本阶段主要针对C++泛型编程和STL技术做详细学习,探讨C++更深层的使用 谨记:温故而知新。
1 模板
1.1 模板的概念
模板就是建立通用的模具,大大提高复用性。
- 例如生活中的模板:
- 模板的特点:
- 模板不可以直接使用,它只是一个框架
- 模板的通用并不是万能的
- C++另一种编程思想称为泛型编程,主要利用的技术就是模板。
- C++提供两种模板机制:
首先我们来看看函数模板吧~
1.2 函数模板
1.2.1 函数模板语法
函数模板作用: 建立一个通用函数,其函数返回值类型和形参类型可以不具体制定,用一个虚拟的类型来代表。 语法: template<typename T> 函数声明或定义 解释:
template —声明创建模板typename — 表示其后面的符号是一种数据类型,可以用class 代替T ---- 通用的数据类型,名称可以替换,统称为大写字母
示例代码:
#include <iostream>
using namespace std;
void swapInt(int &a, int &b){
int temp = a;
a = b;
b = temp;
}
void swapDouble(double &a,double &b){
double temp = a;
a = b;
b = temp;
}
template<typename T>
void mySwap(T &a, T &b){
T temp = a;
a = b;
b = temp;
}
void test01(){
int a = 10;
int b = 20;
mySwap(a, b);
mySwap<int>(a, b);
cout << "a: " << a << " b: " << b << endl;
double c = 1.1;
double d = 2.2;
swapDouble(c, d);
cout << "c = " << c << " d = " << d << endl;
}
int main(){
test01();
system("pause");
return 0;
}
总结:
- 函数模板利用关键字
template - 使用函数模板有两种方式:自动类型推导、显示指定类型
- 模板的目的是为了提高复用性,将类型参数化
1.2.2 函数模板注意事项
注意事项:
- 自动类型推导,必须推导出一致的数据类型T,才可以使用
- 模板必须要确定出T的数据类型,才可以使用
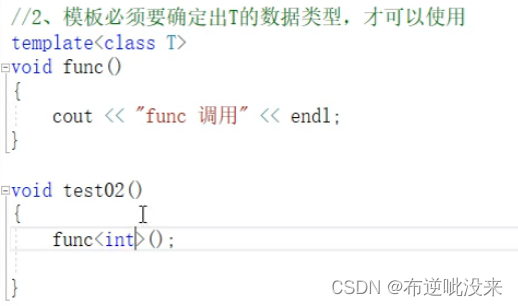
1.2.3 函数模板案例
案例描述:
- 利用函数模板封装一个排序的函数,可以对不同数据类型数组进行排序
- 排序规则从大到小,排序算法为选择排序
- 分别利用char数组和int数组进行测试
代码示例:
#include <iostream>
using namespace std;xzvx
template<class T>
void mySwap(T &a, T &b){
T temp = a;
a = b;
b = temp;
}
template<class T>
void mySort(T arr[], int len){
for(int i = 0; i < len; i++){
int max = i;
for(int j = i + 1; j < len; j++){
if(arr[max] < arr[j]){
max = j;
}
}
if (max != i){
mySwap(arr[max], arr[i]);
}
}
}
template<class T>
void printArray(T arr[], int len){
for(int i = 0; i < len; i++){
cout << arr[i] <<" ";
}
cout << endl;
}
void test01(){
char charArr[] = "jklae";
int num = sizeof(charArr) / sizeof(char);
mySort(charArr, num);
printArray(charArr, num);
}
void test02(){
int intArr[] = {3, 5, 2, 9, 6, 7};
