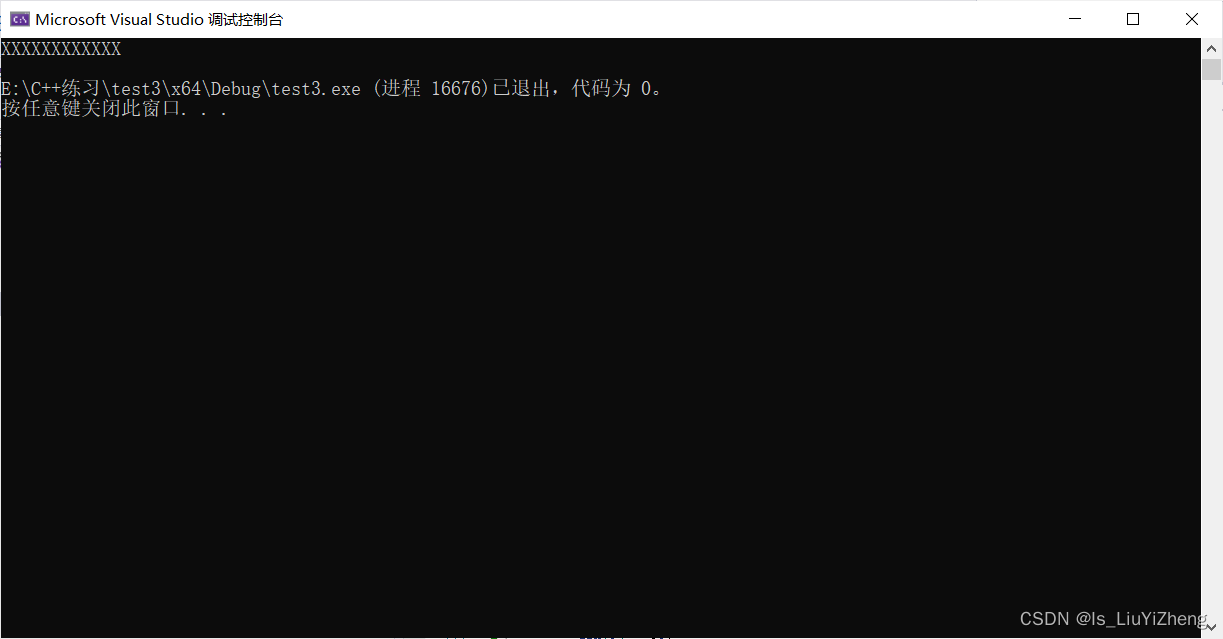
练习3.6 编写一段程序,使用范围for语句将字符串内的所有字符用X代替 法四
#include<string>
using namespace std;
int main()
{
string str = "Hello World!";
for (int i = 0; i <= str.size();i++) {
str[i] = 'X';
}
cout << str << endl;
}
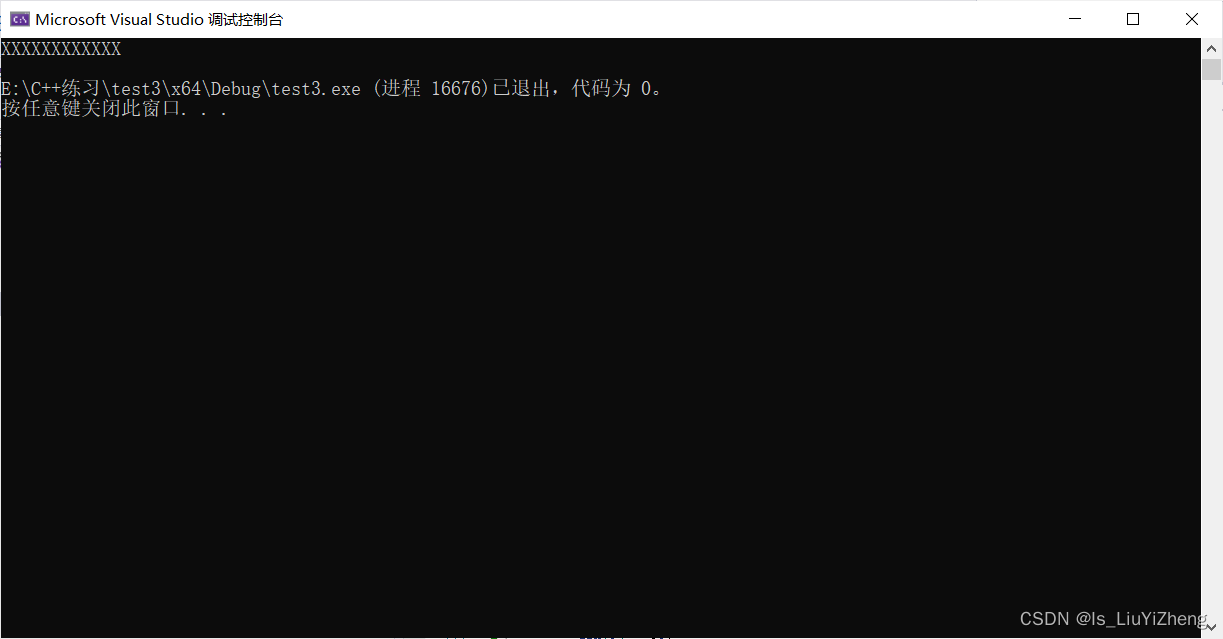
练习3.7 就上一题如果将循环控制变量的类型设为char将发生什么?先估计一下结果,然后实际编程进行验证
