spring-boot重头再来 3
SpringData简介
对于数据访问层,无论是 SQL(关系型数据库) 还是 NOSQL(非关系型数据库),Spring Boot 底层都是采用 Spring Data 的方式进行统一处理。
Sping Data 官网:https://spring.io/projects/spring-data
数据库相关的启动器 :可以参考官方文档:
https://docs.spring.io/spring-boot/docs/2.2.5.RELEASE/reference/htmlsingle/#using-boot-starter
整合JDBC
创建用于测试数据源的项目
-
新建一个项目,引入三个依赖项
- SQL->JDBC API/关系型数据库->JDBC API
- SQL->MySQL Driver/关系型数据库->MySQL Driver
- Web->Spring Web
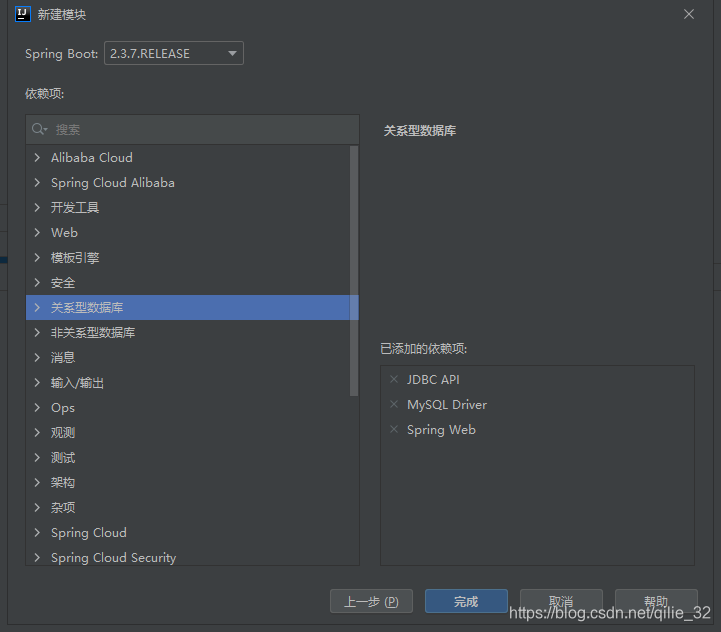
-
查看pom.xml可得引入了以下三个依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
-
编写yaml配置文件 spring:
datasource:
username: root
password: root
url: jdbc:mysql://localhost:3306/jdbczh?serverTimezone=Asia/Shanghai&useUnicode=true&characterEncoding=utf-8
driver-class-name: com.mysql.cj.jdbc.Driver
补充说明一下,url中的这个时区是必要的,如果没有这个时区会报错,一般值设置为以下两个
- Asia/Shanghai
- UTC
还有一个需要注意的是,新版的IDEA可能会帮你在peoperties中先写一大段数据库相关的东西,我们知道,同级下properties优先于yaml,如果咱们直接新建一个yaml并在其中写入内容的话,显然是不会采用yaml的配置的,所以咱们可以把内容写在properties中,也可以将properties删除 # 应用名称
spring.application.name=springboot-04
# 应用服务 WEB 访问端口
server.port=8080
# 数据库驱动:
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
# 数据源名称
spring.datasource.name=defaultDataSource
# 数据库连接地址
spring.datasource.url=jdbc:mysql://localhost:3306/blue?serverTimezone=UTC
# 数据库用户名&密码:
spring.datasource.username=***
spring.datasource.password=***
-
测试类测试 package com.example.springbootfour;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import javax.sql.DataSource;
import java.sql.Connection;
import java.sql.SQLException;
@SpringBootTest
class Springboot04ApplicationTests {
@Autowired
DataSource dataSource;
@Test
void contextLoads() throws SQLException {
System.out.println(dataSource.getClass());
Connection connection = dataSource.getConnection();
System.out.println(connection);
connection.close();
}
}
运行结果如下

可以得出数据源的类型是class com.zaxxer.hikari.HikariDataSource
这个在不同的版本有着不同的默认值,可见在当前版本(2.3.7 RELEASE)默认的是HikariDatSource,HikariDataSource 号称 Java WEB 当前速度最快的数据源,相比于传统的 C3P0 、DBCP、Tomcat jdbc 等连接池更加优秀,当然这个数据源类型实可以改变的,可以通过修改spring.datasource.type来改变
spring:
datasource:
type: com.zaxxer.hikari.HikariDataSource
JDBCTemplate
- 我们知道,原生的JDBC只有你获取到了Connection就可以通过连接来操作数据库
- Spring本身对原生的JDBC也进行了一定的封装,即JDBCemplate,所有的CRUD方法都已经封装于其中
- execute方法:可以用于执行任何SQL语句,一般用于执行DDL语句;
- update方法及batchUpdate方法:
- update方法用于执行新增、修改、删除等语句;
- batchUpdate方法用于执行批处理相关语句;