int num = sizeof (intArr) / sizeof (int);
mySort(intArr, num);
printArray(intArr, num);
}
int main(){
test01();
test02();
system("pause");
return 0;
}
运行结果: 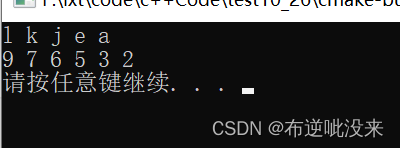
1.2.4 普通函数与函数模板的区别
普通函数与函数模板区别:
- 普通函数调用时可以发生自动类型转换(隐式类型转换)
- 函数模板调用时,如果利用自动类型推导,不会发生隐式类型转换
- 如果利用显示指定类型的方式,可以发生隐式类型转换
为了对两者区别有更清晰的认知,接下来我们来看几个例子。
- 首先写一个普通函数,返回值为两个数之和。
代码示例:
#include <iostream>
using namespace std;
int myAdd01(int a, int b){
return a+b;
}
void test01(){
int a = 10;
int b = 20;
char c = 'c';
cout << myAdd01(a, c) << endl;
}
int main(){
test01();
system("pause");
return 0;
}
- 运行结果:
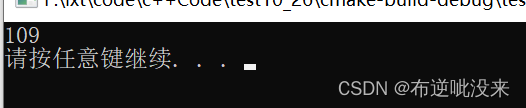 - 由运行结果可知,当调用普通函数时,
char 类型的c 被转换为int型的与之对应的ASSCI 码值,再与a 相加。
- 而当直接调用函数模板时,则不会发生隐式类型转换,反而会报错。
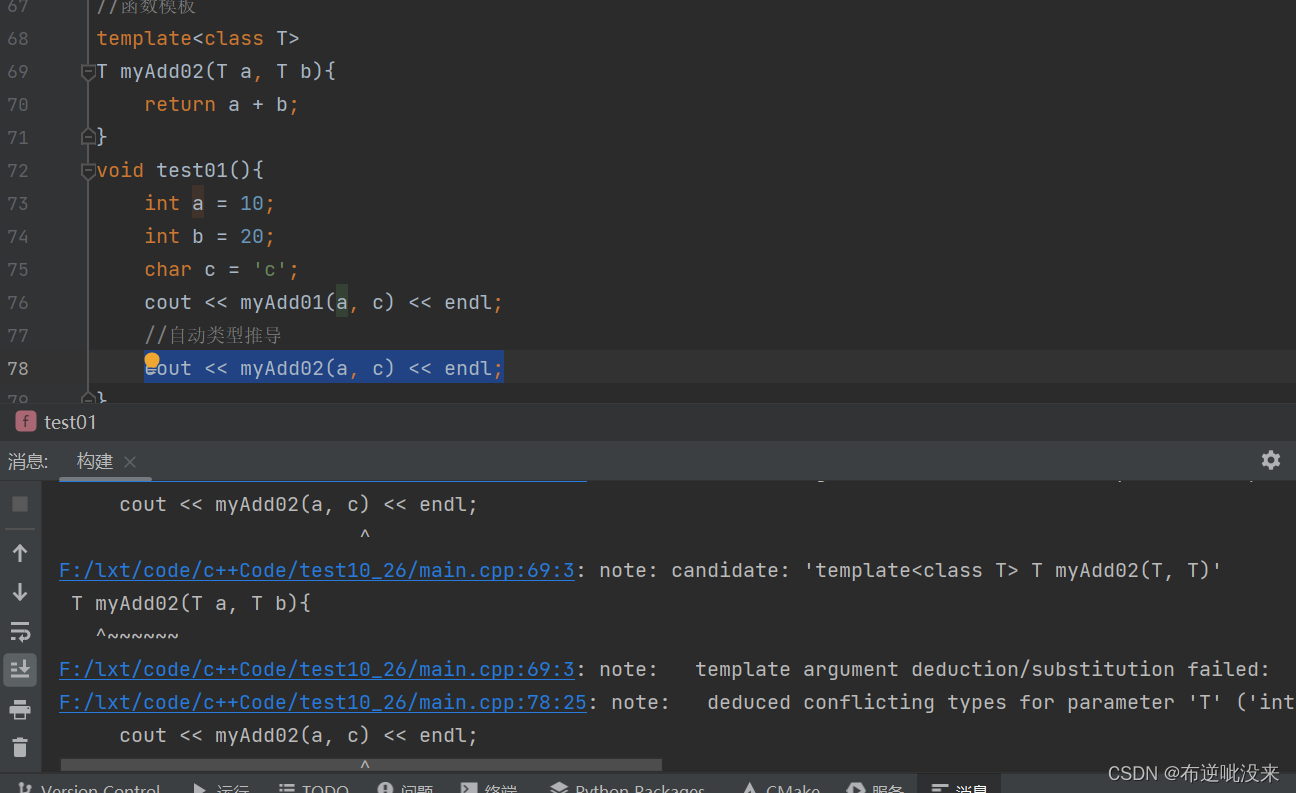 - 因此当调用函数模板时,需利用显示指定类型的方式,才可以发生隐式类型转换。
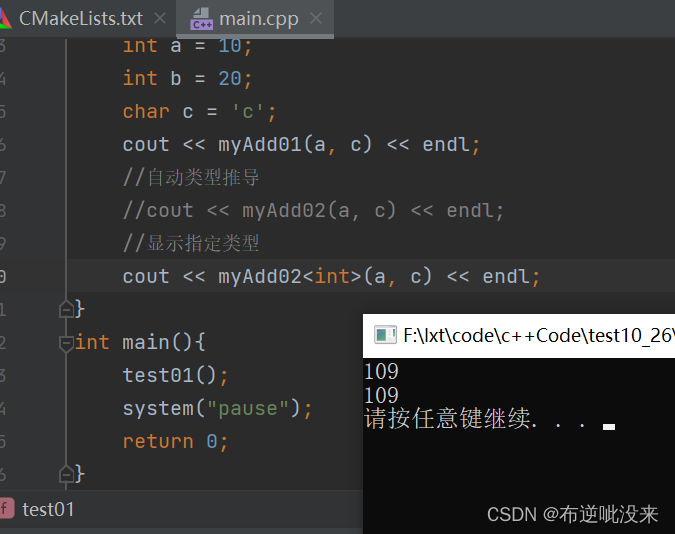 - 总结:建议使用显示指定类型的方式,调用函数模板,因为可以自己确定通用类型T。
1.2.5 普通函数与函数模板的调用规则
调用规则如下:
- 如果函数模板和普通函数都可以实现,优先调用普通函数
- 可以通过空模板参数列表来强制调用函数模板
- 函数模板也可以发生重载
- 如果函数模板可以产生更好的匹配,优先调用函数模板
示例代码:
#include <iostream>
using namespace std;
void myPrint(int a, int b){
cout << "普通函数调用" << endl;
}
template<class T>
void myPrint(T a, T b){
cout << "函数模板调用" << endl;
}
template<class T>
void myPrint(T a, T b, T c){
cout << "调用重载的模板" << endl;
}
void test01(){
int a = 10;
int b = 10;
myPrint(a, b);
myPrint<>(a, b);
myPrint(a, b, 20);
char c1 = 'a';
char c2 = 'b';
myPrint(c1, c2);
}
int main(){
test01();
system("pause");
return 0;
}
运行结果: 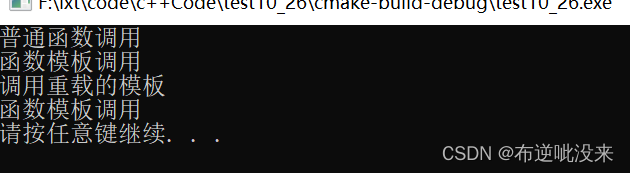
1.2.6 模板的局限性
局限性:
模板的通用性并不是万能的
代码示例1:
void f(T a, T b){
a = b;
}
在上述代码中提供的赋值操作,如果传入的a和b是一个数组,就无法实现了 代码示例2:
template<class T>
void f(T a, T b){
if(a > b) {...}
}
在上述代码中,如果T的数据类型传入的是像Person 这样的自定义数据类型,也无法正常运行
因此C++为了解决这种问题,提供模板的重载,可以为这些特定的类型提供具体化的模板(前面所说过的运算符重载也可以实现,不过比较麻烦,此处不考虑)
代码示例:
#include <iostream>
#include <string>
using namespace std;
class Person{
public:
Person(string name, int age){
this->m_Name = name;
this->m_age = age;
}
string m_Name;
int m_age;
};
template<class T>
bool myCompare(T &a, T &b){
if(a == b){
return true;
} else{
return false;
}
}
template<> bool myCompare(Person &p1, Person &p2){
if(p1.m_Name == p2.m_Name && p1.m_age == p2.m_age){
return true;
} else{
return false;
}
}
void test01(){
int a = 10;
int b = 20;
bool ret = myCompare(a, b);
if(ret){
cout << "a == b" << endl;
} else{
cout << "a != b" << endl;
}
}
void test02(){
Person p1("Tom", 10);
Person p2("Tom", 10);
bool ret = myCompare(p1, p2);
if(ret){
cout << "p1 == p2" << endl;
}else{
cout << "p1 != p2" << endl;
}
}
int main(){
test02();
system("pause");
return 0;
}
总结:
- 利用具体化的模板,可以解决自定义类型的通用化
- 学习模板并不是为了写模板,而是在STL能够运用系统提供的模板
1.3 类模板
1.3.1 类模板语法
类模板作用
- 建立一个通用类,类中的成员 数据类型可以不具体制定,用一个虚拟的类型来代表
语法: template<typename T> 类
解释:
template —声明创建模板typename — 表示其后面的符号是一种数据类型,可以用class 代替T ---- 通用的数据类型,名称可以替换,统称为大写字母
示例代码:
#include <iostream>
#include <string>
using namespace std;
template<class NameType, class AgeType>
class Person{
public:
Person(NameType name, AgeType age){
this->m_Name = name;
this->m_Age = age;
}
void showPerson(){
cout << "name : " << this->m_Name << endl;
cout << "age : " << this->m_Age << endl;
}
NameType m_Name;
AgeType m_Age;
};
void test01(){
Person<string, int>p1("孙悟空", 999);
p1.showPerson();
}
int main(){
test01();
system("pause");
return 0;
}
总结:类模板和函数模板语法相似,在声明模板template 后面加类,此类称为类模板。
1.3.2 类模板和函数模板区别
类模板与函数模板区别主要有两点:
- 类模板没有自动类型推导的使用方式
- 类模板在模板参数列表中可以有默认参数
在类模板参数列表中使用默认参数(AgeType 的默认参数为int )
template<class NameType, class AgeType = int>
class Person{
public:
Person(NameType name, AgeType age){
this->m_Name = name;
this->m_Age = age;
}
void showPerson(){
cout << "name : " << this->m_Name << endl;
cout << "age : " << this->m_Age << endl;
}
NameType m_Name;
AgeType m_Age;
};
- 当说明了默认参数时,调用模板时没向有默认参数那一项声明类型时会自定使用声明的默认类型。
