JAVA小白编程题练习2
可能有很多刚入门的小白不知道自己如何能快速提升编程技巧与熟练度
其实大佬进阶之路只有一个~ 那就是疯狂码代码!!!实践出真知!!!
所以为了大家能够想练习的时候有素材,泡泡给大家整理了一些练习题
本帖是2帖,如果觉得难,可以先从1帖开始练习哦~
由于平时比较忙,所以我在不定时努力更新中,欢迎监督~
练习题:实现二分法查找/折半查找
注意:折半查找的前提:数据必须是有序的
package cn.cxy.exec;
import java.util.Arrays;
import java.util.Random;
import java.util.Scanner;
public class BinarySearch {
public static void main(String[] args) {
int[] a = suiJi();
Arrays.sort(a);
System.out.println(Arrays.toString(a));
while(true) {
System.out.print("查找的目标值:");
int t = new Scanner(System.in).nextInt();
int index = binarySearch(a, t);
System.out.println(index);
}
}
private static int[] suiJi() {
int n = 5+ new Random().nextInt(6);
int[] a = new int[n];
for (int i = 0; i < a.length; i++) {
a[i] = new Random().nextInt(100);
}
return a;
}
private static int binarySearch(int[] a, int t) {
int lo = 0;
int hi = a.length-1;
int mid;
while(lo <= hi) {
mid = (lo+hi) / 2;
if(a[mid] < t) {
lo = mid+1;
} else if(a[mid] > t) {
hi = mid-1;
} else {
return mid;
}
}
return -(lo+1);
}
}
练习题:二分法实现数据动态插入
注意:折半查找的前提:数据必须是有序的
package cn.cxy.exec;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Random;
import java.util.Scanner;
public class BinaryInsert {
public static void main(String[] args) {
ArrayList<Integer> list = new ArrayList<>();
System.out.println("回车继续");
while(true) {
new Scanner(System.in).nextLine();
int n = new Random().nextInt(100);
int index = Collections.binarySearch(list, n);
if(index < 0) {
index = (-index)-1;
}
list.add(index, n);
System.out.println(list.toString());
}
}
}
练习题 : 求数字阶乘(递归解法版)
需求:接收用户输入的数字,计算该数字的阶乘结果
已知:负数不可以有阶乘,0的阶乘结果是1,
5 ! = 5 x 4 x 3 x 2 x 1
package cn.cxy.design;
public class TestRecursion {
public static void main(String[] args) {
int result = f(15);
System.out.println(result);
}
public static int f(int n) {
if(n == 1) {
return 1;
}else {
return n*f(n-1);
}
}
}
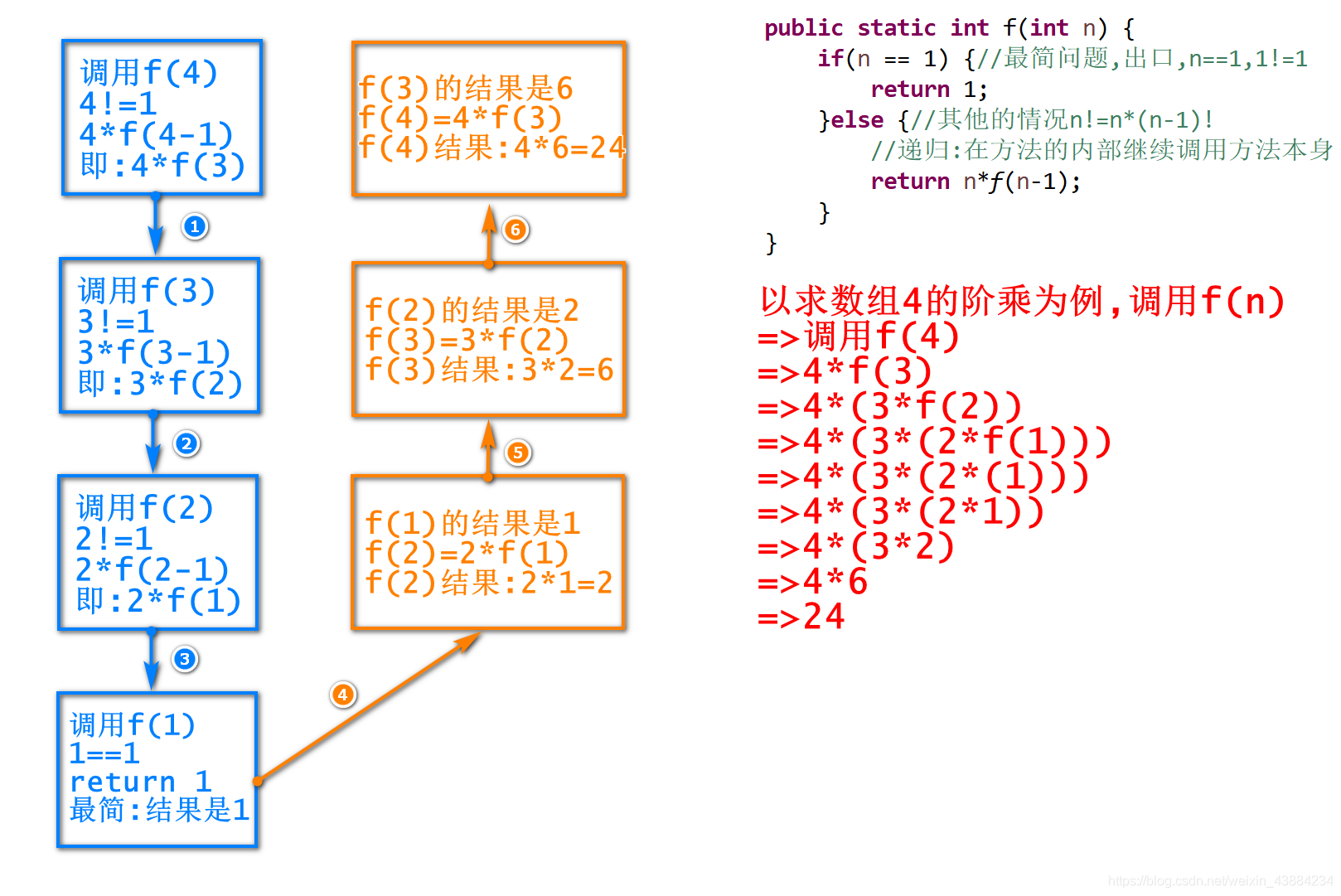
练习题 : 递归求目录总大小
需求:递归求目录的总大小 D:\ready,步骤分析如下: 1.列出文件夹中的所有资源–listFiles()–>File[] 2.判断,当前资源是文件还是文件夹–文件夹大小为0,文件大小需要累加 –是文件,求文件的字节量大小length(),累加就行 –是文件夹,继续列出文件夹下的所有资源–listFiles()–>File[] –判断,是文件,求文件的字节量大小length(),累加就行 –判断,是文件夹,再一次列出文件夹下的所有资源 –…重复操作 也就是说,规律就是:只要是文件夹,就需要重复步骤1 2
