暑假在家无聊,提前学习一下下学期的内容,java,第一天,从最基本的循环结构开始,实现九九乘法表。
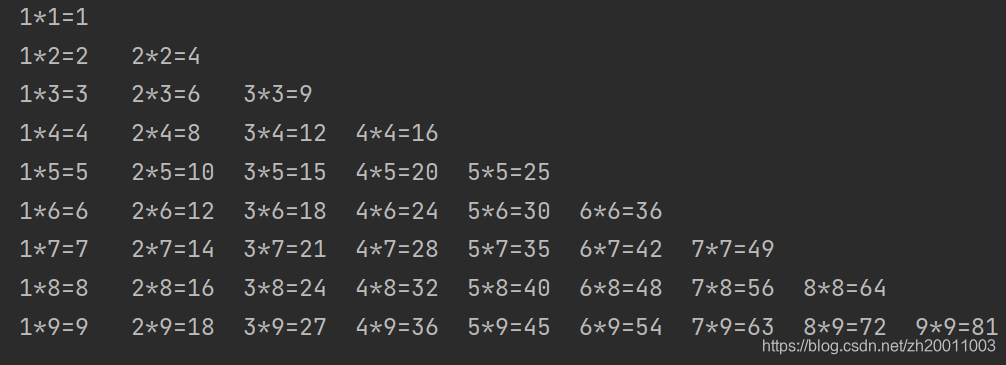
1)for循环
?
public class practive {
public static void main(String[] args) {
int i,j;
//i控制行数,j控制列数
for(i=1;i<=9;i++)
{
for(j=1;j<=i;j++)
System.out.print(j+"*"+i+"="+(i*j)+"\t");
System.out.println();//用于换行
}
}
}
?
2)while循环
public class practive2 {
public static void main(String[] args) {
int x=1;
//x控制行数,y控制列数
while (x<=9)
{
int y=1;
while(y<=x)
{
System.out.print(y+"*"+x+"="+(x*y)+"\t");
y++;
}
x++;
System.out.println();//用于换行
}
}
}
3)do...while循环?
public class practive3 {
public static void main(String[] args) {
int m=1;
do
{
//m控制行数,n控制列数
int n=1;
do {
System.out.print(n+"*"+m+"="+(m*n)+"\t");
n++;
}while(n<=m);
System.out.println();//用于换行
m++;
}while(m<=9);
}
}
总结:for循环相对于另外两种循环,具有一定的简洁性,代码比较简单。在很多时候,三种循环相辅相成,都有各自的优点。
源代码:
import java.util.Scanner;
public class practive4 {
public static void main(String[] args) {
System.out.println("请选择打印9*9乘法表格的方式");
System.out.println("1]for循环 2]do while循环 3]while in循环");
Scanner sc=new Scanner(System.in);
int a=sc.nextInt();
if(a==1)
{
int i,j;
for(i=1;i<=9;i++)
{
for(j=1;j<=i;j++)
System.out.print(j+"*"+i+"="+(i*j)+"\t");
System.out.println();
}
}
else if(a==2) {
int m = 1;
do {
int n = 1;
do {
System.out.print(n + "*" + m + "=" + (m * n) + "\t");
n++;
} while (n <= m);
System.out.println();
m++;
} while (m <= 9);
}
else if(a==3)
{
int x=1;
while (x<=9)
{
int y=1;
while(y<=x)
{
System.out.print(y+"*"+x+"="+(x*y)+"\t");
y++;
}
x++;
System.out.println();
}
}
else System.out.println("error");
}
}
|