void test01(){
Person<string>p1("孙悟空", 999.1);
p1.showPerson();
}
运行结果: 
void test01(){
Person<string, double>p1("孙悟空", 999.1);
p1.showPerson();
}
运行结果: 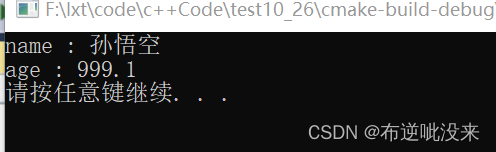
总结:
- 类模板使用只能用显示指定类型方式
- 类模板中的模板参数列表可以有默认参数
1.3.4 类模板对象做函数参数
学习目标:
一共有三种传入方式:
- 指定传入的类型 (最常用) – 直接显示对象的数据类型
void PrintPerson01(Person<string, int>&p){
p.showPerson();
}
void test01(){
Person<string, int>p("absdui", 23);
PrintPerson01(p);
}
- 参数模板化 – 将对象中的参数变为模板进行传递
template<class T1, class T2>
void PrintPerson02(Person<T1, T2>&p){
p.showPerson();
cout << "T1的类型为:" << typeid(T1).name() << endl;
cout << "T2的类型为:" << typeid(T2).name() << endl;
}
void test02(){
Person<string, int>p("absdui", 23);
PrintPerson02(p);
}
- 整个类模板化 – 将这个对象类型模板化进行传递
template<class T>
void PrintPerson03(T &p){
p.showPerson();
cout << "T的数据类型为: " << typeid(T).name() << endl;
}
void test03(){
Person<string, int>p("absdui", 23);
PrintPerson03(p);
}
总结:
- 通过类模板创建的对象,可以有三种方式向函数中进行传参
- 使用比较广泛是第一种:指定传入的类型
1.3.5 类模板与继承
当类模板碰到继承时,需要注意一下几点:
- 当子类继承的父类是一个类模板时,子类在声明的时候,要指定出父类中T的类型
- 如果不指定,编译器无法给子类分配内存
- 如果想灵活指定出父类中
T 的类型,子类也需变为类模板
接下来看看代码示例:
- 当子类继承的父类是一个类模板时,子类在声明的时候,要指定出父类中T的类型
template<class T>
class Base{
T m;
};
class Son :public Base<int>{
};
void test01(){
Son s1;
}
- 如果想灵活指定出父类中
T 的类型,子类也需变为类模板
template<class T>
class Base{
T m;
};
template<class T1, class T2>
class Son2 :public Base<T2>{
public:
Son2(){
cout << "T1类型:" << typeid(T1).name() << endl;
cout << "T2类型:" << typeid(T2).name() << endl;
}
T1 m1;
};
void test02(){
Son2<int, char>s2;
}
总结:如果父类是类模板,子类需要指定出父类中T的数据类型
1.3.6 类模板成员函数类外实现
代码示例:
#include <iostream>
using namespace std;
template<class T1, class T2>
class Person{
public:
Person(T1 name, T2 age);
void showPerson();
T1 m_Name;
T2 m_Age;
};
template<class T1, class T2>
Person<T1, T2>::Person(T1 name, T2 age){
this->m_Name = name;
this->m_Age = age;
}