package cn.cxy.file;
import java.io.File;
public class FileSumRecursion {
public static void main(String[] args) {
File file = new File("D:\\ready");
long total = size(file);
System.out.println("文件夹的总大小为:"+total);
}
private static long size(File file) {
File[] fs = file.listFiles();
long sum = 0;
for(int i = 0;i < fs.length ; i++) {
File f = fs[i];
if(f.isFile()) {
sum += f.length();
}else if(f.isDirectory()) {
sum += size(f);
}
}
return sum;
}
}
练习题 : 递归删除文件夹
需求:递归删除文件夹 D:\ready\a 1.列出文件夹下的所有资源listFiles() 2.判断,当前资源是文件还是文件夹 –判断,是文件,直接删除delete() –判断,是文件夹,继续重复操作1 2 具体思路可以分为这么几步: 1.首先,我们需要指定一个根目录作为要删除的对象 2.列出文件夹下的所有资源listFiles(),并进行遍历 3.判断当前的资源,如果是文件,直接删除;如果是文件夹,则执行步骤2 4.将文件夹中的内容删除完毕后,删除文件夹本身
package cn.tedu.file;
import java.io.File;
public class TestFileDeleteRecursion {
public static void main(String[] args) {
File file = new File("D:\\ready\\a");
boolean result = del(file);
System.out.println("删除的结果为:"+result);
}
public static boolean del(File file) {
File[] fs = file.listFiles();
for (int i = 0; i < fs.length; i++) {
File f = fs[i];
if(f.isFile()) {
f.delete();
System.out.println(file.getName()+"文件删除成功!");
}else if(f.isDirectory()) {
del(f);
}
}
file.delete();
System.out.println(file.getName()+"文件夹删除成功!");
return true;
}
}
练习题 : 复制文件(字节流批量读取)
需求:接收用户目标文件的路径,复制目标文件到指定路径
import java.io.*;
import java.util.Scanner;
public class TestCopyFiles {
public static void main(String[] args) {
System.out.println("请输入源文件路径:");
String f = new Scanner(System.in).nextLine();
System.out.println("请输入目标文件的路径:");
String t = new Scanner(System.in).nextLine();
File from = new File(f);
File to = new File(t);
copy(from,to);
}
public static void copy(File from, File to) {
InputStream in = null;
OutputStream out = null;
try {
in = new BufferedInputStream(new FileInputStream(from));
out = new BufferedOutputStream(new FileOutputStream(to));
int b = 0;
byte[] bs = new byte[8*1024];
while( (b=in.read(bs)) != -1 ) {
out.write(bs);
}
System.out.println("恭喜您!文件复制成功!");
} catch (IOException e) {
System.out.println("很抱歉!文件复制失败!");
e.printStackTrace();
}finally {
try {
out.close();
