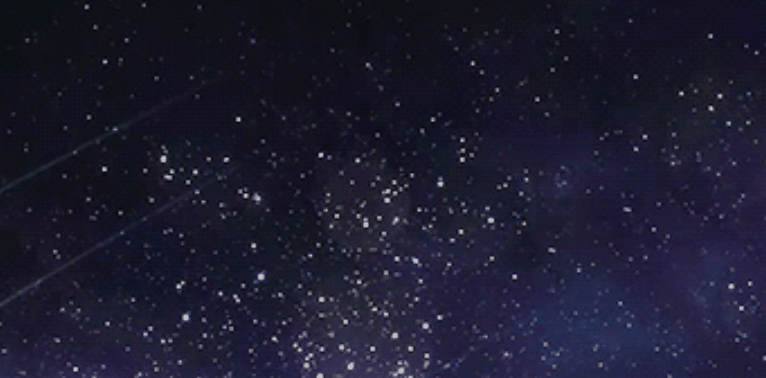 每一个不曾起舞的日子都是对生命的辜负
本节目标
熟练掌握各种string类的函数并将其应用。
注:本文参考以下两篇优秀文章,将其结合并加上额外的知识用自己的理解进行描述:
C++之string类型详解
C++string类型详解
1. string类概览
1.1 string的由来
之所以抛弃char*的字符串而选用C++标准程序库中的string类,是因为他和前者比较起来,不必担心内存是否足够、字符串长度等等,而且作为一个泛型类出现,他集成的操作函数足以完成我们大多数情况下(甚至是100%)的需要。我们可以用 = 进行赋值操作,== 进行比较,+ 做串联(是不是很简单?)。我们尽可以把它看成是C++的基本数据类型。
C++中对于string的定义为:typedef basic_string<char> string ; 也就是说C++中的string类是一个泛型类,由模板而实例化的一个标准类,本质上不是一个标准数据类型。
在我们的程序中使用string类型,我们必须包含头文件 。如下: #include<string> using namespace std; 此语句必不可少,否则有的编译器无法识别
1.2 string函数列表
begin | 得到指向字符串开头的Iterator |
---|
end | 得到指向字符串结尾的Iterator | rbegin | 得到指向反向字符串开头的Iterator | rend | 得到指向反向字符串结尾的Iterator | size | 得到字符串的大小 | length | 和size函数功能相同 | max_size | 字符串可能的最大大小 | capacity | 在不重新分配内存的情况下,字符串可能的大小 | empty | 判断是否为空 | operator[] | 取第几个元素,相当于数组 | c_str | 取得C风格的const char* 字符串 | data | 取得字符串内容地址 | operator= | 赋值操作符 | reserve | 预留空间 | swap | 交换函数 | insert | 插入字符 | append | 追加字符 | push_back | 追加字符 | operator+= | += 操作符 | erase | 删除字符串 | clear | 清空字符容器中所有内容 | resize | 重新分配空间 | assign | 和赋值操作符一样 | replace | 替代 | copy | 字符串到空间 | find | 查找 | rfind | 反向查找 | find_first_of | 查找包含子串中的任何字符,返回第一个位置 | find_first_not_of | 查找不包含子串中的任何字符,返回第一个位置 | find_last_of | 查找包含子串中的任何字符,返回最后一个位置 | find_last_not_of | 查找不包含子串中的任何字符,返回最后一个位置 | substr | 得到字串 | compare | 比较字符串 | operator+ | 字符串链接 | operator== | 判断是否相等 | operator!= | 判断是否不等于 | operator<<//td> | 判断是否小于 | operator>> | 从输入流中读入字符串 | operator<< | 字符串写入输出流 | getline | 从输入流中读入一行 |