template<class T1, class T2>
void Person<T1, T2>::showPerson(){
cout << "姓名: " << this->m_Name << " 年龄:" << this->m_Age << endl;
}
void test01(){
Person<string, int>p("tom", 20);
p.showPerson();
}
int main(){
test01();
system("pause");
return 0;
}
总结:类模板中成员函数类外实现时,需要加上模板参数列表。
1.3.7 类模板分文件编写
学习目标:
- 掌握类模板成员函数分文件编写产生的问题以及解决方式
问题:
- 类模板中成员函数创建时机是在调用阶段,导致分文件编写时链接不到
解决:
main.cpp :
#include <iostream>
#include "person.cpp"
using namespace std;
void test01(){
Person<string, int>p("Jerry", 18);
p.showPerson();
}
int main(){
test01();
system("pause");
return 0;
}
person.h :
#pragma once
#include <iostream>
#include <string>
using namespace std;
template<class T1, class T2>
class Person{
public:
Person(T1 name, T2 age);
void showPerson();
T1 m_Name;
T2 m_Age;
};
person.cpp :
#include "person.h"
template<class T1, class T2>
Person<T1, T2>::Person(T1 name, T2 age){
this->m_Name = name;
this->m_Age = age;
}
template<class T1, class T2>
void Person<T1, T2>::showPerson(){
cout << "姓名: " << this->m_Name << " 年龄:" << this->m_Age << endl;
}
- 解决方式2:将声明和实现写到同一个文件中,并更改后缀名为
.hpp ,hpp 是约定的名称,并不是强制
main.cpp :
#include <iostream>
#include "person.hpp"
using namespace std;
void test01(){
Person<string, int>p("Jerry", 18);
p.showPerson();
}
int main(){
test01();
system("pause");
return 0;
}
person.hpp :
#pragma once
#include <iostream>
#include <string>
using namespace std;
template<class T1, class T2>
class Person{
public:
Person(T1 name, T2 age);
void showPerson();
T1 m_Name;
T2 m_Age;
};
template<class T1, class T2>
Person<T1, T2>::Person(T1 name, T2 age){
this->m_Name = name;
this->m_Age = age;
}
template<class T1, class T2>
void Person<T1, T2>::showPerson(){
cout << "姓名: " << this->m_Name << " 年龄:" << this->m_Age << endl;
}
总结:主流的解决方式是第二种,将类模板成员函数写到一起,并将后缀名改为.hpp
1.3.8 类模板与友元
- 学习目标:
- 全局函数类内实现:直接在类内声明友元即可
#include <iostream>
#include <string>
using namespace std;