答案是类型不匹配,字符字面值可以匹配,变成char型就会显示无法给const char*赋char型,所以3.6中我所用的法一到法三都是为了解决这个问题
练习3.8 分别用while循环和传统的for循环重写第一题的程序,你觉得哪种形式更好呢?为什么?
我认为for循环更好,一般情况所声明的变量不会对其他程序产生影响。
#include<string>
using namespace std;
int main()
{
string str = "Hello World!";
string::size_type n = 0;
while (n<=str.size()) {
str[n] = 'X';
n++;
}
cout << str << endl;
}
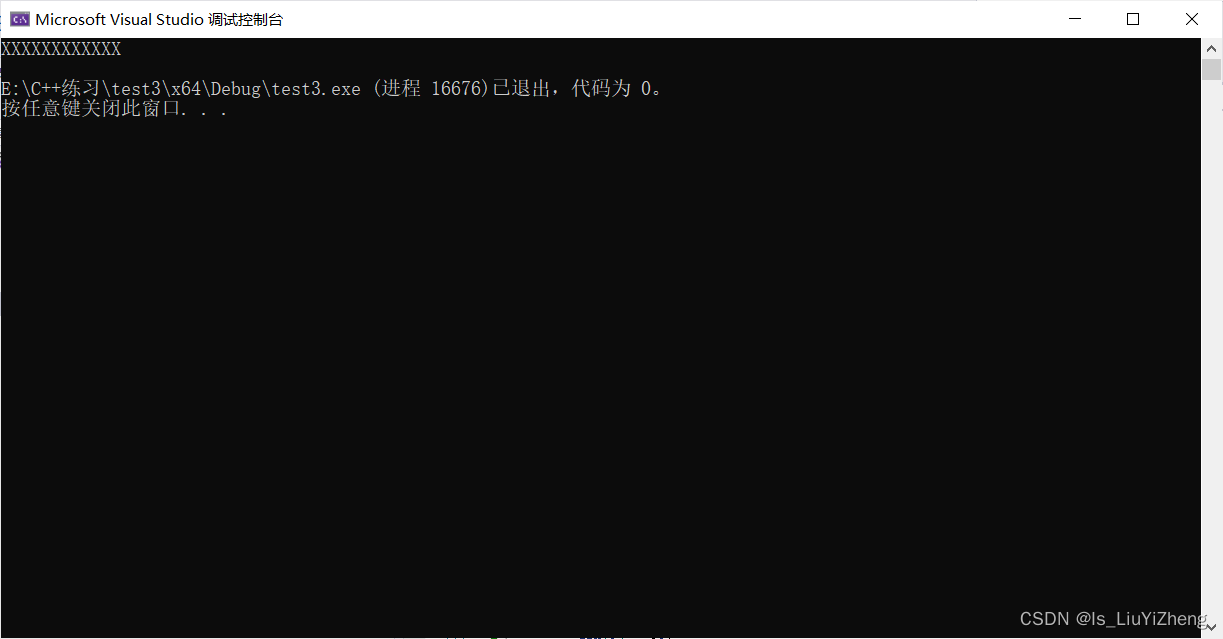
练习3.9 下面的程序有何作用?它合法吗?如果不合法,为什么?