对于这些函数,我们在下面将会逐个讲解:
2.string常用接口
对于这些用法来说,下面传入的参数个数在同一个用法中都有所差异,这是因为每一个成员函数都支持了重载。
在介绍下述接口之前,需要知道的是,由于每一个函数都有重载,因此有的有const的重载,有的没有,其实这是以函数的需求从而判断其有无const类型的重载函数,这里提前进行总结:
- 只读功能函数 const版本
- 只写功能函数 非const版本
- 读写功能的函数 const+非const版本
1. 初始化
初始化有两种方式,其中使用等号的是拷贝初始化,不使用等号的是直接初始化。(注释后面是打印的结果)
但对于使用等号的和str(str1),即一个变量通过另一变量初始化的,都是拷贝构造。(深拷贝)
string str1 = "hello world";
string str2("hello world");
string str3 = str1;
string str4(str2);
string str5(10,'h');
string str6 = string(10,'h');
string str7(str1,6);
string str_7(str1,6,3);
string str8 = string(str1,6);
string str9(str1,0,5);
string str10 = string(str1,0,5);
char c[] = "hello world";
string str11(c,5);
string str12 = string(c,5);
2. string::npos
我们观察一下上面str7与str_7的区别,想必大家已经看出,这里的str7和str_7是同一个重载函数,并且这个函数具有缺省值,当我们不传入最后一个参数时,其就会一直拷贝到字符串的末尾为止。那这个缺省参数是什么呢?我们查阅文档得知,是npos: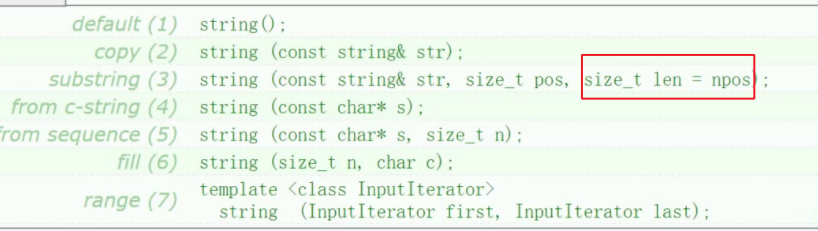 我们发现,npos的值规定为-1,但实际上因为是size_t类型,所以这是一个无符号的数字,即此-1并不是十进制的-1,而是:4294967295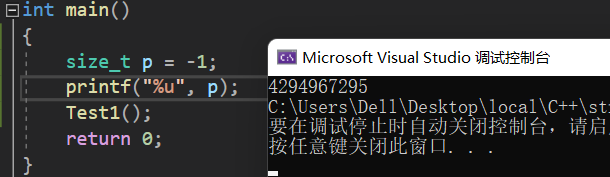
因此,此位置重载在不输入数值时默认为此值,也就能够遍历到字符串的末尾了。
此外,对于内置的string类,是支持运算符重载的,因此同样的也支持流的相关重载,即cin、cout:
string str1 = "hello world";
cout << "str1 = " << str1;
所以我们可以把string这个内部类当成内置类型使用。
3. c_str()

对于string类来说,其内部有这么一个成员变量,c_str,正如此图,c_str本身和指向的值均不能改变,返回值是char* 实际上返回的就是string类中的内容的地址,也就是字符串的地址。