- query方法及queryForXXX方法:用于执行查询相关语句;
- call方法:用于执行存储过程、函数相关语句。
测试JdbcTemplate
新建controller
package com.example.springbootfour.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
import java.util.Map;
@RestController
@RequestMapping("/jdbc")
public class JdbcController {
@Autowired
JdbcTemplate jdbcTemplate;
@GetMapping("/selectAll")
public List<Map<String, Object>> selectAll(){
String sql = "SELECT * FROM user";
return jdbcTemplate.queryForList(sql);
}
@GetMapping("/select")
public List<Map<String, Object>> select(@RequestParam("id") int id){
String sql = "SELECT * FROM user WHERE id=?";
return jdbcTemplate.queryForList(sql, id);
}
@GetMapping("/add")
public String add(@RequestParam("name") String name){
String sql = "INSERT INTO user VALUES (DEFAULT,?)";
return "数据变更"+jdbcTemplate.update(sql, name)+"条";
}
@GetMapping("/update")
public String update(@RequestParam("id") int id,@RequestParam("name") String name){
String sql = "UPDATE user SET name=? WHERE id = ?";
return "数据变更"+jdbcTemplate.update(sql, name,id)+"条";
}
@GetMapping("/delete")
public String delete(@RequestParam("id") int id){
String sql = "DELETE FROM user WHERE id = ?";
return "数据变更"+jdbcTemplate.update(sql, id)+"条";
}
}
在数据库配置没有问题的情况下,这个controller可以比较好的展现JdbcTemplate的功能
相关配置:
- 有一个叫jdbczh的数据库
- 其中有一个叫user的表
- user表中的字段为
- id int 主键 自增
- name varchar(64) 非空
集成Druid
简介
Java程序很大一部分要操作数据库,为了提高性能操作数据库的时候,不得不使用数据库连接池。
Druid 是阿里巴巴开源平台上一个数据库连接池实现,结合了 C3P0、DBCP 等 DB 池的优点,同时加入了日志监控,可以很好的监控 DB 池连接和 SQL 的执行情况。
基本配置参数
com.alibaba.druid.pool.DruidDataSource 常见基本配置参数如下:
参数 | 参数含义 |
---|
initialSize | 初始化时建立物理连接的个数。初始化发生在显示调用init方法,或者第一给getConnection时 | minIdle | 最小连接池数量 | maxActive | 最大连接池数量 | maxWait | 获取链接是最大等待时间,单位毫秒。配置了maxWait之后,缺省启用公平锁,并发效率会有所下降,如果需要可以通过配置useUnfairLock属性为true使用非公平锁 | timeBetweenEvictionRunsMillis | 有两个含义: 1)Destroy线程回检测连接的间隔时间,如果连接空闲时间大于等于minEvictableIdleTimeMillis则关闭物理连接;2)testWhileIdle的判断依据,详细看testWhileIdle属性的说明 | minEvictableIdleTimeMillis | 连接保持空闲而不被驱逐的最长时间 | validationQuery | 用于检测连接是否有效的sql,要求是一个查询语句,如果validationQuery为null,testOnBorrow、testOnReturn、testWhileIdle都不会起作用 | testWhileIdle | 建议配置为true,不影响性能,并且保证安全性。申请连接的时候检测,如果空闲时间大于timeBetweenEvictionRunsMillis,执行validationQuery检测连接是否有效 | testOnBorrow | 申请连接时执行validationQuery检测连接是否有效。做了这个配置会降低性能 | testOnReturn | 归还连接时执行validationQuery检测连接是否有效,做了这个配置会降低性能 | poolPreparedStatements | 是否混啊村preparedStatement,也就是PSCache。PSCache对支持游标的数据库性能提升巨大,比如说oracle,在mysql下建议关闭 | filters | 属性类型是字符串,通过别名的方式配置扩展插件,常用的插件有:监控统计用的filter:stat日志用的filter:log4j防御sql注入的filter:wall |