合法
#include<string>
using namespace std;
int main()
{
string s;
cout << s[0] << endl;
}
练习3.10 编写一段程序,读入一个包含标点符号的字符串,将标点符号去除后输出字符串剩余的部分
#include<string>
using namespace std;
int main()
{
string str,output;
cout << "读入一个包含标点符号的字符串,将标点符号去除后输出字符串剩余的部分" << endl;
getline(cin, str);
for (auto& n : str) {
if (!ispunct(n)) {
output += n;
}
}
cout << "将标点符号去除后输出字符串剩余的部分为:" << output << endl;
}
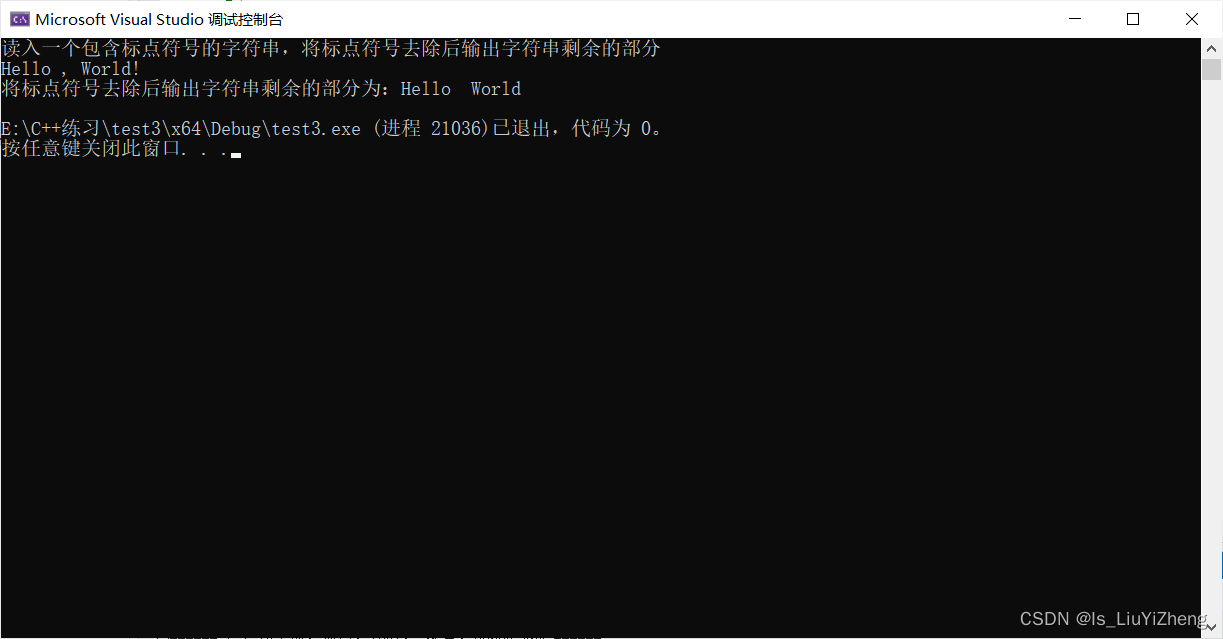
练习3.11 下面的范围for语句合法吗?如果合法,c的类型是什么?
合法:对const char的引用,但是不能对c进行改变,如果改变就会像下面一样报错
#include<string>
using namespace std;
int main()
{
const string s = "Keep out!";
for (auto& c : s) {
c = 'X';
}
}
练习3.12 下列vector对象的定义有不正确的吗?如果有,请指出来。对于正确的,描述其结果;对于不正确的,说明其错误的原因
#include<string>
#include<vector>
using namespace std;
int main()
{
vector<vector<int>>ivec;
vector<string>svec(10, "null");
}
练习3.13 下列的vector对象各包含多少个元素?这些元素的值分别是多少?
#include<string>
#include<vector>
using namespace std;
int main()
{
vector<int> v1;
vector<int>v2(10);
vector<int>v3(10, 42);
vector<int>v4{ 10 };
vector<int>v5{ 10,42 };
vector<string>v6{ 10 };
vector<string>v7{ 10,"hi" };
}
练习3.14 编写一段程序,用cin读入一组整数并把它们存入一个vector对象
#include<string>
#include<vector>
using namespace std;
int main()
{
vector<int> digit;
int i = 0;
while (cin >> i) {
digit.push_back(i);
}
}
练习3.15 改写上一题程序,不过这次读入的是字符串
#include<string>
#include<vector>
using namespace std;
int main()
{
vector<string> str;
string i;
while (cin >> i) {
str.push_back(i);
}
}
|