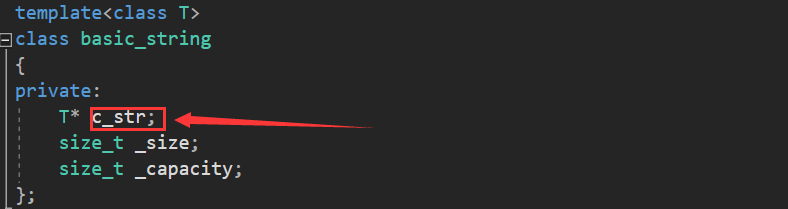
那c_str有什么作用呢?事实上对于一些线程,网络,Linux内核等都是通过C实现的,因此c_str很好的充当了一个C++中string与C之间的互通,因为我们知道,对于string定义的变量名,不是内部字符串的地址,因此就出现了c_str()返回内容的地址,从而解决这个问题。
演示:
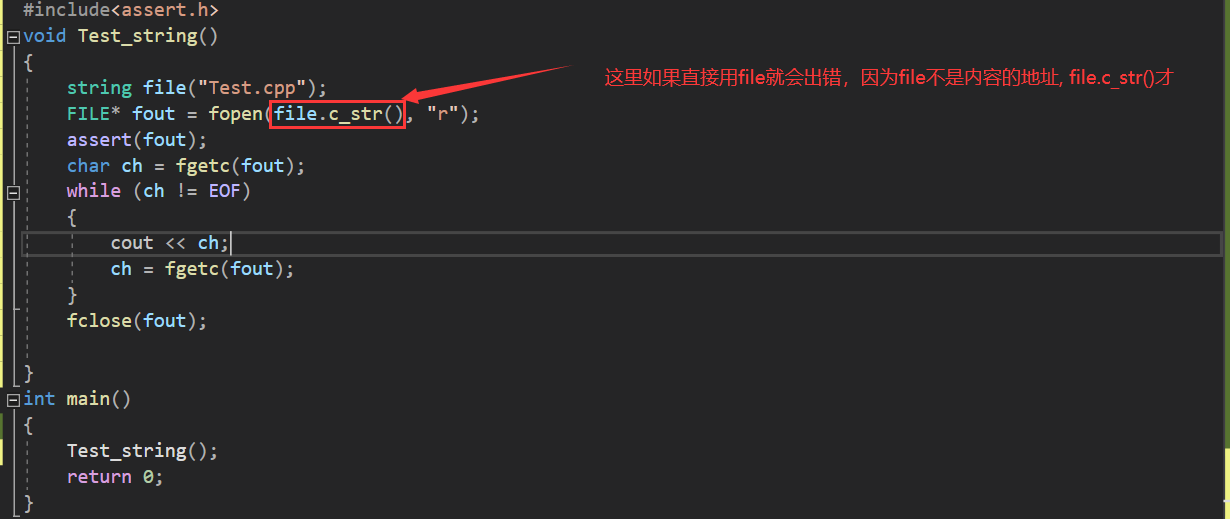
结果:
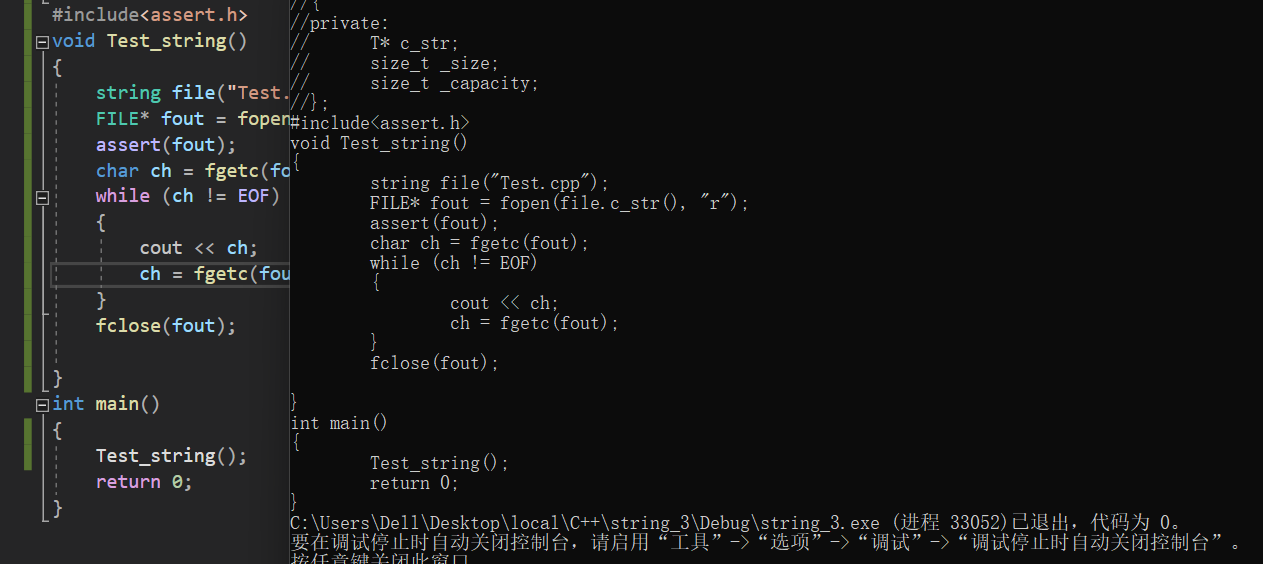
这样就将其内容正确的打开了。
4. 获取长度(length、size)
length()函数与size()函数均可获取字符串长度。但除了string,其他类型就只有size()。
string str = "hello world";
cout << str.length() << str.size();
当str.length()与其他类型比较时,建议先强制转换为该类型,否则会意想之外的错误。 比如:-1 > str.length() 返回 true。
5. 容量(size、capacity)
对于size和capacity来说,大家在学了顺序表之后并不陌生,size是实际长度,而capacity代表着容量的大小,对于string类来说,其也具有这样的成员变量(对应值C语言顺序表中结构体内部的的size、capacity),而这里的扩容规则在每一个平台也是不一样的,比如我的linux和vs2019二者之间就有很大的区别,不过我们并不需要关心他,因为string作为内部类,其扩容的机制已经被写在该类之中。
string str;
str += "hello world hello world";


可以看出,其大概是是扩容了二倍的大小。
此外,还有其他函数也属于容量的范畴:

resize可以改变成员的size()的大小。
reserve可以改变成员的capacity()的大小。
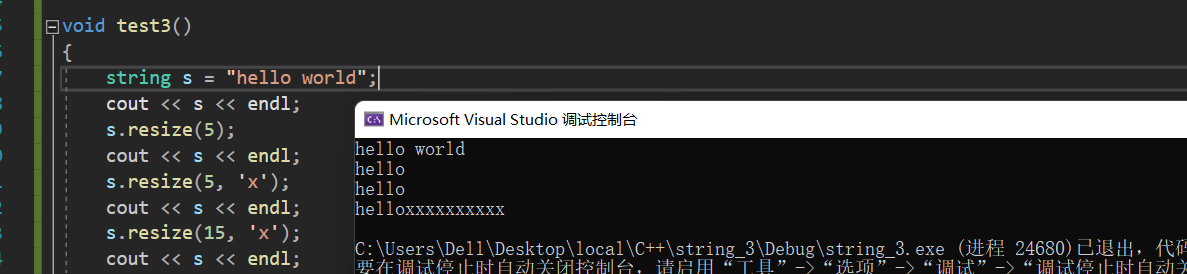
而对于max_size(),这里我们只打印结果就知道其具体的含义:
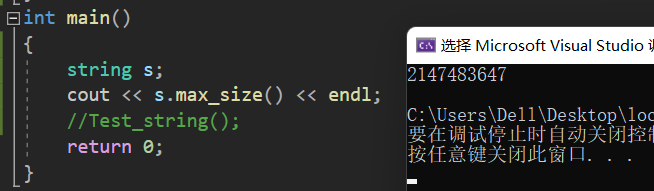
6. 插入(insert)
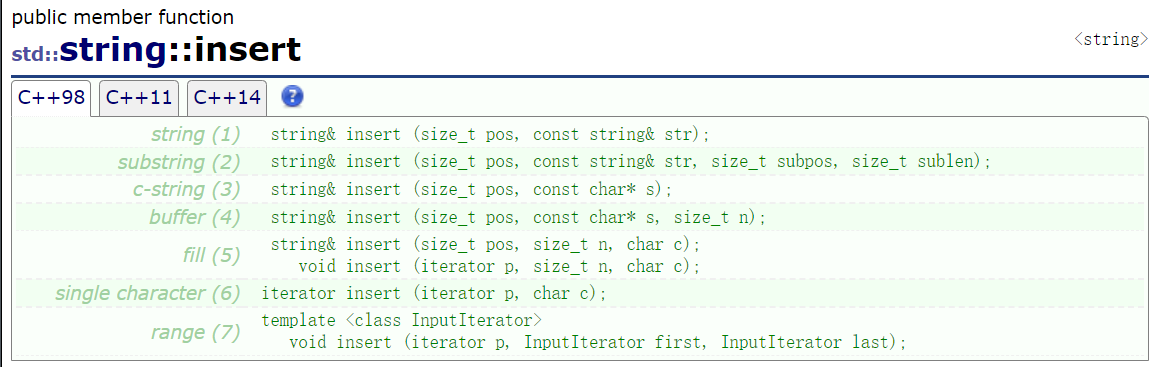
string str = "hello world";
string str2 = "hard ";
string str3 = "it is so happy wow";
str.insert(6,4,'z');
str.insert(6,str2);
str.insert(6,str3,6,9);
str.insert(6,"it is so happy wow",6);
虽然有这样的接口,但是我们知道对于类似于顺序表的结构来说,这样的插入,实际上底层都会将后面的数据进行挪动,因此效率难免会低一些。
7. 替换(replace)
替换与插入对应,对比理解更为简单。
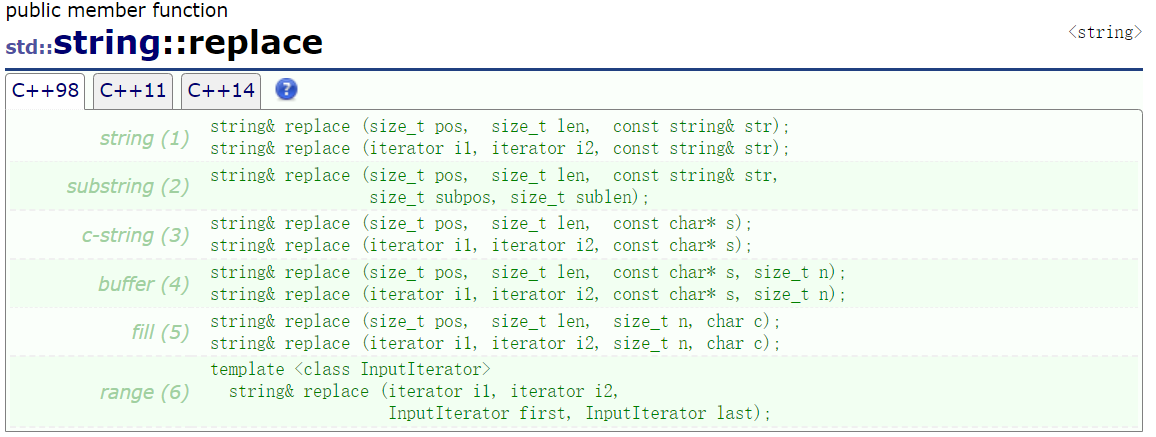
string str = "hello world";