template<class T1, class T2>
class Person{
friend void printPerson(Person<T1, T2>p){
cout << " 姓名: " << p.m_Name << " 年龄: " << p.m_Age << endl;
}
public:
Person(T1 name, T2 age){
this->m_Name = name;
this->m_Age = age;
}
T1 m_Name;
T2 m_Age;
};
void test01(){
Person<string, int>p("Tom", 20);
printPerson(p);
}
int main(){
test01();
system("pause");
return 0;
}
- 全局函数类外实现:需要提前让编译器知道全局函数的存在
#include <iostream>
#include <string>
using namespace std;
template<class T1, class T2>
class Person;
template<class T1, class T2>
void printPerson2(Person<T1, T2>p){
cout << " 姓名: " << p.m_Name << " 年龄: " << p.m_Age << endl;
}
template<class T1, class T2>
class Person{
friend void printPerson(Person<T1, T2>p){
cout << " 姓名: " << p.m_Name << " 年龄: " << p.m_Age << endl;
}
friend void printPerson2<>(Person<T1, T2>p);
public:
Person(T1 name, T2 age){
this->m_Name = name;
this->m_Age = age;
}
T1 m_Name;
T2 m_Age;
};
void test02(){
Person<string, int>p("Tom", 20);
printPerson2(p);
}
int main(){
test02();
system("pause");
return 0;
}
总结:建议全局函数做类内实现,用法简单,而且编译器可以直接识别
1.3.9 类模板案例
案例描述:实现一个通用的数组类,要求(7个)如下:
- 可以对内置数据类型以及自定义数据类型的数据进行存储(运用本篇普通函数与函数模板调用规则的内容/1.2.5)
- 将数组中的数据存储到堆区
- 构造函数中可以传入数组的容量
- 提供对应的拷贝构造函数以及
operator= 防止浅拷贝问题(核心编程篇:运算符重载/4.5.4) - 提供尾插法和尾删法对数组中的数据进行增加和删除(提供一些接口)
- 可以通过下标的方式访问数组中的元素
- 可以获取数组中当前元素个数和数组的容量 (容量私有化,只提供对外接口)
思路图: 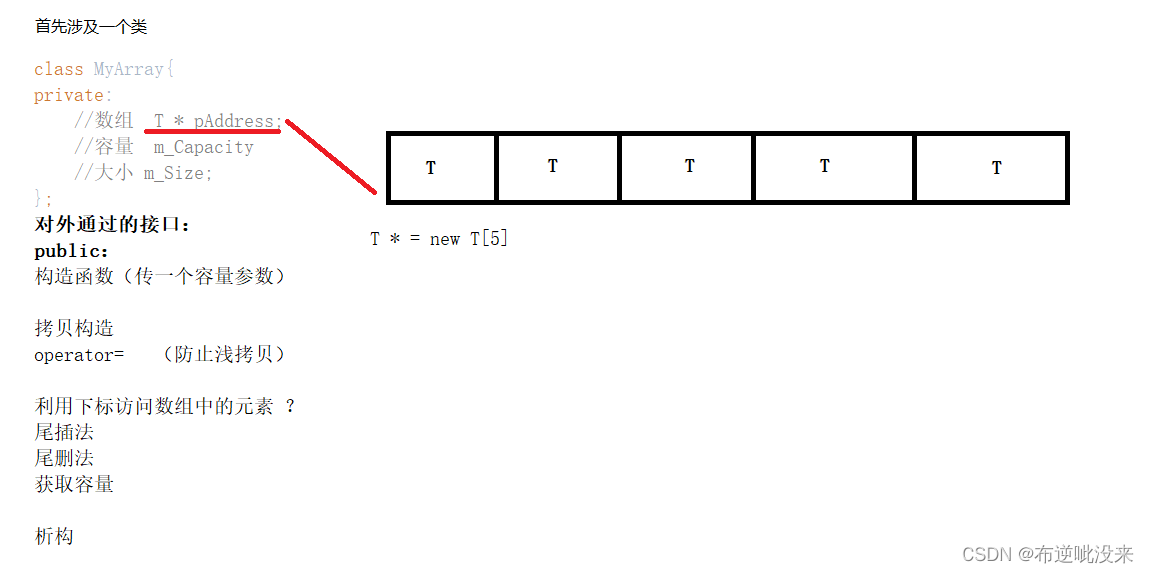
先声明数组类和各种构造、析构函数,保证基本的代码运行正常。
MyArray.hpp :
#pragma once
#include <iostream>
using namespace std;
template<class T>
class MyArray{
public:
MyArray(int capacity){
cout << "MyArray的有参构造调用" << endl;
this->m_Capacity = capacity;
this->m_Size = 0;
this->pAddress = new T[this->m_Capacity];
}
MyArray(const MyArray& arr){
cout << "MyArray的拷贝构造调用" << endl;
this->m_Capacity = arr.m_Capacity;
this->m_Size = arr.m_Size;
this->pAddress = new T[arr.m_Capacity];
for(int i = 0; i < this->m_Size; i++){