更多可查看druid 参数配置详解 - halberd.lee - 博客园 (cnblogs.com)
配置数据源
- 依赖项导入
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.1.21</version>
</dependency>
- 更改数据源
spring:
datasource:
username: root
password: BIANG0216
url: jdbc:mysql://localhost:3306/jdbczh?serverTimezone=Asia/Shanghai&useUnicode=true&characterEncoding=utf-8
driver-class-name: com.mysql.cj.jdbc.Driver
type: com.alibaba.druid.pool.DruidDataSource
server:
port: 8090
- 数据源切换后使用测试类测试一下

可知数据源已经成功更改
-
那继续写入相关的配置 spring:
datasource:
username: root
password: BIANG0216
url: jdbc:mysql://localhost:3306/jdbczh?serverTimezone=Asia/Shanghai&useUnicode=true&characterEncoding=utf-8
driver-class-name: com.mysql.cj.jdbc.Driver
type: com.alibaba.druid.pool.DruidDataSource
initialSize: 5
minIdle: 5
maxActive: 20
maxWait: 60000
timeBetweenEvictionRunsMillis: 60000
minEvictableIdleTimeMillis: 300000
validationQuery: SELECT 1 FROM DUAL
testWhileIdle: true
testOnBorrow: false
testOnReturn: false
poolPreparedStatements: true
filters: stat,wall,log4j
maxPoolPreparedStatementPerConnectionSize: 20
useGlobalDataSourceStat: true
connectionProperties: druid.stat.mergeSql=true;druid.stat.slowSqlMillis=500
server:
port: 8090
-
导入Log4j依赖
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
-
为 DruidDataSource 绑定全局配置文件中的参数,再添加到容器中:我们需要自己添加DruidDataSource 组件到容器中,并绑定属性 package com.example.springbootfour.config;
import com.alibaba.druid.pool.DruidDataSource;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import javax.sql.DataSource;
@Configuration
public class DruidConfig {
@ConfigurationProperties(prefix = "spring.datasource")
@Bean
public DataSource druidDataSource() {
return new DruidDataSource();
}
}
-
修改测试类并运行 package com.example.springbootfour;
import com.alibaba.druid.pool.DruidDataSource;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import javax.sql.DataSource;
import java.sql.Connection;
import java.sql.SQLException;
@SpringBootTest
class Springboot04ApplicationTests {
@Autowired
DataSource dataSource;
@Test
void contextLoads() throws SQLException {
System.out.println(dataSource.getClass());
Connection connection = dataSource.getConnection();