string str2 = "hard ";
string str3 = "it is so happy wow";
str.replace(0,6,4,'z');
str.replace(0,6,str2);
str.replace(0,6,str3,6,9);
str.replace(0,6,"it is so happy wow",6);
8. 添加(append、push_back、+=)
append函数用在字符串的末尾添加字符和字符串。(同样与插入、替换对应理解)而push_back只适用于添加单个字符,此外,对于添加来说,如果是在末尾添加字符或者字符串我们仍然可以像初始化中的拷贝构造一样,即通过+=进行添加。
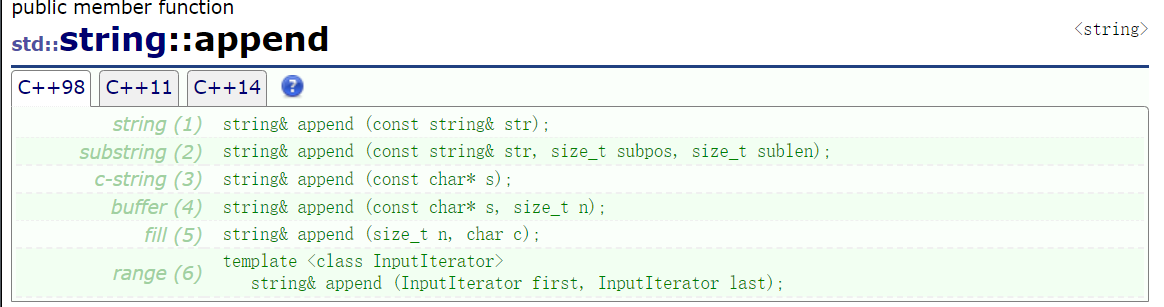


string str = "hello world";
string str2 = "hard ";
string str3 = "it is so happy wow";
string str4 = "hello world"
str.append(4,'z');
str.append(str2);
str.append(str3,6,9);
str.append("it is so happy wow",6);
str4.push_back('c');
str4 += 'c';
st4 += "cdef";
9. 赋值(assign)
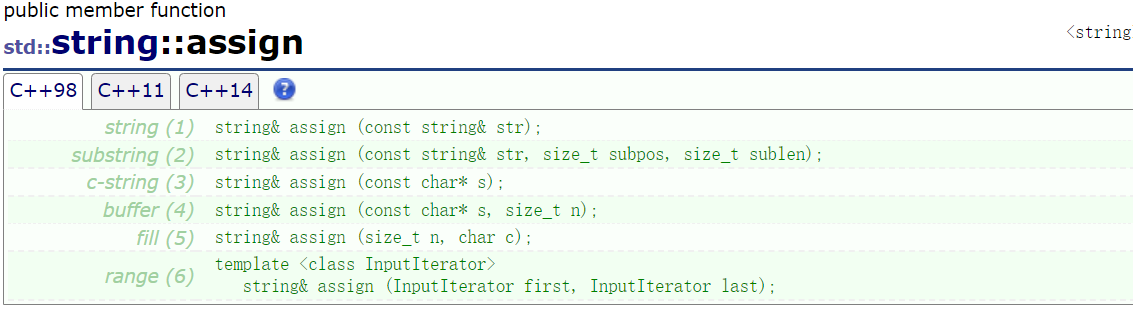
赋值也是一种初始化方法,与插入、替换、添加对应理解较为简单。
string str;
string temp = "welcome to my blog";
str.assign(10,'h');
str.assign(temp);
str.assign(temp,3,7);
str.assign("welcome to my blog",7);
10. 删除与判空(erase、clear、empty)
对于clear,其具有清空的功能,也就是从任意的字符串使用clear,都会将其清空至空字符串;对于erase来说,其可以指定的删除,也就是说可以删除一部分,也可以删除全部。
string str = "welcome to my blog";
str.erase(11,3);
str.clear();
str.empty();
clear()实际上不会将capacity的空间也清除掉,即size会改变,但capacity并不会改变。
对于empty来说,实际上是判断是否为空的函数,是空就返回1,不是空就返回0,因此empty是根据size()是否为0判断的。
11. 剪切(substr)

string str = "The apple thinks apple is delicious";
string s1 = str.substr(4, 5);
string s2 = str.substr(17);
string s3 = str.substr(4, 100);
string s4 = str.substr(4, string::npos);
12. 比较(compare)
