异常
1、自定义异常
public class Test {
public static void main(String[] args) {
try{
int ret = divide(10, 0);
}catch (ArithmeticException e){
e.printStackTrace();
}
}
public static int divide(int x ,int y){
if(y == 0){
throw new ArithmeticException("此处抛出一个算术异常");
}
return x / y;
}
}
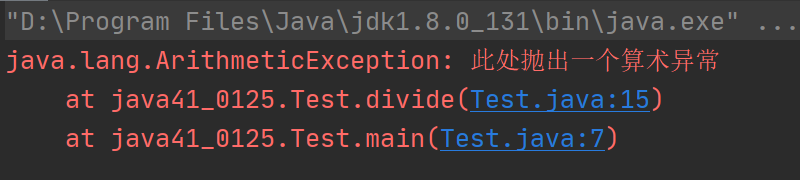
2、throw 和 throws
2.1 throw
主动抛出异常,被抛出的异常其实就是一个对象。
2.2 throws
标注当前的方法,可能抛出啥样的异常。
public class Test {
public static void main(String[] args) {
try{
int ret = divide(10, 0);
}catch (ArithmeticException e){
e.printStackTrace();
}
}
public static int divide(int x ,int y) throws ArithmeticException{
if(y == 0){
throw new ArithmeticException("此处抛出一个算术异常");
}
return x / y;
}
}
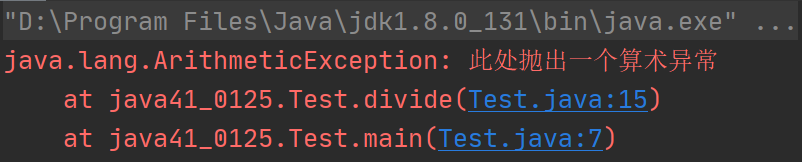
3、finally 注意事项
public class Test {
public static void main(String[] args) {
System.out.println(func());
}
public static int func(){
try{
return 10;
}finally {
{
return 20;
}
}
}
}
 finally 执行是在 try 中 return 10 返回之前,又因为 finally 中已经存在 return 20 ,所以最后运行结果为 20.
4、java 中的异常体系
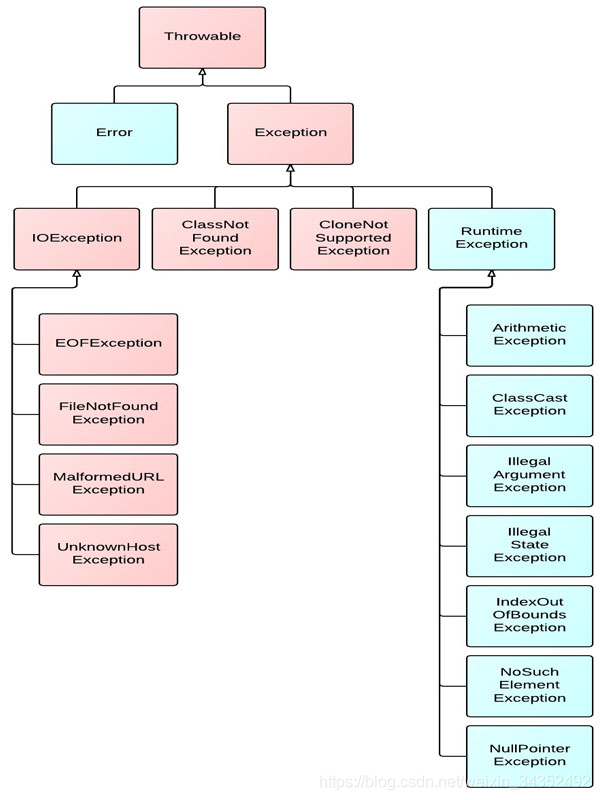
Error:系统级别的异常。JVM 内部使用的。 Exception:应用级别的异常(开发者常用) 橙色框图:受查异常–若某方法中抛出此异常,必须对此异常显示处理。
- 显示处理方式
1、使用 try catch
2、使用 throws 声明可能会抛出此异常
蓝色框图:非受查异常–可不显示处理。
5、自定义异常类
import java.util.Scanner;
public class Login {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入用户名: ");
String name = scanner.next();
System.out.println("请输入密码: ");
String password = scanner.next();
try {
login(name,password);
} catch (NameException | PasswordException e) {
e.printStackTrace();
}
}
public static void login(String name,String password) throws NameException,
PasswordException {
if (!name.equals("admin")){
throw new NameException("用户名错误");
}
if(!password.equals("123456")){
throw new PasswordException("密码错误");
}
}
}
public class PasswordException extends Exception{
public PasswordException (String message){
super(message);
}
}
public class NameException extends Exception{
public NameException(String message) {
super(message);
}
}
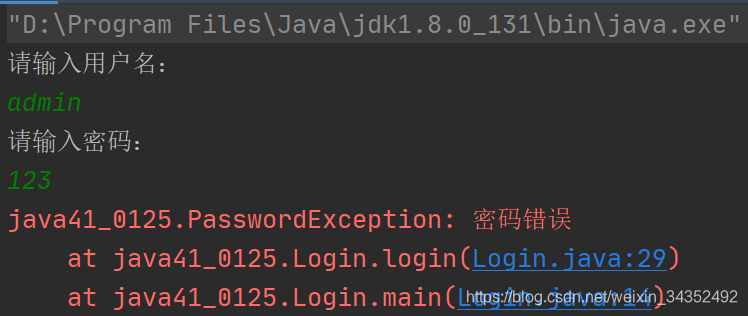 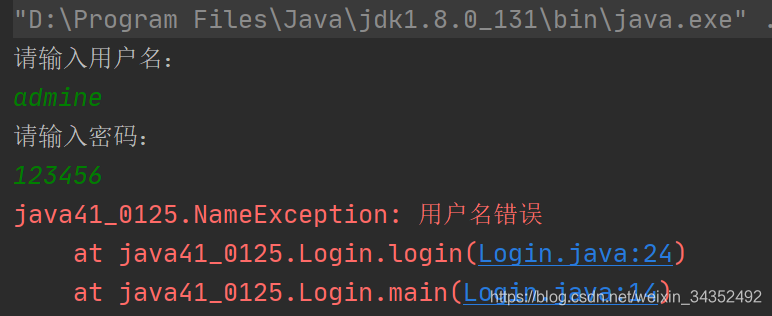
|