Spring集成mybatis的分析
整合的目的:把mybatis框架和spring集成在一起,像一个框架一样使用。 用的技术:ioc 为什么是ioc:能把mybatis和spring集成在一起,像一个框架,是因为ioc能创建对象。可以把mybatis框架中的对象交给spring统一创建,开发人员从spring中获取对象。开发人员就不用同时面对两个或多个框架了,就面对一个spring
mybatis使用步骤分析
- 定义dao接口,StudentDao
- 定义mapper文件 StudentDao.xml
- 定义mybatis的主配置文件 mybatis.xml
- 创建dao的代理对象,StudentDao dao=SqlSession.getMapper(StudentDao.class);
要使用dao对象,需要使用getMapper()方法 怎么能使用getMapper()方法,需要哪些条件
- 获取SqlSession对象,需要使用SqlSessionFactory的openSession()方法
- 创建SqlSessionFactory对象,通过读取mybatis的主配置文件,能创建SqlSessionFactory对象
需要SqlSessionFactory对象,使用Factory能获取SqlSession,有了SqlSession就能有dao,目的就是获取dao对象,SqlSessionFactory创建需要读取配置文件
我们会使用独立的连接池替换mybatis默认自带的,把连接池类也交给spring创建 主配置文件: 1.数据库信息
<environment id="mydev">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/springdb"/>
<property name="username" value="root"/>
<property name="password" value="123456"/>
</dataSource>
<enviroment>
- mapper文件的位置
<mappers>
<mapper resource="com/bjpowernode/dao/StudentDao.xml"/>
</mappers>
============================================================== 通过以上的说明,我们需要让spring创建以下对象 1.独立的连接池类对象,使用ali的druid连接池 2.SqlSessionFactory对象 3.创建出dao对象
整合演示
整合步骤: 1.新建maven项目 2.加入maven的依赖 1、spring依赖 2、mybatis依赖 3、mysql驱动 4、spring的事务的依赖 5、mybatis和spring集成的依赖:mybatis官方提供的,用来在spring项目中创建mybatis的 SqlSessionFactory,dao对象的 3.创建实体类 4.创建dao接口和mapper文件 5.创建mybatis主配置文件 6.创建Service接口和实现类,属性是dao
以上步骤都是mybatis的流程
7.创建spring的配置文件:声明mybatis的对象交给spring创建 1.数据源(使用德鲁伊连接池) 2.SqlSessionFactory 3.Dao对象 4.声明自定义的service 8.创建测试类,获取service对象,通过service调用dao完成数据库的访问
1.新建maven项目:ch08-springconvergemybatis 2.加入maven的依赖如下是pom.xml中的内容:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.yongbin</groupId>
<artifactId>ch08-springconvergemybatis</artifactId>
<version>1.0-SNAPSHOT</version>
<name>ch08-springconvergemybatis</name>
<url>http://www.example.com</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.11</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-tx</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
<dependency> <groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.1</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>1.3.1</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.9</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.1.12</version>
</dependency>
</dependencies>
<build>
<resources>
<resource>
<directory>src/main/java</directory>
<includes>