DruidDataSource druidDataSource = (DruidDataSource) dataSource;
System.out.println("druidDataSource 数据源初始化连接数:" + druidDataSource.getInitialSize());
System.out.println("druidDataSource 数据源最大连接数:" + druidDataSource.getMaxActive());
connection.close();
}
}
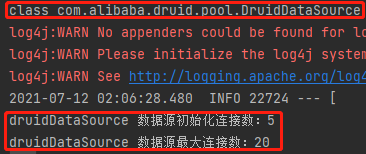
显然是注入成功了,和咱们的值一摸一样,要知道initialSize的默认值是0,而maxActive默认值是8
配置Druid数据源监控
Druid 数据源具有监控的功能,并提供了一个 web 界面方便用户查看,类似安装 路由器 时,人家也提供了一个默认的 web 页面。
要是用这个界面,我们需要配置后台管理页面的一些数据,比方说账号密码、允许登入的ip地址以及不允许登入的ip地址等等
-
在DruidConfig类中添加这么一个方法
@Bean
public ServletRegistrationBean statViewServlet() {
ServletRegistrationBean bean = new ServletRegistrationBean(new StatViewServlet(), "/druid/*");
Map<String, String> initParams = new HashMap<>(4);
initParams.put(ResourceServlet.PARAM_NAME_USERNAME, "admin");
initParams.put(ResourceServlet.PARAM_NAME_PASSWORD, "123456");
initParams.put(ResourceServlet.PARAM_NAME_ALLOW, "");
initParams.put(ResourceServlet.PARAM_NAME_DENY, "192.168.31.202");
bean.setInitParameters(initParams);
return bean;
}
-
运行Application类,进入http://localhost:8090/druid/
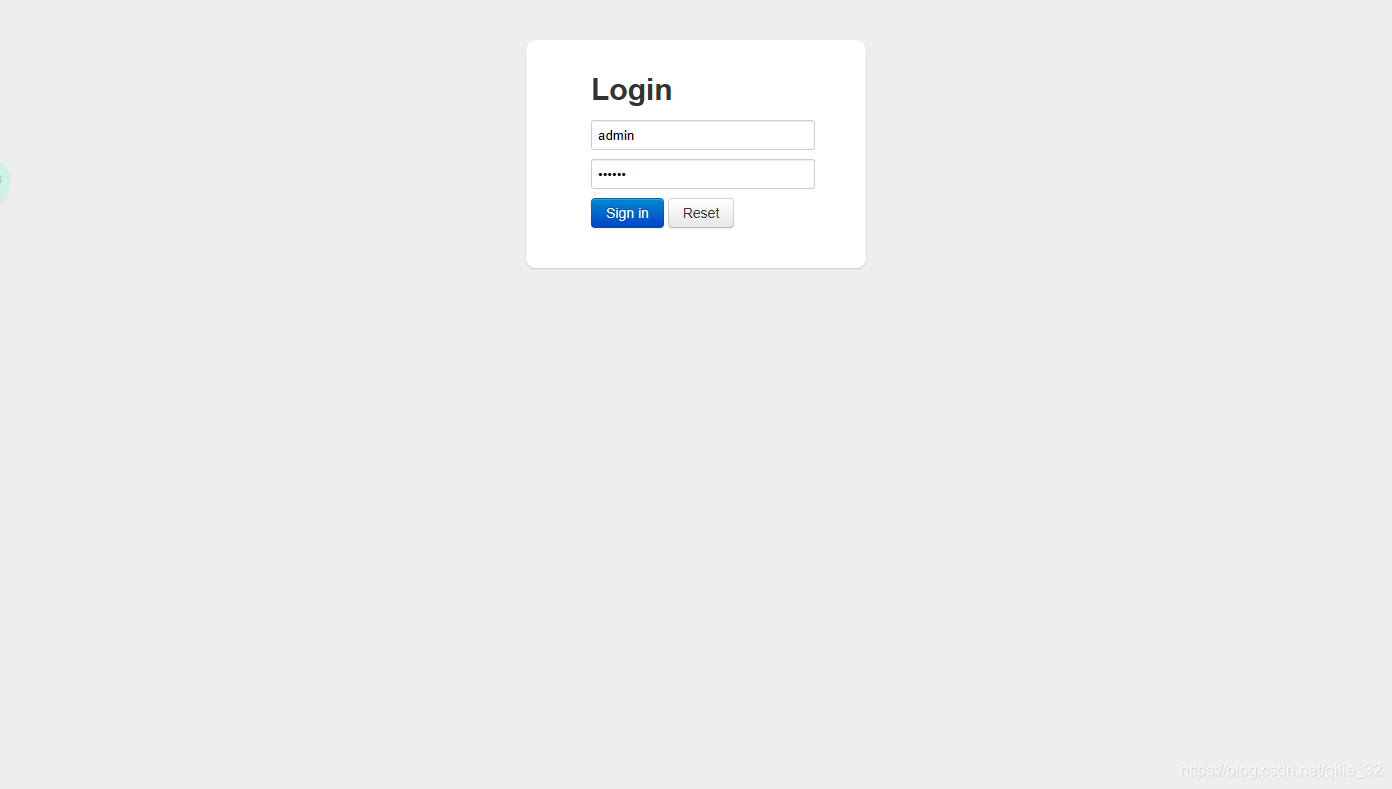
输入statViewServlet中说的username和password,就可以成功登录以及用其中的功能啦
-
如果有过滤的需要,可以在DruidConfig中添加这么一个方法
@Bean
public FilterRegistrationBean webStatFilter() {
FilterRegistrationBean bean = new FilterRegistrationBean();
bean.setFilter(new WebStatFilter());
Map<String, String> initParams = new HashMap<>();
initParams.put(WebStatFilter.PARAM_NAME_EXCLUSIONS, "*.js,*.css,/druid/*,/jdbc/*");
bean.setInitParameters(initParams);
bean.setUrlPatterns(Arrays.asList("/*"));
return bean;
}
具体什么功能可以查看WebStatFilter类的源码或者去网上寻找相应的教程,据说这个用的不会很多
整合MyBatis
官方文档:mybatis – MyBatis 3 | 简介
-
导入依赖 <dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.1</version>
</dependency>
-
测试数据库是否连接成功 -
创建pojo目录以及实体类 这里提到一个很方便的自动生成实体类的方法,使用lombok的各种注解
- @Data 生成 getter、setter
- @NoArgsConstructor 无参构造方法
- @AllArgsConstructor 全部参数构造方法
这么方便的东西自然需要自己导入依赖
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.20</version>
<scope>provided</scope>
</dependency>
那么实体类就这么创建: package com.example.springbootfour.pojo;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
@Data
@NoArgsConstructor
@AllArgsConstructor
public class Pokemon {
private Integer id;
private String name;
}
-
创建mapper目录以及对应的mapper接口 package com.example.springbootfour.mapper;
import com.example.springbootfour.pojo.Pokemon;