} catch (IOException e) {
e.printStackTrace();
}
try {
in.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
练习题 : 回文问题
需求,如果一个用户输入的数据,从前到后或者从后到前读到的内容都是一样的,我们就称这种数据为"回文",比如123321 或者 12321 或者上海自来水来自海上等等
import java.util.Scanner;
public class TestNumber {
public static void main(String[] args) {
System.out.println("请输入一个字符串:");
String s = new Scanner(System.in).nextLine();
if(!stringJudge(s)){
System.out.println(s + "不是回文字符串");
}else{
System.out.println(s + "是回文字符串");
}
stringJudge2(s);
}
private static boolean stringJudge(String str) {
for (int i = 0; i < str.length() - i - 1; i++){
if(str.charAt(i) == str.charAt(str.length() - i - 1)){
continue;
}else{
return false;
}
}
return true;
}
private static void stringJudge2(String s) {
String s2 = new StringBuffer(s).reverse().toString();
if(s.compareTo(s2) == 0){
System.out.println(s + "是回文字符串");
}else{
System.out.println(s + "不是回文字符串");
}
}
}
练习题 : 手写数组列表
手写一个数组列表,实现基本的数据操作功能
数组列表类:
package cn.cxy.exec;
import java.util.Arrays;
public class ShuZuLieBiao<T> {
T[] a;
int index;
public ShuZuLieBiao() {
this(10);
}
public ShuZuLieBiao(int length) {
a = (T[]) new Object[length];
}
public void add(T t) {
queRenRongLiang();
a[index] = t;
index++;
}
private void queRenRongLiang() {
if(index<a.length) {
return;
}
int n = a.length + a.length/2 + 2;
a = Arrays.copyOf(a, n);
}
public T get(int i) {
jianChaFanWei(i);
return a[i];
}
private void jianChaFanWei(int i) {
if(i<0 || i>=index) {
throw new IndexOutOfBoundsException(
"下标:"+i);
}
}
public int size() {
return index;
}
public T remove(int i) {
jianChaFanWei(i);
T t = a[i];
System.arraycopy(a, i+1, a, i, index-i-1);
a[index-1] = null;
index--;
return t;
}
}
测试类:
package cn.cxy.exec;
public class TestSZLB {
public static void main(String[] args) {
ShuZuLieBiao<String> lb = new ShuZuLieBiao<>();
lb.add("aaa");
lb.add("vvv");
lb.add("ggg");
lb.add("rrr");
lb.add("qqq");
lb.add("uuu");
lb.add("aaa");
System.out.println(lb.size());
System.out.println(lb.get(0));
System.out.println(lb.get(lb.size()-1));
System.out.println(lb.remove(4));
for(int i=0;i<lb.size();i++) {
System.out.println(lb.get(i));
}
}
}
|