两个字符串自左向右逐个字符相比(按ASCII值大小相比较),直到出现不同的字符或遇’\0’为止。若是遇到‘\0’结束比较,则长的子串大于短的子串,如:“9856” > “985”。如果两个字符串相等,那么返回0,调用对象大于参数返回1,小于返回-1。
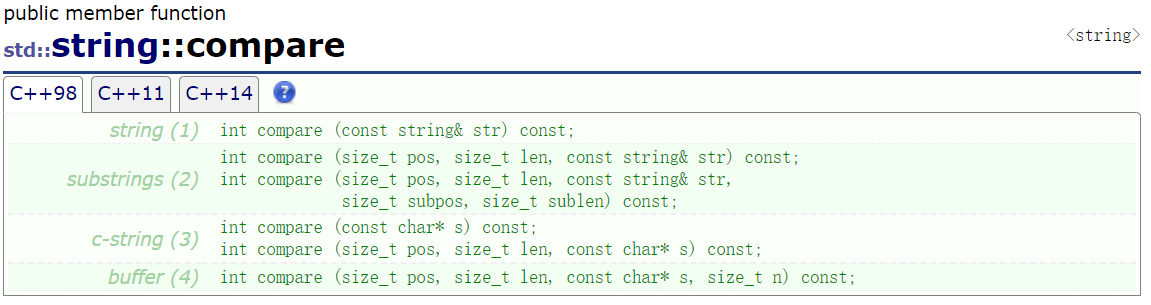
string str1 = "small leaf";
string str2 = "big leaf";
cout << str1.compare(str2);
cout << str1.compare(2, 7, str2);
cout << str1.compare(6, 4, str2, 4, 4);
cout << str1.compare(6, 4, "big leaf", 4);
13. 交换(swap)
string str1 = "small leaf";
string str2 = "big leaf";
swap(str1,str2);
swap(str1[0],str1[1]);
14. 反转(reverse)
反转字符串。
string str = "abcdefghijklmn";
string::iterator it1 = str.begin();
string::iterator it2 = str.end();
reverse(str.begin(), str.end());
reverse(it1, it2);
对于str.begin()和str.end()类型,其返回值可以看成指针,但实际上并不是指针,因此我们用char* 定义的it1和it2是不对的,其在不同的平台上有不同的含义,事实上其有着迭代器的作用。(下面讲解迭代器的使用)
15. 迭代器(iterator)
迭代器实际上是一个像指针一样的东西,这是对行为来说的。需要注意的是,这里不能用char* ,虽然对于vs这个平台可以使用char* ,但难保其他的平台是char* ,因此迭代器的底层不一定是char* ,这在模拟实现中将会详细介绍。(对于迭代器来说,只要会用string类,那么vector、list等容器与其方法是一样的)
15.1 正向迭代器
string s1("1234");
string::iterator it1 = s1.begin();
while (it1 != s1.end())
{
*it1 += 1;
++it1;
}
it1 = s1.begin();
while (it1 != s1.end())
{
cout << *it1 << " ";
++it1;
}
15.2 反向迭代器
当然,还有反向迭代器,其访问顺序与默认的迭代器相反。
string s1("1234");
string::reverse_iterator rit = s1.rbegin();
while (rit != s1.rend())
{
cout << *rit << " ";
++rit;
}
此外,我们发现此定义名过长,因此我们也可以用auto去定义变量rit接收rbegin()。
15.3 const迭代器
当我们需要只读的时候,为了避免改变其中的值,在迭代器使用时我们就会选择const迭代器,顾名思义const迭代器能够保护迭代指向的变量不被改变,那我们实际来看一下const迭代器如何使用:
void Print(const string& s)
{
string::const_iterator it = s.begin();
while (it != s.end())
{
cout << *it << " ";
it++;
}
cout << endl;
string::const_reverse_iterator rit = s.rbegin();
while (rit != s.rend())
{
cout << *rit << " ";
rit++;
}
cout << endl;
}
int main()
{
string s1("1234");
Print(s1);
return 0;
}
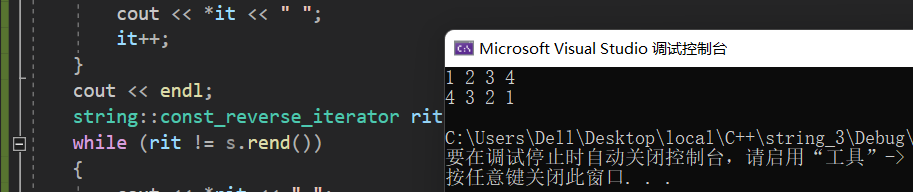
总结:迭代器经过上述的描述,一共有四种,即正向、反向、正向const、反向const,其不能混合定义,否则会出现错误。
16. 搜索与查找(find等函数)
16.1 find()函数
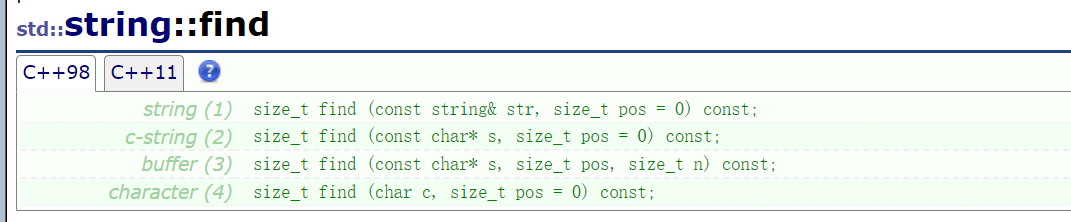
string str = "The apple thinks apple is delicious";
string key = "apple";
int pos1 = str.find(key);
int pos2 = str.find(key, 10);
int pos3 = str.find("delete", 0, 2);
int pos4 = str.find('s', 0);
16.2 rfind函数
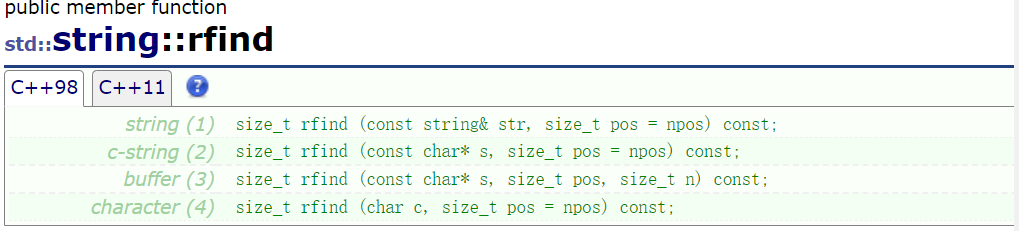
rfind与find的区别就是:find是从左往右找(即从前往后),而rfind是从后往前找。
string str = "The apple thinks apple is delicious";
string key = "apple";
int pos5 = str.rfind(key);
int pos6 = str.rfind(key, 16);
int pos7 = str.rfind("apple", 40, 2);
int pos8 = str.rfind('s', 30);
16.3 find_xxx_of()函数(功能强大,但不常用)
xxx是需要确定的名字,因此下面将介绍这种类型的查找函数:(4个)
- find_first_of
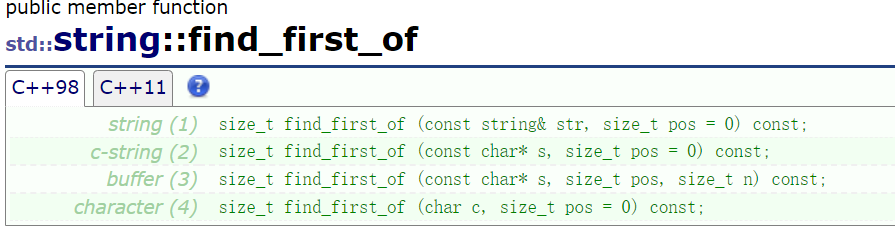
int pos1 = str.find_first_of(key);
int pos2 = str.find_first_of(key, 10);
int pos3 = str.find_first_of("aeiou", 7, 2);
int pos4 = str.find_first_of('r', 0);
最好的解释就是举例子: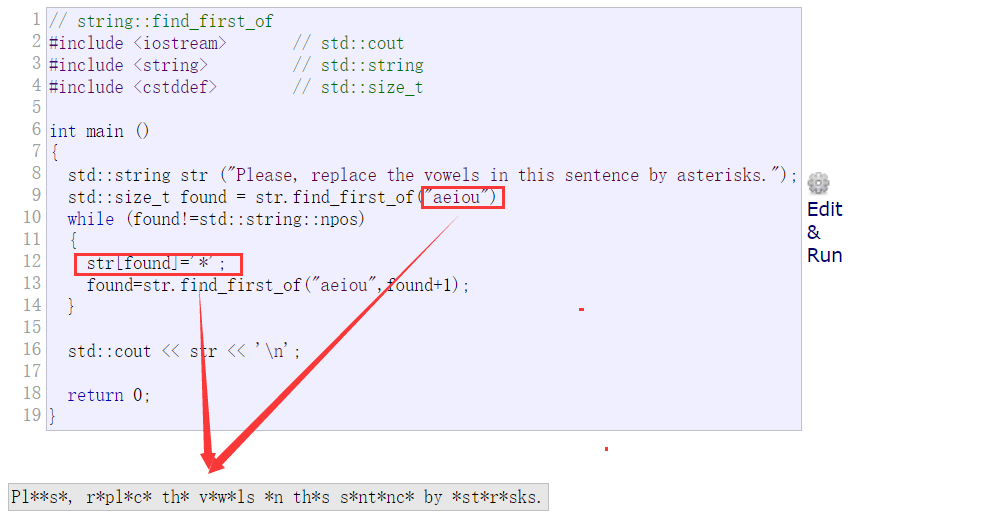
即找到每个str中的字符进行替换,与find和rfind的区别是:此查找找的是字符串中的所有字符,而不是字符串。下面的几个同样如此:
- find_last_of
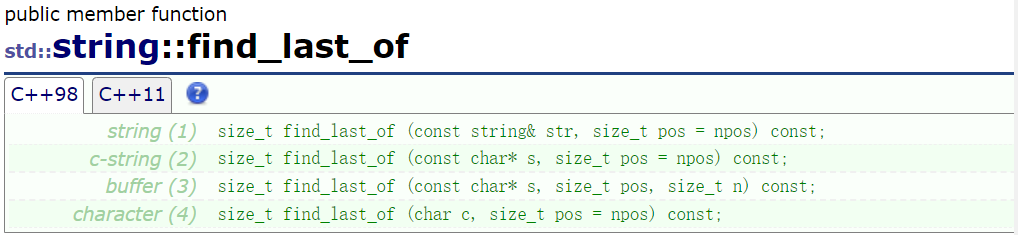
int pos1 = str.find_last_of(key);
int pos2 = str.find_last_of(key, 15);
int pos3 = str.find_last_of("aeiou", 20, 2);
int pos4 = str.find_last_of('r', 30);
- find_first_not_of
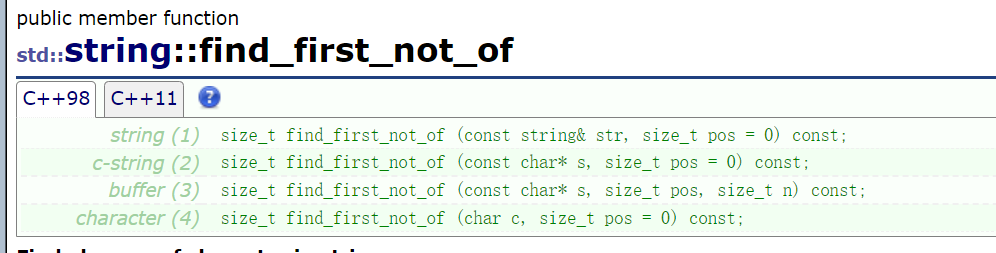
int pos1 = str.find_first_not_of(key);
int pos2 = str.find_first_not_of(key, 10);
int pos3 = str.find_first_not_of("aeiou", 7, 2);
int pos4 = str.find_first_not_of('r', 0);
- find_last_not_of
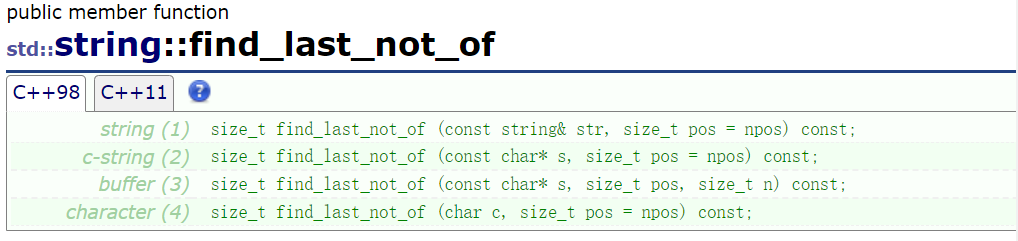
int pos1 = str.find_last_not_of(key);
int pos2 = str.find_last_not_of(key, 15);
int pos3 = str.find_last_not_of("aeiou", 20, 2);
int pos4 = str.find_last_not_of('r', 30);
3. string类的应用
3.1 三种遍历方式
int main()
{
string s1("1234");
for (size_t i = 0; i < s1.size(); ++i)
{
s1[i]++;
}
cout << s1 << endl;
for (auto& ch : s1)
{
ch--;
}
cout << s1 << endl;
size_t begin = 0, end = s1.size() - 1;
while (begin < end)
{
swap(s1[begin++], s1[end--]);
}
cout << s1 << endl;
cout << s1 << endl;
string::iterator it1 = s1.begin();
while (it1 != s1.end())
{
*it1 += 1;
++it1;
}
it1 = s1.begin();
while (it1 != s1.end())
{
cout << *it1 << " ";
++it1;
}
cout << endl;
}
3.2 替换空格
题目:替换空格
解法1:
对于这道题,如果按照c语言的方式会很麻烦,但是通过C++string中的函数,我们可以先find,再replace:
class Solution {
public:
string replaceSpace(string s) {
size_t pos = s.find(' ');
while(pos != string::npos)
{
s.replace(pos, 1, "%20");