this->pAddress[i] = arr.pAddress[i];
}
}
MyArray& operator=(const MyArray& arr){
cout << "MyArray的operator=调用" << endl;
if(this->pAddress!=NULL){
delete[] this->pAddress;
this->pAddress = NULL;
this->m_Capacity = 0;
this->m_Size = 0;
}
this->m_Capacity = arr.m_Capacity;
this->m_Size = arr.m_Size;
this->pAddress = new T[arr.m_Capacity];
for(int i = 0; i < this->m_Size; i++){
this->pAddress[i] = arr.pAddress[i];
}
return *this;
}
~MyArray(){
if(this->pAddress != NULL){
cout << "MyArray的析构函数调用" << endl;
delete[] this->pAddress;
this->pAddress = NULL;
}
}
private:
T * pAddress;
int m_Capacity;
int m_Size;
};
main.cpp :
#include <iostream>
#include "MyArray.hpp"
void test01(){
MyArray<int>arr1(5);
MyArray<int>arr2(arr1);
MyArray<int>arr3(100);
arr3 = arr1;
}
int main() {
test01();
system("pause");
return 0;
}
测试结果(成功): 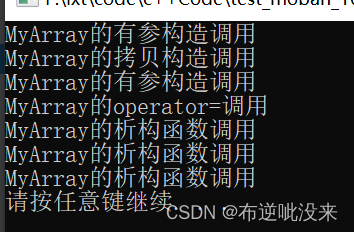
最后实现其余具体功能,并进行测试(增删、通过下标访问、获取当前大小与容量):
MyArray.hpp :
#pragma once
#include <iostream>
using namespace std;
template<class T>
class MyArray{
public:
MyArray(int capacity){
this->m_Capacity = capacity;
this->m_Size = 0;
this->pAddress = new T[this->m_Capacity];
}
MyArray(const MyArray& arr){
this->m_Capacity = arr.m_Capacity;
this->m_Size = arr.m_Size;
this->pAddress = new T[arr.m_Capacity];
for(int i = 0; i < this->m_Size; i++){
this->pAddress[i] = arr.pAddress[i];
}
}
MyArray& operator=(const MyArray& arr){
if(this->pAddress!=NULL){
delete[] this->pAddress;
this->pAddress = NULL;
this->m_Capacity = 0;
this->m_Size = 0;
}
this->m_Capacity = arr.m_Capacity;
this->m_Size = arr.m_Size;
this->pAddress = new T[arr.m_Capacity];
for(int i = 0; i < this->m_Size; i++){
this->pAddress[i] = arr.pAddress[i];
}
return *this;
}
void Push_Back(const T & val){
if(this->m_Capacity == this->m_Size){
cout << "Sorry, the arr was full!" << endl;
return;
}
this->pAddress[this->m_Size] = val;