<include>**/*.properties</include>
<include>**/*.xml</include>
</includes>
<filtering>false</filtering>
</resource>
</resources>
<plugins>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
</build>
</project>
3.创建实体类,包结构如下图: 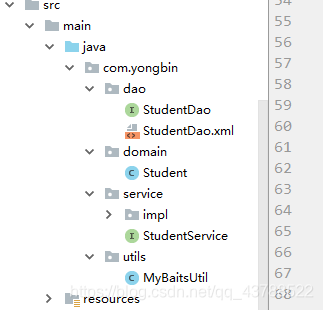 在domain下新建Student的实体类,如下:
public class Student {
private Integer id;
private String name;
private String email;
private Integer age;
public Student() {
}
public Student(Integer id, String name, String email, Integer age) {
this.id = id;
this.name = name;
this.email = email;
this.age = age;
}
@Override
public String toString() {
return "Student{" +
"id=" + id +
", name='" + name + '\'' +
", email='" +
email + '\'' +
", age=" + age +
'}';
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
}
4.创建dao接口和mapper文件 dao接口的声明如下:
public interface StudentDao {
List<Student> selectAllStudents();
int addStudent(Student student);
}
StudentDao.xml(mapper文件)声明如下:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.yongbin.dao.StudentDao">
<select id="selectAllStudents" resultType="com.yongbin.domain.Student">
select * from student
</select>
<insert id="addStudent" >
insert into student VALUE (#{id},#{name},#{email},#{age})
</insert>
</mapper>
5.在src/main/java/resources下面,创建mybatis主配置文件注意的是environments标签不再配置了
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<properties resource="jdbc.properties"></properties>
<settings>
<setting name="logImpl" value="STDOUT_LOGGING"/>
</settings>
<typeAliases>
<package name="com.yongbin.domain"></package>
</typeAliases>
<mappers>
<package name="com.yongbin.dao"/>
</mappers>
</configuration>
6.创建service接口和实现类,里面有一个dao属性
public interface StudentService {
int addStudent(Student student);
List<Student> queryStudents();
}
以上步骤都是mybatis的流程,唯一的改变是mybatis的主配置文件mybatis.xml中去掉了environments标签
7.创建spring的配置文件:声明mybatis的对象交给spring创建 1)数据源(使用德鲁伊连接池) 2)SqlSessionFactory 3)dao对象 4)声明自定义的service对象
在src/main/java/resources下面创建jdbc.properties和applicationContext.xml(spring配置文件) jdbc.properties的内容如下:
jdbc.driverClass=com.mysql.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/mybatis
jdbc.userName=root
jdbc.password=123456
applicationContext.xml(spring配置文件)内容如下:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd">
<context:property-placeholder location="classpath:jdbc.properties"/>
<bean id="myDataSource" class="com.alibaba.druid.pool.DruidDataSource">