pos = s.find(' ',pos+3);
}
return s;
}
};
+3实际上就是对代码的优化,因为%20就对应了3个位置,因此+3跳过这三个字符查找的效率更快。
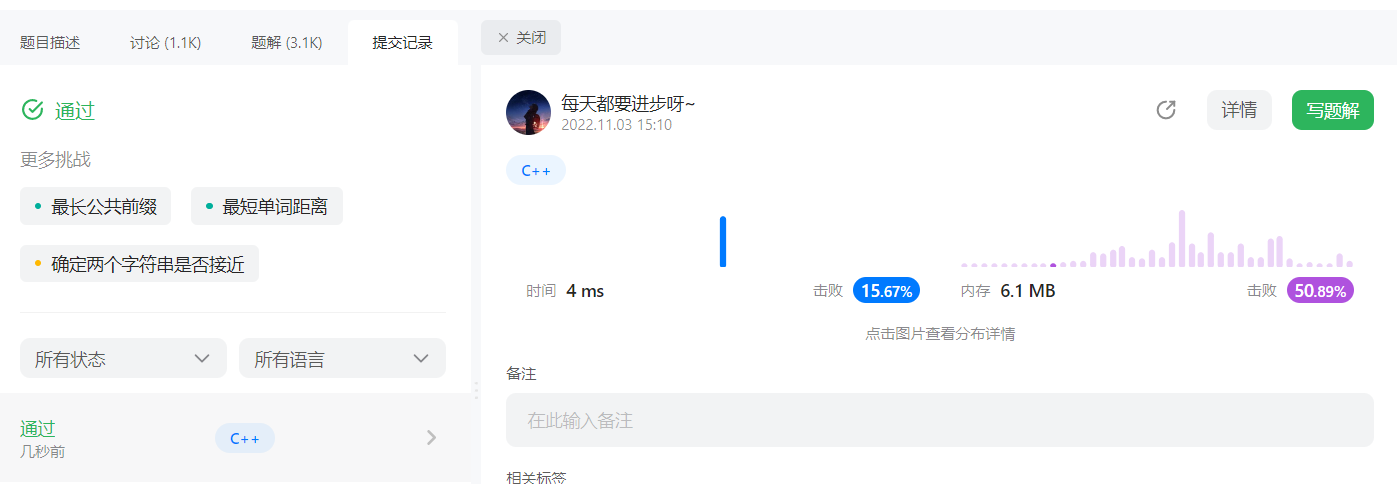
解法2: 我们还可以新建一个string的空字符串,遍历传入的string s如果没有碰到空格就+=该字符,碰到了空格就+=%20
class Solution {
public:
string replaceSpace(string s) {
string ret;
ret.reserve(s.size()*3);
for(auto ch : s)
{
if(ch != ' ')
{
ret += ch;
}
else
{
ret += "%20";
}
}
return ret;
}
};
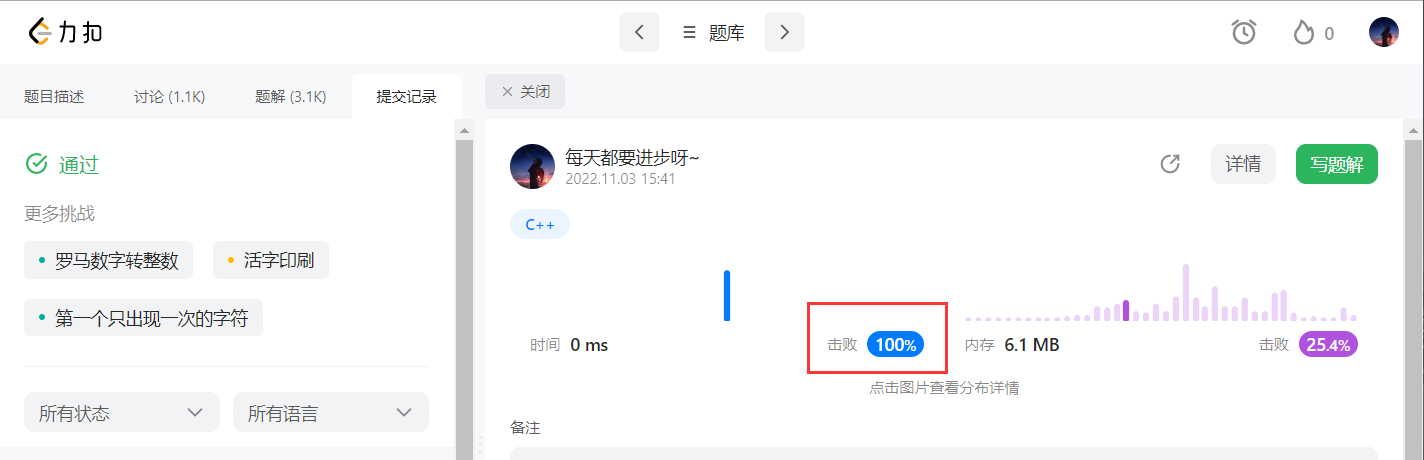
ret.reserve(s.size()*3); 是面对全是空格的情况,这样的极端情况就可以作为扩容的标准。这样我们发现,以空间换时间的做法就可以有效的提高效率。
3.3 通过find取后缀
如果我们想确定一个文件是什么类型,我们需要知道其后缀,最后一个. 后面的名字就是其后缀,那我们就可以先通过rfind()找到最后一个.的位置,再通过substr拷贝下来,这样就获得了相应文件的后缀了。(找最后一个. 是由于在Linux下的文件可能存在类似于test.cpp.zip.tar的文件名,而其文件类型其实是tar)
void test_string11()
{
string file;
cin >> file;
size_t pos = file.rfind('.');
if (pos != string::npos)
{
string suffix = file.substr(pos);
cout << suffix << endl;
}
}
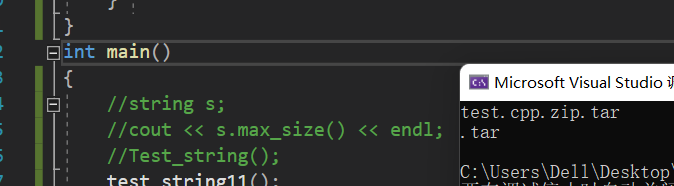
3.4 getline的应用
将getline放在这里是因为这里直接利用会更具体的描述。题目:
HJ1 字符串最后一个单词的长度
这道题如果我们直接用scanf或者cin的话,对于输入hello nowcoder来说,实际上输入的只有hello,因为到空格就截止了,因此这里就需要了getline输入,getline的作用是到换行符时才结束输入。那我们看看代码实现:
#include <iostream>
#include<string>
using namespace std;
int main()
{
string s;
getline(cin , s);
size_t pos = s.rfind(' ');
cout << s.size() - pos - 1 <<endl;
return 0;
}
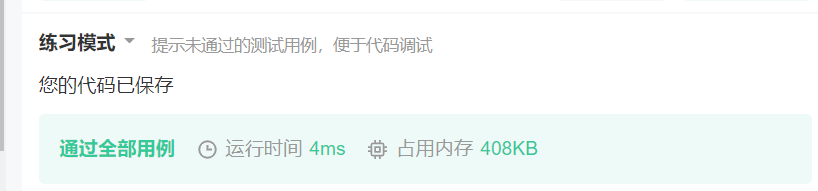
4. string总结
本文详细介绍了string中各个函数功能以及接口,通过这些的灵活运用,才能更好的掌握C++,而对于这些函数的底层实现,下一篇将用的模拟实现string加深对各种函数的理解。
|