this->m_Size++;
}
void Pop_Back(){
if(this->m_Size == 0){
cout << "the size was zero!" << endl;
return;
}
this->m_Size--;
}
T& operator[](int index){
return this->pAddress[index];
}
int getCapacity(){
return this->m_Capacity;
}
int getSize(){
return this->m_Size;
}
~MyArray(){
if(this->pAddress != NULL){
delete[] this->pAddress;
this->pAddress = NULL;
}
}
private:
T * pAddress;
int m_Capacity;
int m_Size;
};
main.cpp :
#include <iostream>
#include <string>
#include "MyArray.hpp"
void printArray(MyArray<int>& arr){
for(int i = 0; i < arr.getSize(); i++){
cout << arr[i] << endl;
}
}
void test01(){
MyArray<int>arr1(5);
for(int i = 0; i < 5; i++){
arr1.Push_Back(i);
}
cout << "arr1的打印输出为:" << endl;
printArray(arr1);
cout << "arr1的容量为:" << arr1.getCapacity() << endl;
cout << "arr1的大小为:" << arr1.getSize() << endl;
MyArray<int>arr2(arr1);
cout << "arr2的打印输出为:" << endl;
arr2.Pop_Back();
cout << "arr2尾删后" << endl;
cout << "arr2的容量为:" << arr2.getCapacity() << endl;
cout << "arr2的大小为:" << arr2.getSize() << endl;
}
class Person{
public:
Person(){}
Person(string name, int age){
this->m_Name = name;
this->m_Age = age;
}
string m_Name;
int m_Age;
};
void printPersonArray(MyArray<Person>& arr){
for(int i = 0; i < arr.getSize(); i++){
cout << "姓名:" << arr[i].m_Name << " 年龄:" << arr[i].m_Age << endl;
}
}
void test02(){
MyArray<Person>arr(10);
Person p1("mary", 23);
Person p2("fire", 34);
Person p3("lus", 67);
Person p4("miss", 45);
Person p5("li", 23);
arr.Push_Back(p1);
arr.Push_Back(p2);
arr.Push_Back(p3);
arr.Push_Back(p4);
arr.Push_Back(p5);
printPersonArray(arr);
cout << "arr的容量为:" << arr.getCapacity() << endl;
cout << "arr的大小为:" << arr.getSize() << endl;
}
int main() {
test02();
system("pause");
return 0;
}
测试运行结果: 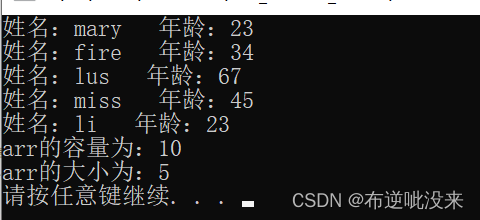
至此,模板学习告一段落。 能够将前面所学的运算符重载、函数重载、深浅拷贝等等知识和当前知识(各种模板的定义声明与调用、以及各种情况下的调用规则)合理运用,综合掌握,这是非常重要的一件事。
|