<property name="driverClassName" value="${jdbc.driverClass}"></property>
<property name="url" value="${jdbc.url}"></property>
<property name="username" value="${jdbc.userName}"></property>
<property name="password" value="${jdbc.password}"></property>
<property name="maxActive" value="20"></property>
</bean>
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="myDataSource"></property>
<property name="configLocation" value="classpath:mybatis.xml"></property>
</bean>
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="sqlSessionFactoryBeanName" value="sqlSessionFactory"></property>
<property name="basePackage" value="com.yongbin.dao"></property>
</bean>
<bean id="studentService" class="com.yongbin.service.impl.StudentServiceImpl">
<property name="studentDao" ref="studentDao"></property>
</bean>
</beans>
至此,整合完毕了,下面测试。 在src/test/java/下面新建一个TestSprintConvergeMybatis的类,声明一个查看容器中所有对象的方法,如下:
@Test
public void test01(){
String config="applicationContext.xml";
ApplicationContext context=new ClassPathXmlApplicationContext(config);
String[] names = context.getBeanDefinitionNames();
for (int i = 0; i < names.length; i++) {
System.out.println("容器中对象的名称"+names[i]+":"+context.getBean(names[i]));
}
}
运行如下: 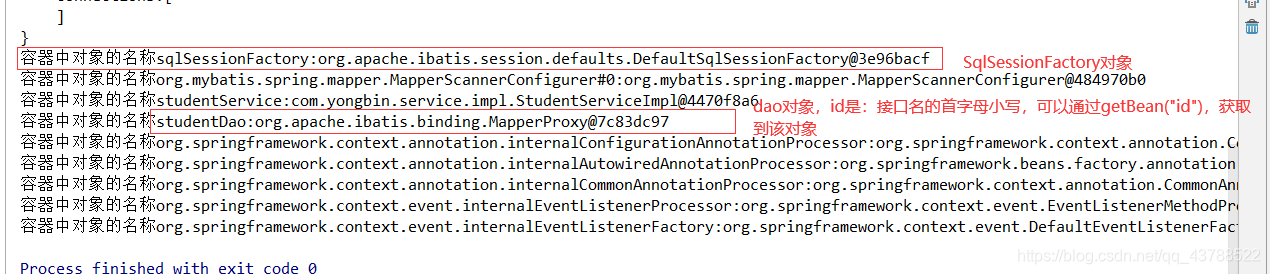 下面创建StudentServiceImpl来实现StudentService接口,内容如下:
public class StudentServiceImpl implements StudentService {
private StudentDao studentDao;
public void setStudentDao(StudentDao studentDao){
this.studentDao=studentDao;
}
@Override
public int addStudent(Student student) {
int nums = studentDao.addStudent(student);
return nums;
}
@Override
public List<Student> queryStudents() {
List<Student> stuList = studentDao.selectAllStudents();
return stuList;
}
}
由于我们在spring的配置文件里已经使用bean标签创建了service对象,且通过set方式注入了dao对象,所以下面是通过service来测试方法。 测试查询操作和插入操作的代码如下,代码都能够正常运行。
public class StudentServiceImpl implements StudentService {
private StudentDao studentDao;
public void setStudentDao(StudentDao studentDao){
this.studentDao=studentDao;
}
@Override
public int addStudent(Student student) {
int nums = studentDao.addStudent(student);
return nums;
}
@Override
public List<Student> queryStudents() {
List<Student> stuList = studentDao.selectAllStudents();
return stuList;
}
}
使用idea保存spring集成mybatis的applicationContext配置文件
通过配置模板保存一下applicationContext文件,可以供自己下次使用,下次在使用的时候改改就行。 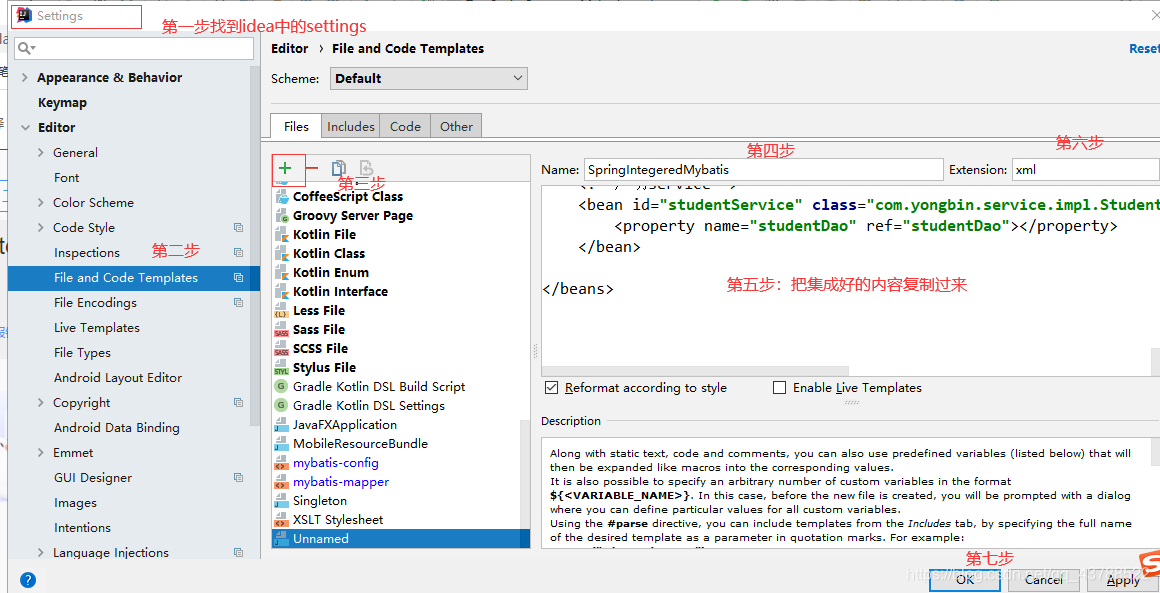
|