import org.apache.ibatis.annotations.Mapper;
import org.springframework.stereotype.Repository;
import java.util.List;
@Mapper
@Repository
public interface PokemonMapper {
List<Pokemon> getAllPokemons();
Pokemon getPokemonById(Integer id );
int insertPokemon(String name);
int updatePokemon(Pokemon pokemon);
int deletePokemon(Integer id );
}
-
在resource下创建一个mybatis文件夹,再在其中创建一个mapper文件夹,再在其中创建一个同名的PokemonMapper.xml <?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.springbootfour.mapper.PokemonMapper">
<select id="getAllPokemons" resultType="Pokemon">
SELECT * FROM pokemon
</select>
<select id="getPokemonById" resultType="Pokemon">
SELECT * FROM pokemon WHERE id = #{id}
</select>
<insert id="insertPokemon" parameterType="String">
INSERT INTO pokemon VALUES (DEFAULT,#{name})
</insert>
<update id="updatePokemon" parameterType="Pokemon">
UPDATE pokemon SET name = #{name} WHERE id = #{id}
</update>
<delete id="deletePokemon" parameterType="int">
DELETE FROM pokemon WHERE id = #{id}
</delete>
</mapper>
-
application.yml添加一些配置 mybatis:
type-aliases-package: com.example.springbootfour.pojo
mapper-locations: classpath:mybatis/mapper/*.xml
-
为这个mapper新建一个controller
PokemonController
package com.example.springbootfour.controller;
import com.example.springbootfour.mapper.PokemonMapper;
import com.example.springbootfour.pojo.Pokemon;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
@RequestMapping("/pokemon")
public class PokemonController {
@Autowired
PokemonMapper pokemonMapper;
@GetMapping("/getAll")
public List<Pokemon> getAllPokemons(){
return pokemonMapper.getAllPokemons();
}
@GetMapping("/get")
public Pokemon getPokemonById(@RequestParam("id") Integer id){
return pokemonMapper.getPokemonById(id);
}
@GetMapping("/insert")
public String insertPokemon(@RequestParam("name") String name){
return "数据变更"+pokemonMapper.insertPokemon(name)+"条";
}
@GetMapping("/update")
public String updatePokemon(@RequestParam("id") Integer id,@RequestParam("name") String name){
return "数据变更"+pokemonMapper.updatePokemon(new Pokemon(id,name))+"条";
}
@GetMapping("/delete")
public String deletePokemon(@RequestParam("id") Integer id){
return "数据变更"+pokemonMapper.deletePokemon(id)+"条";
}
}
-
在数据库配置完成后, 相关数据库配置:
- 新建一个pokemon的表
- pokemon中有两个字段
- id int 主键 自增
- name varchar(64) 非空
运行Application,在浏览器输入对应的各种url,都可以成功运行!
|